Understanding Asynchronous JavaScript: A Simple Guide with Real-Life Analogies 👨‍💻
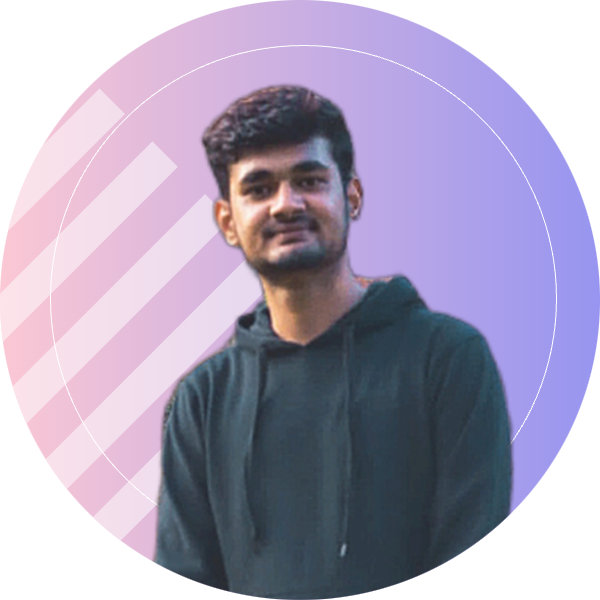
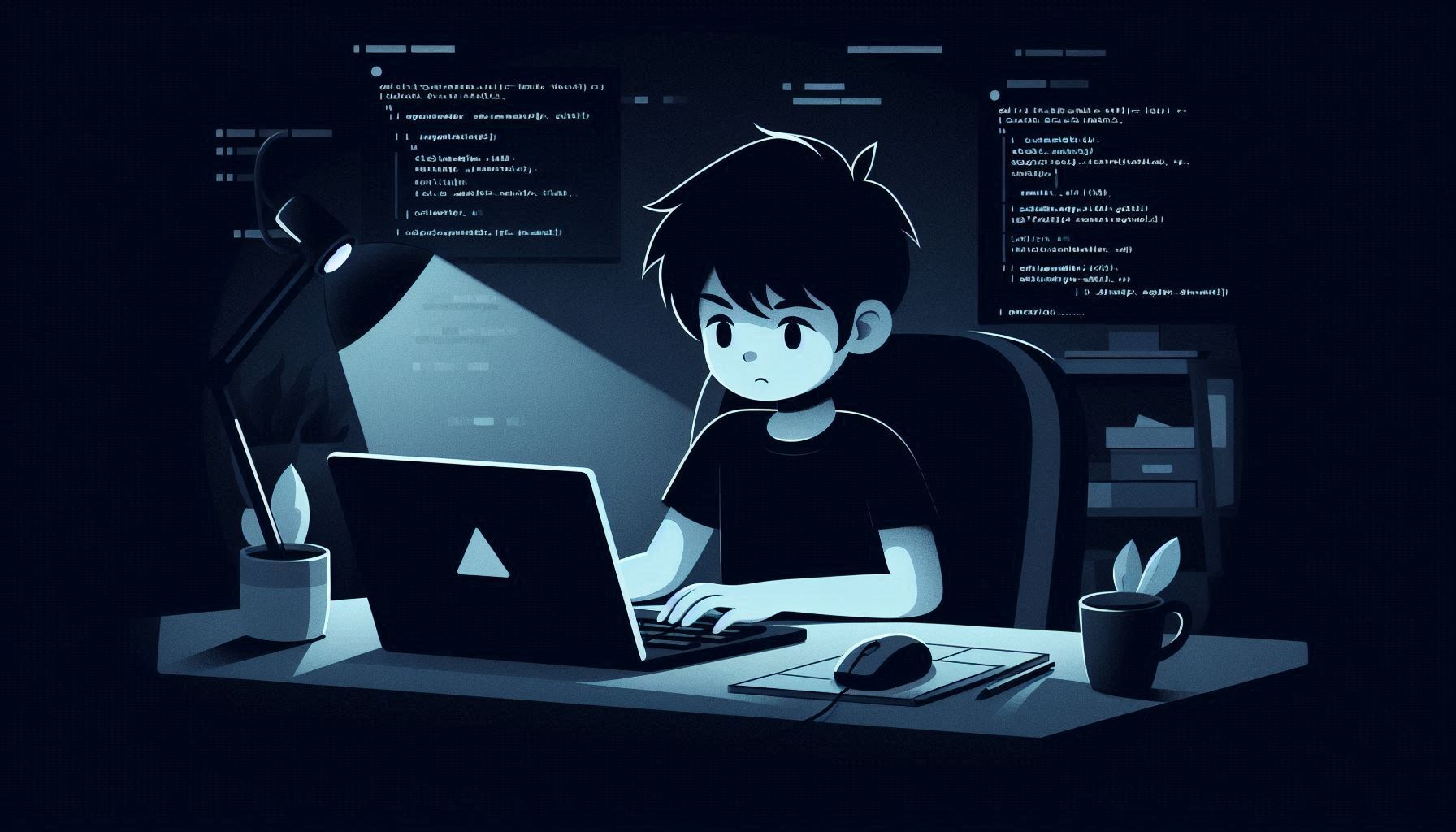
JavaScript is designed to be single-threaded, meaning it can only execute one task at a time. Imagine you're in a kitchen with only one chef (JavaScript). The chef can only prepare one dish at a time, but sometimes tasks like boiling water or waiting for a delivery take longer. Instead of waiting around, the chef can handle other quick tasks while those time-consuming operations are still happening in the background. This is exactly how asynchronous JavaScript works!
In this blog, we'll break down asynchronous JavaScript using relatable analogies, and explore key concepts like callbacks, promises, and async/await.
What Is Asynchronous JavaScript?
Think of asynchronous JavaScript as multitasking. While a slow operation (like fetching data from an API) is being processed, the rest of your code can continue to execute. This ensures that time-consuming tasks don’t freeze your entire application, making it more efficient and responsive.
Kitchen Analogy:
In a kitchen, if you are waiting for water to boil (a slow task), you don’t stand still and wait. You chop vegetables, set the table, or prep other ingredients while the water is heating up. Once it’s ready, you can return to the boiling pot. This is how asynchronous JavaScript handles slow tasks in the background, allowing the program (or "chef") to work on something else while waiting.
Key Concepts in Asynchronous JavaScript
1. Callbacks: Asking Someone Else to Help
