Case Study: Building react-form-interactions - A High-Performance React Library for Form State and Validation
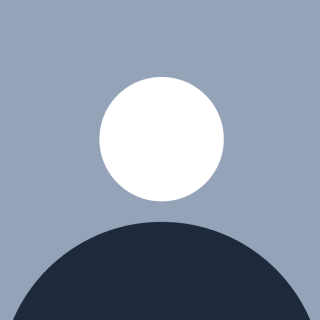
Project Overview
react-form-interactions
is a sophisticated and reusable library for managing form state and validation in React applications. The library is engineered to streamline the handling of form interactions, validations, and state updates. With an emphasis on performance and scalability, it simplifies complex form management tasks while maintaining high efficiency.
Challenges and Solutions
1. Preventing Unnecessary Re-renders
Challenge: One of the primary challenges was to handle state updates efficiently without triggering unnecessary re-renders. This was particularly important when form fields were updated but did not require immediate validation.
Solution: To address this, we implemented a debounced validation function using debouncedValidateField
. This function ensures that validation runs only after a brief delay, thus preventing excessive validations and re-renders during rapid input changes. The debounce technique helps in managing performance by batching updates and validations:
typescriptCopy codeimport { debounce } from "./debounce";
export const debouncedValidateField = <T>(
validationRulesConfig: Partial<Record<keyof T, ValidationRule<T>[]>>,
setFormState: React.Dispatch<React.SetStateAction<FormState<T>>>
): ((fieldName: keyof T, newState: FormState<T>) => void) => {
return debounce(
async (fieldName: keyof T, newState: FormState<T>) => {
const error = await validateField(
fieldName,
newState,
validationRulesConfig
);
// Update errors only if there's a change
if (newState.errors[fieldName] !== error) {
setFormState((prevState) => ({
...prevState,
errors: { ...prevState.errors, [fieldName]: error },
}));
}
},
300 // Debounce delay in milliseconds
);
};
2. Handling Real-time Form State Updates
Challenge: Another challenge was to ensure that form state updates in real-time while applying validation rules dynamically without causing immediate re-rendering.
Solution: We utilized the setFieldValue
function to manage form field values and trigger validation. This function integrates debounced validation, which helps in maintaining performance while keeping the form responsive:
typescriptCopy codeconst setFieldValue = useCallback(
(fieldName: keyof T, value: any) => {
setFormState((prevState) => {
const newValues = { ...prevState.values, [fieldName]: value };
const newState = { ...prevState, values: newValues };
// Debounce the validation call
debouncedValidateFieldFn(fieldName, newState);
return newState;
});
},
[debouncedValidateFieldFn]
);
3. Optimizing Validation Error Messaging
Challenge: Managing error messages effectively while avoiding frequent updates was crucial to prevent error message flickering and maintain a stable UI.
Solution: We applied a strategy to update error messages only if there is a change in validation results. This approach prevents unnecessary flickering and ensures a more stable user interface:
typescriptCopy codeif (newState.errors[fieldName] !== error) {
setFormState((prevState) => ({
...prevState,
errors: { ...prevState.errors, [fieldName]: error },
}));
}
4. Providing Comprehensive Form Reset and Field Reset
Challenge: Implementing a robust reset mechanism for both the entire form and individual fields was essential for ensuring a fresh state and optimal user experience.
Solution: We developed resetFormState
and resetFormField
functions to handle the resetting of form state and specific fields. These functions ensure that the form can be reset to its initial values efficiently:
typescriptCopy codeconst resetFormState = useCallback(() => {
setFormState(getDefaultFormState(initialValues));
}, [initialValues]);
const resetFormField = useCallback(
(fieldName: keyof T) => {
setFormState((prevState) => ({
...prevState,
values: { ...prevState.values, [fieldName]: initialValues[fieldName] },
errors: { ...prevState.errors, [fieldName]: "" },
touched: { ...prevState.touched, [fieldName]: false },
}));
},
[initialValues]
);
Key Technologies
TypeScript: Ensures type safety and robustness in the codebase.
React.js: Provides the foundation for building the user interface and managing component state.
Debounce: A utility function that helps to limit the rate of function execution, thereby enhancing performance during rapid input changes.
GitHub Repository
For more details and to explore the code, visit the react-form-interactions repository.
Subscribe to my newsletter
Read articles from Eaysin Arafat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Eaysin Arafat
Eaysin Arafat
Exploring the syntax of life through code and words.