How to Use Axios for API Requests in Node.js
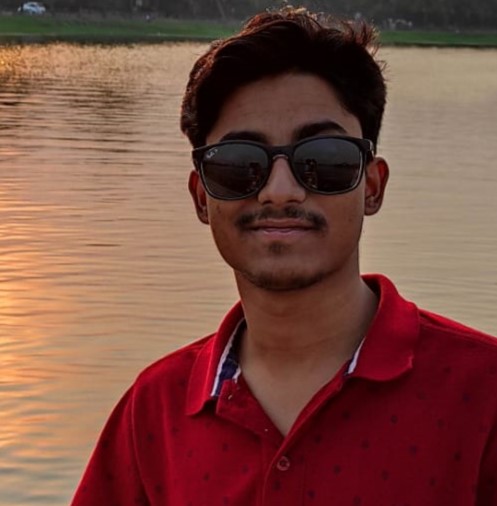
Table of contents
- What is Axios and Why Use It?
- How to Set Up Axios in an Express App
- Step 1: Install Axios
- Step 2: Basic Axios Example in an Express Server
- How This Code Works:
- Why Axios is a Great Tool for Server-Side API Calls
- Making Other Types of API Requests with Axios
- Making a POST Request
- Making a PUT Request
- Making a DELETE Request
- In a nutshell 🥜
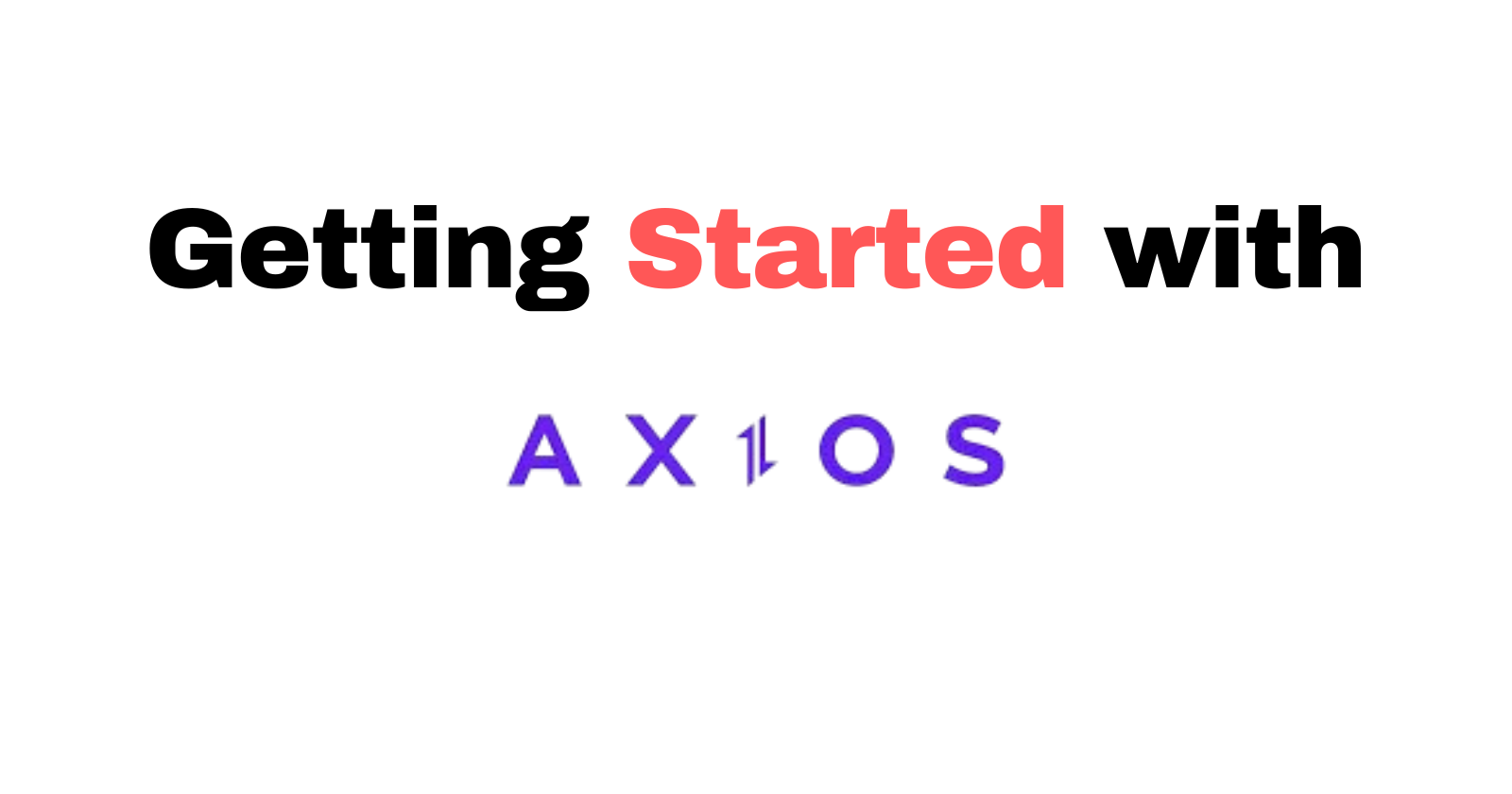
Yesterday, I learned Axios, a promise-based HTTP client, and it’s mainly used for making API requests from the server. If you're working with Node.js and Express backends, chances are you'll need to fetch or send data to other APIs. That’s where Axios steps in to make things easy!
