Cypress Parallel Testing: A Step-by-Step Tutorial with Code Examples
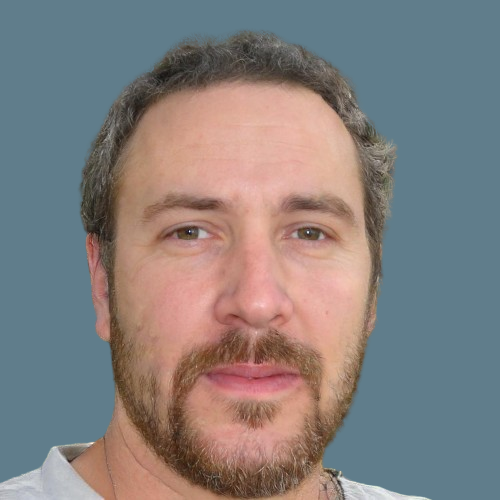
Cypress is a popular tool that is used for front-end testing in a simple manner, enabling easy execution of the tests. One feature of Cypress that can help you enhance the speed and efficiency of your test execution is running tests in parallel. In this article, we will dive into the details of executing tests in parallel using Cypress. We will first discuss parallel testing and then talk about how we can implement parallel testing in our Cypress automation tests.
What is Parallel Testing?
Parallel testing is a technique to execute the tests in parallel across multiple threads or processes. This technique allows for the concurrent execution of tests, in a distributed manner, rather than the sequential run. Parallel execution can be achieved by splitting the tests in multiple batches. These batches are assigned to different threads so that they can execute concurrently in isolation from each other. By following the approach, the overall test execution time is divided by the number of threads. More the number of threads, faster the execution.
Benefits of Cypress Parallel Testing
Increase in speed of execution by magnitudes, reducing the execution time from hours to minutes.
Increases regression coverage by running more tests concurrently.
Resource utilisation is improved by ensuring that idle time is not wasted.
Enables you to execute multiple tests in reasonable time.
Runs test in complete isolation by default.
Configuring Parallel Tests in Cypress
We will first create different tests and execute them normally, in sequence to see the execution time for the tests. To do so, we’ll write three tests, two to perform google search, and one to perform image search. Below is the code snippet for each of these tests.
GoogleSearch.cy.js
describe('Google Search',() => {
it('should be searching keyword on google',() => {
//Navigate to google search page
cy.visit('https://www.google.com/');
//Adding wait time to load the page
cy.wait(500);
//Entering search value in the textbox and performing th eenter key simulation
cy.get('.gLFyf').type('Automation Testing Cypress{enter}');
})
})
GoogleSearch1.cy.js
describe('Google Search 1',() => {
it('should be searching keyword on google',() => {
//Navigate to google search page
cy.visit('https://www.google.com/');
//Adding wait time to load the page
cy.wait(500);
//Entering search value in the textbox and performing th eenter key simulation
cy.get('.gLFyf').type('TestGrid{enter}');
})
})
GoogleImage.cy.js
describe('Google Image Search',() => {
it('should be searching image on google',() => {
//Navigate to google search page
cy.visit('https://www.google.com/');
//Adding wait time to load the page
cy.wait(500);
//Clicking on Images link on the google search page
cy.contains('Images').click();
//Entering search value in the textbox and performing th eenter key simulation
cy.get('.gLFyf').type('Tesgrid automation{enter}');
})
})
Note that I am keeping these three test specs in a folder parallel-tests as can be seen in the snapshot below:
Let us execute these tests using the command below:
npx cypress run --spec "cypress/e2e/parallel-tests/*.js"
As you can see, the execution time is 49 seconds for the tests, we will see how we can decrease the execution time by using cypress-plugin.
Using cypress-parallel plugin
The first step in implementation of the parallel test feature is to install the cypress-parallel plugin. To install it, execute the below command:
npm i cypress-parallel
After installation of the plugin, you will need to update the package.json file by adding below lines of code in the scripts section:
"cy:run": "cypress run",
"cy:parallel": "cypress-parallel -s cy:run -t 3 -d
'cypress/e2e/parallel-tests/*.js'"
Here, -t denotes the number of threads that you would want to invoke, and -d specifies the spec file path.
