Let’s Talk: How to Update and Manipulate Elements in JavaScript

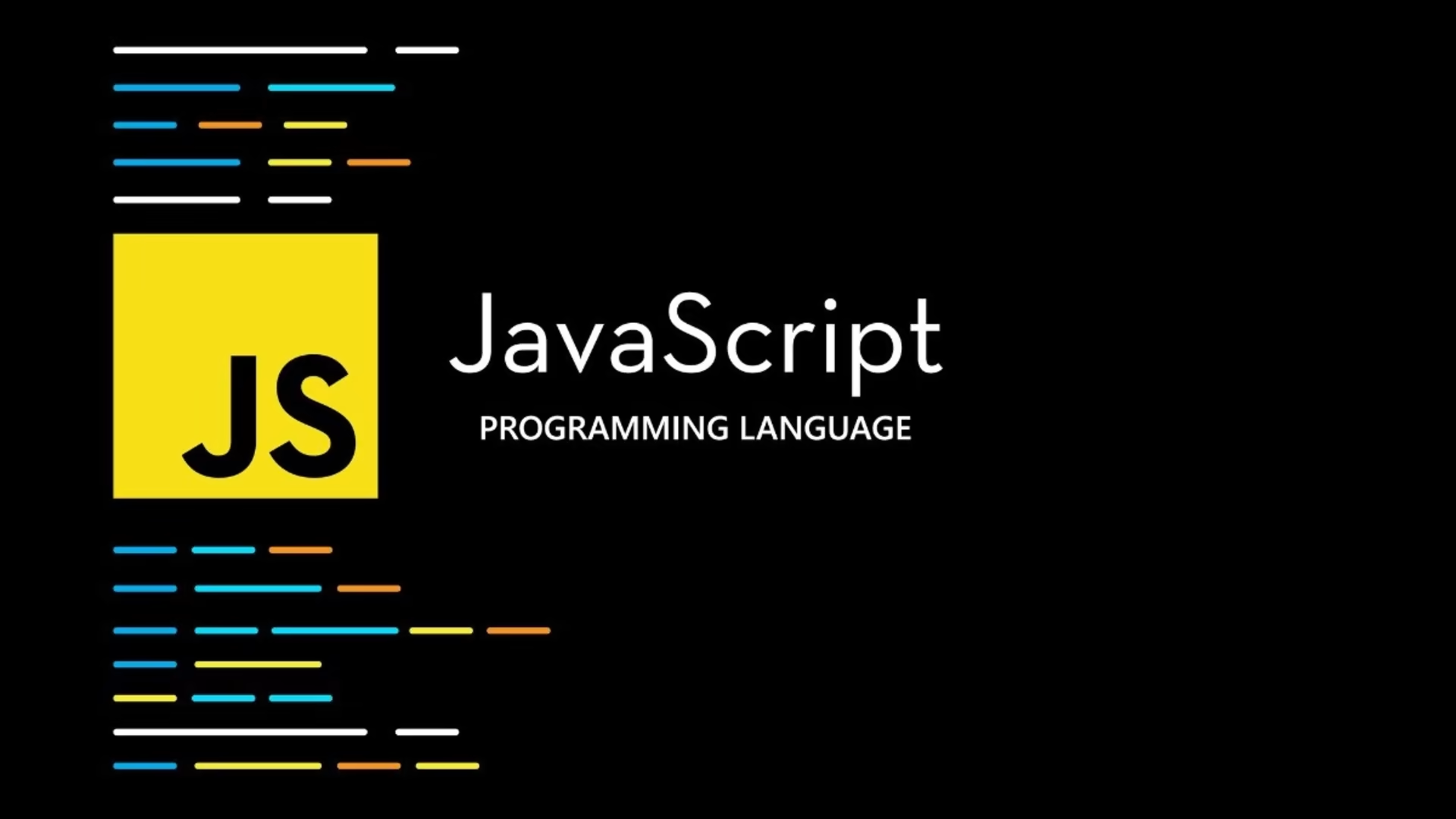
Welcome to another episode of "Let's Talk with Hundredz!" Today, we’re diving into one of the most exciting parts of JavaScript: updating and manipulating DOM elements. If you’ve already mastered selecting elements from the DOM, the next logical step is to learn how to update and manipulate those elements to create dynamic, responsive web pages.
