SuperFetch: The Next-Level HTTP Client Library
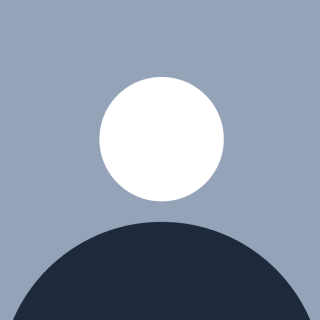
In the fast-paced world of web development, choosing the right tool for managing HTTP requests can significantly impact your application's performance and maintainability. While libraries like axios
and fetch
are popular choices, there's a new contender in town that promises to take data fetching to the next level: SuperFetch. In this article, we'll explore what sets SuperFetch apart, how to integrate it into your project, and how its advanced features can simplify your development process.
What is SuperFetch?
SuperFetch is an advanced JavaScript library designed to provide a superior alternative to traditional HTTP clients. It combines the simplicity of fetch
with advanced features like caching, automatic retries, and concurrency management, offering a comprehensive solution for handling network requests. Whether you're developing a complex web application or a simple project, SuperFetch aims to enhance your data-fetching capabilities and improve overall performance.
Why Choose SuperFetch?
Enhanced Performance with Caching
One of the standout features of SuperFetch is its built-in caching mechanism. Unlike traditional HTTP clients that make a network request every time data is needed, SuperFetch caches responses to minimize redundant requests. This not only speeds up your application by reducing latency but also reduces the load on your server. By configuring the cache settings, you can control how long data is stored and ensure that your application remains responsive and efficient.
Robust Error Handling with Automatic Retries
Network issues are an inevitable part of web development. SuperFetch addresses this with its automatic retry feature. Instead of failing silently or requiring manual intervention, SuperFetch can automatically retry failed requests based on configurable backoff strategies. This ensures that temporary network issues don't disrupt the user experience and provides a more resilient solution for handling unreliable connections.
Efficient Concurrency Management
Managing multiple concurrent requests can be challenging, especially when dealing with high traffic or complex applications. SuperFetch simplifies this with its concurrency management feature. You can set limits on the number of simultaneous requests, helping to prevent server overload and manage resources effectively. This feature is particularly useful in scenarios where you need to handle a large number of requests while maintaining optimal performance.
Flexible Interceptors for Custom Handling
SuperFetch offers a high degree of flexibility with its interceptor functionality. Interceptors allow you to modify requests and responses globally, providing a way to implement custom logic or handle common scenarios. For instance, you can add authentication headers to all requests, log response data, or handle errors in a centralized manner. This level of customization makes it easier to integrate SuperFetch into your existing workflows and maintain a consistent approach to data fetching.
Getting Started with SuperFetch
Installation and Setup
Getting started with SuperFetch is straightforward. Follow these steps to integrate it into your project:
Clone the Repository
Begin by cloning the SuperFetch repository from GitHub:
git clone https://github.com/Bahrul-Rozak/Super-Fetch.git cd Super-Fetch
Build the Library
Install the necessary dependencies and build the library:
npm install npm run build
The built library will be available in the
dist/
folder.Include in Your Project
For browser usage, include the built script in your HTML:
<script src="path/to/superfetch.min.js"></script>
For Node.js usage, require the built library:
const SuperFetch = require('./path/to/superfetch.min.js');
Basic Usage
SuperFetch is designed to be easy to use. Here's how you can make HTTP requests with SuperFetch in different environments:
In the Browser
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>SuperFetch Example</title>
<script src="path/to/superfetch.min.js"></script>
<script>
document.addEventListener('DOMContentLoaded', () => {
// Making a GET request
SuperFetch.get('https://jsonplaceholder.typicode.com/posts/1')
.then(data => console.log('GET Data:', data))
.catch(error => console.error('GET Error:', error));
// Making a POST request
SuperFetch.post('https://jsonplaceholder.typicode.com/posts', {
title: 'foo',
body: 'bar',
userId: 1
})
.then(response => console.log('POST Response:', response))
.catch(error => console.error('POST Error:', error));
});
</script>
</head>
<body>
</body>
</html>
In Node.js
const SuperFetch = require('./path/to/superfetch.min.js');
// Making a GET request
SuperFetch.get('https://jsonplaceholder.typicode.com/posts/1')
.then(data => console.log('GET Data:', data))
.catch(error => console.error('GET Error:', error));
// Making a POST request
SuperFetch.post('https://jsonplaceholder.typicode.com/posts', {
title: 'foo',
body: 'bar',
userId: 1
})
.then(response => console.log('POST Response:', response))
.catch(error => console.error('POST Error:', error));
Advanced Configuration
Global Settings
SuperFetch allows you to configure global settings for timeout, retries, and caching. Customize these settings to suit your needs:
const config = {
timeout: 5000, // Request timeout in milliseconds
retries: 3, // Number of retry attempts
cacheTTL: 60000, // Cache time-to-live in milliseconds
concurrencyLimit: 5 // Maximum concurrent requests
};
SuperFetch.setConfig(config);
Interceptors
Enhance your data-fetching logic with request and response interceptors:
SuperFetch.addRequestInterceptor((url, options) => {
// Add custom headers or modify the request options
options.headers['Authorization'] = 'Bearer your-token';
return { url, options };
});
SuperFetch.addResponseInterceptor(response => {
// Log or modify the response data
console.log('Response received:', response);
return response;
});
Real-World Use Cases
SuperFetch is designed to handle various scenarios and use cases effectively:
Single Page Applications (SPAs): Improve performance and user experience with caching and efficient data fetching.
Progressive Web Apps (PWAs): Handle offline scenarios and network reliability with automatic retries and caching.
Complex Data Dashboards: Manage multiple concurrent requests and display data efficiently with concurrency control.
Conclusion
SuperFetch is a game-changer for developers seeking a powerful, flexible, and efficient HTTP client library. With its advanced features and ease of use, it offers a significant improvement over traditional libraries like axios
and fetch
. By incorporating SuperFetch into your project, you can enhance performance, manage network requests more effectively, and provide a better user experience.
Explore SuperFetch today and see how it can transform your data-fetching approach! For more details, visit the SuperFetch GitHub repository.
Subscribe to my newsletter
Read articles from Bahrul Rozak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Bahrul Rozak
Bahrul Rozak
Software Developer who love JavaScript Ecosystem