Mastering the io Package in Go: A Detailed Look

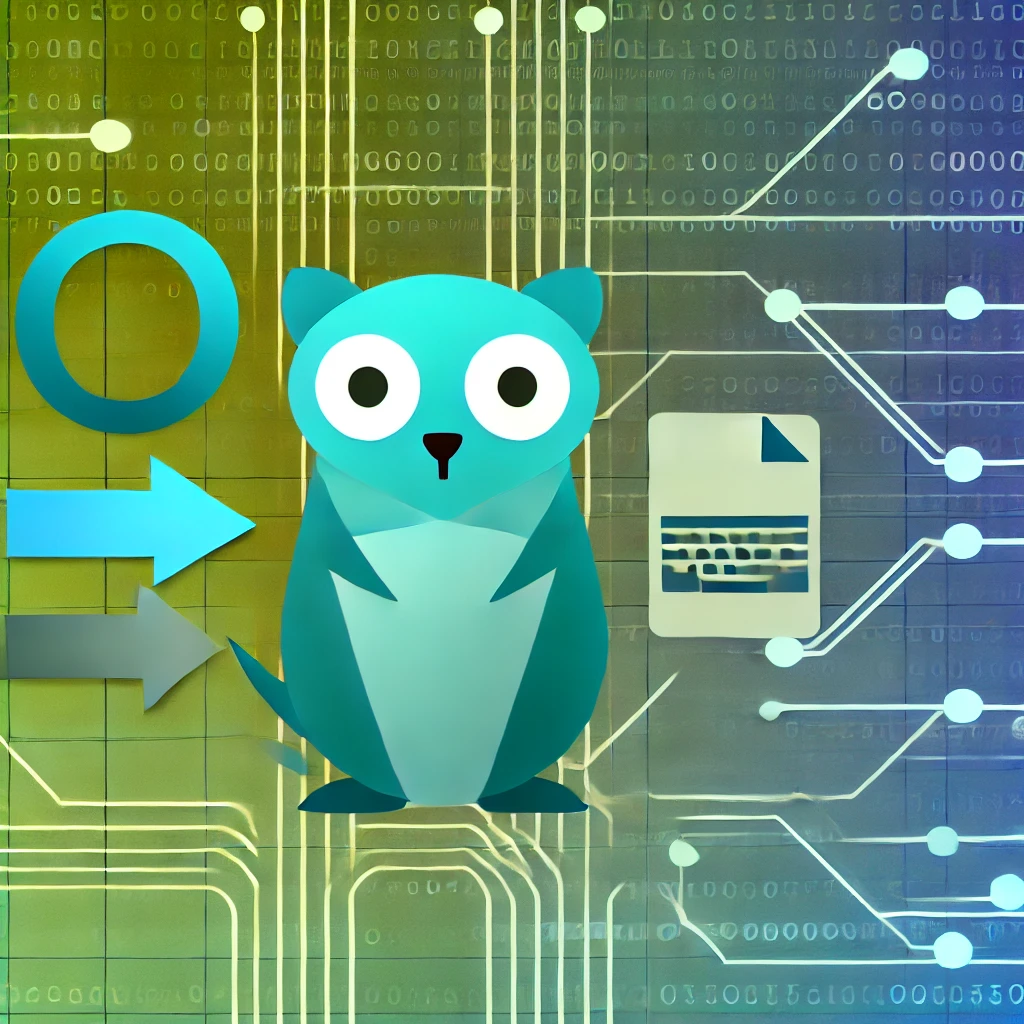
Welcome! Building on your understanding of Go's basics and the os
package, let's delve into the io
package—a cornerstone of Go's standard library. This guide will provide an in-depth exploration of the io
package, covering its fundamental interfaces, utility functions, and best practices. Let's get started!
Table of Contents
Introduction to the io
Package
The io
package in Go provides basic interfaces for I/O primitives, facilitating the reading and writing of byte streams. It is designed to:
Abstract data sources and destinations, allowing for flexible and interchangeable components.
Provide a common foundation for input/output operations across the standard library and third-party packages.
Enable developers to write generic code that can operate on any data stream implementing the core interfaces.
By understanding the io
package, you'll be able to harness Go's powerful I/O capabilities, write more modular code, and effectively handle data from various sources such as files, network connections, in-memory buffers, and more.
