Mastering Kotlin Flows: Simplifying Reactive Programming in Android
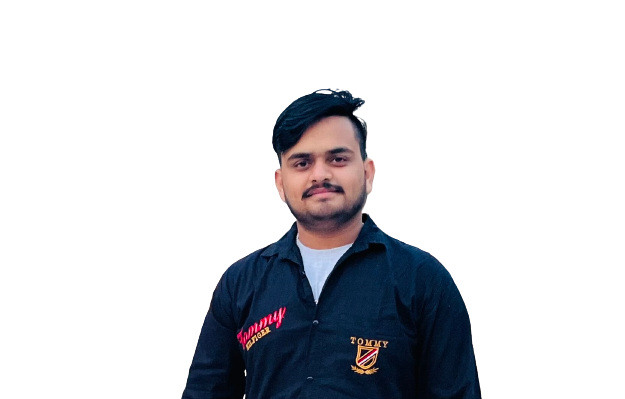
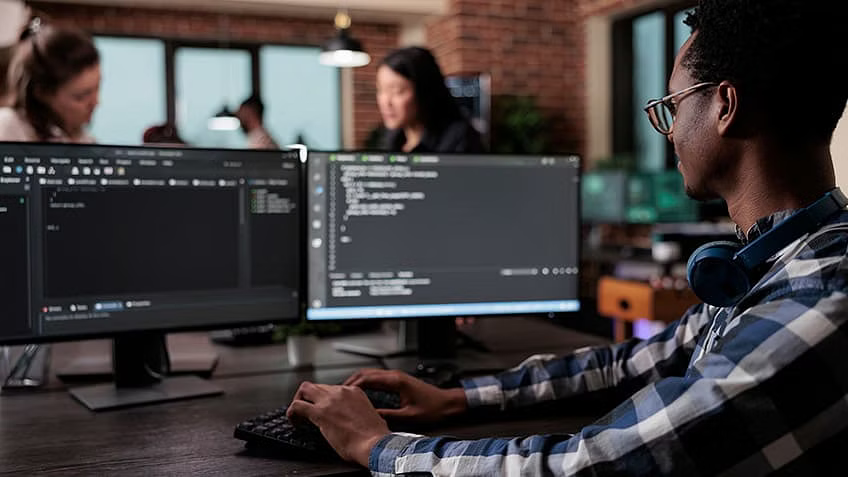
In modern Android development, handling data streams and asynchronous tasks is crucial, especially when dealing with real-time data updates, network requests, and UI changes. Kotlin Flow, part of Kotlin Coroutines, simplifies reactive programming and helps developers manage asynchronous data flows in a clean and structured way.
In this blog, we’ll dive into Kotlin Flow, understand its core concepts, and walk through simple examples to get you started.
What is Kotlin Flow?
Kotlin Flow is a reactive stream API that allows data to be emitted sequentially over time. Unlike traditional callbacks or RxJava, Flow is a type-safe, lightweight, and straightforward solution for handling asynchronous streams in Kotlin.
Flow can be seen as a pipeline that emits values over time, allowing you to handle these emissions in a clean, reactive manner. This is particularly useful when you want to observe changes in data, like monitoring user inputs, network requests, or database updates.
Why Use Kotlin Flow?
Simpler than RxJava: Kotlin Flow is much easier to understand and doesn’t require additional libraries.
Lightweight: Flows are built on top of Coroutines, making them more efficient.
Structured Concurrency: Flow is well-integrated with coroutines, providing structured concurrency and better cancellation support.
Key Components of Kotlin Flow
Flow: Represents the data stream that emits values over time.
Collector: Receives and processes the values emitted by Flow.
Operators: Functions like
map
,filter
, andcollect
that transform and handle data within the Flow.
How to Create and Use a Flow
Let’s break it down with an example:
import kotlinx.coroutines.*
import kotlinx.coroutines.flow.*
fun main() = runBlocking {
val numberFlow = flow {
for (i in 1..5) {
delay(1000) // Simulate some work
emit(i) // Emit each number
}
}
numberFlow.collect { value ->
println("Received number: $value")
}
}
Explanation:
Creating the Flow: We create a
flow
that emits numbers from 1 to 5 with a delay of 1 second.Emitting Values: The
emit()
function is used to send values from the Flow.Collecting Values: We use
collect { }
to handle the emitted values. Each number is printed when received.
