Controlled Elements in React: A Practical Demo
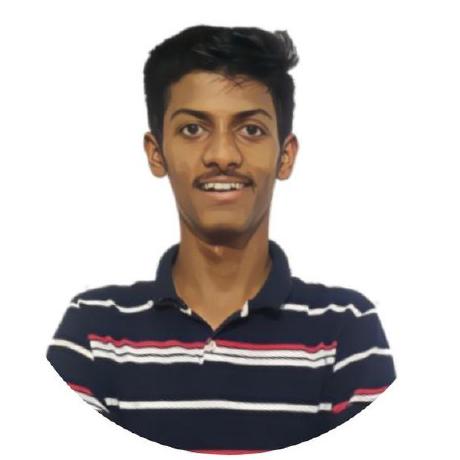
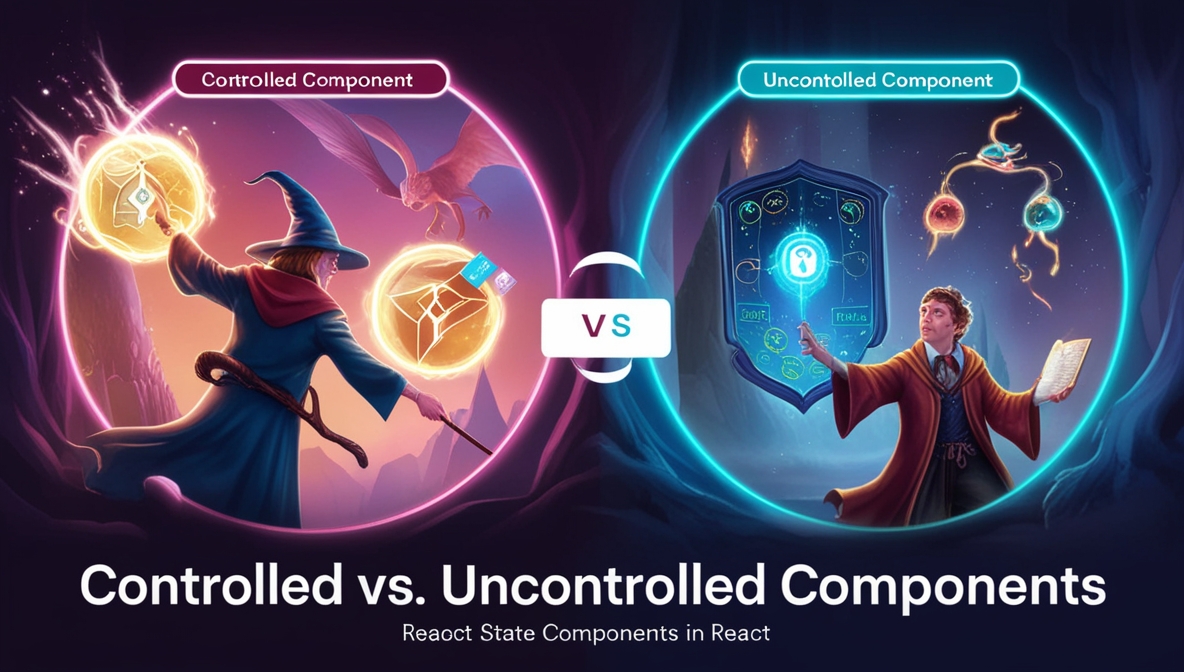
In React, controlled components are a fundamental concept that allow you to manage the state of form elements through React's state management. This approach ensures that form inputs are controlled by React, making your UI more predictable and easier to debug.
Demo: Creating Controlled Components
Let's walk through a simple example of a controlled form component in React. We will create a form with a text input and a submit button. The input's value will be controlled by React state.
Step 1: Create a Piece of State
First, we'll need to create a state variable to hold the value of the input field. We use the useState
hook for this purpose.
jsxCopy codeimport React, { useState } from 'react';
const ControlledForm = () => {
// Step 1: Create a piece of state
const [inputValue, setInputValue] = useState('');
return (
<div>
<h1>Controlled Form</h1>
<form>
<label>
Input:
{/* Step 2: Use the state as a value of the input field */}
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)} // Step 3: Set the state on change
/>
</label>
<button type="submit">Submit</button>
</form>
<p>Current Value: {inputValue}</p>
</div>
);
};
export default ControlledForm;
Explanation
Create a Piece of State: We initialize
inputValue
with an empty string usinguseState
. This state will store the current value of the input field.Use the State as a Value: The
value
attribute of the<input>
field is set toinputValue
. This means that the input field's value is controlled by the state.Set the State on Change: We use the
onChange
event handler to update the state whenever the user types into the input field. ThesetInputValue
function updates the state with the new value of the input field.
Enhancing the Demo
To make the example more practical, let's add some additional features:
Validation: Add a simple validation to check if the input is not empty before allowing the form to be submitted.
Resetting Input: Add functionality to clear the input field after submission.
Error Handling: Display an error message if the validation fails.
Here's the enhanced version:
jsxCopy codeimport React, { useState } from 'react';
const EnhancedControlledForm = () => {
const [inputValue, setInputValue] = useState('');
const [error, setError] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
if (inputValue.trim() === '') {
setError('Input cannot be empty');
} else {
setError('');
alert(`Form submitted with value: ${inputValue}`);
setInputValue(''); // Clear input after submission
}
};
return (
<div>
<h1>Enhanced Controlled Form</h1>
<form onSubmit={handleSubmit}>
<label>
Input:
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
/>
</label>
<button type="submit">Submit</button>
</form>
{error && <p style={{ color: 'red' }}>{error}</p>}
<p>Current Value: {inputValue}</p>
</div>
);
};
export default EnhancedControlledForm;
Summary
Controlled components in React help maintain form state consistently by using React state to manage input values. This approach ensures that your form elements behave predictably and are easier to manage. By following the steps outlined above, you can implement controlled components effectively in your React applications.
Feel free to adapt and expand upon this example based on your specific use cases and requirements. Happy coding!
Subscribe to my newsletter
Read articles from Aman Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
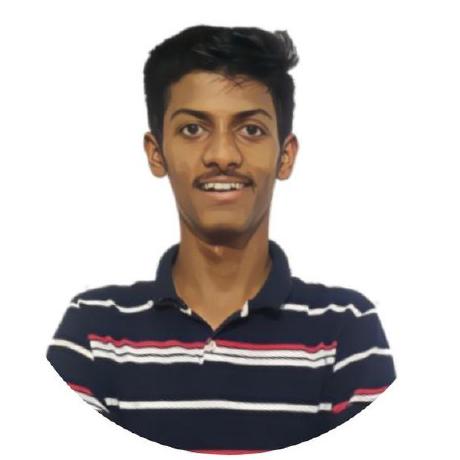
Aman Mishra
Aman Mishra
🧠Data Structures and Algorithms | Python | Java | FullStack Development | Open Source