A Beginner’s Guide to Methods in Java :

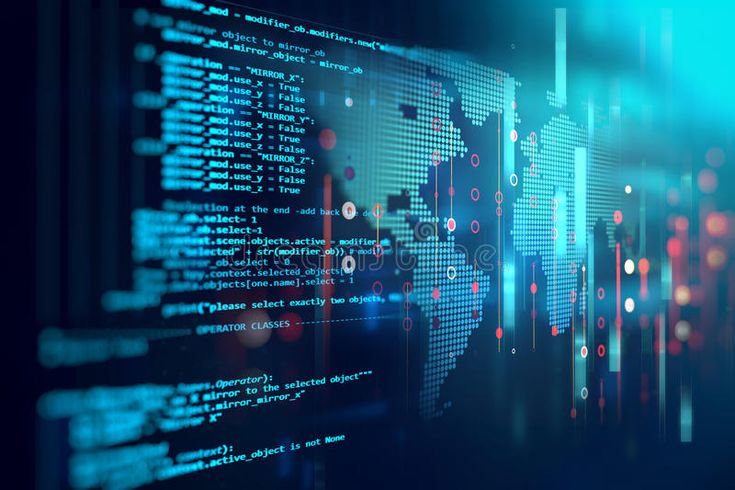
If you’re just getting started with Java, one of the key concepts you'll come across early on is methods. Methods are like little mini-programs inside your Java class that do a specific job. They help you organize your code and avoid writing the same code over and over.
What is a Method?
A method is simply a block of code that you can call to perform an action. For example, if you want to add two numbers, you can write a method that takes in two numbers, adds them, and gives back the result.
Think of methods as a way to give instructions to your program. You write the instructions once, and then you can use them whenever you need.
Here’s the basic structure of a method:
returnType methodName(parameter1, parameter2, ...) {
// method body
// the code that does the task
}
returnType: What the method will give back (like an
int
,String
, or nothing at all, in which case you usevoid
).methodName: The name you give the method, which should describe what it does (e.g.,
addNumbers
).parameters: Inputs to the method (optional). If your method needs some data to do its job, you provide it through parameters.
Example of a simple method :
Let’s look at a method that adds two numbers together.
public int addNumbers(int a, int b) {
int sum = a + b;
return sum;
}
Here’s what’s happening:
public
: This means the method can be called from anywhere (you don’t have to worry about this too much yet).int
: The method returns an integer (a whole number).addNumbers
: This is the name of the method.(int a, int b)
: These are the inputs, or parameters. The method takes two integers as input.return sum;
: This sends the result (the sum) back to where the method was called.
Now that we have seen how to declare a method, let’s explore how to call it. In simple terms, how can we use it?
Calling a Method :
Once you’ve written a method, you can call it from another part of your code. Here’s how you would use the addNumbers
method:
public class Calculator {
public static void main(String[] args) {
Calculator calculator = new Calculator();
int result = calculator.addNumbers(5, 10);
System.out.println("The sum is: " + result);
}
public int addNumbers(int a, int b) {
return a + b;
}
}
In this example:
We create an instance of the
Calculator
class.Then, we call the
addNumbers
method using that instance, passing in5
and10
as inputs.The method adds the numbers and returns the result, which we print out.
Why Use Methods?
Methods help make your code:
Reusable: Once a method is written, you can use it multiple times without repeating the code.
Organized: Your code is easier to understand because each method does one thing.
Readable: You can break down complex tasks into smaller, simpler methods.
Methods Without Return Values :
Sometimes, a method performs an action but doesn’t need to give anything back. In that case, you can use void
as the return type. For example:
public void sayHello() {
System.out.println("Hello, world!");
}
Here, the sayHello
method just prints a message, but it doesn’t return anything. You call it the same way as any other method:
sayHello();
Passing Data to Methods :
Methods can accept inputs (called parameters) to work with. You’ve seen examples where methods take two numbers and return a result. Here’s a simple method that takes a String
as a parameter:
public void greet(String name) {
System.out.println("Hello, " + name);
}
If you call greet("Alice")
, it will print:
Hello, Alice
You can pass different values to the same method to get different results.
Returning Values from Methods
When you want a method to return a value, you need to:
Specify the return type (like
int
,String
, etc.).Use the
return
statement to send the result back.
For example, this method returns the square of a number:
public int square(int number) {
return number * number;
}
Now, when you call square(4)
, it will return 16
.
Conclusion :
Methods are one of the most basic building blocks in Java. They help you write cleaner, more organized code. By creating methods, you can make your programs easier to understand, reuse code, and break complex problems into simpler parts. Whether it’s a simple task like printing a message or something more complex like performing calculations, methods are your go-to tool for getting the job done.
Keep practicing writing methods, and soon you’ll see how powerful they can be!
Happy Coding !!!
Subscribe to my newsletter
Read articles from Akash Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akash Das
Akash Das
Java Developer | Tech Blogger | Spring Boot Enthusiast | DSA Advocate*I specialize in Java, Spring Boot, and Data Structures & Algorithms (DSA). Follow my blog for in-depth tutorials, best practices, and insights on Java development, Spring Boot, and DSA. Join me as I explore the latest trends and share valuable knowledge to help developers grow.