Understanding the os Package in Go Programming Language

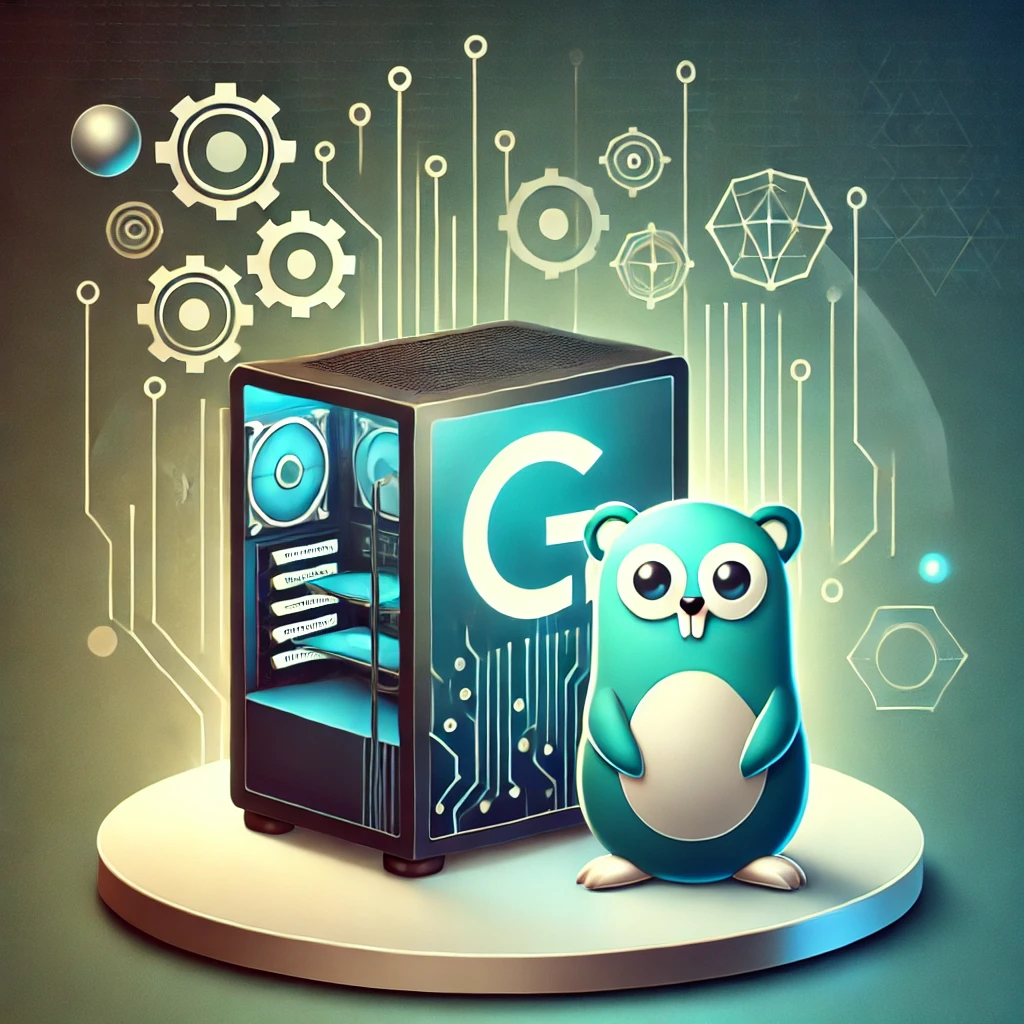
Welcome! Now that you've grasped the basics of Go, let's delve deeper into one of its most essential packages: the os
package. This guide will provide an in-depth exploration of the os
package, covering everything from file operations to process management. Let's get started!
Table of Contents
Introduction to the os
Package
The os
package in Go provides a platform-independent interface to operating system functionality. It allows you to perform:
File and directory operations
Process and signal handling
Environment variable manipulation
File permission and ownership management
Interactions with the underlying file system
The os
package abstracts differences between operating systems, enabling you to write code that works across Windows, macOS, Linux, and other supported platforms.
