Understanding the Foundations of Programming: From Microprocessors to High-Level Languages
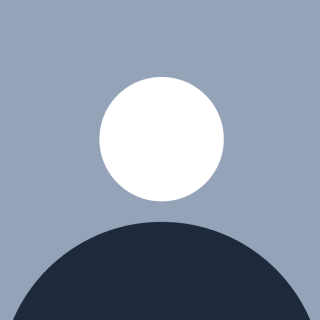
Table of contents
- Full Stack Java Development - Chapter 1: Fundamentals of Programming
- 2) Machine-Level Language (MLL)
- 3) Assembly-Level Language (ALL)
- 4) High-Level Language (HLL)
- What is High-Level Language?
- Example of HLL (Java Code)
- The Role of Compilers
- Visual: Conversion from HLL to MLL
- Relationship Between HLL, ALL, and MLL
- Why High-Level Languages?
- 5. Memory Hierarchy: RAM, Cache, HDD, SSD
- Memory Hierarchy in Computers
- Visual Representation:
- 6. Loading and Saving Programs
- Visual Representation: Program Loading and Saving Process
- Process Breakdown:
- 7. Buses: How Data Travels Between Components
- Visual Representation: Data Flow Between HDD, RAM, and CPU
- Process Breakdown:
- 8. Registers, Cache Memory, and RAM
- Example of Cache Memory Usage
- 9. Types of Files
- Visual Representation: File Lifecycle
- File Lifecycle Breakdown:
- 10. Linkers and Loaders
- Visual: Linking and Loading
- Explanation:
- Conclusion
- Acknowledgements
Full Stack Java Development - Chapter 1: Fundamentals of Programming
Introduction
Programming is the art of giving instructions to a machine. From the most basic binary code to high-level languages, computers have come a long way. This chapter dives into the core concepts that underpin all programming – from how microprocessors understand instructions to how human-friendly languages like Java work through compilers.
We'll explore:
Microprocessors and Transistors
Machine-Level Language (MLL)
Assembly-Level Language (ALL)
High-Level Language (HLL)
How Memory Works (HDD, RAM, Cache, SSD)
Loading and Saving Programs
Execution Cycles and Buses
1. Microprocessor and Semiconductor Technology
A microprocessor is a semiconductor device that processes instructions by understanding binary codes (0’s and 1’s). These binary codes correspond to voltage levels, typically 0 volts (low) and 5 volts (high). The transistors inside the microprocessor switch between these voltages to perform operations.
A Central Processing Unit (CPU) is the brain of a computer. It processes instructions, performs calculations, and manages data flow in the system. The CPU relies on semiconductor technology to operate, and it processes instructions in the form of binary codes (0’s and 1’s). The binary codes correspond to different voltage levels:
0 volts (representing a 0)
5 volts (representing a 1)
The CPU is composed of millions (or even billions) of transistors, which act as tiny switches that can be either on (allowing current to flow) or off (blocking current). These transistors allow the CPU to perform complex operations by manipulating voltage levels.
Transistors: NPN and PNP
Transistors form the fundamental building blocks of the CPU. They are used to control electrical currents, enabling logical and arithmetic operations. Two main types of transistors are commonly used: NPN and PNP transistors.
NPN Transistor
- An NPN transistor allows current to flow from the Collector to the Emitter when voltage is applied to the Base. When the base is activated (with a small current), it switches "on," allowing a larger current to flow through the circuit.
+---------------------+
| NPN Transistor |
|---------------------|
| | | |
| Base | Emitter | Collector |
+---------------------+
| ^
v |
- NPN transistors are widely used in logic circuits within the CPU for operations such as addition and comparison of binary numbers.
PNP Transistor
- A PNP transistor allows current to flow from the Emitter to the Collector when the Base is grounded (i.e., no voltage is applied). When the base is at a lower voltage than the emitter, the transistor switches "on," allowing current to pass.
+---------------------+
| PNP Transistor |
|---------------------|
| | | |
| Emitter | Base | Collector |
+---------------------+
v |
| ^
- PNP transistors work oppositely to NPN transistors and are used in situations where the circuit requires current to flow in the opposite direction.