A callback is like asking a kitchen helper to notify you when a certain task is done. Imagine you’re baking bread and have to wait for the dough to rise. Instead of sitting idle, you ask your helper, “Hey, let me know when the dough is ready, and I’ll start baking it.” This is essentially how callbacks work— you pass a function that will be called when the task finishes.
Example:
function prepareDough(callback) {
setTimeout(() => {
console.log("Dough is ready!");
callback(); // Notify that the dough is ready
}, 3000); // Simulate waiting time
}
function bakeBread() {
console.log("Baking bread...");
}
prepareDough(bakeBread);
In the code above, prepareDough
waits for the dough to be ready. While waiting, the program can handle other tasks, and when the dough is done, the callback bakeBread
is executed.
Problem: Callback Hell
If you have too many tasks dependent on each other, callbacks can become deeply nested, like asking your kitchen helper to do many things in a specific order. This can make the code hard to follow and is known as callback hell.
2. Promises: A Delivery Service
A promise is like ordering groceries from an online store. You don't know exactly when the delivery will arrive, but you’re promised that either:
It will be delivered successfully (resolve),
Or something went wrong (reject).
You can keep doing other things while waiting for the delivery. Once the groceries arrive, you can resume cooking, or deal with the problem if the order is missing.
Example:
const groceryDelivery = new Promise((resolve, reject) => {
let isDelivered = true; // Simulating delivery status
setTimeout(() => {
if (isDelivered) {
resolve("Groceries delivered successfully!");
} else {
reject("Delivery failed.");
}
}, 2000); // Simulate waiting time
});
groceryDelivery
.then((message) => {
console.log(message); // "Groceries delivered successfully!"
