How to Use Python for Natural Language Processing (NLP)
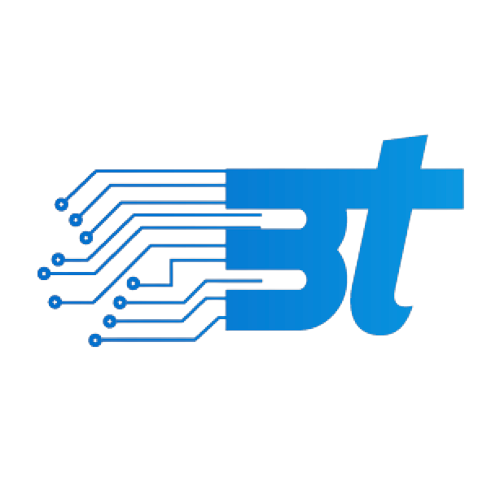
Table of contents
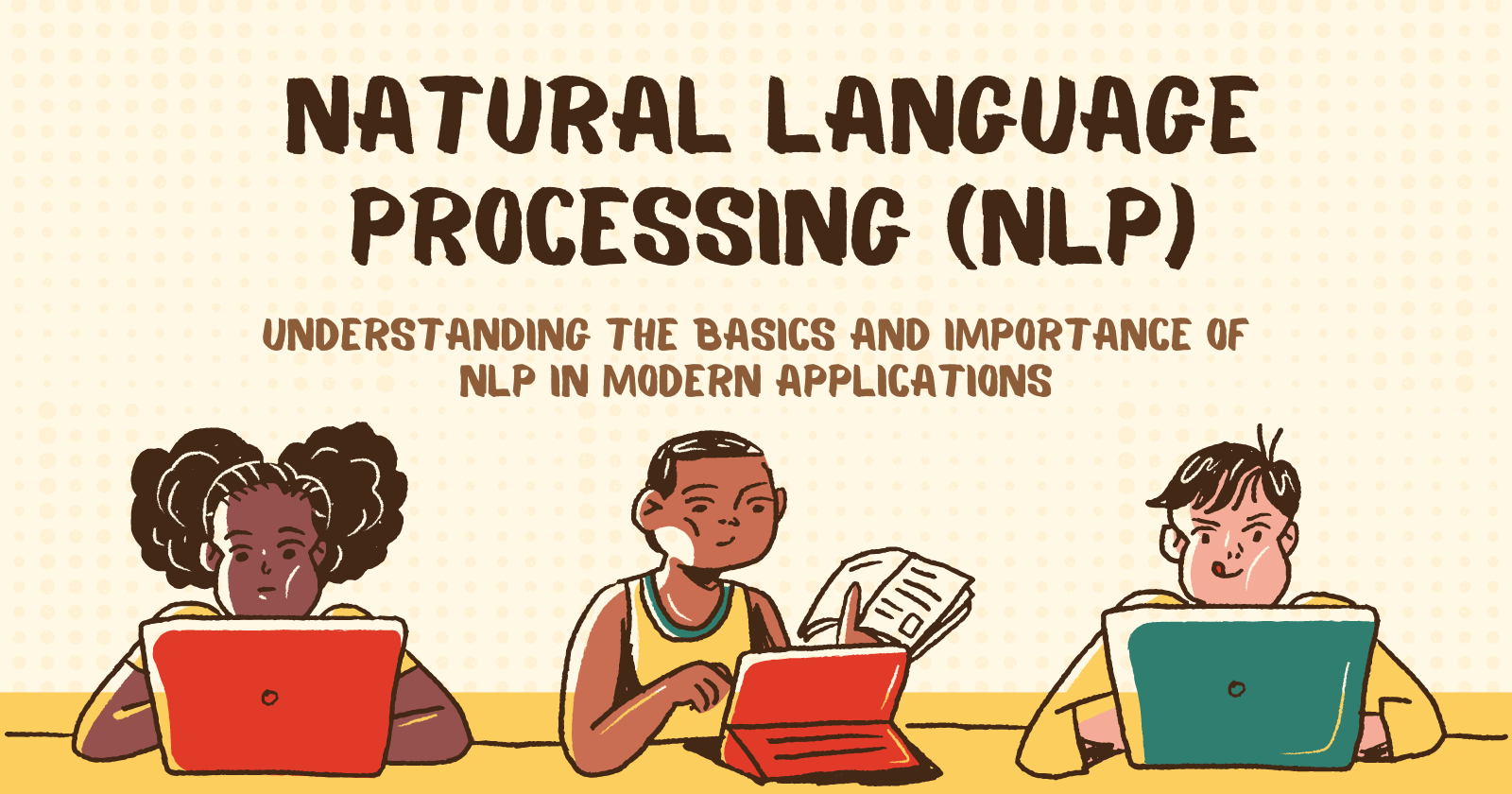
Natural Language Processing (NLP) is a branch of artificial intelligence that focuses on enabling computers to understand, interpret, and respond to human language. NLP has applications in various fields, such as chatbots, sentiment analysis, machine translation, and more. Python is one of the most popular languages for NLP due to its robust libraries and ease of use.