Core Interfaces in io
Reader Interface
The io.Reader
interface represents the ability to read data.
type Reader interface {
Read(p []byte) (n int, err error)
}
Method:
Read(p []byte) (n int, err error)
: Reads up tolen(p)
bytes intop
. It returns the number of bytes read (n
) and any error encountered.
Behavior:
Blocks until at least one byte is read, an error occurs, or EOF (end-of-file) is reached.
Returns
n > 0
if any data was read successfully.Returns
err == io.EOF
when no more data is available.
Example Usage:
func ReadFromReader(r io.Reader) error {
buf := make([]byte, 1024)
for {
n, err := r.Read(buf)
if n > 0 {
// Process data in buf[:n]
fmt.Printf("Read %d bytes: %s\n", n, string(buf[:n]))
}
if err != nil {
if err == io.EOF {
break
}
return err
}
}
return nil
}
Writer Interface
The io.Writer
interface represents the ability to write data.
type Writer interface {
Write(p []byte) (n int, err error)
}
Method:
Write(p []byte) (n int, err error)
: Writeslen(p)
bytes fromp
to the underlying data stream. Returns the number of bytes written and any error encountered.
Behavior:
Writes may not accept all bytes in a single call;
n
may be less thanlen(p)
.Should return a non-nil error when
n < len(p)
.
Example Usage:
func WriteToWriter(w io.Writer, data []byte) error {
total := 0
for total < len(data) {
n, err := w.Write(data[total:])
if err != nil {
return err
}
total += n
}
return nil
}
Closer Interface
The io.Closer
interface represents the ability to close a data stream.
type Closer interface {
Close() error
}
Method:
Close() error
: Closes the underlying resource, releasing any associated resources.
Usage:
- Commonly used with files, network connections, and other resources that need explicit closure.
Seeker Interface
The io.Seeker
interface allows moving the read/write cursor within a data stream.
type Seeker interface {
Seek(offset int64, whence int) (int64, error)
}
Method:
Seek(offset int64, whence int) (int64, error)
: Sets the offset for the nextRead
orWrite
tooffset
, interpreted according towhence
.
Whence Values:
0
(io.SeekStart
): Relative to the origin of the file.1
(io.SeekCurrent
): Relative to the current offset.2
(io.SeekEnd
): Relative to the end.
Example Usage:
func SeekAndRead(r io.ReadSeeker) error {
// Seek to the beginning
_, err := r.Seek(0, io.SeekStart)
if err != nil {
return err
}
buf := make([]byte, 100)
n, err := r.Read(buf)
if err != nil {
return err
}
fmt.Printf("Read %d bytes: %s\n", n, string(buf[:n]))
return nil
}
Composed Interfaces
ReadWriter Interface
type ReadWriter interface {
Reader
Writer
}
Represents something that can both read and write.
ReadCloser, WriteCloser, ReadWriteCloser
io.ReadCloser
: Combinesio.Reader
andio.Closer
.io.WriteCloser
: Combinesio.Writer
andio.Closer
.io.ReadWriteCloser
: Combinesio.Reader
,io.Writer
, andio.Closer
.
These interfaces are used to represent resources that need to be closed after use, such as files and network connections.
Example Usage:
func ProcessData(rwc io.ReadWriteCloser) error {
defer rwc.Close()
// Read from rwc
// Write to rwc
return nil
}
Utility Functions in io
The io
package provides several utility functions that operate on the core interfaces.
Copy and CopyN
io.Copy()
Copies from a Reader
to a Writer
until EOF
or an error occurs.
func Copy(dst Writer, src Reader) (written int64, err error)
Example Usage:
func CopyData(dst io.Writer, src io.Reader) error {
_, err := io.Copy(dst, src)
return err
}
io.CopyN()
Copies n
bytes from a Reader
to a Writer
.
func CopyN(dst Writer, src Reader, n int64) (written int64, err error)
ReadFull and ReadAtLeast
io.ReadFull()
Reads exactly len(buf)
bytes from Reader
into buf
.
func ReadFull(r Reader, buf []byte) (n int, err error)
- Returns
io.ErrUnexpectedEOF
if theReader
reachesEOF
before filling the buffer.
io.ReadAtLeast()
Reads at least min
bytes into buf
.
func ReadAtLeast(r Reader, buf []byte, min int) (n int, err error)
- Returns
io.ErrUnexpectedEOF
if fewer thanmin
bytes are read.
