Day 1: Setting Up a React Demo Project in VS Code and STS

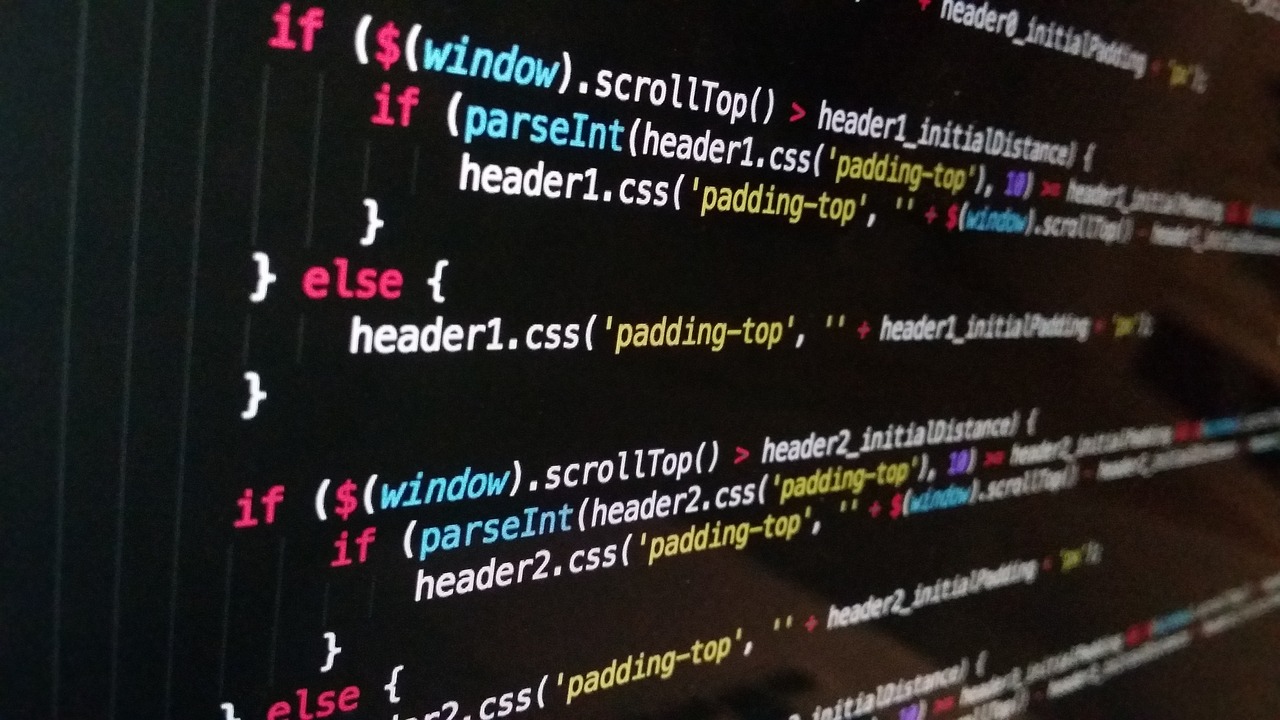
Welcome to my project journey blog, where I’ll document my experiences and challenges while working on a Spring Boot and React REST API architecture. For Day 1, we’ll start by setting up a React demo project using VS Code and Spring Tool Suite (STS). Here’s a guide to getting started, along with the project structure I’ll be following.
Step 1: Creating a React App
To kick things off, let’s create a new React app. Open your terminal and run the following command:
npx create-react-app demo-app
cd demo-app
Once you’ve set up your project, open it in VS Code or STS.
Step 2: Installing Necessary Dependencies
For this project, we’ll be using several key libraries to streamline development. Here’s what we’ll install:
npm install formik react-router-dom @mui/material axios yup sweetalert2
Formik: Helps with form handling and validation.
react-router-dom: Enables routing for our app.
MUI (Material UI): Provides UI components to help speed up frontend development.
Axios: For making HTTP requests.
Yup: For schema-based form validation.
SweetAlert2 (Optional): For beautiful, customizable alerts.
Step 3: Structuring the Project
Now that we have the app set up, let’s structure it for better scalability and maintainability. Here’s how we’ll organize our folders inside the src
directory:
src
│
├── Assets
├── Common
│ ├── Apiclient
│ ├── Header
│ ├── Footer
│ ├── Layout
│ ├── Strings
│ ├── Themes
│ ├── Utils
│ └── Urls
├── Components
├── Pages
├── Routes
└── Validations
Why This Structure?
Assets: For images, fonts, and other static files.
Common: Contains reusable utilities and layouts. For instance,
Apiclient.js
helps us manage HTTP requests, andUrls.js
stores all API endpoint URLs.Components: Houses small, reusable components like buttons, forms, etc.
Pages: Contains the core pages or views of the application.
Routes: Handles the routing between different pages.
Validations: Centralizes Yup schemas for validation across forms.
Step 4: Understanding Apiclient.js
Apiclient.js is a critical part of our project. It manages HTTP requests using Axios and handles token-based authentication. Here’s a breakdown of what it does:
Axios Configuration: We create an instance of Axios to centralize our API requests.
Token Handling: We fetch and include the authentication token in the headers of every request, ensuring secure API communication.
Rate Limiting: We call a specific endpoint (
rateLimitTokenGenerate
) to check rate limits, ensuring that our API requests remain within limits and avoid throttling.
Having a centralized API client helps in keeping our code clean and reduces redundancy in handling HTTP requests across different components. By setting up interceptors, we automatically append tokens to outgoing requests, which is crucial for secure and efficient API consumption.
Conclusion
On Day 1, we’ve successfully set up our React app, installed essential libraries, and laid the groundwork for our project structure. This structure will allow for clean, maintainable code, as well as scalable development practices. Stay tuned for Day 2, where we’ll dive deeper into building reusable components and implementing routing.
This is just the beginning of my journey with Spring Boot and React in this project. Keep following for more updates as I progress!
Subscribe to my newsletter
Read articles from Sivanand GKamath directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