How the CPU Uses Transistors to Perform Operations
The CPU uses NPN and PNP transistors to process binary instructions. These instructions consist of simple operations, such as addition, subtraction, multiplication, and logical comparisons, which are all performed using binary arithmetic (0’s and 1’s). Each operation is executed by switching the transistors on or off to manipulate the flow of current.
Example: Binary Addition in a CPU
Let’s see how binary addition works inside the CPU.
- Binary addition involves adding two binary numbers, much like decimal addition, but only using 0’s and 1’s. Transistors in the CPU help perform this addition by manipulating voltages (0V = 0, 5V = 1).
Example: Addition of 2 Binary Numbers
1010 (10 in decimal)
+ 0110 (6 in decimal)
----------
10000 (16 in decimal)
The CPU uses its transistors to process the binary input. For example, if we add
1010
(10) and0110
(6), the CPU switches the appropriate transistors to compute the result, which is10000
(16 in decimal).This process happens incredibly fast, as the transistors rapidly change states (on and off), allowing the CPU to handle millions of calculations per second.
Why Microprocessors Use Binary
The reason we use binary (0’s and 1’s) is that transistors inside the microprocessor can easily switch between two states: on or off, which correspond to low voltage (0) and high voltage (1). These two states are the simplest way to represent data, and the CPU is built to process this binary data efficiently.
By utilizing these transistors, the CPU can handle multiple complex instructions per second, whether it’s performing a simple addition or controlling more complex logic. The use of binary and transistors is what enables computers to understand and execute high-level code written by humans.
2) Machine-Level Language (MLL)
Machine-Level Language (MLL) is the most basic and fundamental language a computer understands. It communicates directly with the computer’s hardware, using binary digits (0’s and 1’s) to give instructions. Every operation is represented by a sequence of binary codes, with each bit controlling specific electrical signals in the processor.
Think of it as the native language of the CPU, where each instruction is made up of a combination of 0’s and 1’s, corresponding to different operations.
What is a Program?
A program is simply a series of machine-level instructions that tell the computer what to do. Each instruction performs a specific task, such as:
Adding two numbers
Moving data from one location to another
Comparing values
Each instruction consists of two parts:
Opcode – This specifies the operation (like ADD, SUB, etc.).
Operands – These specify the data or memory locations the operation should act on.
The program can have hundreds or even thousands of such binary instructions.
Visual Representation of Machine-Level Language
Below is a simplified visual representation of a program written in machine-level language. Each block represents a single instruction, written in binary, and the arrows show the flow of instructions.
+---------------------------+ +---------------------------+
| 1010 0101 (ADD) | ----> | 1100 0011 (Operand 1) |
+---------------------------+ +---------------------------+
|
v
+---------------------------+
| 1000 0001 (Operand 2) |
+---------------------------+
Here, the first instruction (1010 0101
) is the binary code for the operation ADD. The next two blocks are the operands, which are the binary representations of the two numbers being added.
Example: Machine-Level Instruction for Addition
Let's break down an example where the computer is told to add two numbers:
1010 0101 – This binary code represents the ADD operation.
1100 0011 – This is Operand 1 in binary (let's say it represents the number 195).
1000 0001 – This is Operand 2 in binary (let's say it represents the number 129).
When this program runs, the CPU takes these binary instructions and performs the operation. It adds the two binary numbers (195 + 129 = 324) and stores the result in a memory location.
Why Machine-Level Language?
Direct communication: Since MLL directly interacts with the hardware, it is the fastest and most efficient way to give instructions to a computer.
Complex for humans: Writing instructions in MLL is very complex and error-prone for humans, as it requires precise control over every aspect of the computer’s behavior.
Assembly and higher-level languages: To make programming easier, higher-level languages (like C, Java) were created, which are later translated into MLL by a compiler. This makes it easier for programmers to write code without worrying about low-level details.
By understanding the basics of Machine-Level Language, we can appreciate how far programming languages have evolved to help humans communicate complex instructions more efficiently!
3) Assembly-Level Language (ALL)
While Machine-Level Language (MLL) directly communicates with the hardware, it is too complex and difficult for humans to read and write. To make programming easier, Assembly-Level Language (ALL) was introduced.
