React Project with Tailwind CSS Installation: A Beginner’s Guide

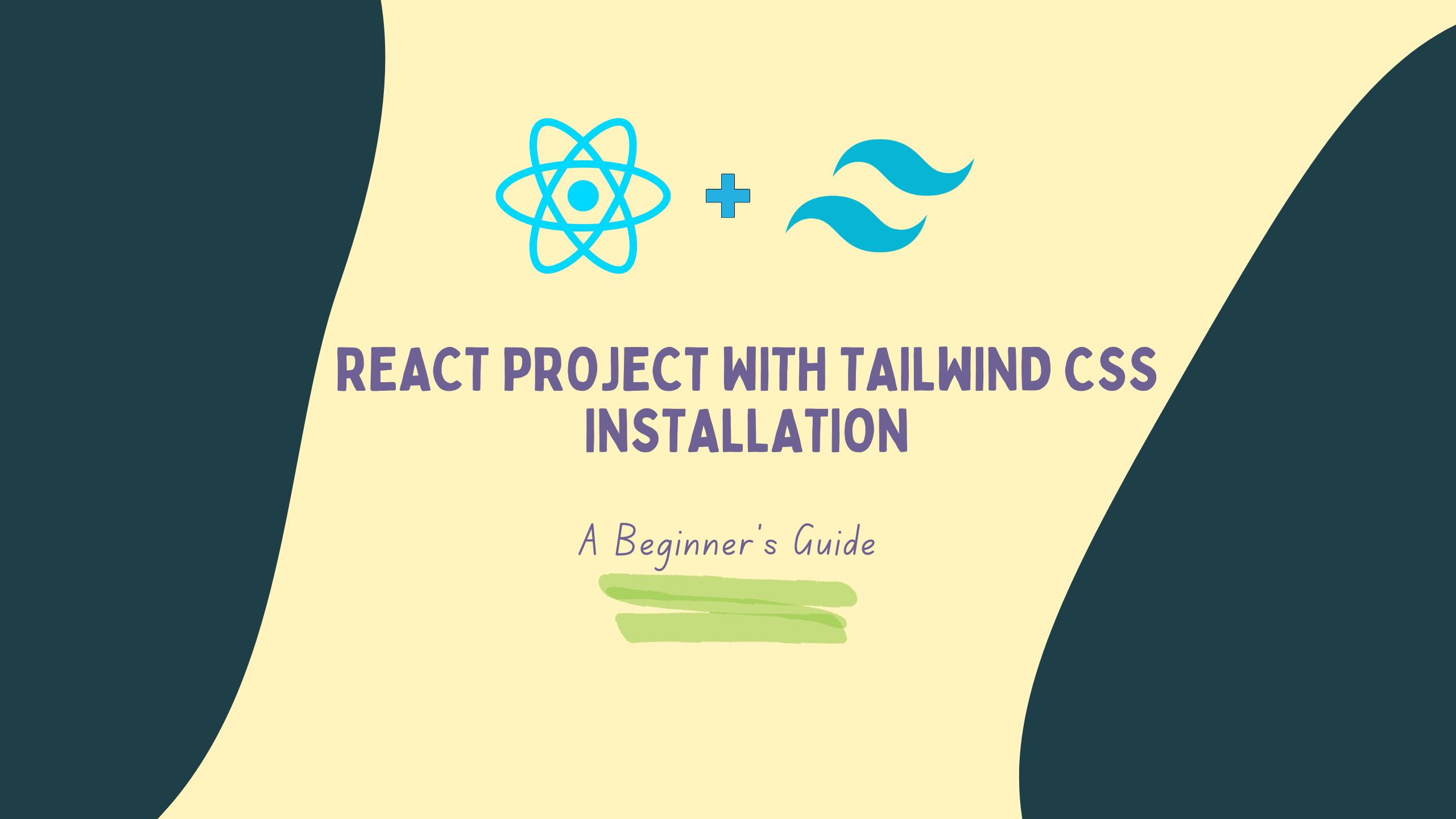
If you’re a developer looking to build responsive and modern user interfaces with ease, using React alongside Tailwind CSS is a fantastic choice. React’s component-based architecture combined with Tailwind’s utility-first CSS approach allows you to build beautiful UIs quickly.
In this blog, I’ll guide you through the step-by-step process of setting up React and Tailwind CSS in your project from scratch.
