How to implement custom fonts for the Expo React Native app with Tailwind/Nativewind CSS?

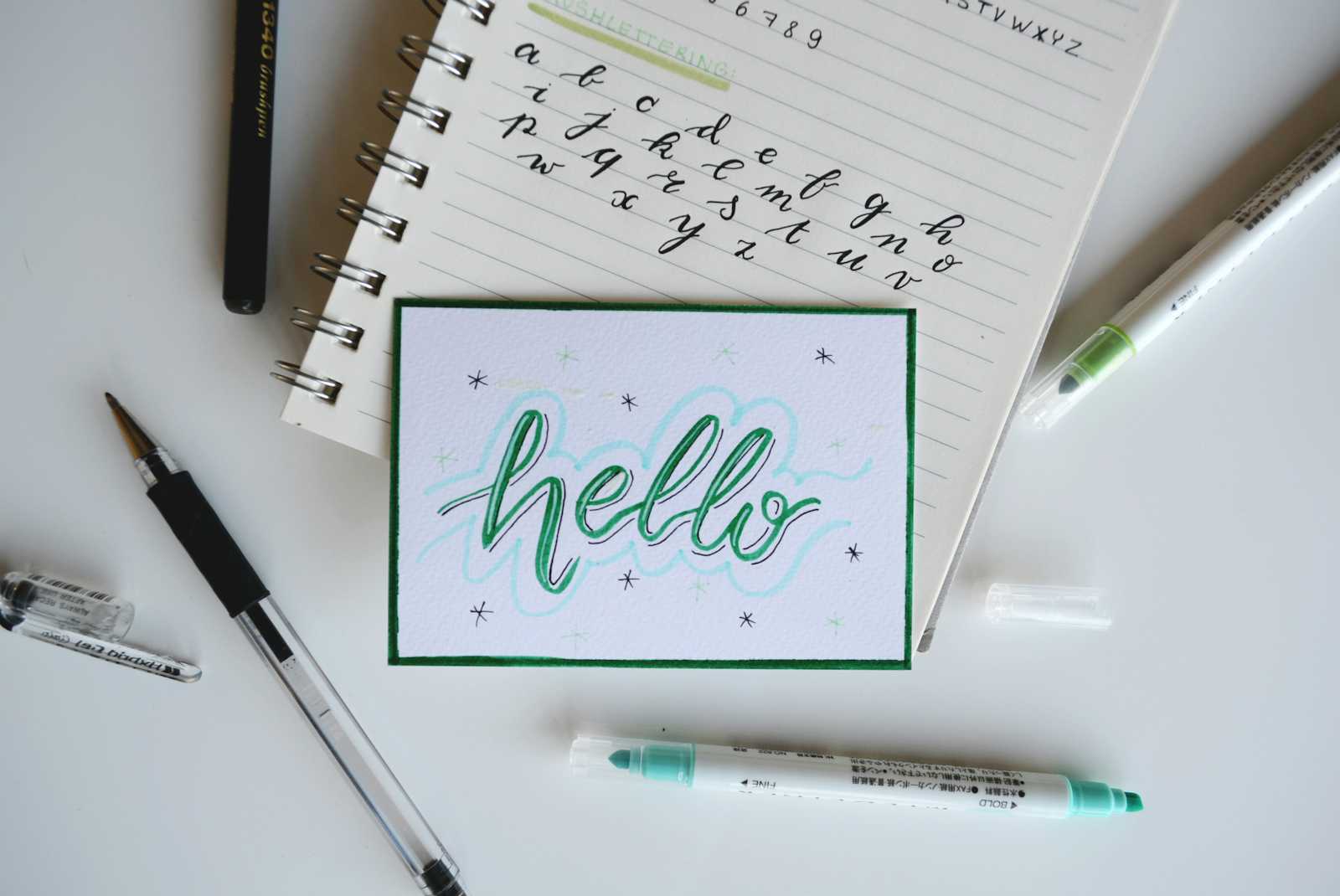
In mobile app development, aesthetics are key to creating a memorable user experience. Using custom fonts is one of the best ways to personalize and improve your app's look. Whether you want a modern, playful, or classic design, custom fonts can help define your app's identity and make it unique.
