Module Pattern
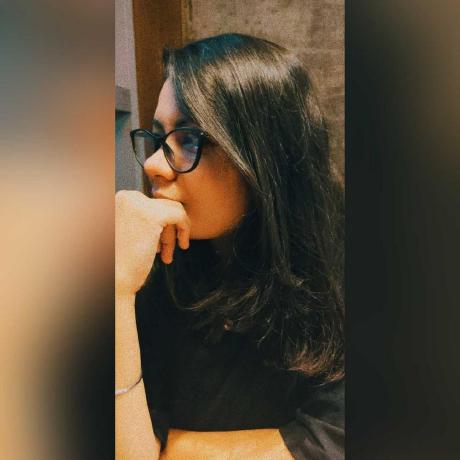
The module pattern in javascript allows you to split up your code into smaller, reusable pieces along with keeping certain values within your file private.
How to use this technique in React in an effective manner?
import React, { useState } from 'react';
import inventoryModule from './inventory-module';
const App = () => {
const [inventory, setInventory] = useState(inventoryModule.getInventoryItems());
const [itemName, setItemName] = useState('');
const [itemPrice, setItemPrice] = useState(0);
const [itemQuantity, setItemQuantity] = useState(1);
const handleAddToInventory = () => {
const newItem = {
id: Math.random().toString(36).substring(7),
name: itemName,
price: itemPrice,
quantity: itemQuantity,
}
inventoryModule.addToInventory(newItem);
setInventory(inventoryModule.getInventoryItems());
setItemName("");
setItemPrice(0);
setItemQuantity(1);
};
const handleRemoveFromInventory = (itemId) => {
inventoryModule.removeFromInventory(itemId);
setInventory(inventoryModule.getInventoryItems());
};
return (
<div>
<h1>Inventory</h1>
<ul>
{inventory.map((item) => (
<li key={item.id}>
{item.name} - ${item.price} - Quantity: {item.quantity}
<button onClick={() => handleRemoveFromInventory(item.id)}>
Remove
</button>
</li>
))}
</ul>
<h3>Total Price: ${inventoryModule.getTotalPrice.toFixed()}</h3>
<input type="text" placeholder="Item Name" value={itemName}
onChange={(e) => setItemName(e.target.value)} />
<input type="number" placeholder="Item Price" value={itemPrice}
onChange={(e) => setItemPrice(parseFloat(e.target.value))} />
<input type="number" placeholder="Item Quantity" value={itemQuantity}
onChange={(e) => setItemQuantity(parseInt(e.target.value))} />
<button onClick={handleAddToInventory}>Add to Inventory</button>
</div>
);
};
export default App;
Module file:
const inventoryModule = (() => {
let inventory = [];
const TAX_RATE = 0.1;
const calculateTotalPrice = () => {
const subTotal = inventory.reduce((acc, item) => {
return acc + item.price * item.quantity;
}, 0);
const tax = subTotal * TAX_RATE;
return tax + subTotal;
};
return {
addToInventory: (item) => {
inventory.push();
},
removeFromInventory: (itemId) => {
inventory = inventory.filter((item) => item.id !== itemId);
},
getInventoryItems: () => inventory,
getTotalPrice: () => calculateTotalPrice();
},
}
})();
export default inventoryModule;
The above was just a simple demonstration on how to use module pattern.
Hope you got a brief idea on how to implement the same.
Thank you for reading!
Subscribe to my newsletter
Read articles from Palak Bansal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
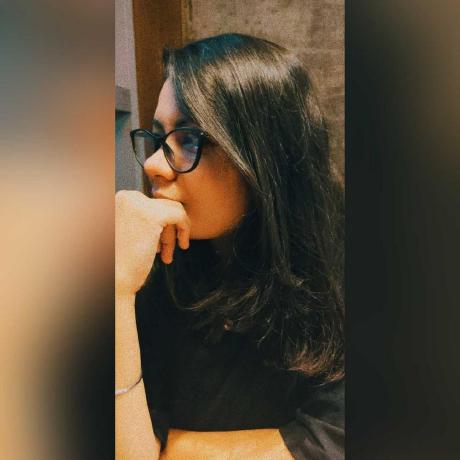
Palak Bansal
Palak Bansal
Experienced Frontend Developer with a demonstrated history of working in the financial services industry along with having 5+ years of hands on professional experience efficiently coding websites and applications using modern HTML, CSS and Javascript along with their respective frameworks viz.Vue.JS, React.JS. Instituting new technologies and building easy to use and user-friendly websites is truly a passion of mine. I actively seek out new libraries and stay up-to-date on industry trends and advancements. Translated designs to frontend code along with streamlining existing code to improve site performance. Collaborated with UX designers and Back End Developers to ensure coherence between all parties. Also tested some feature prototypes for bugs and user experience.