What is React?
React is a popular JavaScript library for building user interfaces. It helps developers create reusable components, manage states, and build complex user interfaces in a more efficient and scalable way.
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that allows you to rapidly build custom designs without leaving your HTML. It provides low-level utility classes, which enable you to build designs quickly and efficiently by composing classes directly in your markup.
Step 1: Set Up a React Project
First things first, let’s set up a basic React project. We’ll use Create React App, which sets up a new React project with all necessary configurations out of the box.
To create a new React project:
Open your terminal or command prompt and run the following commands:
npx create-react-app react-tailwind-app
cd react-tailwind-app
This will create a folder named react-tailwind-app
and install all the necessary dependencies for React. Once the installation is complete, you can start the development server with:
npm start
This will open your React app in the browser at http://localhost:3000.
Step 2: Install Tailwind CSS
Now that our React project is up and running, let’s install Tailwind CSS. Tailwind CSS requires a few additional configurations compared to regular CSS, but don’t worry – it’s simple!
To install Tailwind CSS:
- In your React project directory, install Tailwind CSS via npm:
npm install -D tailwindcss postcss autoprefixer
- After installation, create the Tailwind configuration file by running the following command:
npx tailwindcss init
This command will create a tailwind.config.js
file in your project root, which allows you to customize your Tailwind setup.
Step 3: Configure Tailwind CSS
Next, we need to configure Tailwind CSS to work with our React project.
- Configure Tailwind in the
tailwind.config.js
file:
Open the tailwind.config.js
file that was just created and update the content
section to include your React files:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
This tells Tailwind to scan all your React files inside the src
folder and apply the necessary styles.
Step 4: Add Tailwind Directives to Your CSS
Now, let’s integrate Tailwind into your React app’s styles.
- Open the
src/index.css
file (which comes with Create React App by default), and replace its contents with the following Tailwind directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
These directives import Tailwind’s base styles, components, and utility classes into your project.
Step 5: Test Tailwind CSS
You’re almost done! To test that Tailwind CSS is working, let’s add some Tailwind utility classes to our App.js
file.
- Open
src/App.js
and modify it like this:
function App() {
return (
<div className="min-h-screen flex items-center justify-center bg-gray-100">
<h1 className="text-4xl font-bold text-blue-500">
Hello, React with Tailwind CSS!
</h1>
</div>
);
}
export default App;
Here, we’re using several Tailwind classes:
min-h-screen
: Ensures the div takes up the minimum height of the viewport.flex items-center justify-center
: Centers the content both vertically and horizontally.bg-gray-100
: Applies a light gray background.text-4xl font-bold text-blue-500
: Sets the text size, weight, and color.
Step 6: Run the Development Server
Now, let’s make sure everything is working. In your terminal, run:
npm start
If everything is configured correctly, your browser should display a centered, bold, blue heading that says “Hello, React with Tailwind CSS!” with a light gray background.
Step 7: Customizing Tailwind
Tailwind is incredibly customizable. You can extend its default theme by adding your custom colors, fonts, and other design tokens in the tailwind.config.js
file. For example, to add a custom color, you can modify the theme section:
module.exports = {
theme: {
extend: {
colors: {
primary: '#1D4ED8', // Custom blue color
},
},
},
}
Now you can use the class bg-primary
anywhere in your project to apply this custom color.
Conclusion
Congratulations! You’ve successfully set up a React project with Tailwind CSS. Now you can enjoy the best of both worlds – React’s powerful UI management and Tailwind’s flexible, utility-first approach to styling.
With this setup, you can rapidly build beautiful, responsive UIs without writing custom CSS from scratch.
What's next?
Explore more Tailwind utility classes to style your components.
Learn how to use Tailwind’s responsive modifiers to create mobile-friendly designs.
Customize your Tailwind config file to fit your project’s unique needs.
If you enjoyed this guide or have any questions, feel free to leave a comment.
~Happy coding with React and Tailwind!🧑💻
Subscribe to my newsletter
Read articles from Pranab K.S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranab K.S
Pranab K.S
I'm a passionate web developer with a love for coding challenges and a knack for making well-informed decisions. Proficient in a variety of web technologies, including HTML, CSS, JavaScript, jQuery, Node.js, Express.js, MongoDB and React. I'm an avid open source enthusiast dedicated to both learning and contributing to the developer community.