In this blog, we’ll explore the basics of NLP with Python, covering key concepts and providing step-by-step examples using popular libraries such as NLTK, spaCy, and TextBlob.
1. Understanding NLP Concepts
Before diving into the code, it’s essential to grasp a few fundamental NLP concepts:
Tokenization: Breaking down text into smaller pieces like words or sentences.
Stop Words: Common words (e.g., "the", "is", "in") that are usually removed to focus on more meaningful terms.
Stemming and Lemmatization: Reducing words to their root or base form. For example, "running" becomes "run."
Bag of Words (BoW): A representation of text where each word corresponds to a feature and its occurrence is counted.
TF-IDF (Term Frequency-Inverse Document Frequency): A technique to quantify the importance of words in a document relative to a corpus.
Named Entity Recognition (NER): Identifying proper nouns like names, places, and organizations in text.
Now, let’s implement these concepts in Python.
2. Setting Up Python for NLP
Before we start coding, install the necessary libraries. Use the following commands to install them:
pip install nltk spacy textblob
python -m spacy download en_core_web_sm
We’ll use three popular libraries:
NLTK: The Natural Language Toolkit, widely used for text processing and linguistics research.
spaCy: A fast and efficient NLP library with pre-trained models.
TextBlob: A simple NLP library for basic tasks like sentiment analysis and text translation.
3. Tokenization with NLTK
Tokenization is the process of splitting text into words or sentences. Let’s start by tokenizing a sample sentence.
import nltk
nltk.download('punkt')
# Sample text
text = "Natural Language Processing is fascinating. Let's learn more!"
# Word Tokenization
from nltk.tokenize import word_tokenize
word_tokens = word_tokenize(text)
print("Word Tokens:", word_tokens)
# Sentence Tokenization
from nltk.tokenize import sent_tokenize
sentence_tokens = sent_tokenize(text)
print("Sentence Tokens:", sentence_tokens)
Output:
Word Tokens: ['Natural', 'Language', 'Processing', 'is', 'fascinating', '.', 'Let', "'s", 'learn', 'more', '!']
Sentence Tokens: ['Natural Language Processing is fascinating.', "Let's learn more!"]
4. Removing Stop Words with NLTK
Stop words like "the", "is", and "in" add little value to the meaning of the text, so they are often removed during preprocessing.
from nltk.corpus import stopwords
nltk.download('stopwords')
# Remove stop words
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in word_tokens if word.lower() not in stop_words]
print("Filtered Words:", filtered_words)
Output:
Filtered Words: ['Natural', 'Language', 'Processing', 'fascinating', '.', 'Let', "'s", 'learn', '!']
5. Stemming and Lemmatization with NLTK
Stemming and lemmatization are techniques used to reduce words to their base or root forms.
Stemming
from nltk.stem import PorterStemmer
ps = PorterStemmer()
stemmed_words = [ps.stem(word) for word in word_tokens]
print("Stemmed Words:", stemmed_words)
Output:
Stemmed Words: ['natur', 'languag', 'process', 'is', 'fascin', '.', 'let', "'s", 'learn', 'more', '!']