Importing the os
Package
To use the os
package, import it in your Go program:
import "os"
Optionally, you may import other related packages like fmt
, io
, or path/filepath
as needed.
Working with Files
Opening and Creating Files
Opening Existing Files
Use os.Open
()
to open an existing file for reading.
file, err := os.Open("example.txt")
if err != nil {
// Handle error
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
Creating or Truncating Files
Use os.Create()
to create a new file or truncate an existing one.
file, err := os.Create("newfile.txt")
if err != nil {
// Handle error
fmt.Println("Error creating file:", err)
return
}
defer file.Close()
Opening Files with Specific Flags and Permissions
Use os.OpenFile()
to open a file with specific flags and permissions.
file, err := os.OpenFile("example.txt", os.O_RDWR|os.O_CREATE, 0755)
if err != nil {
// Handle error
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
Flags:
os.O_RDONLY
: Open the file read-only.os.O_WRONLY
: Open the file write-only.os.O_RDWR
: Open the file read-write.os.O_APPEND
: Append data to the file when writing.os.O_CREATE
: Create a new file if none exists.os.O_TRUNC
: Truncate file when opened.
Permissions:
- File mode bits (e.g.,
0644
,0755
) specify the file's permission and mode bits.
Reading and Writing Files
Reading from Files
Use methods from the io
package or os.File
methods to read data.
Using Read()
method:
buffer := make([]byte, 100)
bytesRead, err := file.Read(buffer)
if err != nil {
// Handle error
fmt.Println("Error reading file:", err)
return
}
fmt.Printf("Read %d bytes: %s\n", bytesRead, string(buffer[:bytesRead]))
Using bufio
package:
import (
"bufio"
// ...
)
scanner := bufio.NewScanner(file)
for scanner.Scan() {
fmt.Println(scanner.Text())
}
if err := scanner.Err(); err != nil {
fmt.Println("Error scanning file:", err)
}
Writing to Files
Use Write()
or WriteString()
methods.
data := []byte("Hello, World!\n")
bytesWritten, err := file.Write(data)
if err != nil {
// Handle error
fmt.Println("Error writing to file:", err)
return
}
fmt.Printf("Wrote %d bytes to file.\n", bytesWritten)
Using fmt.Fprintf()
:
import "fmt"
fmt.Fprintf(file, "Formatted number: %d\n", 42)
Closing Files
Always close files when done to release resources.
defer file.Close()
Using defer
ensures that the file is closed when the function exits, even if an error occurs.
File Permissions and Modes
File permissions are specified using Unix-style permission bits.
Common Permission Bits:
0644
: Owner can read and write; others can read.0755
: Owner can read, write, and execute; others can read and execute.
Example:
file, err := os.OpenFile("script.sh", os.O_CREATE|os.O_WRONLY, 0755)
Working with Directories
Creating Directories
Create a Single Directory
Use os.Mkdir()
to create a single directory.
err := os.Mkdir("testdir", 0755)
if err != nil {
fmt.Println("Error creating directory:", err)
return
}
Create Nested Directories
Use os.MkdirAll()
to create nested directories.
err := os.MkdirAll("parent/child/grandchild", 0755)
if err != nil {
fmt.Println("Error creating directories:", err)
return
}
Listing Directory Contents
Use os.ReadDir()
(Go 1.16 and later) or ioutil.ReadDir()
(deprecated in Go 1.16) to list directory contents.
Using os.ReadDir()
:
entries, err := os.ReadDir(".")
if err != nil {
fmt.Println("Error reading directory:", err)
return
}
for _, entry := range entries {
fmt.Println(entry.Name())
}
Entry Attributes:
entry.Name
()
: Name of the file or directory.entry.IsDir()
: Returnstrue
if the entry is a directory.