Assembly language provides a more human-readable format for giving instructions to the computer, using mnemonics instead of binary. These mnemonics are short, easily recognizable codes that represent basic machine instructions.
What Are Mnemonics?
Mnemonics are symbolic representations of machine-level instructions. Instead of writing complex binary sequences like 1010 0101
, we use simple commands like MOV
, ADD
, and SUB
.
Example: Assembly Code vs. Machine Code
Here’s a simple example showing how Assembly-Level Language works:
MOV A, 5 – Move the value 5 into register A.
ADD A, B – Add the value stored in register B to the value in register A.
In Assembly, these instructions are easy to understand, and they correspond to machine-level operations, which the computer translates into binary.
+---------------------------+ +---------------------------+
| Assembly Language | ---> | Machine Language |
| (ADD, MOV, SUB, etc.) | | (Binary 0’s and 1’s) |
| Example: MOV A, 5 | | Example: 1010 0101 |
+---------------------------+ +---------------------------+
In the above visual:
Assembly Language (ALL) is easier for humans to understand.
The CPU converts these assembly instructions into Machine-Level Language (MLL) (binary code) to execute them.
Example Breakdown
Let’s go step-by-step through the example of assembly instructions:
MOV A, 5
This command tells the computer to move the value5
into a specific memory location (called register A). It's much more intuitive than writing the machine-level equivalent in binary.ADD A, B
This command tells the computer to add the value stored in register B to the value in register A. Again, using a mnemonic likeADD
is far simpler for humans than binary codes.
Visual: Assembly to Machine Language
Assembly Language is converted into Machine Language for the CPU to understand. Here’s a visual to demonstrate this transformation:
+---------------------+ +---------------------+
| Assembly Language | ---> | Machine Language |
| (ADD, MOV, etc.) | | (Binary 0's and 1's)|
+---------------------+ +---------------------+
In Assembly, the code is human-readable. Once the code is written, an Assembler converts it into binary code so that the CPU can process it. This is why Assembly Language is seen as a bridge between high-level languages (like C or Java) and Machine-Level Language.
Why Use Assembly-Level Language?
Efficiency: Assembly language allows for precise control over the hardware while still being easier to read and write than binary code.
Readable: It provides a more readable format compared to the zeros and ones of machine code.
Fast execution: Since it is close to machine code, programs written in Assembly run extremely fast.
Assembly-Level Language makes programming easier without sacrificing the control and performance of Machine-Level Language, making it a powerful tool in low-level programming.
4) High-Level Language (HLL)
As programming grew more complex, High-Level Languages (HLL) were developed to simplify the coding process. HLLs use syntax that is more understandable to humans, often resembling English commands or mathematical expressions. They make programming easier and faster by abstracting away the details of the computer's hardware.
What is High-Level Language?
High-Level Languages (HLLs) are designed to be easy for humans to read and write. They use symbols and English-like commands, which makes writing complex programs more intuitive.
Common Examples of HLLs:
Java
Python
C
Example of HLL (Java Code)
Here’s a simple example of a program written in Java, a popular high-level language:
int a = 5;
int b = 3;
int sum = a + b;
System.out.println(sum);
This program performs the following actions:
Defines two variables,
a
andb
.Calculates the sum of
a
andb
.Prints the result.
The Role of Compilers
Even though HLLs are easy for humans to understand, computers only understand binary code (Machine-Level Language). Therefore, a compiler is needed to translate HLL code into MLL so that the computer can execute it.
Visual: Conversion from HLL to MLL
Here's a visual representation of how High-Level Language is converted into Machine-Level Language:
+-----------------------+
| High-Level Language |
| (Java, Python, C) |
|-----------------------|
| int a = 5; |
| int b = 3; |
| int sum = a + b; |
| System.out.println(sum); |
+-----------------------+
|
v
+-----------------------+
| Compiler |
| (Translates HLL to MLL)|
+-----------------------+
|
v
+-----------------------+
| Machine-Level Language|
| (Binary 0's and 1's) |
|-----------------------|
| 1010 0101 (ADD) |
| 1100 0011 (Operand 1) |
| 1000 0001 (Operand 2) |
+-----------------------+
HLL: Human-readable code.
