Vue + TS-based text to QR code

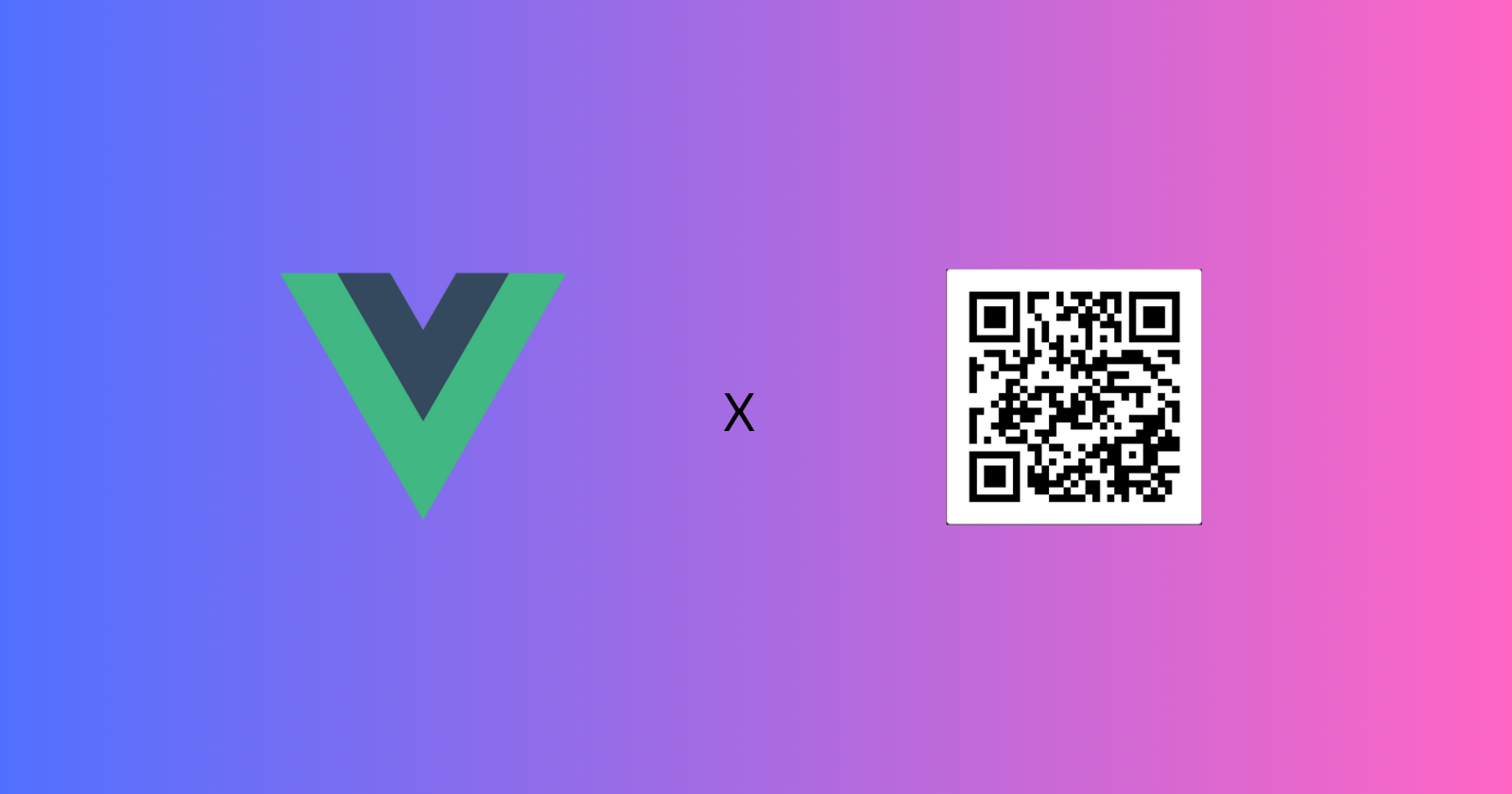
Introduction
This project was developed to solve the issue of sharing text-based data between devices, which will be available for all platforms. The project is broken down into two parts. We will build a QR code generator and scanner using modern web technologies like Vue 3, TypeScript, and various AWS services in this two-part series. Each part of this series will focus on a specific aspect:
Part 1: We'll build a QR code generator where users can input text, generate a QR code, and download it as an image.
Part 2: We'll add QR code scanning functionality, where users can scan QR codes using their camera or upload QR code images to get the information.
You can see this project live here. It is built until part 1 and will be updated throughout this series.
Introduction to Part 1: QR Code Generator
This first part will focus on building the QR code generator. By the end of this tutorial, you will have an app where users can input text to generate a QR code and download it as an image. This section will cover everything from setting up a Vue 3 project to implementing the QR code generation logic and adding a download feature.
We will use Vue for the front end and VueUse to handle QR code generation.
2. Setting Up the Project
We will start with setting up the environment and installing the necessary tools to develop the QR code generator.
Step 1: Create a Vue 3 Project Using Vite
We will start by setting up a Vue 3 project using Vite, a fast and lightweight build tool.
Open your terminal, run the following command, and select the “Vue” and “Typescript” options to create a new project:
npm create vite@latest
This command initializes a new Vue 3 project using TypeScript with Vite.
Navigate to the project directory:
cd <your project name>
Install dependencies:
npm install
Test that everything is set up by running the development server:
npm run dev
This will start the Vite development server. Open your browser and go to the URL provided to see the default Vue 3 app.
Step 2: Install Required Dependencies
Now that the basic project setup is done, we will install the necessary dependencies for the QR code generator.
VueUse - This is a collection of useful utilities for Vue 3, including the QR code generator we'll use.
