Remove Duplicates from the Sorted Array
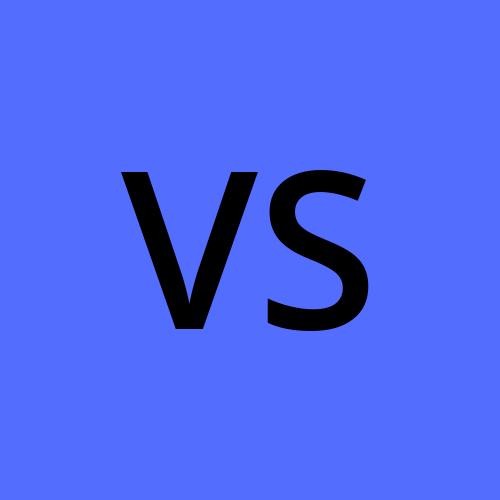
Remove Duplicate from Sorted Array:-
This problem flows the use of two pointer approach
To solve this we need to first develop the intuition behind this so let’s start building the intuition behind this problem.
Intuition building
Whenever we talk about sorted arrays we generally look for a two-pointer approach solution, This is a fairly simple thing that will come to mind when we move to solve this problem
When we think we will have two-pointers, we should know where to keep the first and second-pointers.
Now we know we have to keep these pointers at some index but now (where and why)
So let’s break this:-
We know we have to to match the current value along with the value present in the array what I mean we need to check whether the current value is present in the array or not. This will determine pointer 1 index must be at the starting index
Now the starting index we go next we need something that can iterate value, for that we need pointer 2 which will through the entire array
Logic Building
The pointer-1 index starts at 0.
The pointer-2 index starts at 0.
The loop will start with the pointer-2 from 0 to arr. length
whenever we find the value at the pointer-1 matching with the value at the pointer-2, we do nothing to let the for loop move ahead which ultimately will increase the pointer-2 value by 1
If the value at pointer-1 is not equal to the value at the pointer-2 then:-
we first increment the value of pointer-1
once the value of pointer-1 is increased then we assign the value present at pointer-2 to pointer-1 position (we can also say we swapped the values at this index)
The loop will continue doing these exchanges finally when pointer-2 exits the loop
The pointer-1 will tell me to what position we have the value which is unique.
Code
function getRemoveDuplicateSorted(arr) {
let i = 0;
for (let j = 1; j < arr.length; j++) {
if (arr[i] !== arr[j]) {
i++;
arr[i] = arr[j]
}
}
return arr.splice(0, i + 1)
}
function main() {
console.log(getRemoveDuplicateSorted([1, 1, 2, 2, 2, 3, 3]))
}
main();
You can read more from this link (Github)
Please do like and follow also if you find this helpful like or star the github link
Thanks
Subscribe to my newsletter
Read articles from Virat Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
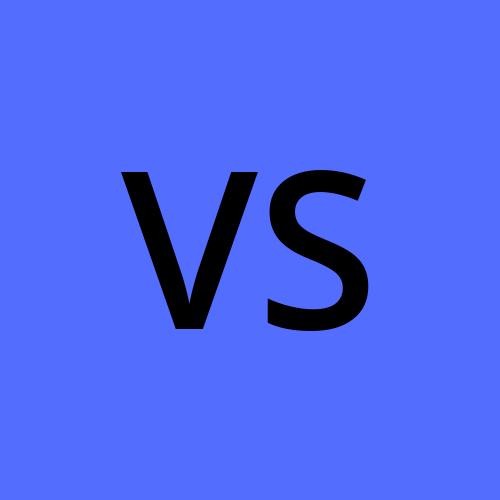