Mastering Asynchronous JavaScript: Why Async/Await Is Your Best Friend

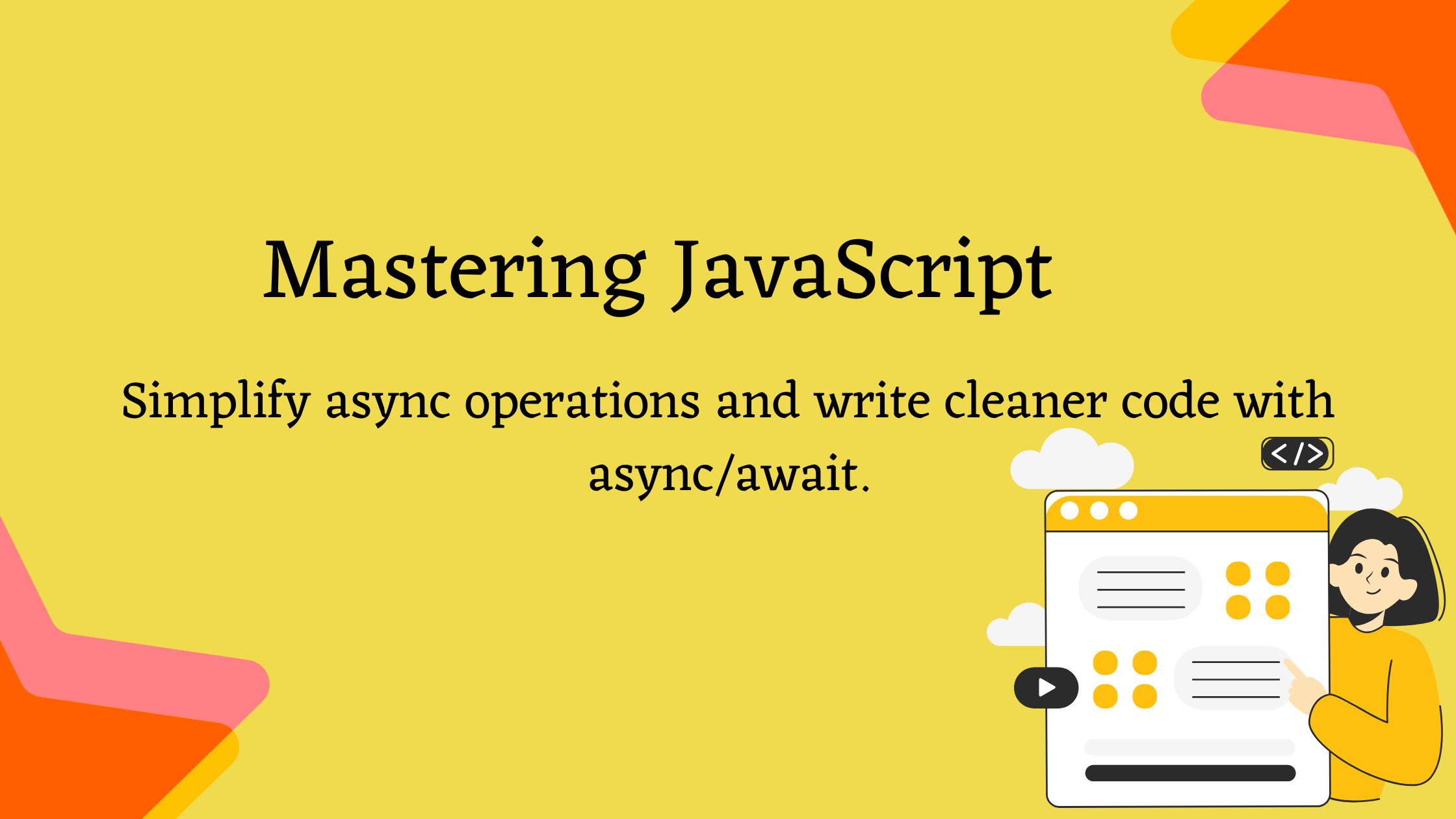
JavaScript’s asynchronous nature is one of its most powerful features, allowing developers to write non-blocking code that enhances performance and user experience. But handling asynchronous operations used to be more complex and sometimes even confusing, thanks to the traditional use of callbacks and, later, Promises.
