The Importance of Component Reusability in React: How to Write DRY Code
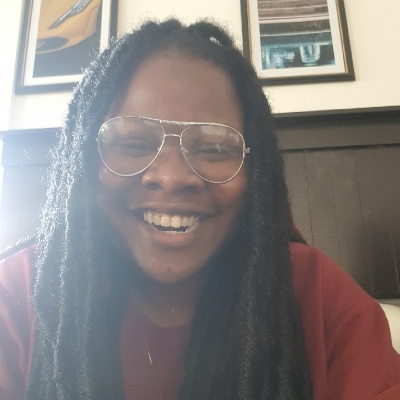
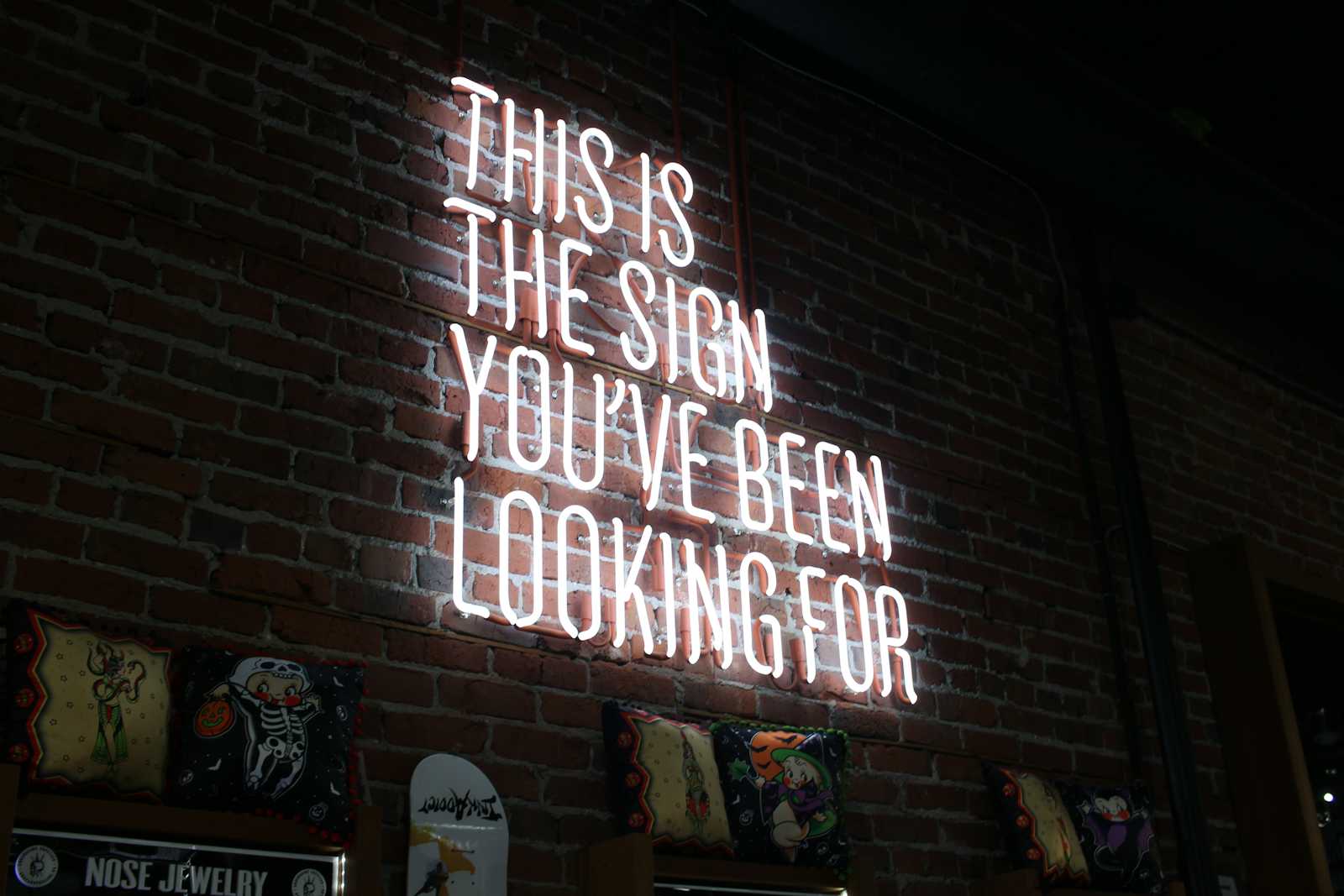
In software development, writing clean, maintainable, and scalable code is important. One principle that plays a crucial role in achieving this is DRY—Don't Repeat Yourself. DRY encourages reducing repetition in code by centralizing logic and components, making it easier to maintain, extend, and debug.
