Python Guide: Writing a "Caesar Cipher" Program
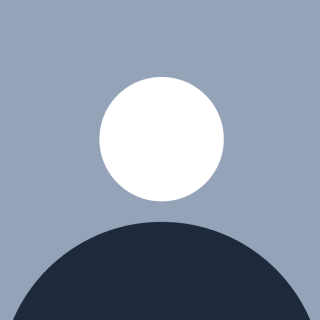
The alphabet is a list that contains all the lowercase letters of the English alphabet.
alphabet = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm',
'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z']
In Encryption function , “Plain text” contains the message user wants to encrypt. “Shift key” contains the no. of positions by which user wants to shift each letter in message.
If the character is found in the alphabet list, it calculates its position, then shifts its position by adding the shift key using modular arithmetic (pos + shift_key) % 26*. The* “Cipher text” holds the encrypted message.
If the character is not in the list (like spaces or punctuation), it is added to cipher text unchanged.
def encryption(plain_text, shift_key):
cipher_text = ""
for i in plain_text:
if i in alphabet:
pos = alphabet.index(i)
new_pos = (pos + shift_key) % 26
cipher_text += alphabet[new_pos]
else:
cipher_text += i
return cipher_text
In Decryption function , “Cipher text” contains the encrypted message user wants to decrypt. “Shift key” contains the no. of positions by which user wants to shift reverse each letter in message.
If the character is in the alphabet list, it calculates its org position by subtracting the shift key from the current pos (pos - shift_key) % 26*. The “Plain text” holds the decrypted message.*
If the character is not in the list, it is added to plain text unchanged.
def decryption(cipher_text, shift_key):
plain_text = ""
for i in cipher_text:
if i in alphabet:
pos = alphabet.index(i)
new_pos = (pos - shift_key) % 26
plain_text += alphabet[new_pos]
else:
plain_text += i
return plain_text
In main , starts by setting the variable “try_again” to false, and enters a “while” loop that continues until the user no longer wants to try encryption or decryption.
The user choose either encrypt or decrypt using the variable “n”. Based on the user's choice, either the encryption or decryption function is called.
while True:
n = input("Type 'encrypt' for encryption, type 'decrypt' for decryption:\n")
message = input("Type your message:\n").lower()
shift = int(input("Type the shift number:\n"))
if n == 'encrypt':
result=encryption(plain_text=message, shift_key=shift)
print(f"The encrypted message is {result}")
elif n == 'decrypt':
result=decryption(cipher_text=message, shift_key=shift)
print(f"The decrypted message is {result}")
a = input("Type 'yes' if you want to go again. Otherwise type 'no':\n")
if a == 'no':
try_again = True
print("Goodbye")
break
Subscribe to my newsletter
Read articles from Arunmathavan K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by