"Mastering the Dining Philosophers Problem: A Guide to Concurrency and Deadlock Prevention in C"

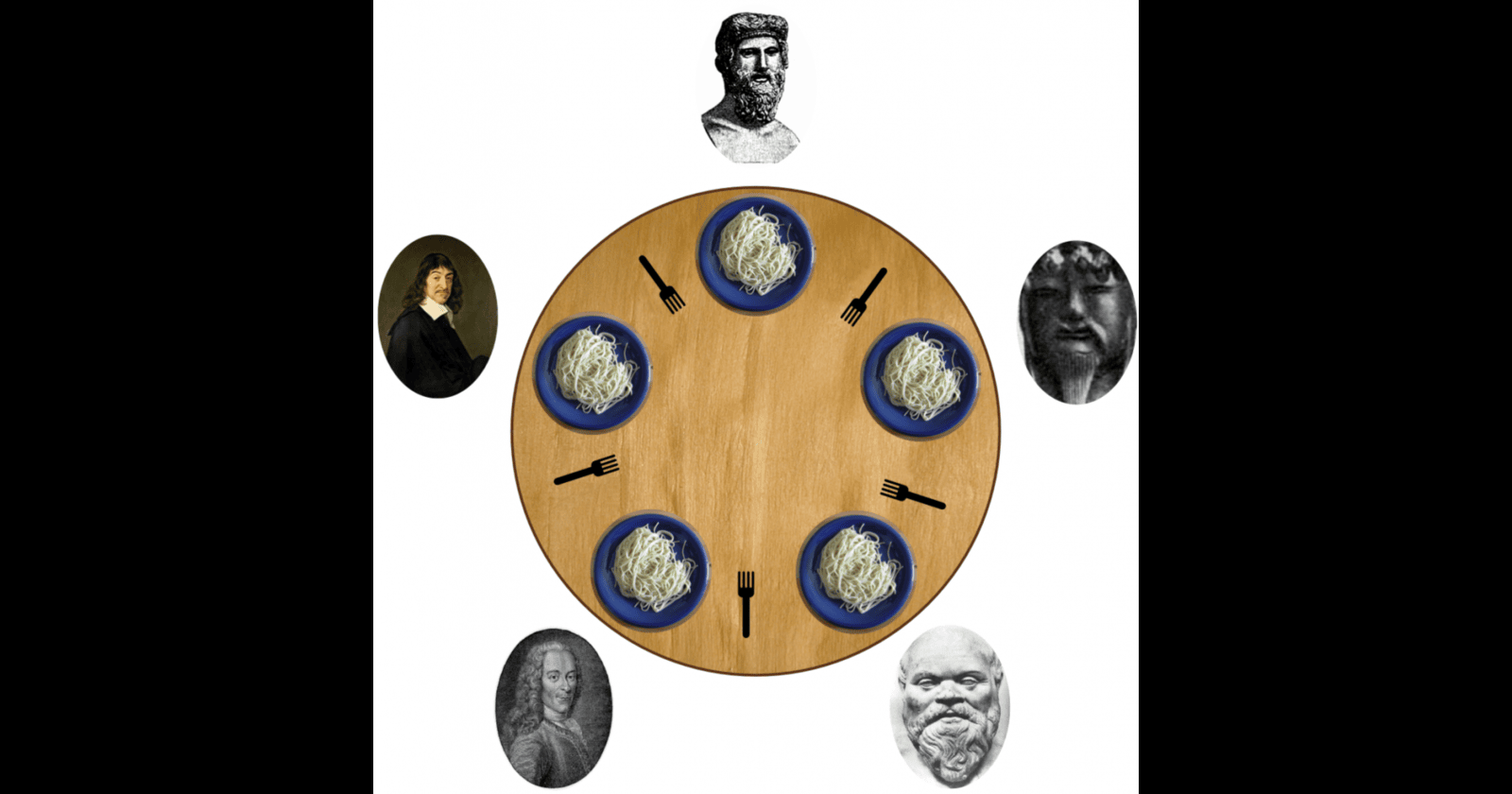
Introduction
The Dining Philosophers Problem is a classical synchronization problem that beautifully demonstrates the challenges of avoiding deadlock and resource starvation in concurrent programming. Originally formulated by E. W. Dijkstra, it’s widely used in operating systems and threading literature to explain issues surrounding resource sharing.
In this blog, we'll dive deep into understanding this problem, the underlying theory, and a practical implementation in C using threads and semaphores.
What is the Dining Philosophers Problem?
Imagine five philosophers sitting around a circular table. Each philosopher alternates between thinking and eating. Between every pair of philosophers, there is one chopstick. A philosopher needs two chopsticks (one from the left and one from the right) to eat.
