Capturing Screenshots in React with html2canvas: A Detailed Guide

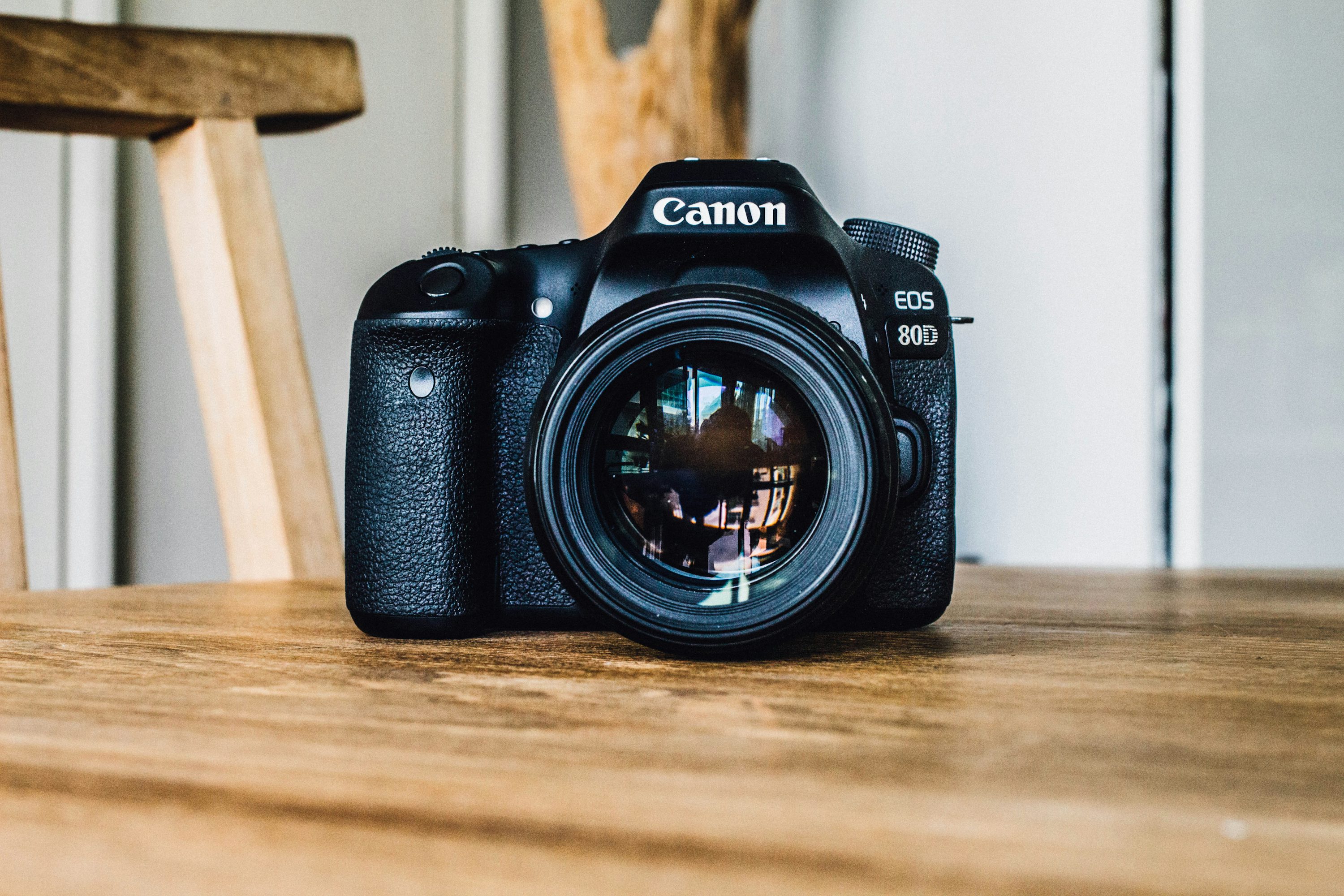
In modern web development, the ability to capture screenshots directly from the web application can be immensely valuable. Whether you want to generate PDFs, create shareable content, or save snapshots of user-generated content, html2canvas is a powerful library that allows you to capture screenshots of HTML elements.
