Understanding Zod: A TypeScript-first Schema Declaration and Validation Library
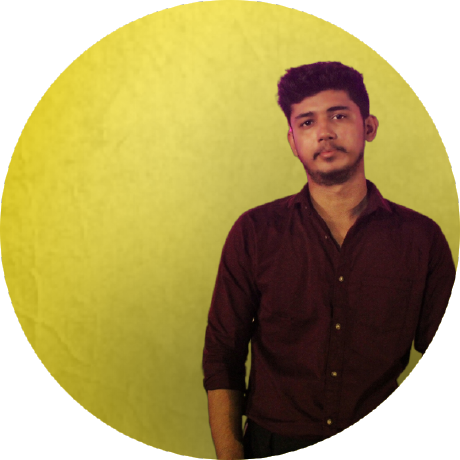
In modern web development, especially when working with TypeScript, ensuring that data structures conform to expected types and formats is crucial. Whether it’s form validation, API responses, or ensuring the integrity of data throughout your app, reliable validation can prevent a wide range of bugs. That's where Zod comes into play.
What is Zod?
Zod is a TypeScript-first schema declaration and validation library. Unlike other libraries like Yup or Joi, which also handle validation, Zod is tightly integrated with TypeScript, allowing you to declare schemas that mirror your TypeScript types. It enables a more seamless development experience by ensuring that your data is validated while keeping your TypeScript types consistent.
Why Use Zod?
Zod stands out for several reasons:
TypeScript-first: Zod is designed with TypeScript in mind. It generates TypeScript types from your schemas, ensuring type safety.
Simplicity: Its API is easy to learn and intuitive, making it ideal for both simple and complex validation requirements.
Zero dependencies: Zod is lightweight and doesn’t rely on any external dependencies, keeping your project’s footprint minimal.
1. Defining a Schema
At its core, Zod allows you to define schemas that represent the shape and type of your data. Here's an example:
import { z } from 'zod';
const userSchema = z.object({
name: z.string(),
age: z.number(),
email: z.string().email(),
});
In the example above, we define a schema for a user object with name
, age
, and email
fields. Zod ensures that any data we validate against this schema adheres to these types and formats.
2. Validating Data
Once a schema is defined, Zod allows you to validate data using the .parse()
method:
const validUser = {
name: "Shivam",
age: 25,
email: "shivam@example.com",
};
userSchema.parse(validUser); // No errors
const invalidUser = {
name: "Shivam",
age: "not a number",
email: "invalidemail.com",
};
userSchema.parse(invalidUser); // Throws a validation error
If the data is valid, parse()
will return the validated object. If it's invalid, Zod will throw a detailed error describing what went wrong.
3. Type Inference
One of Zod's superpowers is its ability to infer TypeScript types directly from your schema. This means you don’t have to manually define types for your data:
type User = z.infer<typeof userSchema>;
4. Transformations
Zod allows you to transform data while validating it. This is useful for normalizing input:
const schema = z.string().transform((val) => val.toUpperCase());
const result = schema.parse("hello");
console.log(result); // "HELLO"
5. Asynchronous Validations
For cases where validations are asynchronous (like checking a username's availability from an API), Zod provides .parseAsync()
:
const asyncSchema = z.string().refine(async (val) => {
const isAvailable = await checkUsernameAvailability(val);
return isAvailable;
});
asyncSchema.parseAsync("username").then((result) => {
console.log(result);
});
6. Extending Schemas
Zod allows you to extend existing schemas, making it easy to reuse and modify them as needed:
const baseUserSchema = z.object({
name: z.string(),
age: z.number(),
});
const extendedUserSchema = baseUserSchema.extend({
email: z.string().email(),
});
7. Handling Optional and Default Values
Zod also supports optional and default values in schemas:
const optionalSchema = z.object({
name: z.string(),
age: z.number().optional(),
});
const defaultSchema = z.object({
name: z.string(),
age: z.number().default(30),
});
Conclusion
Zod is a powerful and lightweight tool for schema declaration and validation, especially when working with TypeScript. Its simplicity, type inference, and ability to transform data on the fly make it a go-to library for ensuring the integrity of your data throughout your application.
If you're looking for a TypeScript-first approach to validation with minimal boilerplate, give Zod a try!
Subscribe to my newsletter
Read articles from Shivam barthwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
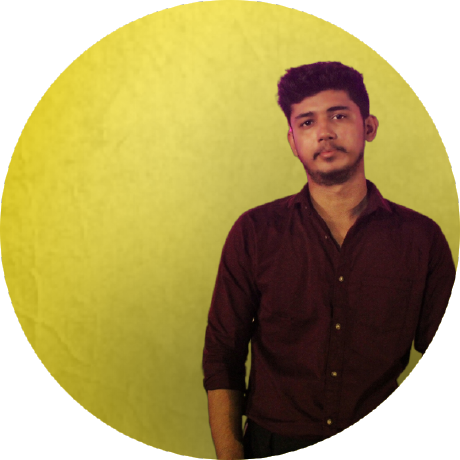