Removing Files and Directories
Remove a File
Use os.Remove()
.
err := os.Remove("oldfile.txt")
if err != nil {
fmt.Println("Error removing file:", err)
return
}
Remove a Directory
Use os.Remove()
if the directory is empty.
err := os.Remove("emptydir")
Remove a Directory and Its Contents
Use os.RemoveAll()
.
err := os.RemoveAll("parent")
if err != nil {
fmt.Println("Error removing directory and its contents:", err)
return
}
File Information
Getting File Info
Use os.Stat()
or os.Lstat()
to get file information.
info, err := os.Stat("example.txt")
if err != nil {
if os.IsNotExist(err) {
fmt.Println("File does not exist.")
} else {
fmt.Println("Error stating file:", err)
}
return
}
fmt.Printf("File Name: %s\n", info.Name())
fmt.Printf("Size: %d bytes\n", info.Size())
fmt.Printf("Permissions: %s\n", info.Mode())
fmt.Printf("Modification Time: %s\n", info.ModTime())
fmt.Printf("Is Directory: %t\n", info.IsDir())
FileInfo Interface
os.FileInfo
is an interface providing methods to access file metadata.
Methods:
Name() string
: Base name of the file.Size() int64
: Length in bytes.Mode() FileMode
: File mode bits.ModTime() time.Time
: Modification time.IsDir() bool
: Returnstrue
if the file is a directory.Sys() interface{}
: Underlying data source (can be used for platform-specific information).
Environment Variables
Getting Environment Variables
Use os.Getenv()
to get the value of an environment variable.
path := os.Getenv("PATH")
fmt.Println("PATH:", path)
Setting Environment Variables
Use os.Setenv()
to set an environment variable.
err := os.Setenv("MY_VAR", "my_value")
if err != nil {
fmt.Println("Error setting environment variable:", err)
return
}
Unsetting Environment Variables
Use os.Unsetenv()
to remove an environment variable.
err := os.Unsetenv("MY_VAR")
if err != nil {
fmt.Println("Error unsetting environment variable:", err)
return
}
Listing All Environment Variables
Use os.Environ()
to get a slice of all environment variables in the format "KEY=value"
.
envVars := os.Environ()
for _, envVar := range envVars {
fmt.Println(envVar)
}
To split the key and value:
for _, envVar := range envVars {
pair := strings.SplitN(envVar, "=", 2)
key := pair[0]
value := pair[1]
fmt.Printf("%s: %s\n", key, value)
}
Process Management
Process IDs and Exit Codes
Getting the Current Process ID
pid := os.Getpid()
fmt.Println("Process ID:", pid)
Getting the Parent Process ID
ppid := os.Getppid()
fmt.Println("Parent Process ID:", ppid)
Exiting the Program with a Status Code
Use os.Exit()
.
func main() {
// ...
if errorOccurred {
fmt.Println("An error occurred.")
os.Exit(1) // Non-zero exit code indicates an error
}
os.Exit(0) // Zero exit code indicates success
}
Note: Deferred functions are not run when os.Exit()
is called.
Executing External Commands
For executing external commands, use the os/exec
package.
import (
"os/exec"
// ...
)
cmd := exec.Command("ls", "-l")
output, err := cmd.Output()
if err != nil {
fmt.Println("Error executing command:", err)
return
}
fmt.Println(string(output))
Signals
Handling Signals
Use the os/signal
package to handle operating system signals.
import (
"os"
"os/signal"
"syscall"
// ...
)
func main() {
sigs := make(chan os.Signal, 1)
done := make(chan bool, 1)
signal.Notify(sigs, syscall.SIGINT, syscall.SIGTERM)
go func() {
sig := <-sigs
fmt.Println()
fmt.Println("Received signal:", sig)
done <- true
}()
fmt.Println("Awaiting signal")
<-done
fmt.Println("Exiting")
}
This program waits for SIGINT
(Ctrl+C) or SIGTERM
signals and exits gracefully when received.