})
.catch((error) => {
console.error(error); // "Delivery failed."
});
Here, the groceryDelivery
promise simulates waiting for a grocery order. The .then()
handles the successful delivery, and .catch()
handles any issues, such as a failed delivery.
3. Async/Await: Scheduling and Monitoring
Async/Await is like hiring a kitchen manager who oversees everything. They know when each task will be ready, and instead of you constantly checking (like chaining .then()
methods in promises), they wait until each task finishes and inform you when it’s time to act.
async: This marks the function as asynchronous, allowing it to use
await
inside.await: This pauses the function until the promise is resolved, making the process more readable.
Example:
async function cookMeal() {
try {
let groceries = await new Promise((resolve) => {
setTimeout(() => resolve("Groceries delivered!"), 2000);
});
console.log(groceries); // "Groceries delivered!"
console.log("Start cooking...");
} catch (error) {
console.error(error);
}
}
cookMeal();
In this example, cookMeal
waits for the groceries to be delivered using await
. Once they’re delivered, it continues with the cooking process. If something goes wrong (groceries are not delivered), the catch
block will handle the error.
With async/await
, the code looks cleaner and easier to understand compared to the chaining of .then()
and .catch()
.
When Should You Use Asynchronous JavaScript?
Imagine running a restaurant kitchen:
Fetching data from an API: Similar to checking if ingredients have arrived, you don't want the entire kitchen to stop while waiting for delivery.
File I/O operations: Just like waiting for the oven to preheat, reading or writing files takes time, so other tasks should continue.
Timers and intervals: Like setting a timer for a dish in the oven, other tasks can happen while the timer is running.
Conclusion
In the fast-paced kitchen of JavaScript, asynchronous techniques like callbacks, promises, and async/await
help ensure the chef (your code) isn’t stuck waiting on slow tasks. Callbacks are like relying on a kitchen helper, promises are like waiting for a delivery, and async/await
is your trusted kitchen manager.
By mastering these asynchronous concepts, you’ll ensure your JavaScript code runs smoothly, even when handling long-running tasks. Keep practicing, and soon you’ll be whipping up responsive, efficient applications like a pro!
Let me know if this analogy-based guide helped you understand asynchronous JavaScript better :->
Subscribe to my newsletter
Read articles from Musab Rayan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
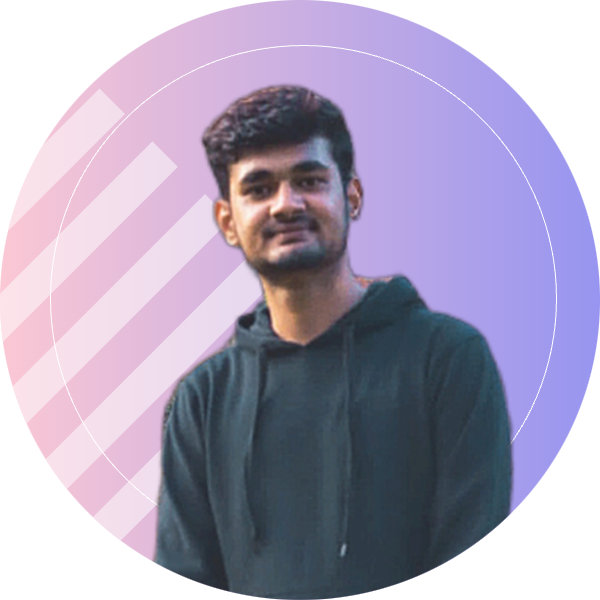
Musab Rayan
Musab Rayan
Hello, I'm Musab Rayan, a third-year CSE student at BSA Crescent Institute of Science and Technology. I love building websites! Since I was a kid, I've been curious about how they work, and now that curiosity drives me into the exciting world of tech. Currently honing my skills in DSA, I aspire to carve a successful career as a software engineer with a focus on innovation.