Compiler: Translates HLL to MLL.
MLL: Machine-readable binary code.
Relationship Between HLL, ALL, and MLL
High-Level Languages (HLLs) provide an easy and readable way to write programs, while Assembly-Level Languages (ALLs) and Machine-Level Languages (MLLs) operate closer to the hardware. Here's a visual showing their relationship:
+-----------------------+
| High-Level Language |
| (Java, Python, C) |
+-----------------------+
|
v
+-----------------------+
| Assembly-Level Language|
| (MOV, ADD, etc.) |
+-----------------------+
|
v
+-----------------------+
| Machine-Level Language|
| (Binary 0's and 1's) |
+-----------------------+
HLL: Abstracts complex operations into human-readable commands.
ALL: Provides a slightly lower-level, more detailed view but still uses mnemonics.
MLL: The lowest level of code, which directly controls the hardware.
Why High-Level Languages?
Simplicity: Easier to write, understand, and maintain compared to ALL and MLL.
Productivity: Allows for faster development of complex programs.
Portability: High-level languages can be used across different types of hardware with minimal changes.
By translating HLL code into MLL through a compiler, programmers can write complex software efficiently while still allowing the computer to execute it at the most fundamental level.
5. Memory Hierarchy: RAM, Cache, HDD, SSD
Memory Hierarchy in Computers
Computers use different types of memory to balance speed, size, and cost. Understanding these helps us see why certain parts of a computer are fast and expensive, while others are slower but can store a lot of data.
1. Hard Disk Drive (HDD)
What It Is: HDDs are a type of storage that keeps your data safe even when your computer is turned off. Think of it as a big, slow, but very reliable warehouse where all your files are kept.
Speed: HDDs are relatively slow when it comes to reading and writing data. Imagine having to find a specific book in a massive library—HDDs are like that library, where finding the book takes time.
Capacity: They offer a lot of storage space, so you can keep many files, photos, and programs.
Technology: They use spinning disks and magnetic technology to store data. Data is saved on the surface of these disks, and a moving arm reads or writes the data.
2. Random Access Memory (RAM)
What It Is: RAM is like your computer’s short-term memory. It holds data that the computer is currently using or processing. When the computer is turned off, everything in RAM disappears.
Speed: RAM is much faster than HDDs. It’s like having a desk where you can quickly grab and work with documents. RAM helps the computer to access data quickly, speeding up performance.
Capacity: RAM has limited space compared to HDDs. It’s used for data that needs to be accessed quickly, so you don’t need as much storage.
Technology: It uses semiconductor technology, which is faster and more efficient than the magnetic technology used in HDDs.
3. Cache Memory
What It Is: Cache is a small but very fast type of memory that sits close to the CPU (the brain of the computer). It holds data that the CPU frequently needs, so it can access this data extremely quickly.
Speed: Cache is the fastest memory in the computer. It’s like having a small, organized set of drawers right next to your desk for the most important documents you use often.
Capacity: Because it’s so fast, it’s also very small in size. It doesn’t store a lot of data, just the most important bits that need to be accessed right away.
Function: By keeping frequently used data close to the CPU, cache helps to speed up the overall performance of the computer.
4. Solid State Drive (SSD)
What It Is: SSDs are a type of storage that’s faster than HDDs but still retains data even when the computer is off. It’s like a more modern and speedy version of the HDD warehouse.
Speed: SSDs are much faster than HDDs. They use flash memory, which allows for quick access to data, similar to how RAM works but for storage.
Capacity: SSDs offer less storage space than HDDs but are improving. They are typically used for the operating system and frequently accessed files for faster performance.
Technology: SSDs use semiconductor technology, like RAM, which is why they are faster than HDDs. They don’t have moving parts, which makes them more durable and quicker.
Visual Representation:
+---------------------+
| Cache Memory | ---> Fastest, but very small storage
+---------------------+
^
|
+---------------------+
| RAM | ---> Fast, but less storage
+---------------------+
^
|
+---------------------+
| SSD | ---> Faster than HDD, moderate storage
+---------------------+
^
|
+---------------------+
| HDD | ---> Slower, large storage capacity
+---------------------+
In Summary:
Cache Memory is the quickest but stores the least data.