Temporary Files and Directories
Creating Temporary Files
Use os.CreateTemp()
(Go 1.17 and later) or ioutil.TempFile()
(deprecated) to create a temporary file.
file, err := os.CreateTemp("", "tempfile_*.txt")
if err != nil {
fmt.Println("Error creating temporary file:", err)
return
}
defer os.Remove(file.Name()) // Clean up
fmt.Println("Temporary file created:", file.Name())
Creating Temporary Directories
Use os.MkdirTemp()
(Go 1.17 and later) or ioutil.TempDir()
(deprecated).
dir, err := os.MkdirTemp("", "tempdir_")
if err != nil {
fmt.Println("Error creating temporary directory:", err)
return
}
defer os.RemoveAll(dir) // Clean up
fmt.Println("Temporary directory created:", dir)
Symlinks and File System Operations
Creating Symlinks
Use os.Symlink()
.
err := os.Symlink("target.txt", "link.txt")
if err != nil {
fmt.Println("Error creating symlink:", err)
return
}
Reading Symlinks
Use os.Readlink()
.
target, err := os.Readlink("link.txt")
if err != nil {
fmt.Println("Error reading symlink:", err)
return
}
fmt.Println("Symlink points to:", target)
Changing File Permissions
Use os.Chmod()
.
err := os.Chmod("example.txt", 0644)
if err != nil {
fmt.Println("Error changing file permissions:", err)
return
}
Changing File Ownership
Use os.Chown()
.
err := os.Chown("example.txt", uid, gid)
if err != nil {
fmt.Println("Error changing file ownership:", err)
return
}
Note: Changing ownership may require elevated privileges.
Renaming and Moving Files
Use os.Rename()
.
err := os.Rename("oldname.txt", "newname.txt")
if err != nil {
fmt.Println("Error renaming file:", err)
return
}
Copying Files
The os
package does not provide a direct method to copy files. You can read from the source and write to the destination.
func copyFile(src, dst string) error {
sourceFile, err := os.Open(src)
if err != nil {
return err
}
defer sourceFile.Close()
destFile, err := os.Create(dst)
if err != nil {
return err
}
defer destFile.Close()
_, err = io.Copy(destFile, sourceFile)
if err != nil {
return err
}
// Flush file to storage
err = destFile.Sync()
return err
}
Constants and Variables in os
File Mode Constants
The os
package provides constants to represent file modes and permissions.
File Types:
os.ModeDir
: Is a directory.os.ModeSymlink
: Is a symbolic link.os.ModeNamedPipe
: Is a named pipe (FIFO).os.ModeSocket
: Is a Unix domain socket.os.ModeDevice
: Is a device file.
Permission Bits:
os.ModePerm
: 0777, Unix permission bits.
Common Variables
os.PathSeparator
: Platform-specific path separator ('/'
on Unix,'\'
on Windows).os.PathListSeparator
: Separator for list of paths (':'
on Unix,';'
on Windows).os.DevNull
: Name of the null device ("/dev/null"
on Unix,"NUL"
on Windows).
Best Practices
Error Handling: Always check for errors when performing file and directory operations.
Resource Management: Use
defer
to ensure files are closed and resources are released.Platform Independence: Be cautious of platform-specific behavior. Use
filepath
package for manipulating file paths in a platform-independent way.Permissions: Be mindful of file permissions, especially when creating files and directories.
Security Considerations: Validate inputs when dealing with file paths to prevent path traversal vulnerabilities.
Concurrency: Be cautious when accessing files from multiple goroutines. Use synchronization mechanisms if necessary.
Conclusion
The os
package is a powerful tool for interacting with the operating system in Go. It provides a rich set of functions for file and directory manipulation, environment variable management, process control, and more. Understanding how to use the os
package effectively is essential for building robust and efficient applications.
By mastering the os
package, you'll be able to:
Read and write files efficiently.
Manage file permissions and ownership.
Navigate and manipulate the file system.
Handle environment variables and configurations.
Interact with processes and handle signals.
Create utilities and tools that require low-level OS interactions.
Additional Resources
Official Documentation:
Go by Example:
Blogs and Tutorials:
Keep exploring and experimenting with the os
package to deepen your understanding and enhance your Go programming skills!
Subscribe to my newsletter
Read articles from Sundaram Kumar Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sundaram Kumar Jha
Sundaram Kumar Jha
I Like Building Cloud Native Stuff , the microservices, backends, distributed systems and cloud native tools using Golang