Mastering Enums in Laravel: Handling Enum Values in Blade Templates

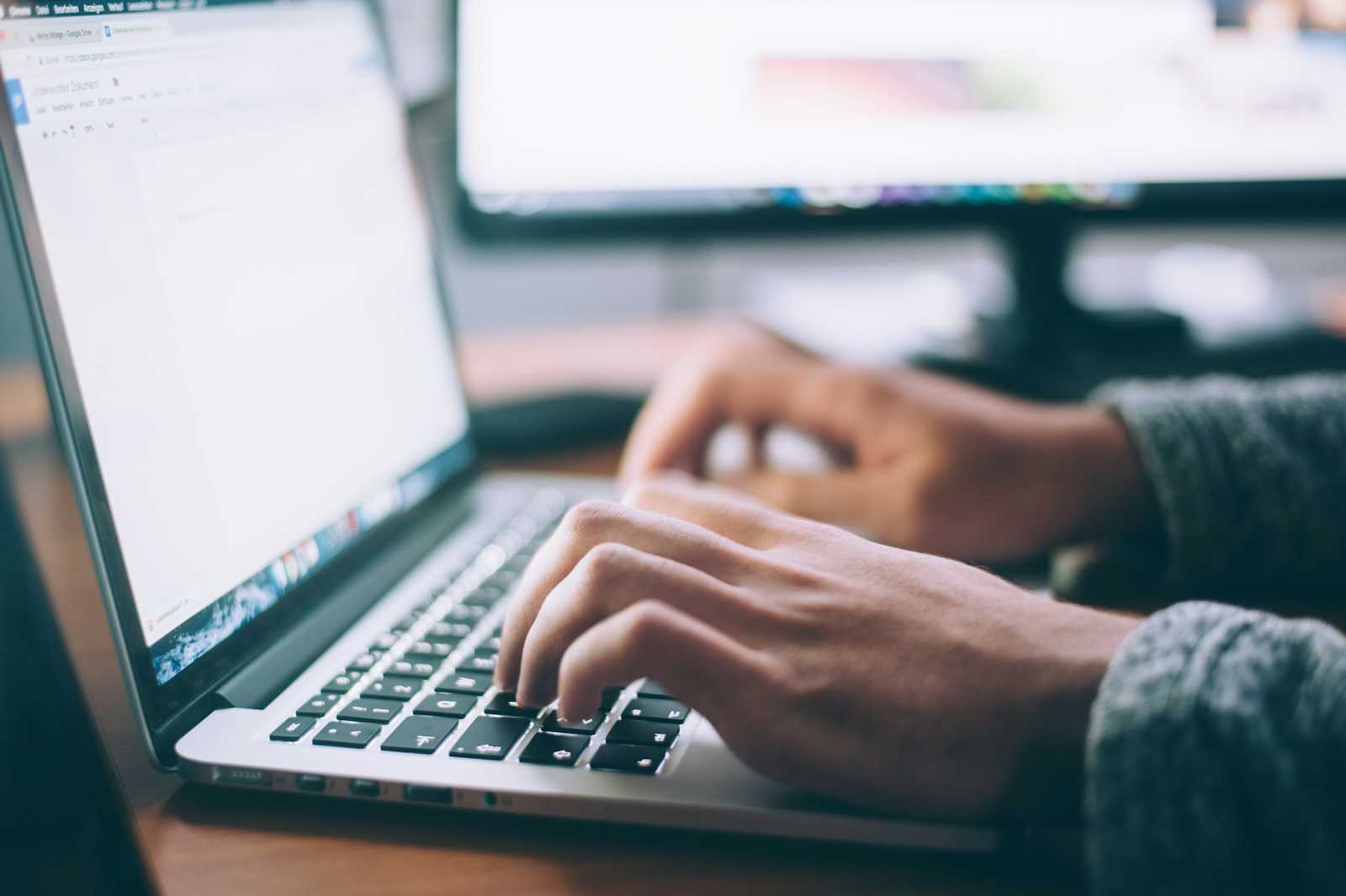
Enums are a powerful feature in Laravel that help maintain clean and expressive code. However, when working with enums in Blade templates, it is important to understand how to handle them correctly to avoid unexpected behavior. In this post, we will explore how to effectively use enums, particularly when comparing their values within Blade templates.
The Challenge: Comparing Enum Values in Blade Templates
A common scenario is when you want to conditionally display certain sections of your Blade template based on an enum field in your model. For example, suppose you have a UserType
enum that defines various user roles such as 'admin', 'seller', 'agent', and 'agency'. You may want to display specific navigation options or UI elements based on the user’s type.