Qrcode - The VueUse needs this package to generate QrCode.
npm install @vueuse/integrations @vueuse/core qrcode
Now, the project is fully set up with the required dependencies and a clear structure. Next, we'll build the QR code generator component by component.
3. Building the QR Code Generator
Now that the project is set up let’s start building the core components for the QR code generator. We will create two key components: one for inputting text (TextBox.vue
) and one for displaying and downloading the generated QR code (QRCodeDisplay.vue
). These will then be integrated into the main App.vue
file.
Step 1: Create the TextBox.vue
Component
This component will allow the user to input text, which will be used to generate a QR code.
Create the
TextBox.vue
file in thecomponents/
directory:<template> <div class="textbox-container"> <textarea cols="50" rows="10" v-model="text" placeholder="Enter text to generate QR code" /> <button @click="generateQRCode" class="generate-button"> Generate QR Code </button> </div> </template> <script setup lang="ts"> import { ref } from "vue"; const text = ref(""); // Define props and emits defineProps<{ text: string }>(); const emit = defineEmits(["update:text"]); const emitUpdate = (newText: string) => { emit("update:text", newText); }; const generateQRCode = () => { emitUpdate(text.value); }; </script> <style scoped> .textbox-container { display: flex; flex-direction: column; justify-content: center; align-items: center; padding: 1rem; gap: 1rem; } .generate-button { padding: 0.5rem 1rem; font-size: 1rem; background-color: #9251f9; color: white; border: none; border-radius: 4px; cursor: pointer; } .generate-button:hover { background-color: #7b3dbf; } </style>
The component contains a
textarea
for input and a button to trigger the QR code generation.The
v-model
directive is used for two-way data binding to capture user input.The
emitUpdate
function emits the updated text back to the parent component (App.vue
).
Step 2: Create the QRCodeDisplay.vue
Component
This component will display the generated QR code and allow users to download it as an image.
Create the
QRCodeDisplay.vue
file in thecomponents/
directory:<template> <div class="qrcode-container"> <img v-if="qrCode" class="qr-code" :src="qrCode" alt="QR Code" @click="toggleDialog(true)" /> <div v-else class="note">Enter text to display QR code</div> </div> <!-- Dialog for enlarged QR code --> <div v-if="isDialogOpen" class="dialog-overlay" @click="toggleDialog(false)"> <div class="dialog-content"> <img :src="qrCode" alt="QR Code Enlarged" class="qr-code-enlarged" /> <button class="download-button" @click="downloadQRCode"> Download </button> </div> </div> </template> <script setup lang="ts"> import { ref, defineProps } from "vue"; const props = defineProps<{ qrCode: string }>(); const isDialogOpen = ref(false); const toggleDialog = (value: boolean) => { isDialogOpen.value = value; }; const downloadQRCode = () => { const link = document.createElement("a"); link.href = props.qrCode; link.download = "qrcode.png"; link.click(); }; </script> <style scoped> .qrcode-container { display: flex; justify-content: center; align-items: center; padding: 1rem; } .qr-code { max-width: 20vw; border: 1px solid #ccc; border-radius: 4px; cursor: pointer; } .dialog-overlay { position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.5); display: flex; justify-content: center; align-items: center; } .dialog-content { background-color: #fff; padding: 1rem; border-radius: 4px; } .qr-code-enlarged { width: 300px; height: auto; margin-bottom: 1rem; } .download-button { background-color: #9251f9; color: white; padding: 0.5rem 1rem; border: none; border-radius: 4px; cursor: pointer; } .download-button:hover { background-color: #7b3dbf; } </style>
This component takes a
qrCode
prop, which is the QR code image URL.The image is displayed with an option to enlarge it in a dialog and download it.
The
downloadQRCode
function allows the QR code to be downloaded as an image.
Step 3: Integrate Components in App.vue
Finally, we’ll integrate these components into the App.vue
file and implement the QR code generation logic.
Edit the
App.vue
file:<template> <div class="container"> <TextBox v-model:text="text" class="textbox" /> <QRCodeDisplay :qrCode="qrCode" class="qrcode-display" /> </div> </template> <script setup lang="ts"> import { ref, watch } from "vue"; import { useQRCode } from "@vueuse/integrations/useQRCode"; import TextBox from "./components/TextBox.vue"; import QRCodeDisplay from "./components/QRCodeDisplay.vue"; const text = ref(""); const qrCode = ref(""); // Generate the QR code using vueuse's useQRCode const generateQRCode = useQRCode(text, { errorCorrectionLevel: "H", margin: 3, width: 500, }); watch(text, () => { qrCode.value = generateQRCode.value; }); </script> <style scoped> .container { display: flex; justify-content: space-between; align-items: start; padding: 2rem; width: 100%; } .textbox, .qrcode-display { width: 48%; } </style>
The
TextBox
andQRCodeDisplay
components are imported and integrated into the main app.The
useQRCode
function from VueUse is used to generate a QR code when the text changes dynamically.The
watch
function ensures that the QR code is updated whenever the input text is modified.
With the built QR code generator, users can now input text, generate a QR code, and download it.
Conclusion
In this first part of the series, we have successfully built a QR code generator using Vue 3, TypeScript, and Vite. We learned how to set up a Vue 3 project, create reusable components for input and QR code display, and generate QR codes dynamically based on user input. We also added functionality to download the generated QR code as an image.
You can check out the complete code for this project on GitHub.
In the next part of this series, we’ll cover how to scan a QR code and get the text embedded in it. You can also learn how to automate the deployment of this app or any other web app by following the guide in the blog: Automate Deployment to AWS with GitHub Actions.
Subscribe to my newsletter
Read articles from Rabinson Thapa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
