Docker Compose Deep Dive: Advanced Techniques for Multi-Container Applications

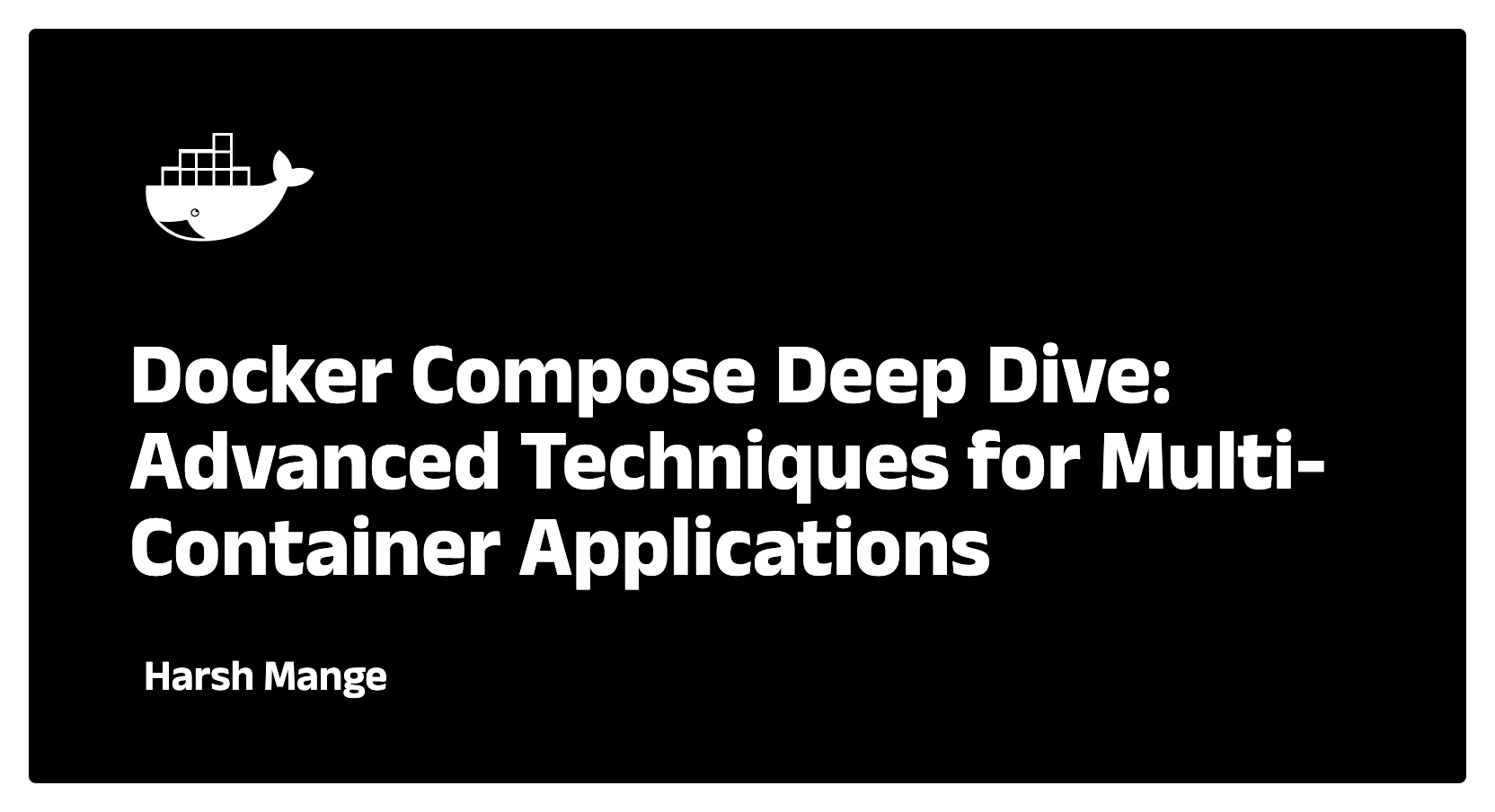
Introduction
In this final blog of the Docker series, we'll take a deep dive into Docker Compose and advanced Docker topics, focusing on how to orchestrate multi-container applications. We'll cover how Docker Compose simplifies the management of complex systems, the nuances of networking, handling environment variables and secrets, as well as production-ready techniques such as scaling services, dependency management, and testing strategies.
If you're a seasoned developer working with microservices, databases, or any multi-container environment, Docker Compose is an essential tool to streamline your workflows and boost productivity.
What is Docker Compose?
Docker Compose is a tool designed to define and run multi-container Docker applications. Instead of manually starting multiple containers, linking them, and managing their configurations, you can describe your entire application stack using a simple YAML file. This enables you to easily start, stop, and manage complex applications with a single command.
A typical Docker Compose file (named docker-compose.yml
) defines the services your application consists of, along with networks, volumes, and other configurations.
Basic Structure of a docker-compose.yml
The basic structure of a docker-compose.yml
file includes:
services: The individual containers that make up your application.
volumes: The persistent storage shared among containers.
networks: Defines custom networks for communication between containers.
build: Specifies Dockerfiles to build custom images.
ports: Maps container ports to host ports.
Here’s a basic example of a Docker Compose file for a simple web application with a database:
version: '3.8'
services:
web:
build: .
ports:
- "8080:80"
volumes:
- .:/app
depends_on:
- db
db:
image: postgres:13
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
volumes:
- db_data:/var/lib/postgresql/data
volumes:
db_data:
In this example:
The web service builds the image from the Dockerfile in the current directory and maps port
80
in the container to port8080
on the host.The db service uses the official
postgres:13
image, passing environment variables for the database credentials.The volumes section defines a named volume (
db_data
) to store PostgreSQL data persistently.