With the introduction of async
and await
in ES2017 (ES8), JavaScript developers gained a much simpler, more readable way to handle asynchronous code. But what exactly are the differences between Promises and async/await, and why should you make the switch?
Let’s dive in!
The Problem with Promises
Promises were a big improvement over callbacks (goodbye, callback hell! 🔥). However, they introduced their own challenges, particularly when it came to readability and debugging.
Here’s a typical example using Promises:
fetchData()
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
As the complexity of your application grows, chaining multiple .then()
calls can result in "Promise hell," where the logic becomes more difficult to follow. Error handling becomes another headache, requiring you to include .catch()
at the end of every chain to avoid unhandled errors.
Enter async/await
The async/await
syntax makes asynchronous code look almost synchronous, dramatically improving code readability. Let’s break down the two key players:
async
: You mark a function asasync
to signal that it will handle asynchronous operations.await
: This keyword pauses the execution of the function until thePromise
is resolved. The beauty of this is that you can handle asynchronous code almost like it's synchronous, eliminating the need for multiple.then()
calls.
Here’s the same example, but rewritten using async/await
:
async function getData() {
try {
const response = await fetchData();
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
getData();
This code is much easier to read and understand. Instead of chaining .then()
, we can simply await
each step and catch any errors using a single try/catch
block. 🧑💻
Key Benefits of async/await
Improved Readability: With
async/await
, asynchronous code looks just like synchronous code. This makes it easier to follow the program flow, especially in large applications.Error Handling: Instead of chaining
.catch()
after everyPromise
, you can handle errors more cleanly with atry/catch
block. This simplifies both debugging and maintaining the code.Chaining Promises: When working with multiple asynchronous operations, you can use
await
to pause and wait for each one to complete before moving on to the next. No more messy chains of.then()
!
Here’s an example with multiple async operations:
async function processData() {
try {
const data1 = await fetchData1();
const data2 = await fetchData2();
const result = await process(data1, data2);
console.log(result);
} catch (error) {
console.error('Error:', error);
}
}
processData();
In the async/await
version, it’s clear what each step of the process does, and the logic is much easier to follow.
Gotchas of async/await
Despite its many benefits, there are a few caveats to keep in mind when using async/await
:
- Sequential Execution: If you
await
multiple asynchronous operations in sequence, they will run one after another, which can be slower. If the operations are independent of each other, consider usingPromise.all()
to run them concurrently.
Example:
const [data1, data2] = await Promise.all([fetchData1(), fetchData2()]);
- Blocking Execution: Since
await
pauses execution until thePromise
is resolved, make sure you only use it in situations where you actually need to wait for the result. Misuse could lead to performance issues.
Conclusion
async/await
is a significant improvement over Promises in terms of readability, error handling, and maintaining code quality. If you haven’t already switched to async/await
in your JavaScript projects, now is the time to make the leap! 🚀
While Promises are still very much part of the language, especially for concurrent tasks, the simplicity and power of async/await
make it the go-to choice for most scenarios.
Thank You!
Thank you for reading!
I hope you enjoyed this post. If you did, please share it with your network and stay tuned for more insights on software development. I'd love to connect with you on LinkedIn or have you follow my journey on HashNode for regular updates.
Happy Coding!
Darshit Anjaria
Subscribe to my newsletter
Read articles from Darshit Anjaria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshit Anjaria
Darshit Anjaria
An experienced professional with 5.5+ years in the industry, adept at collaborating effectively with developers across various domains to ensure timely and successful project deliveries. Proficient in Android/Flutter development and currently excelling as a backend developer specializing in Node.js. I bring a strong enthusiasm for learning new frameworks, paired with a quick-learning mindset and a passion for writing bug-free, optimized code. I am always ready to adapt to and learn cloud technologies, ensuring continuous growth and improvement. I actively contribute to communities by writing insightful articles on my blog and am seeking support from you all to create more valuable content and tutorials like this.