In this article, I will show you how to integrate custom fonts into your Expo React Native app with Tailwind CSS seamlessly. We'll cover everything from installing the necessary packages to loading and applying the fonts in your components.
Get the fonts
To implement custom fonts in your app, start by visiting the Google Fonts website, where you'll find a wide selection of fonts. If you're unsure which ones to pick, try browsing through the ‘Trending’ or ‘Most Popular’ sections for inspiration. Once you've chosen your fonts, download the zip file and extract its contents. Inside the extracted folder, locate the .ttf
files. Copy these font files and place them into the fonts
directory within the assets
folder of your Expo app.
Implement custom fonts in the app
In this section, we'll go through how to integrate custom fonts in an Expo React Native app and manage the splash screen during the font-loading process. I am using the PT Serif fonts from the Google Fonts.
To load and use custom fonts, we'll utilize the expo-font
package, which provides a convenient hook called useFonts
. Additionally, we'll use expo-splash-screen
to ensure that the app's splash screen remains visible until the fonts are fully loaded.
Here's the code snippet that demonstrates this process:
import React, { useEffect } from 'react';
import { useFonts } from 'expo-font';
import * as SplashScreen from 'expo-splash-screen';
export default function App() {
// Prevent the splash screen from auto-hiding
SplashScreen.preventAutoHideAsync();
const [fontsLoaded, error] = useFonts({
'PTSerif-Bold': require('../assets/fonts/PTSerif-Bold.ttf'),
'PTSerif-Regular': require('../assets/fonts/PTSerif-Regular.ttf'),
});
useEffect(() => {
if (error) {
console.error('Error loading fonts:', error);
throw error; // You might want to handle this error in a more user-friendly way
}
if (fontsLoaded) {
SplashScreen.hideAsync();
}
}, [fontsLoaded, error]);
if (!fontsLoaded) {
return null; // Render nothing until fonts are loaded
}
return (
// Your main app component goes here
);
}
Now we can use our custom fonts like this:
<View style={styles.container}>
<Text
style={{ fontFamily: 'PTSerif-Bold', fontSize: 30 }}>
This is PTSerif-Bold fonts.</Text>
<Text style={{ fontSize: 30 }}>Platform Default</Text>
</View>
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
Implement custom fonts with Nativewind CSS
Apart from this, we can implement the custom fonts with Tailwind CSS also assuming you already have implemented Nativewind CSS. If you haven’t installed Nativewind in your expo react-native app you can follow the step-by-step guide on their official guide here.
After implementing Nativewind in your app you just need to update the tailwind.config.js
file. I have named the PTSerif fonts PTBold
and PTRegular
for my convenience but you can give any name as we will use this in the className
property.
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ["./app/**/*.{js,jsx,ts,tsx}", "./components/**/*.{js,jsx,ts,tsx}"],
theme: {
extend: {
fontFamily:{
PTBold:['PTSerif-Bold','sans-serif'],
PTRegular:['PTSerif-Regular','sans-serif']
}
},
},
plugins: [],
}
Finally, we can use our custom fonts in the app like this:
import { Text, View } from "react-native";
export default function Index() {
return (
<View className="flex-1 items-center justify-center">
<Text className="font-PTBold text-2xl">This is my custom fonts.</Text>
</View>
);
}
Conclusion
Incorporating custom fonts into your Expo React Native app is a simple yet effective way to enhance its visual identity and user experience. By using expo-font
and managing the splash screen with expo-splash-screen
, you can ensure that your app looks polished and professional from the moment it's launched. With these tools, you can easily customize the typography to match your brand's style, making your app stand out to users.
Subscribe to my newsletter
Read articles from Hiren Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