Running Docker Compose
Once you've defined your services in the docker-compose.yml
file, you can use Docker Compose commands to manage your application:
Starting services:
docker-compose up
This command builds and starts all services. The
-d
flag runs containers in detached mode:docker-compose up -d
Stopping services:
docker-compose down
Viewing service logs:
docker-compose logs
This command displays logs from all services, and you can filter logs by service using:
docker-compose logs <service_name>
Scaling services:
docker-compose up --scale web=3
This command scales the web service to run three replicas. Scaling is particularly useful when testing distributed systems or load balancing in development environments.
Advanced Docker Compose Concepts
1. Dependency Management with depends_on
In complex applications, certain services depend on others (e.g., a web app might depend on a database). Docker Compose makes it easy to manage such dependencies with the depends_on
directive, which ensures that containers are started in the right order.
services:
web:
depends_on:
- db
However, it’s important to note that depends_on
only controls the start order. It doesn’t wait for the db
service to be fully up and running. For full health-checking and waiting for service readiness, consider using a more robust solution, such as wait-for-it
scripts or implementing HEALTHCHECK
in your Dockerfiles.
2. Docker Networks in Compose
Docker Compose allows you to define custom networks to facilitate communication between services. By default, all services defined in a Compose file are placed on a common network, and they can communicate with each other using their service name as the hostname.
version: '3.8'
services:
web:
build: .
networks:
- frontend
db:
image: postgres:13
networks:
- backend
networks:
frontend:
backend:
In this example, the web
service is placed on the frontend
network, while the db
service is on the backend
network. This allows you to separate concerns and restrict communication between certain services. If you want to allow communication across networks, you can add services to multiple networks.
3. Environment Variables and Secrets
Managing configuration and sensitive data is crucial in production environments. Docker Compose provides an easy way to inject environment variables and secrets into containers.
Environment Variables:
You can pass environment variables in a few ways:
Directly in the
docker-compose.yml
:services: web: environment: - API_KEY=your-api-key
Using an external
.env
file:services: web: env_file: - .env
The
.env
file might look like this:API_KEY=your-api-key
Secrets:
For sensitive data (like database passwords or API keys), Docker supports secrets management. Here’s an example using secrets in Docker Compose:
services:
db:
image: postgres:13
secrets:
- db_password
secrets:
db_password:
file: ./db_password.txt
This mounts the secret inside the container at runtime, and it’s only accessible to the service that needs it.
Docker Compose in Production
While Docker Compose is great for development environments, it can also be used in production. Here are some best practices and tips:
1. Multi-Environment Configurations
You can extend and override Compose files to manage different configurations for development, staging, and production environments. Docker Compose allows you to define multiple files using the -f
flag.
Example:
docker-compose -f docker-compose.yml -f docker-compose.prod.yml up
The docker-compose.prod
.yml
might look like this:
version: '3.8'
services:
web:
image: myapp:latest
environment:
- NODE_ENV=production
deploy:
replicas: 3
Here, we scale the web
service to 3 replicas in production and set the environment to production
.
2. Scaling and Load Balancing
In production, you can use Docker Compose to scale services for load balancing. By leveraging Docker Swarm or Kubernetes (Compose has support for Swarm natively), you can deploy and manage multi-node clusters that distribute traffic among scaled containers.
For example, using docker-compose.yml
:
services:
web:
image: myapp:latest
deploy:
replicas: 5
resources:
limits:
cpus: "0.5"
memory: "512M"
In this setup, five replicas of the web
service are created, each limited to 0.5 CPUs and 512 MB of memory.
3. Service Health Checks
Adding health checks to services ensures that Docker only routes traffic to healthy containers. This is essential for ensuring the reliability of applications in production.
services:
web:
image: myapp:latest
healthcheck:
test: ["CMD", "curl", "-f", "http://localhost"]
interval: 1m30s
timeout: 10s
retries: 3
This health check pings the service every 90 seconds and retries up to three times before marking the service as unhealthy.
Testing with Docker Compose
Testing in containerized environments is simplified with Docker Compose. By defining test environments with separate configurations, you can spin up isolated containers, run your test suite, and then tear down the environment after tests complete.
For instance, a test-specific Docker Compose configuration could spin up a mock database, run the application, execute tests, and then clean everything up:
services:
test_runner:
build: .
command: ["pytest"]
depends_on:
- db
db:
image: postgres:13
environment:
POSTGRES_USER: testuser
POSTGRES_PASSWORD: testpassword
You can then run your tests with:
docker-compose -f docker-compose.test.yml up --abort-on-container-exit
Conclusion
Docker Compose is a powerful tool for orchestrating multi-container applications, and when combined with advanced practices like scaling, health checks, and secrets management, it becomes a critical part of any developer's toolkit. In production, Compose can streamline the deployment and scaling of applications, making it easier to manage complex architectures.
Now that we’ve covered Docker Compose and some advanced Docker topics, you’re well-equipped to handle even the most intricate containerized environments. Whether in development or production, Docker and Docker Compose provide the flexibility, scalability, and simplicity required to efficiently manage multi-container applications.
Subscribe to my newsletter
Read articles from Harsh Mange directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harsh Mange
Harsh Mange
This is Harsh Mange, working as a Software Engineer - Backend at Argoid. I love building apps. Working on contributing to open-source projects and the dev community.