TeeReader
io.TeeReader
returns a Reader
that writes to a Writer
what it reads from another Reader
.
func TeeReader(r Reader, w Writer) Reader
Use Case:
- Reading data while simultaneously writing it elsewhere (e.g., logging or hashing input data).
Example Usage:
func ReadAndLog(r io.Reader) error {
var buf bytes.Buffer
tee := io.TeeReader(r, &buf)
data, err := io.ReadAll(tee)
if err != nil {
return err
}
fmt.Println("Original Data:", string(data))
fmt.Println("Logged Data:", buf.String())
return nil
}
MultiReader and MultiWriter
io.MultiReader()
Combines multiple Reader
s into a single Reader
.
func MultiReader(readers ...Reader) Reader
Example Usage:
func ConcatenateReaders(r1, r2 io.Reader) io.Reader {
return io.MultiReader(r1, r2)
}
io.MultiWriter()
Writes to multiple Writer
s simultaneously.
func MultiWriter(writers ...Writer) Writer
Example Usage:
func WriteToMultiple(w io.Writer, data []byte) error {
mw := io.MultiWriter(os.Stdout, w)
_, err := mw.Write(data)
return err
}
Pipe
io.Pipe
creates a synchronous in-memory pipe.
func Pipe() (*PipeReader, *PipeWriter)
PipeReader
andPipeWriter
implementio.Reader
andio.Writer
respectively.Useful for connecting code expecting a
Reader
with code expecting aWriter
.
Example Usage:
func UsePipe() error {
pr, pw := io.Pipe()
go func() {
defer pw.Close()
pw.Write([]byte("Data sent through pipe"))
}()
buf := make([]byte, 1024)
n, err := pr.Read(buf)
if err != nil {
return err
}
fmt.Println("Received:", string(buf[:n]))
return nil
}
Working with Buffers
While not part of the io
package, bytes.Buffer
and bufio
are closely related and frequently used with io
interfaces.
Using bytes.Buffer
bytes.Buffer
is an in-memory buffer that implements io.Reader
, io.Writer
, and io.ByteScanner
.
Example Usage:
func UseBuffer() {
var buf bytes.Buffer
buf.WriteString("Hello, ")
buf.Write([]byte("World!"))
fmt.Println(buf.String()) // Outputs: Hello, World!
// Read from buffer
data := make([]byte, buf.Len())
buf.Read(data)
fmt.Println(string(data)) // Outputs: Hello, World!
}
Using bufio
Package
The bufio
package provides buffered Reader
and Writer
implementations, improving efficiency by reducing the number of system calls.
bufio.Reader
func UseBufioReader(r io.Reader) {
reader := bufio.NewReader(r)
line, err := reader.ReadString('\n')
if err != nil {
// Handle error
}
fmt.Println("Read line:", line)
}
bufio.Writer
func UseBufioWriter(w io.Writer) {
writer := bufio.NewWriter(w)
writer.WriteString("Buffered Write\n")
writer.Flush() // Ensure data is written to the underlying writer
}
Error Handling in io
EOF and Unexpected EOF
io.EOF
: Indicates that no more data is available; not considered an error in some contexts.io.ErrUnexpectedEOF
: Occurs whenEOF
is encountered before the expected amount of data is read.
Example Handling:
n, err := r.Read(buf)
if err != nil {
if err == io.EOF {
// Handle end-of-file condition
} else {
// Handle other errors
}
}
Sentinel Errors
The io
package defines several sentinel errors:
io.EOF
io.ErrClosedPipe
io.ErrNoProgress
io.ErrShortBuffer
io.ErrShortWrite
io.ErrUnexpectedEOF
These can be compared directly using ==
to handle specific conditions.
