Understanding the Git Workflow: Commits, Branching, and Merging

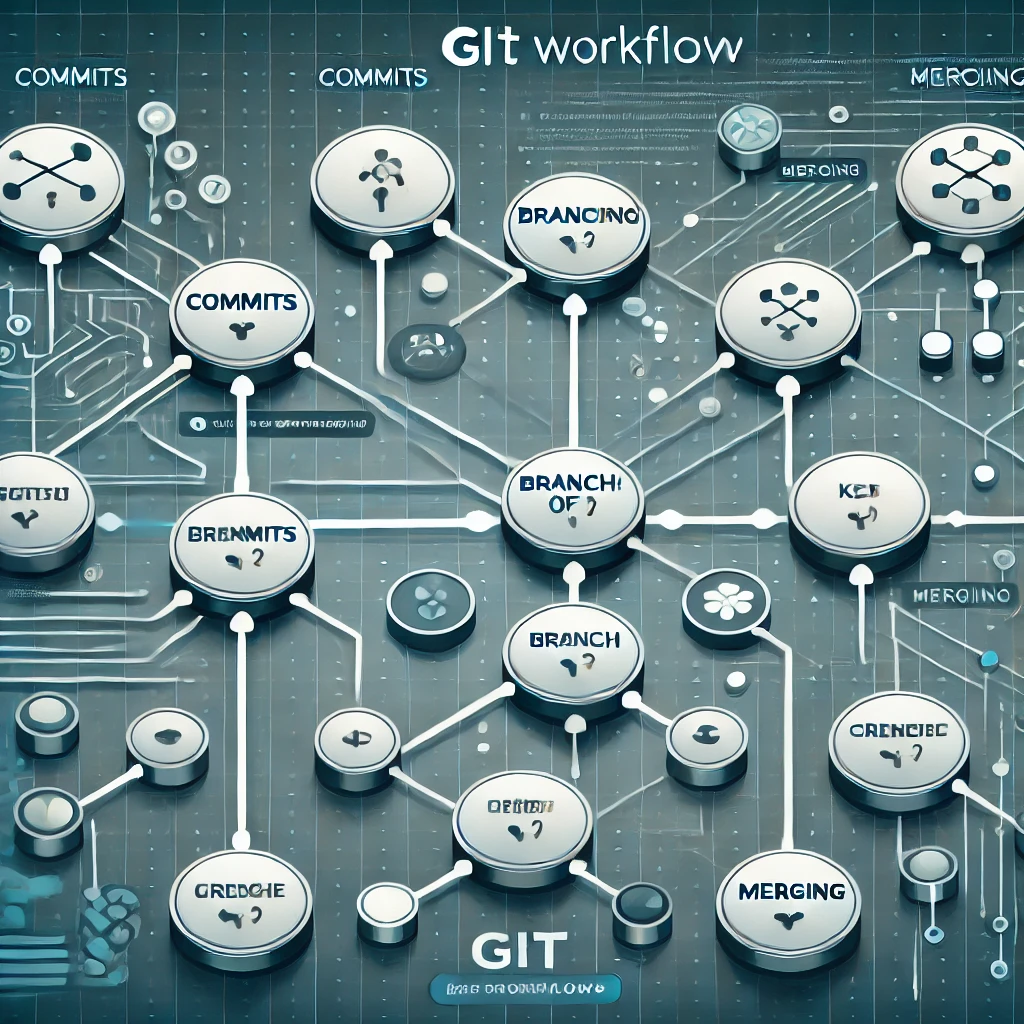
Git is a powerful version control system that allows developers to manage and track changes in their codebase effectively. In this blog post, we'll dive into the core Git workflow, focusing on commits, branching, and merging. Whether you're new to Git or looking to refine your understanding, this guide will help you navigate the essential Git operations with confidence.
The Git Commit Workflow
At the heart of Git's functionality is the commit. A commit represents a snapshot of your project at a specific point in time. Here's the typical workflow for creating a commit:
Make changes: Edit, add, or delete files in your working directory.
Stage changes: Use
git add
to stage the changes you want to include in your commit.Commit changes: Use
git commit
to create a new commit with your staged changes.
Let's look at this process in more detail:
# Edit files in your project
# Stage all changes
git add .
# Or stage specific files
git add file1.txt file2.py
# Create a commit
git commit -m "Add new feature: user authentication"
Best practices for commits:
Make small, focused commits that encompass a single logical change.
Write clear, descriptive commit messages that explain the "why" behind the change.
Commit often to create a detailed history of your project's evolution.
The Git Branching Workflow
Branching in Git allows you to diverge from the main line of development and work on different features or experiments independently. Here's a typical branching workflow:
Create a branch: Start a new line of development.
Work on the branch: Make commits to your new branch.
Finish work: Complete the feature or experiment.
Merge or rebase: Incorporate your changes back into the main branch.
Here's how this looks in practice:
# Create and switch to a new branch
git checkout -b feature-login
# Work on your feature, making commits as you go
git add .
git commit -m "Implement login form"
git commit -m "Add password validation"
# When ready, switch back to the main branch
git checkout main
# Merge your feature branch
git merge feature-login
Popular branching strategies:
Feature branching: Create a branch for each new feature or bug fix.
Git Flow: A more structured approach with dedicated branches for features, releases, and hotfixes.
GitHub Flow: A simpler workflow centered around the main branch and feature branches.
The Git Merging Workflow
Merging is the process of combining the history of two or more branches. Here's a typical merging workflow:
Complete work on your feature branch: Finish and test your changes.
Update your main branch: Switch to main and pull the latest changes.
Merge the feature branch: Combine your feature branch into main.
Resolve conflicts: If there are conflicting changes, resolve them manually.
Push the merged changes: Update the remote repository with your merge.
Here's an example of this workflow:
# Ensure your feature branch is up-to-date
git checkout feature-awesome
git pull origin feature-awesome
# Switch to main and update it
git checkout main
git pull origin main
# Merge the feature branch
git merge feature-awesome
# If there are conflicts, resolve them and then:
git add .
git commit -m "Merge feature-awesome into main"
# Push the merged changes
git push origin main
Handling merge conflicts:
Git will mark conflicting areas in your files.
Open the conflicting files and manually resolve the conflicts.
Use
git add
to mark the conflicts as resolved.Complete the merge with
git commit
.
Advanced Git Workflows
As you become more comfortable with Git, you might explore more advanced workflows:
Rebasing
Rebasing is an alternative to merging that can create a cleaner project history:
git checkout feature-branch
git rebase main
Cherry-picking
Cherry-picking allows you to apply specific commits from one branch to another:
git cherry-pick <commit-hash>
Interactive Rebasing
Interactive rebasing allows you to modify a series of commits before moving them to a new base:
git rebase -i HEAD~3
Conclusion
Understanding the Git workflow for commits, branching, and merging is crucial for effective collaboration and version control. By mastering these concepts, you'll be able to manage your projects more efficiently, collaborate seamlessly with others, and maintain a clean and meaningful project history.
Remember, the key to becoming proficient with Git is practice. Don't be afraid to experiment in a test repository to gain confidence with these workflows. Happy coding!
Subscribe to my newsletter
Read articles from Md Saif Zaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