Once this is done, the package.json file would look like below:
{
"name": "paralleltest",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"cy:run": "cypress run",
"cy:parallel": "cypress-parallel -s cy:run -t 3 -d 'cypress/e2e/parallel-tests/*.js'"
},
"author": "",
"license": "ISC",
"devDependencies": {
"cypress": "^13.5.0",
"cypress-image-diff-js": "^1.32.0"
},
"dependencies": {
"cypress-parallel": "^0.13.0"
}
}
Next step is to execute the parallel tests using below command:
npm run cy:parallel
Upon execution you will notice the execution time has been decreased by a few seconds.
Though you won’t be able to see a huge difference above, but, when you execute a large number of tests you will see a visible decline in execution time.
Using CI parallel integration
Another way to implement parallel test execution is through the CI tool that you must be using. You can refer to the CI tool documentation to set up multiple machines in your CI environment. You can use these multiple machines to execute tests in parallel. Due to the limitation of setting up a CI environment now, we will simply go through the steps that we can follow to make the desired settings.
After machine set up execute the below command:
cypress run --record --key=abc123 --parallel
Here, run tells Cypress to execute all the tests in the spec folder, in headless mode by default. The record option enables the recording of the test run to record the screenshots and the videos. The key attribute is your personal cypress authentication key that can be fetched from the Cypress dashboard under the record keys. And the parallel attribute enables parallelization of tests to distribute across multiple CPU cores and threads.
You can further split your tests in separate files to enable parallelization of tests and can refer to the cypress documentation for details.
Best Practices for Cypress Parallel Testing
It is always better to keep tests focused, isolated and concise.
Try to group tests that take longer to execute separately.
Re-execute tests that fail sequentially to debug the error in a better way.
For flaky tests, add retries.
When using repeatable data sets, use a data-driven approach.
Try to eliminate test execution dependency by using random seeds.
Cypress Parallel Testing – Drawbacks
There might be some pitfalls too in using cypress parallel testing which you might consider while building your framework.
Tests may encounter race conditions due to shared data. To overcome this, try to implement a reset state between tests.
If there are tests that modify global data, there may be data inconsistency. To mitigate this, either avoid using global data or create a reset state between tests.
Some APIs might have limitations of concurrent users, because of which in parallel execution some APIs may get blocked or fail. To avoid such a situation, limit parallelism based on server capacity.
There might be CI failures that are hard to replicate, hence try to match CI parallelism as much as possible.
There might be file lock conflicts between the runner and parallelization tools. You should use only one test runner to prevent clashes.
Conclusion
Automation testing can be vast and is best utilized when you can run a large number of tests and increase your overall test coverage.
With increase in the number of tests, the execution time also increases. Cypress parallel testing offers a way to reduce execution time and execute tests faster with the same results.
Cypress offers using the cypress-parallel plugin to execute tests in parallel mode, by providing the number of threads you would want to execute in parallel.
You can also integrate cypress with CI tools to scale test execution in a shorter duration of time.
Cypress parallel testing when implemented by considering factors like server limit, environment reset state, data-driven approach, etc., one can efficiently scale test execution by not compromising on the overall coverage and efficiency of the framework.
Source: This blog was originally published at https://testgrid.io/blog/cypress-parallel-testing/
Subscribe to my newsletter
Read articles from Jace Reed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
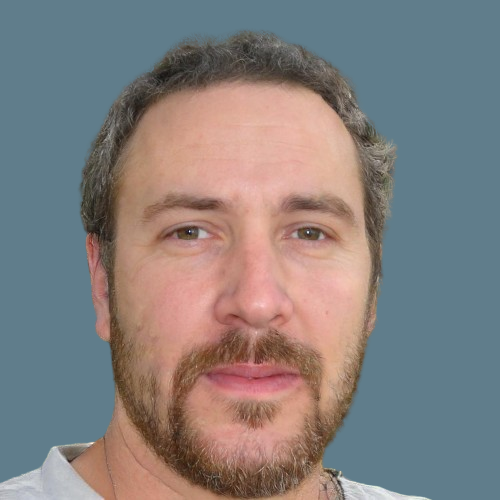
Jace Reed
Jace Reed
Senior Software Tester with 7+ years' experience. Expert in automation, API, and Agile. Boosted test coverage by 30%. Focused on delivering top-tier software.