Examples and Use Cases
Implementing Custom Readers and Writers
Custom Reader
type MyReader struct {
data []byte
pos int
}
func (r *MyReader) Read(p []byte) (n int, err error) {
if r.pos >= len(r.data) {
return 0, io.EOF
}
n = copy(p, r.data[r.pos:])
r.pos += n
return n, nil
}
Usage:
func main() {
r := &MyReader{data: []byte("Hello, Custom Reader!")}
buf := make([]byte, 8)
for {
n, err := r.Read(buf)
if n > 0 {
fmt.Print(string(buf[:n]))
}
if err == io.EOF {
break
} else if err != nil {
fmt.Println("Error:", err)
break
}
}
}
Custom Writer
type MyWriter struct {
data []byte
}
func (w *MyWriter) Write(p []byte) (n int, err error) {
w.data = append(w.data, p...)
return len(p), nil
}
Usage:
func main() {
w := &MyWriter{}
w.Write([]byte("Hello, "))
w.Write([]byte("Custom Writer!"))
fmt.Println(string(w.data)) // Outputs: Hello, Custom Writer!
}
Reading from Various Sources
Reading from a File
func ReadFromFile(filename string) error {
file, err := os.Open(filename)
if err != nil {
return err
}
defer file.Close()
return ReadFromReader(file)
}
Reading from a Network Connection
func ReadFromConnection(conn net.Conn) error {
defer conn.Close()
return ReadFromReader(conn)
}
Reading from a String
Use strings.NewReader
to create a Reader
from a string.
func ReadFromString(s string) error {
r := strings.NewReader(s)
return ReadFromReader(r)
}
Writing to Various Destinations
Writing to a File
func WriteToFile(filename string, data []byte) error {
file, err := os.Create(filename)
if err != nil {
return err
}
defer file.Close()
return WriteToWriter(file, data)
}
Writing to a Network Connection
func WriteToConnection(conn net.Conn, data []byte) error {
defer conn.Close()
return WriteToWriter(conn, data)
}
Writing to a Buffer
func WriteToBuffer(data []byte) (*bytes.Buffer, error) {
var buf bytes.Buffer
err := WriteToWriter(&buf, data)
if err != nil {
return nil, err
}
return &buf, nil
}
Best Practices
Use Interfaces for Abstraction:
Accept
io.Reader
andio.Writer
in function parameters to allow for flexibility and testability.Return interfaces when appropriate to abstract implementation details.
Handle Errors Properly:
Always check and handle errors returned by
Read
,Write
, and other methods.Be mindful of partial reads and writes.
Buffering for Efficiency:
- Use
bufio.Reader
andbufio.Writer
when dealing with small reads and writes to improve performance.
- Use
Close Resources:
- Close any resources that implement
io.Closer
usingdefer
to ensure they are released properly.
- Close any resources that implement
Understand Blocking Behavior:
- Be aware that
Read
andWrite
operations may block, especially when dealing with network connections.
- Be aware that
Use Utility Functions:
- Leverage functions like
io.Copy
,io.CopyN
,io.ReadFull
, and others to simplify code and handle common patterns.
- Leverage functions like
Implement Interfaces Thoughtfully:
- When creating custom types that implement
io
interfaces, ensure they conform to the expected behaviors and handle edge cases.
- When creating custom types that implement
Avoid Unnecessary Data Copies:
- When possible, operate directly on streams to minimize memory usage and improve performance.
Document Behavior:
- Clearly document how your functions and types interact with the
io
interfaces, especially regarding error handling and partial reads/writes.
- Clearly document how your functions and types interact with the
Conclusion
The io
package is fundamental to Go's approach to input and output operations. By mastering its interfaces and utilities, you can write flexible, efficient, and modular code that interacts seamlessly with various data sources and destinations.
Understanding the io
package enables you to:
Build applications that can read from and write to files, network connections, buffers, and more.
Implement custom readers and writers tailored to your application's needs.
Leverage powerful utilities to simplify data transfer and processing.
Write code that is easy to test and maintain due to its adherence to standard interfaces.
Additional Resources
Official Documentation:
Go by Example:
Blogs and Tutorials:
Books:
- The Go Programming Language by Alan A. A. Donovan and Brian W. Kernighan (Chapter on Interfaces)
Keep experimenting with the io
package and its interfaces to deepen your understanding and enhance your Go programming expertise! By doing so, you'll be well-equipped to build robust, efficient, and scalable applications.
Subscribe to my newsletter
Read articles from Sundaram Kumar Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sundaram Kumar Jha
Sundaram Kumar Jha
I Like Building Cloud Native Stuff , the microservices, backends, distributed systems and cloud native tools using Golang