The challenge is: how do you ensure that no philosopher starves (waiting indefinitely for chopsticks) and that no deadlock occurs (all philosophers waiting forever for chopsticks held by their neighbors)?
Why is This Problem Important?
This problem models real-world issues in concurrent computing, where multiple threads or processes need to access shared resources (like memory or files) without conflict. The Dining Philosophers Problem teaches us to handle these shared resources while avoiding deadlock, ensuring fairness, and improving efficiency in multi-threaded applications.
Breaking Down the C Code Implementation
Let’s explore how the code implements this synchronization problem using semaphores.
#include<stdio.h>
#include<stdlib.h>
#include<pthread.h>
#include<semaphore.h>
#include<unistd.h>
// Function prototype for eat()
void eat(int phil);
sem_t room;
sem_t chopstick[5];
In the above section, we include necessary headers: pthread.h
for threading, semaphore.h
for semaphores, and unistd.h
for sleep functionality. We initialize a semaphore room
to limit the number of philosophers in the dining room, ensuring that deadlock doesn’t occur. Each chopstick is represented as a semaphore, ensuring mutual exclusion (i.e., a chopstick can only be used by one philosopher at a time).
void *philosopher(void *num) {
int phil = *(int *)num;
sem_wait(&room); // Philosopher tries to enter the room
printf("\nPhilosopher %d is thinking", phil);
sem_wait(&chopstick[phil]); // Pick up left chopstick
sem_wait(&chopstick[(phil+1)%5]); // Pick up right chopstick
In the philosopher
function, each philosopher starts by "thinking". The philosopher then tries to enter the room using the semaphore room
. Only four out of five philosophers can be in the room simultaneously, preventing deadlock. This is a crucial point: limiting the number of philosophers in the room avoids a situation where all philosophers simultaneously pick up their left chopstick and wait forever for the right one.
After that, each philosopher picks up two chopsticks—one from the left and one from the right—using the sem_wait
function. These operations ensure that no two philosophers can use the same chopstick at the same time.
// Eat
eat(phil);
// Put down chopsticks
sem_post(&chopstick[phil]);
sem_post(&chopstick[(phil+1)%5]);
sem_post(&room); // Philosopher leaves the room
}
When a philosopher successfully picks up both chopsticks, they start eating. The eat
function simulates eating by introducing a short delay using sleep(2)
. Once the philosopher is done eating, they release both chopsticks using sem_post
, and leave the room.
void eat(int phil) {
printf("\nPhilosopher %d is eating", phil);
sleep(2); // Simulate eating time
}
The eat
function is straightforward: it prints a message indicating that the philosopher is eating and pauses for 2 seconds to simulate the action.
int main() {
int i, a[5];
pthread_t tid[5]; // Threads for each philosopher
// Initialize the semaphores
sem_init(&room, 0, 4);
for (i = 0; i < 5; i++)
sem_init(&chopstick[i], 0, 1);
In the main
function, we initialize the semaphores. The room
semaphore allows up to four philosophers to enter the room simultaneously (preventing deadlock). Each chopstick is initialized with a value of 1
, meaning it can be held by only one philosopher at a time.
// Create philosopher threads
for (i = 0; i < 5; i++) {
a[i] = i;
pthread_create(&tid[i], NULL, philosopher, (void *)&a[i]);
}
// Join threads to main thread
for (i = 0; i < 5; i++)
pthread_join(tid[i], NULL);
return 0;
}
Finally, we create threads for each philosopher, passing the philosopher number as an argument. The program waits for all philosopher threads to complete using pthread_join
.
How Does This Solution Prevent Deadlock?
This solution prevents deadlock by limiting the number of philosophers who can attempt to eat simultaneously (through the room
semaphore). If only four philosophers can sit at the table, at least one philosopher is guaranteed to have access to both chopsticks, ensuring the system can always move forward.
Key Takeaways
Deadlock Prevention: Limiting the number of philosophers in the room prevents a circular wait, a common cause of deadlock.
Semaphores for Resource Management: Each chopstick is a semaphore, ensuring mutual exclusion and preventing race conditions.
Practical Utility: Understanding the Dining Philosophers Problem is crucial in operating systems, multi-threaded programming, and resource management.
Conclusion
The Dining Philosophers Problem is a brilliant example of how to handle resource sharing in concurrent systems. By using semaphores and carefully structuring the access to shared resources (chopsticks), we avoid deadlock and starvation. This concept extends beyond theoretical puzzles and helps us in designing real-world systems, from OS kernels to parallel computing applications.
"Stay curious, keep learning, and let your code make a difference!"
~ Sushil Kumar Mishra
Subscribe to my newsletter
Read articles from Sushil Kumar Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