In this article, I’ll walk you through some of the most common techniques used to modify content, change styles, handle attributes, and more—all using JavaScript.
1. Modifying Content Inside Elements
One of the first things you’ll want to do when working with the DOM is change the content inside an element. JavaScript makes this super easy with properties like innerHTML
and textContent
.
innerHTML
: This allows you to replace the entire HTML content of an element. It’s powerful but can be risky if you’re dealing with user inputs because it can parse HTML and even JavaScript.let element = document.getElementById('content'); element.innerHTML = '<h2>New Content Loaded!</h2>';
When to Use It: Use
innerHTML
when you want to swap out large sections of content, such as replacing entire blocks of text, images, or even forms.textContent
: If you only need to update the text (without any HTML tags),textContent
is your friend. It’s more secure because it doesn’t parse HTML, so it’s perfect for displaying plain text.element.textContent = 'Updated Text Content';
When to Use It: Use
textContent
when you need to update simple text, like a headline or a label, and you’re not dealing with any HTML formatting.
2. Changing Styles Dynamically
With JavaScript, you can change how elements look in real-time. This can be as simple as changing the background color of a button or hiding/showing elements based on user actions.
Example:
let button = document.querySelector('.btn'); button.style.backgroundColor = 'blue'; button.style.display = 'none'; // Hide the button
When to Use It: Use the
style
property for quick, inline style updates, such as changing colors on hover or hiding an element after an action (e.g., a form submission button).
3. Working with Attributes
Sometimes, you’ll need to update the attributes of an element, like changing the source of an image or updating a link’s destination. JavaScript gives you several ways to do this.
setAttribute()
: This allows you to set or update an element’s attributes.let imgElement = document.querySelector('img'); imgElement.setAttribute('src', 'new-image.jpg'); imgElement.setAttribute('alt', 'Updated Image Description');
getAttribute()
: This retrieves the current value of an element’s attribute.let link = document.querySelector('a'); let hrefValue = link.getAttribute('href');
When to Use It: Anytime you need to update dynamic attributes, such as changing the image source in a photo gallery or modifying form input values on the fly.
4. Managing Classes Efficiently
CSS classes are one of the most powerful ways to style and animate elements, and JavaScript allows you to dynamically add, remove, or toggle classes.
classList.add()
: Add a class to an element.element.classList.add('active');
classList.remove()
: Remove a class from an element.element.classList.remove('inactive');
classList.toggle()
: Toggle a class on or off, depending on its current state.element.classList.toggle('highlighted');
When to Use It: Use
classList
to control classes when you need to apply styles based on user interactions—like showing a dropdown menu, highlighting a selected button, or applying animations when scrolling.
5. Adding Event Listeners for Interaction
No dynamic web page is complete without user interaction, and event listeners let you add interactivity to your elements. For example, you can listen for clicks, hovers, or form submissions and trigger actions accordingly.
addEventListener()
: Attach an event listener to an element. Here’s how you can trigger an alert when a button is clicked.let button = document.querySelector('#myButton'); button.addEventListener('click', function() { alert('Button clicked!'); });
When to Use It: Use event listeners when you want to add interactive functionality, like handling button clicks, form submissions, or triggering animations.
6. Traversing the DOM
There will be times when you need to navigate between related elements—whether you’re moving from a parent to its children or hopping between sibling elements. DOM traversal helps you navigate your page’s structure programmatically.
Example:
let parentElement = element.parentElement; // Go to the parent let firstChild = element.firstElementChild; // Get the first child let nextSibling = element.nextElementSibling; // Get the next sibling
When to Use It: DOM traversal is great when you need to manipulate related elements, like expanding a section when its parent is clicked, or highlighting sibling elements.
Conclusion
And that’s a wrap for today’s topic on updating and manipulating elements in JavaScript! By now, you should have a good grasp of how to make your web pages truly interactive and dynamic. Whether you’re modifying content, changing styles, or responding to user actions with event listeners, these tools give you the power to build rich, responsive web experiences.
Thanks for tuning in to this episode of "Let's Talk with Hundredz", and I hope you feel more confident in bringing your projects to life with JavaScript.
Subscribe to my newsletter
Read articles from Anusiem Chidubem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