In this post, I'll walk you through how Axios works and show you a simple example of making API requests from your Node.js server.
What is Axios and Why Use It?
If you're new to Axios, here's a quick rundown:
Axios is a popular JavaScript library used to make HTTP requests from the browser and Node.js.
It makes handling promises easier and provides simple methods for making requests (GET, POST, PUT, DELETE, etc.).
Axios handles both client-side and server-side requests, but in this post, we’ll focus on using it on the server-side with Node.js.
The key difference between making API requests with Axios and doing them in the browser or Postman is that Axios allows your server to make API calls to third-party services or other internal APIs in your app.
How to Set Up Axios in an Express App
Let’s dive into some code! Below is a simple boilerplate to get you started with Axios in your Node.js + Express project.
Step 1: Install Axios
Before we get started, you’ll need to install Axios in your project. In your terminal, run:
npm install axios
Step 2: Basic Axios Example in an Express Server
Here’s how you can use Axios to make API requests in a Node.js server:
You can use this as a template for your project.
import express from 'express';
import axios from 'axios';
const app = express();
const PORT = 3000;
app.use(express.json());
app.post('/endpoint', async (req, res) => {
try {
// Make a GET request to an external API
const response = await axios.get('YOUR_API_URL_HERE', {
params: {
// Example of sending parameters to the API
key1: req.body.key1,
key2: req.body.key2,
},
});
// Get the data from the API response
const data = response.data;
// Send the data back to the client
res.status(200).json(data);
} catch (error) {
// Handle errors if the API request fails
console.error('Error fetching data:', error);
res.status(500).json({ error: 'Failed to fetch data from the API' });
}
});
app.listen(PORT, () => {
console.log(`Server is running on <http://localhost>:${PORT}`);
});
How This Code Works:
Setting Up the Server:
- We start by importing Express and Axios and setting up the server with
express.json()
to handle JSON data.
- We start by importing Express and Axios and setting up the server with
Making the API Request:
Inside the
app.post
()
route, we make a GET request usingaxios.get()
to fetch data from a specified API URL.We use
req.body
to send parameters along with the request (likekey1
andkey2
).
Handling the Response:
- After the API responds, we grab the data from the response and send it back to the client using
res.status(200).json()
.
- After the API responds, we grab the data from the response and send it back to the client using
Error Handling:
- If there’s an issue with the API request (e.g., if the API is down or the URL is incorrect), we use
catch
to handle the error and respond with a500
status and an error message.
- If there’s an issue with the API request (e.g., if the API is down or the URL is incorrect), we use
Why Axios is a Great Tool for Server-Side API Calls
Promise-Based: Axios is built on promises, which means you can use
async/await
for cleaner, more readable code.Built-In Error Handling: Axios provides built-in support for error handling. You can use
try/catch
blocks to handle errors gracefully, like we did in the example above.Automatic JSON Transformation: Axios automatically transforms JSON responses and requests, making it easier to work with APIs that deal with JSON.
Easy to Use: The syntax for Axios is super simple. Whether you're making a
GET
,POST
,PUT
, orDELETE
request, it’s always a one-liner.
Making Other Types of API Requests with Axios
Besides GET requests, Axios can handle other types of HTTP requests like POST, PUT, and DELETE. Here’s how you can use Axios for these methods:
Making a POST Request
const response = await axios.post('YOUR_API_URL_HERE', {
key1: 'value1',
key2: 'value2',
});
Making a PUT Request
const response = await axios.put('YOUR_API_URL_HERE', {
key1: 'updatedValue1',
key2: 'updatedValue2',
});
Making a DELETE Request
const response = await axios.delete('YOUR_API_URL_HERE', {
params: { id: 'itemID' }
});
In a nutshell 🥜
Axios is a fantastic tool for simplifying API requests in your Node.js projects. Whether you need to send or fetch data from other services, Axios handles the heavy lifting with its clean syntax and powerful features.
To recap, here’s why you should consider Axios for your next project:
Simple and clean API request handling with promises and
async/await
.Easy to integrate with any Node.js and Express project.
Great error handling with built-in tools for catching and responding to errors.
If you’re working on an Express.js project and need to make API requests, give Axios a try. It makes server-side requests smoother and helps keep your code clean and readable.
That’s all for today! Happy coding! 🚀
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
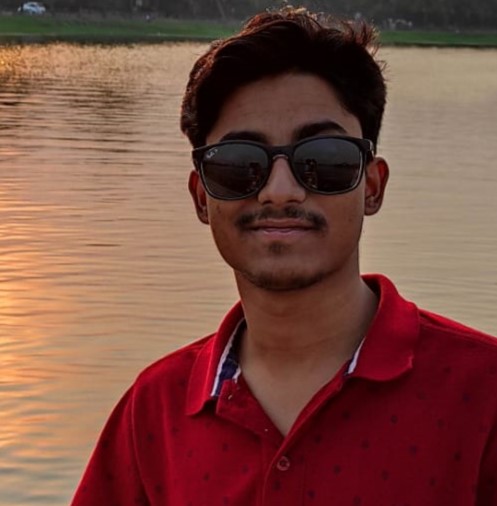
Arkadipta Kundu
Arkadipta Kundu
I’m a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, I’m focused on sharpening my DSA skills and expanding my expertise in Java development.