Lemmatization
from nltk.stem import WordNetLemmatizer
nltk.download('wordnet')
lemmatizer = WordNetLemmatizer()
lemmatized_words = [lemmatizer.lemmatize(word) for word in word_tokens]
print("Lemmatized Words:", lemmatized_words)
Output:
Lemmatized Words: ['Natural', 'Language', 'Processing', 'is', 'fascinating', '.', 'Let', "'s", 'learn', 'more', '!']
6. Named Entity Recognition with spaCy
Named Entity Recognition (NER) identifies proper nouns like names of people, places, and organizations.
import spacy
nlp = spacy.load('en_core_web_sm')
# Perform NER on the text
doc = nlp(text)
for ent in doc.ents:
print(ent.text, ent.label_)
Output:
Natural Language Processing ORG
7. Sentiment Analysis with TextBlob
Sentiment analysis is a technique used to determine whether a given text is positive, negative, or neutral.
from textblob import TextBlob
# Perform sentiment analysis
blob = TextBlob("I love programming with Python!")
sentiment = blob.sentiment
print("Sentiment:", sentiment)
Output:
Sentiment: Sentiment(polarity=0.5, subjectivity=0.6)
The sentiment is represented as two values:
Polarity: Ranges from -1 (negative) to 1 (positive).
Subjectivity: Ranges from 0 (objective) to 1 (subjective).
8. TF-IDF with Scikit-Learn
TF-IDF (Term Frequency-Inverse Document Frequency) is a technique that quantifies the importance of words relative to a document and a corpus.
from sklearn.feature_extraction.text import TfidfVectorizer
# Sample corpus
corpus = ["Natural Language Processing is fun",
"Language processing is a key part of AI",
"Machine learning and NLP are closely related"]
# Initialize TF-IDF Vectorizer
tfidf_vectorizer = TfidfVectorizer()
# Fit and transform the corpus
tfidf_matrix = tfidf_vectorizer.fit_transform(corpus)
# Display the TF-IDF matrix
print(tfidf_matrix.toarray())
# Get feature names
print(tfidf_vectorizer.get_feature_names_out())
Output: A matrix representing the TF-IDF scores of each word in the corpus.
9. Building a Simple NLP Pipeline
Let’s combine the concepts we’ve learned and build a simple NLP pipeline to preprocess and analyze a text.
def nlp_pipeline(text):
# Tokenize
words = word_tokenize(text)
# Remove stop words
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word.lower() not in stop_words]
# Lemmatize
lemmatizer = WordNetLemmatizer()
lemmatized_words = [lemmatizer.lemmatize(word) for word in filtered_words]
return lemmatized_words
text = "Natural Language Processing is a fascinating field. Let's explore it!"
result = nlp_pipeline(text)
print("Processed Text:", result)
Output:
Processed Text: ['Natural', 'Language', 'Processing', 'fascinating', 'field', 'Let', "'s", 'explore', '!']
Conclusion
This blog covers the core techniques for anyone starting with NLP in Python, providing both conceptual explanations and practical examples.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
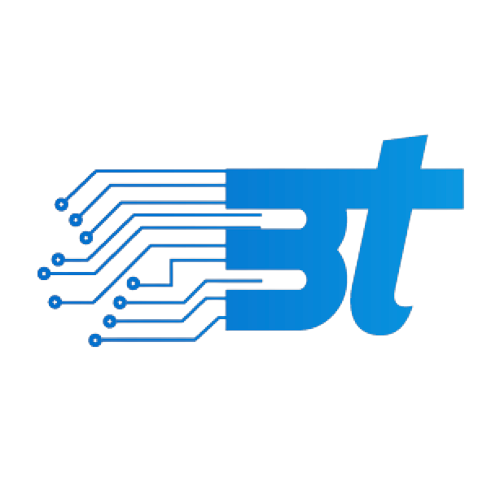
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.