Axios Interceptors in React.js: A Developer’s Guide

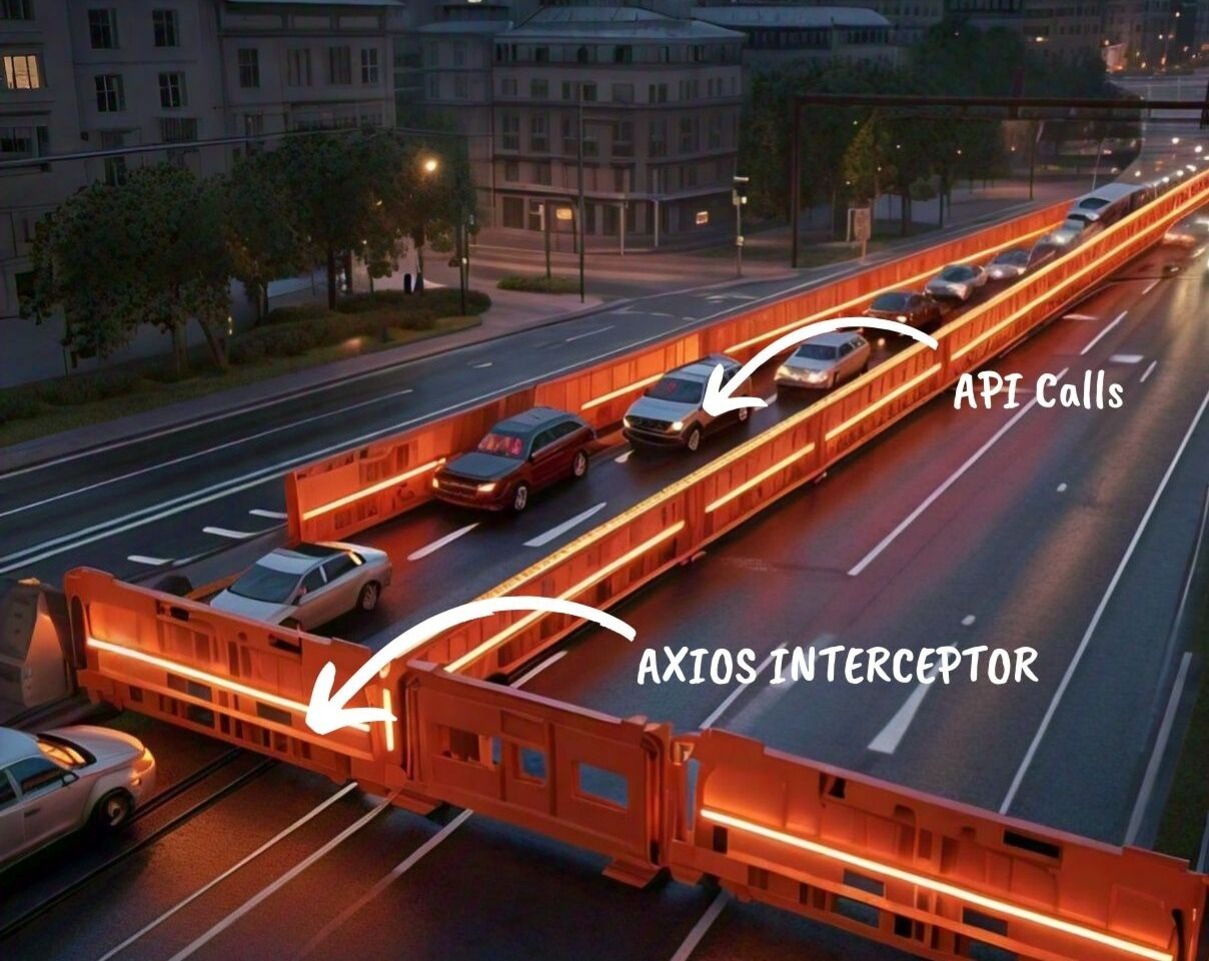
Hey there, fellow React developers! 👋 We’re diving into the world of Axios interceptors — a powerful feature that can seriously level up your HTTP request game. Whether you’re building a small side project or a complex enterprise application, understanding and implementing interceptors can make your life a whole lot easier.
If you’re accustomed to adding tokens to every API request and logging the response for each request individually, using Axios interceptors can greatly simplify this process.
What’s the Deal with Axios and Interceptors?
Before we jump in, let’s quickly recap what Axios is and what’s so special in it. Axios is a reliable method to make HTTP requests in your JavaScript applications. It’s simple, promise-based, and works seamlessly with React.
What is Axios Interceptors?
Now Interceptors — As the name suggests, interceptors handle HTTP requests and responses by intercepting them. They can modify requests before they are sent to the server or alter responses before, they reach your application’s components.
Why Interceptors?
Imagine you’re building an Saas app. You need to:
- Add authentication tokens to every request
- Handle expired tokens gracefully
- Log all API calls for debugging
- Transform response data to fit your app’s needs
Doing this manually for every API call would be a nightmare, right? Replicating same functions for every API call handling errors. That’s where Interceptors comes in. They let you handle all of this in one central place, keeping your code DRY (Don’t Repeat Yourself).
Types of Axios Interceptors
Request Interceptors — For Adding authentication, Custom Headers etc.
Response Interceptors — For Logging, Formatting, Error Handling etc.
Request Interceptors
Assuming an auth token needs to be appended in every Api request.
Request interceptors are like your pre-flight checklist. They run before your request takes off. Here’s how you might use one to add an auth token:
import axios from 'axios';
axios.interceptors.request.use(
async (config) => {
const token = await getNewToken(); // Custom function which fetches active token.
if (token) {
config.headers.Authorization = `Bearer ${token}`;
}
return config;
},
(error) => {
return Promise.reject(error);
}
);
The above piece of code adds a Bearer token to every outgoing Api request.
Let's say you have an Axios post request API call.
const response = await axios.post('https://api.example.com/setadmindata', {
field1:"Rahul",
Admin: true
});
Although the above POST
request does not explicitly include a Bearer token in the header, it will be automatically added when the request is sent, as a global request interceptor has been set up to handle this.
Response Interceptors
Let’s say you want to log every Api response for debugging.
import axios from 'axios';
axios.interceptors.response.use(
(response) => {
console.log(response.data)
// Transform data here if needed
return response;
},
async (error) => {
// Do something with response error
return Promise.reject(error);
return Promise.reject(error);
}
);
Now, every response will be logged and transformed by the response Interceptor.
Best Practices
Keep it clean: Don’t overload your interceptors. They should do one thing and do it well.
Handle cleanup: If you’re adding interceptors in components, remember to remove them when the component unmounts to avoid memory leaks.
Test your interceptors: Yes, you can and should write tests for your interceptors.
Wrapping up
Interceptors provide a clean, centralized way to handle common tasks like authentication, logging, and error handling. By implementing them in your React apps, you’re setting yourself up for more maintainable, robust code.
Don’t forget to checkout Axios official documentation here.
If you come up with some cool uses for interceptors, drop a comment below — I’d love to hear about it! Happy Coding.
Thanks for reading, if you found the article helpful, then you can checkout me on X and LinkedIn.
Subscribe to my newsletter
Read articles from Rahul Krishna directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rahul Krishna
Rahul Krishna
Software Developer. Building with 0-1 Startups.