RAM is fast and used for current tasks but doesn’t retain data when powered off.
SSD is a faster storage solution compared to HDDs and is becoming more common for improving overall performance.
HDD is slower but offers large amounts of storage for files and applications.
This hierarchy helps computers run efficiently by using the right type of memory for the right job.
6. Loading and Saving Programs
1. Loading a Program
When you start a program on your computer, the following steps occur:
From HDD to RAM:
HDD (Hard Disk Drive): Your program (or software) is stored on the HDD, which is like a long-term storage space where files are kept even when the computer is off.
RAM (Random Access Memory): To run the program, it needs to be loaded into the RAM, which is much faster and allows the CPU to access the program’s data quickly. The data is moved from the HDD to RAM, making it ready for execution.
2. Executing a Program
- Microprocessor (CPU): Once the program is loaded into RAM, the CPU (or microprocessor) begins to execute it. The CPU performs calculations and processes instructions as defined by the program.
3. Saving Results
From RAM to HDD:
- HDD (Hard Disk Drive): If the program generates results or makes changes that need to be saved permanently, these results are written back to the HDD. This ensures that the data is stored long-term and will be available the next time you need it.
Visual Representation: Program Loading and Saving Process
+---------------------+ +---------------------+ +---------------------+
| Hard Disk (HDD) | ----> | RAM | ----> | Microprocessor |
| (Permanent Storage)| | (Temporary Storage) | | (Executes Program) |
| (Store File) | | (Load to Execute) | | (Execution) |
+---------------------+ +---------------------+ +---------------------+
Process Breakdown:
Storing Files:
- The program is saved on the HDD, where it remains until needed.
Loading to RAM:
- When you start the program, it’s loaded from the HDD into the RAM for quick access and execution.
Execution by CPU:
- The CPU reads and executes the instructions from the program loaded in RAM, performing the necessary tasks.
Saving Results:
- After execution, if there are results or changes that need to be saved, they are written back to the HDD.
This process illustrates how different types of memory work together to ensure programs run smoothly and results are stored permanently.
7. Buses: How Data Travels Between Components
1. What is a Bus?
- Definition: A bus is a set of electrical pathways (wires) that allows different parts of the computer to communicate with each other. It transmits data, instructions, and control signals between components such as the CPU, RAM, and HDD.
2. Components Involved:
Hard Disk (HDD): Stores data long-term. It is relatively slow compared to other components.
RAM (Random Access Memory): Holds data temporarily while a program is running. It is faster than the HDD.
Microprocessor (CPU): Executes instructions and processes data. It is the fastest component in the computer.
3. Data Flow Process:
From HDD to RAM: When a program needs to run, data is transferred from the HDD to the RAM via the bus. This allows the CPU to access and process the data quickly.
From RAM to CPU: The CPU reads and executes instructions stored in RAM. Data and instructions are moved between the CPU and RAM through the bus.
Saving Results: If the CPU needs to save data or results, they are written back to the HDD via the bus.
Visual Representation: Data Flow Between HDD, RAM, and CPU
+---------------------+ +---------------------+ +---------------------+
| Hard Disk (HDD) | ----> | RAM | ----> | Microprocessor |
| Magnetic Storage | | Main Memory (Fast) | | Executes Instructions|
| (Slow) | | (Faster) | | (Very Fast) |
+---------------------+ +---------------------+ +---------------------+
^ ^
| |
+---------------------+ |
| Bus | ---> Set of wires carrying instructions |
+---------------------+ |
Process Breakdown:
Data Transfer:
- The bus carries data and instructions between the HDD, RAM, and CPU. It enables communication between these components, allowing the computer to function effectively.
Speed Differences:
HDD is the slowest, so data needs to be transferred to RAM for faster processing.
RAM is faster than HDD but slower than the CPU. The bus ensures that data moves quickly between RAM and the CPU.
Execution and Storage:
- The CPU processes data and instructions received from RAM and writes results back to RAM or the HDD as needed. The bus handles the data transfer between these components.