In this blog, we will walk through the implementation of html2canvas in a React project, focusing on a practical example from a project that fetches and displays Hadith texts. We will cover:
Setting Up html2canvas in a React Project
Implementing Screenshot Functionality
Detailed Breakdown of the Example Code
Handling Edge Cases and Enhancements
1. Setting Up html2canvas in a React Project
First, you'll need to install html2canvas in your React project. You can do this using npm or yarn:
npm install html2canvas
or
yarn add html2canvas
2. Implementing Screenshot Functionality
Here's how you can implement a screenshot functionality using html2canvas:
Import html2canvas:
import html2canvas from 'html2canvas';
Create a function to capture the screenshot:
Define a function
downloadSnapshot
that uses html2canvas to take a screenshot of a specific HTML element and then triggers a download of the resulting image.downloadSnapshot = () => { // Hide elements that shouldn't be in the screenshot const elementsToHide = [ document.querySelector('.filter-button-container'), document.querySelector('.dropdown-container'), document.querySelector('.navbar-buttons') ]; elementsToHide.forEach(el => { if (el) el.classList.add('hide-for-snapshot'); }); const backgroundWrapper = document.querySelector('.background-wrapper'); if (backgroundWrapper) { backgroundWrapper.style.opacity = '1'; } this.setState({ isFlashing: true, watermark: true }, () => { setTimeout(() => { this.setState({ isFlashing: false }); html2canvas(document.querySelector('.app'), { backgroundColor: null, useCORS: true, scale: 3, width: document.querySelector('.app').offsetWidth, height: document.querySelector('.app').offsetHeight }).then(canvas => { const link = document.createElement('a'); link.href = canvas.toDataURL('image/png'); link.download = `${this.state.hadithbook}_${this.state.hadithid}.png`; document.body.appendChild(link); link.click(); document.body.removeChild(link); this.setState({ notification: 'Hadith has been downloaded.' }); setTimeout(() => { this.setState({ notification: '', watermark: false }); }, 3000); elementsToHide.forEach(el => { if (el) el.classList.remove('hide-for-snapshot'); }); if (backgroundWrapper) { backgroundWrapper.style.opacity = ''; } }); }, 300); }); };
Add a button to trigger the screenshot:
Ensure that you have a button or other UI element to trigger the screenshot functionality.
<button onClick={this.downloadSnapshot}>Download Screenshot</button>
3. Detailed Breakdown of the Example Code
In the provided example, we have a React component that fetches Hadith texts and displays them on a web page. Here’s a breakdown of the key parts related to html2canvas:
State Management: The component uses the state to manage various aspects like loading status, hadith content, and notification messages.
downloadSnapshot
Function: This function hides certain elements, applies a visual effect to indicate processing, and then captures a screenshot of the.app
element. After capturing, it triggers a download of the screenshot as a PNG file.Handling UI Changes: To ensure that the screenshot looks good, the function temporarily hides elements that should not appear in the screenshot and restores them afterward.
4. Handling Edge Cases and Enhancements
CORS Issues: Ensure that you configure the
useCORS
option in html2canvas to handle cross-origin images correctly.Dynamic Content: If your content changes dynamically (e.g., user interactions or real-time updates), make sure to update the screenshot function to capture the latest content.
Performance: For large or complex pages, consider optimizing the screenshot capture process to avoid performance issues.
User Experience: Provide feedback to users when the screenshot is being processed, and handle errors gracefully if something goes wrong.
By following these steps, you can effectively integrate screenshot functionality into your React projects using html2canvas. This can be a valuable feature for various applications, enhancing the user experience and providing additional functionality.
Subscribe to my newsletter
Read articles from Mohammed Omar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