At first glance, you might attempt to write a Blade condition like this:
@if (auth()->user()->user_type === 'agent' || auth()->user()->user_type === 'agency')
<!-- Show navigation items for agents and agencies -->
@endif
While this may seem correct, it will not work as expected. This is because enums in Laravel are not simple strings—they are objects that require special handling.
The Solution: Accessing the Enum’s value
Property
To properly compare enum values in Blade templates, it is necessary to access the value
property of the enum. This property contains the actual string value, such as 'agent' or 'agency', which can be used for comparison.
Here is the correct approach:
@if (auth()->user()->user_type->value === 'agent' || auth()->user()->user_type->value === 'agency')
<!-- Show navigation items for agents and agencies -->
@endif
Why This Works:
When you define an enum in Laravel, it is treated as an object with various methods and properties. One of these properties is value
, which stores the actual string that you want to compare. Therefore, instead of comparing the enum object directly, you should compare its value
property.
Step-by-Step Example: Defining and Using Enums
Let us walk through a more complete example. Suppose you have defined a UserType
enum in your Laravel application as follows:
<?php
namespace App\Enums;
enum UserType: string
{
case Admin = 'admin';
case Seller = 'seller';
case Agent = 'agent';
case Agency = 'agency';
}
In your User
model, you would reference this enum like this:
<?php
namespace App\Models;
use App\Enums\UserType;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
protected $casts = [
'user_type' => UserType::class,
];
}
Now, in your Blade template, you can compare the user’s type using the value
property:
@if (auth()->user()->user_type->value === UserType::Agent->value || auth()->user()->user_type->value === UserType::Agency->value)
<nav>
<!-- Navigation for agents and agencies -->
</nav>
@endif
By using UserType::Agent->value
and UserType::Agency->value
, you ensure that the comparison is performed correctly against the string values of the enum.
A Cleaner Approach: Enum Methods
To make the code more maintainable, you can add helper methods to your enum class. For example:
<?php
namespace App\Enums;
enum UserType: string
{
case Admin = 'admin';
case Seller = 'seller';
case Agent = 'agent';
case Agency = 'agency';
public function isAgentOrAgency(): bool
{
return $this === self::Agent || $this === self::Agency;
}
}
In your Blade template, the comparison can now be simplified:
if (auth()->user()->user_type->isAgentOrAgency())
<nav>
<!-- Navigation for agents and agencies -->
</nav>
@endif
This approach not only makes the code more readable but also enhances reusability.
Validating Enum Values in Laravel
If you are using enums in your models, you will likely need to validate user input against the enum values. Laravel provides a straightforward way to validate enum values using custom validation rules. Here is an example of how to validate that a user’s user_type
is one of the valid enum values:
$request->validate([
'user_type' => ['required', new Enum(UserType::class)],
]);
Additionally, you can cast database fields to enums using Laravel’s built-in casting feature, which ensures that your application logic consistently works with the enum object:
protected $casts = [
'user_type' => UserType::class,
];
Why Use Enums in Laravel?
Using enums in Laravel offers several advantages:
Type Safety: Enums ensure that only valid values are assigned to a field, reducing the likelihood of bugs.
Self-Documentation: Enums make your code more readable and easier to understand by providing descriptive names for values.
Consistency: Enums help maintain consistent data and behavior across your application.
Reduced Errors: By using enums, you eliminate the risk of typographical errors in string-based comparisons.
Conclusion
Enums in Laravel provide an elegant and type-safe way to represent fixed sets of values. When working with enums in Blade templates, it is crucial to compare the value
property rather than the enum object itself. By using helper methods and proper validation, you can make your code cleaner, more readable, and easier to maintain.
Subscribe to my newsletter
Read articles from Asfia Aiman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfia Aiman
Asfia Aiman
Hey Hashnode community! I'm Asfia Aiman, a seasoned web developer with three years of experience. My expertise includes HTML, CSS, JavaScript, jQuery, AJAX for front-end, PHP, Bootstrap, Laravel for back-end, and MySQL for databases. I prioritize client satisfaction, delivering tailor-made solutions with a focus on quality. Currently expanding my skills with Vue.js. Let's connect and explore how I can bring my passion and experience to your projects! Reach out to discuss collaborations or learn more about my skills. Excited to build something amazing together! If you like my blogs, buy me a coffee here https://www.buymeacoffee.com/asfiaaiman