In React, component reusability is a direct application of the DRY principle. Instead of duplicating code across different parts of your application, React allows you to create reusable components that can be utilized throughout your project. This approach simplifies development and ensures consistency across the user interface.
Why Component Reusability Matters
Reduces Redundancy: Repetitive code can be hard to manage in large applications. By creating reusable components, you minimize the duplication of code. This also reduces inconsistencies throughout the codebase.
Improves Maintainability: Reusable components allow you to make updates in one place, which reflects across all instances of the component. If you need to tweak the design or functionality, you can do so without modifying every instance.
Consistency Across the Applications: Reusable components ensure that the design and functionality are consistent across different parts of the app. For example, a reusable button component ensures all buttons across the app maintain the same look and behavior, contributing to a cohesive user experience.
Speeds Up Development: With Reusable Components, developers can build new features more quickly. Instead of writing everything from scratch, you can simply import and configure pre-built components.
Improved Scalability: As your application grows, so does its complexity. By following the DRY principle and using reusable components, you can easily extend functionality without worrying about breaking parts of your app. Reusing a single component in different places also helps improve performance since React optimizes updates.
How to Write DRY Code in React
Identify Reusable Patterns:
During development, identify patterns in your UI that are repetitive. For example, a form field, button, or card component might be repeated across various pages. Once you notice such patterns, abstract them into individual components.
const Button = ({ label, onClick, type = 'button' }) => ( <button type={type} onClick={onClick}> {label} </button> );
In this example, the Button component is generalized and can be reused wherever a button is needed.
Use Props for Dynamic Data:
React’s props allow you to pass data to components, making them flexible and dynamic. Instead of hardcoding values, use props to make your component adaptable. For example, you can pass different text labels, styles, or actions to a button component.
<Button label="Submit" onClick={handleSubmit} /> <Button label="Cancel" onClick={handleCancel} />
Component Dependency:
A reusable component should be independent of its context. Avoid embedding business logic directly within components. Instead, keep your components pure by focusing only on rendering UI. Let your parent components handle the logic and pass it as props. E.g Instead of writing the
handleSubmit/handleCancel
functions in theButton
component, we write them in their parent component and pass them as props.Keep Components Small and Focused:
Smaller components are easier to reuse. If a component is trying to handle too many responsibilities, it becomes difficult to reuse. Aim to build components that focus on a single task. For example, create a TextInput component to handle just input fields instead of mixing it with form validation or submission logic.
Conclusion
In React, writing DRY code goes hand-in-hand with creating reusable components. By doing so, you not only minimize code duplication but also enhance maintainability, scalability, and development speed. Identifying reusable patterns, leveraging props, and keeping components small and focused are essential strategies for building robust React applications.
By mastering component reusability, you’ll become more efficient as a developer, and your projects will be easier to maintain in the long run. Keep looking for opportunities to refactor and simplify your code as your project evolves, and when you start a new project, think DRY.
Subscribe to my newsletter
Read articles from Chineta Adinnu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
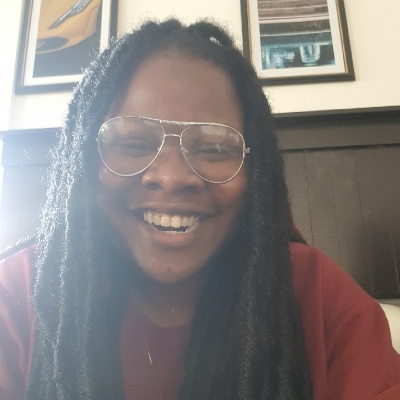
Chineta Adinnu
Chineta Adinnu
Hi, I’m Chineta Adinnu! I’m a frontend developer with a passion for creating dynamic and user-friendly web experiences. On this blog, I share my journey in frontend development, from learning new technologies to implementing them in real projects. I dive into frameworks like React and Next.js, explore state management with Redux Toolkit, and offer practical tips based on my hands-on experience. My goal is to provide valuable insights and tutorials that can help others navigate the ever-evolving world of web development. Join me as I document my learning process, share challenges and solutions, and offer guidance on the latest frontend technologies. If you’re interested in tech or looking for practical advice, you’re in the right place! Feel free to connect if you have questions or want to discuss tech! Check out some of my articles on Medium: https://medium.com/@chinetaadinnu."