In summary, the bus acts as the communication highway within the computer, ensuring that data and instructions are efficiently transmitted between storage, memory, and processing units.
8. Registers, Cache Memory, and RAM
1. Registers:
Definition: Registers are extremely small and fast storage locations inside the CPU.
Purpose: They hold temporary data and instructions that the CPU is currently processing.
Speed: Registers are the fastest type of memory because they are directly part of the CPU, which means data can be accessed almost instantly.
Capacity: They have very limited storage capacity, just enough to hold a few pieces of data or instructions.
2. Cache Memory:
Definition: Cache memory is a small, high-speed buffer between the CPU and RAM.
Purpose: It stores frequently accessed data and instructions so that they can be quickly retrieved by the CPU without having to go back to RAM.
Speed: Cache memory is faster than RAM but slower than registers. It acts as a middle ground, speeding up access to data that’s used often.
Levels: There are different levels of cache (L1, L2, L3), with L1 being the smallest and fastest, and L3 being larger but slower.
3. RAM (Random Access Memory):
Definition: RAM is the main memory where active program data and instructions are stored while the computer is running.
Purpose: It holds the data and instructions that are currently in use by the CPU, allowing for quick access and processing.
Speed: RAM is faster than storage devices like HDDs and SSDs but slower than cache memory.
Capacity: RAM has a larger capacity compared to registers and cache, allowing it to hold more data and programs.
Example of Cache Memory Usage
Scenario: Running a Loop
Imagine a program that runs a loop repeatedly. Each iteration of the loop involves executing the same set of instructions and accessing the same data. Here’s how cache memory helps in this scenario:
Initial Access:
The first time the loop runs, the instructions are fetched from RAM into cache memory.
The CPU retrieves these instructions from RAM and places them into the cache.
Subsequent Iterations:
For every subsequent iteration, the CPU accesses the instructions from the cache rather than fetching them from RAM again.
Since the cache is faster than RAM, accessing the instructions from cache speeds up the execution of the loop.
Visual Representation:
+-------------------+
| CPU Registers | ---> Fastest, very limited capacity
+-------------------+
^
|
+-------------------+
| Cache Memory | ---> Fast, temporary storage for frequently accessed data
+-------------------+
^
|
+-------------------+
| RAM | ---> Holds active data and instructions
+-------------------+
^
|
+-------------------+
| Hard Disk (HDD) | ---> Permanent storage (slowest access)
+-------------------+
In Summary:
Registers hold the most critical and immediate data needed by the CPU, providing the fastest access.
Cache Memory acts as a buffer, speeding up access to frequently used data and instructions by storing them close to the CPU.
RAM stores a larger amount of active data and instructions but is slower compared to cache.
This hierarchical setup ensures that the CPU has the fastest possible access to the data it needs, improving overall performance and efficiency.
9. Types of Files
1. Source File:
Definition: A source file contains human-readable code written in a programming language. It’s where developers write their programs.
File Extensions: Common extensions include
.java
(for Java),.c
(for C),.cpp
(for C++), and.py
(for Python).Purpose: This file is used to write and edit the program’s logic before it’s converted into machine-readable code.
2. Object File:
Definition: An object file is created after the source file is compiled. It contains machine code (binary code) that the CPU can understand but is not yet a complete program.
File Extensions: Common extensions include
.o
(on Unix-like systems) and.obj
(on Windows).Purpose: This file is an intermediate stage in the compilation process, and it may be linked with other object files to create an executable file.
3. Executable File:
Definition: An executable file is a final output file that is ready to be run by the CPU. It contains all the necessary machine code and is the program that can be executed.
File Extensions: Common extensions include
.exe
(on Windows),.out
(on Unix-like systems), and no extension or.elf
(for Linux executable files).Purpose: This file is what you run to execute the program. It has been fully compiled and linked, and it’s ready for execution.
Visual Representation: File Lifecycle
+---------------------+ +---------------------+ +---------------------+
| Source File | ---> | Object File | ---> | Executable File |
| (.java, .c, etc.) | | (Binary Code) | | (.exe, etc.) |
+---------------------+ +---------------------+ +---------------------+
File Lifecycle Breakdown:
Source File:
Creation: Written by developers in a high-level programming language.