Using Operators in Flow
Flow comes with a wide range of operators that help in transforming, filtering, and combining data streams.
Example: Using map
and filter
val transformedFlow = numberFlow
.map { it * 2 } // Multiply each number by 2
.filter { it % 2 == 0 } // Only emit even numbers
transformedFlow.collect { value ->
println("Transformed value: $value")
}
Here, we used the map
operator to multiply each emitted number by 2, and then the filter
operator to only emit even numbers.
Practical Use Case: Real-Time Data Updates
Imagine you are building a weather app that fetches the temperature every few seconds and updates the UI.
fun fetchTemperatureFlow(): Flow<Int> = flow {
while (true) {
val temperature = (20..30).random() // Simulate temperature updates
emit(temperature)
delay(5000) // Update every 5 seconds
}
}
fun main() = runBlocking {
fetchTemperatureFlow().collect { temperature ->
println("Current temperature: $temperature°C")
}
}
In this example:
We simulate temperature updates using a Flow that emits a random temperature between 20°C and 30°C every 5 seconds.
The
collect
function is used to update the UI (or in this case, print the temperature).
Flow in Android: ViewModel Example
In Android, Flows are commonly used to handle data streams within a ViewModel. Let’s say we have a ViewModel that fetches data from a repository and observes changes.
class WeatherViewModel : ViewModel() {
private val _temperatureFlow = MutableStateFlow(0) // Initial temperature
val temperatureFlow: StateFlow<Int> = _temperatureFlow
fun updateTemperature() {
viewModelScope.launch {
flow {
while (true) {
emit((20..30).random())
delay(5000)
}
}.collect { temperature ->
_temperatureFlow.value = temperature
}
}
}
}
In this ViewModel:
We create a
MutableStateFlow
to hold the current temperature.The
updateTemperature()
function fetches temperature updates every 5 seconds.We update the
_temperatureFlow
with the new value, which can be observed in the UI.
Conclusion
Kotlin Flow is a powerful tool that simplifies reactive programming in Android. By understanding the basics of Flow, operators, and how to collect data, you can handle asynchronous streams more efficiently. Whether you’re fetching data from a network, observing database changes, or processing real-time user inputs, Kotlin Flow provides a clean and structured way to manage these tasks.
Give it a try in your next project, and you’ll see how it helps simplify your code!
Feel free to ask any questions in the comments or share your thoughts on how Kotlin Flow has improved your Android development experience!
Subscribe to my newsletter
Read articles from Mukesh Rajput directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
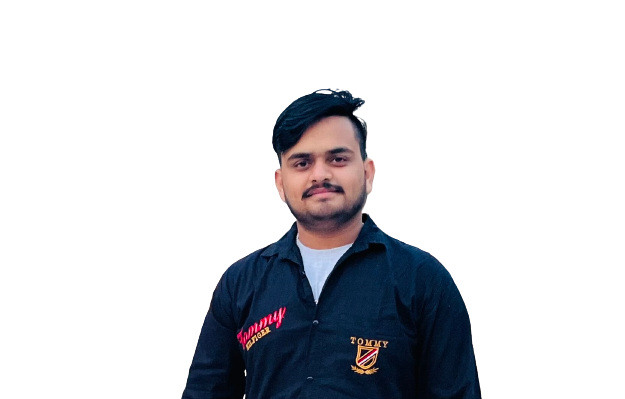
Mukesh Rajput
Mukesh Rajput
Specializing in creating scalable and maintainable applications using MVVM and Clean Architecture principles. With expertise in Ktor, Retrofit, RxJava, View Binding, Data Binding, Hilt, Koin, Coroutines, Room, Realm, and Firebase, I am committed to delivering high-quality mobile solutions that provide seamless user experiences. I thrive on new challenges and am constantly seeking opportunities to innovate in the Android development space.