Example:
program.java
ormain.c
.
Object File:
Compilation: The source file is compiled by a compiler into an object file containing machine code.
Example:
program.o
ormain.obj
.
Executable File:
Linking: The object file(s) are linked together, and any necessary libraries are included to produce the final executable file.
Execution: The executable file is then run by the CPU.
Example:
program.exe
ora.out
.
This lifecycle shows how source code written by humans is transformed through compilation and linking into a format that the computer can execute directly.
10. Linkers and Loaders
1. Linker:
Definition: A linker is a tool that combines one or more object files and libraries into a single executable file.
Function: It resolves references between object files and libraries, ensuring that all necessary code and data are correctly linked together.
Role:
Combining Object Files: If a program is split into multiple source files, each file is compiled into an object file. The linker merges these object files into a cohesive executable.
Linking Libraries: It also links external libraries (precompiled code) that the program uses. For example, if your program uses a standard math library, the linker will include the necessary code from that library.
Output: The result of the linking process is an executable file.
2. Loader:
Definition: A loader is a component of the operating system responsible for loading the executable file into RAM.
Function: It prepares the program for execution by placing it in the computer’s memory.
Role:
Loading into RAM: The loader reads the executable file from storage (e.g., HDD or SSD) and loads it into RAM, where it can be accessed and executed by the CPU.
Memory Management: It also sets up the necessary memory areas for the program, including data and stack areas.
Output: The result of the loading process is a program in RAM, ready for the CPU to execute.
Visual: Linking and Loading
+---------------------+ +---------------------+ +---------------------+
| Object Files | | Libraries | | Executable File |
| (.o, .obj) | | (.lib, .dll) | | (.exe, .out) |
+---------------------+ +---------------------+ +---------------------+
| | |
| | |
| v |
| +---------------------+ |
+------------> | Linker | <---------------+
| (Combines files, |
| resolves links) |
+---------------------+
|
v
+---------------------+
| Loader |
| (Loads executable |
| into RAM) |
+---------------------+
|
v
+---------------------+
| RAM |
| (Program ready to |
| be executed by |
| the CPU) |
+---------------------+
Explanation:
Object Files and Libraries:
Object Files: Compiled pieces of the program (e.g.,
file1.o
,file2.obj
).Libraries: Precompiled code that the program uses (e.g.,
libmath.lib
,libutils.dll
).
Linker:
Combines: The linker merges object files and libraries into a single executable file.
Resolves: It resolves references between different parts of the code and the libraries.
Executable File:
- Output: The result of the linking process. This file is ready to be loaded into RAM.
Loader:
Loads: The loader reads the executable file from storage and loads it into RAM.
Prepares: It sets up the necessary memory spaces for the program.
RAM:
- Program Ready: The executable file is now in RAM, where it can be accessed and executed by the CPU.
This visual representation illustrates how the program goes through the linking and loading stages, from being divided into object files and libraries to becoming an executable program ready for execution.
Conclusion
Understanding the fundamentals of programming—from how microprocessors process binary code to how human-readable instructions are converted and executed—forms the foundation of Full Stack Java Development.
This chapter covered the basics of microprocessors, programming languages, memory, and execution cycles. As we continue, we will dive deeper into Java-specific topics, building upon these core concepts.
Acknowledgements
Thank you for journeying through this extensive exploration of programming fundamentals. We’ve covered a wide range of topics, from the intricate workings of microprocessors to the evolution of programming languages and memory management. Understanding these foundational concepts is crucial for any full stack Java developer and forms the bedrock for further learning and development in the field.
Further Reading and Resources
If you found this post insightful, you might be interested in exploring more detailed topics in my ongoing series:
Full Stack Java Development: A comprehensive guide to becoming a full stack Java developer, covering everything from the basics to advanced concepts.
DSA in Java: Dive into data structures and algorithms in Java with detailed explanations and examples.
HashMap Implementation Explained: Understand the underlying mechanics of Java’s HashMap.
Inner Classes and Interfaces in Java: Explore the intricacies of inner classes and interfaces.
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repositories and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