How to Paginate More Than One List on the Same Blade File in Laravel 11 with Bootstrap 5 and Tailwind CSS

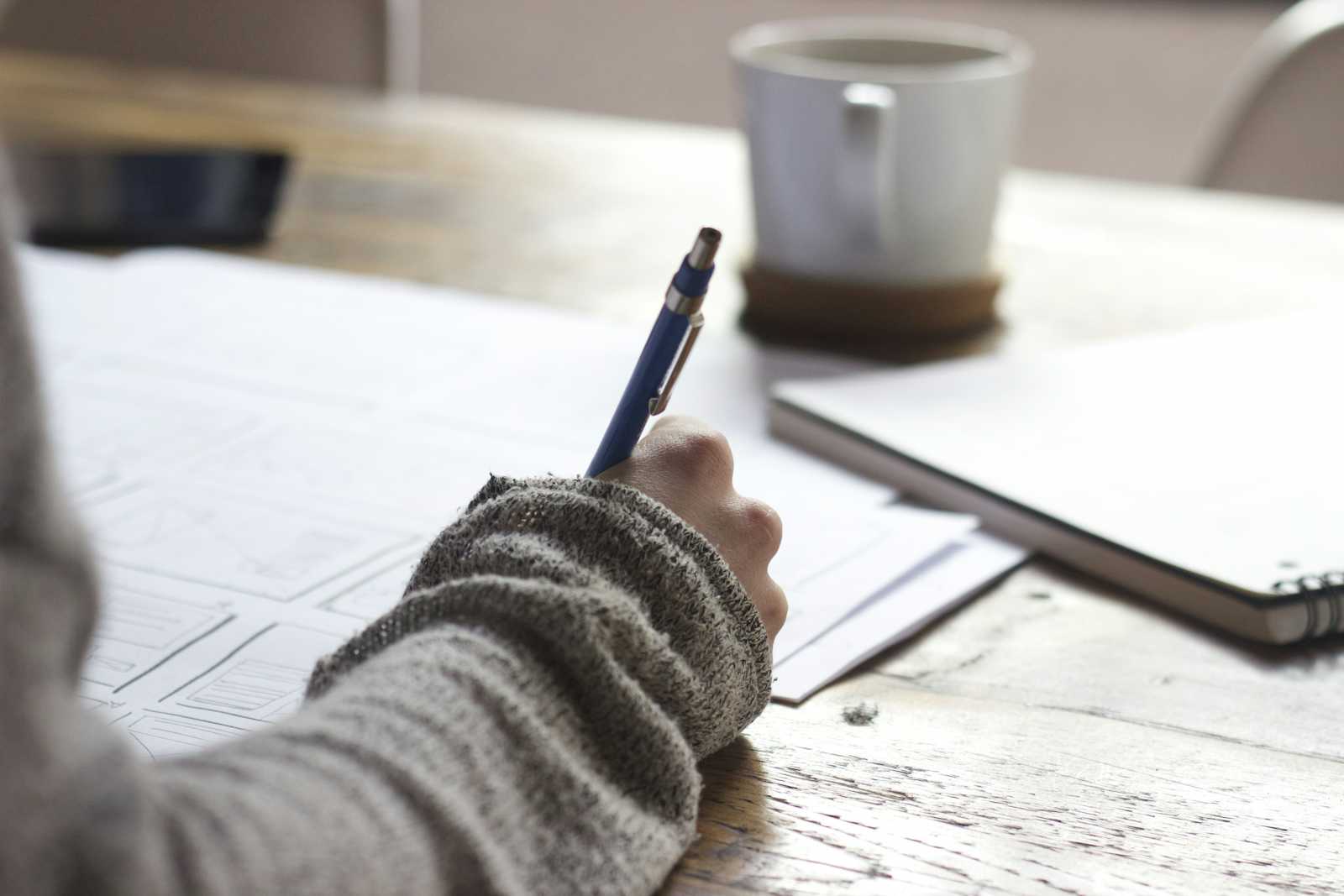
When building complex applications with Laravel, you might find yourself needing to paginate multiple lists on the same Blade template. This could be useful for dashboards, admin panels, or any page where multiple datasets are displayed. In this guide, we'll walk through how to paginate more than one list on the same Blade file using Laravel 11, Bootstrap 5, and Tailwind CSS.
Introduction
Paginating multiple lists on a single Blade file can be challenging but is often required in modern web applications. Laravel provides robust support for pagination, and combining it with Bootstrap 5 or Tailwind CSS can give your pagination a sleek and professional look. In this tutorial, we'll cover how to paginate different lists independently and display them on the same page.
Setting Up Your Laravel Project
First, ensure your Laravel project is set up and running. You can create a new Laravel project with the following command if you haven’t already:
composer create-project --prefer-dist laravel/laravel myProject
Creating the Controller and Model
For this example, let’s assume you have two models: User
and Product
. We’ll create a controller to handle the pagination for both models.
php artisan make:controller DashboardController
In the DashboardController
, you’ll need to fetch paginated data for both User
and Product
models.
Implementing Pagination in Your Controller
Open the DashboardController
and add the following methods:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use App\Models\Product;
use Illuminate\Http\Request;
class DashboardController extends Controller
{
public function index(Request $request)
{
// Paginate users
$users = User::orderBy('name_of_firm')->paginate(10, ['*'], 'agents');
// Paginate products
$products = Product::orderBy('name')->paginate(10, ['*'], 'products');
return view('dashboard', compact('users', 'products'));
}
}
Here, we're paginating User
and Product
models and passing them to the dashboard
view. Note that we use different query string parameters for pagination (agents
for users and products
for products) to keep them independent.
Displaying Multiple Paginated Lists in Blade
Create or edit the resources/views/dashboard.blade.php
file to display the paginated lists:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dashboard</title>
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- Tailwind CSS -->
<link href="https://cdn.jsdelivr.net/npm/tailwindcss@3.3.0/dist/tailwind.min.css" rel="stylesheet">
</head>
<body>
<div class="container mt-4">
<!-- Users List -->
<h2 class="text-2xl font-bold mb-4">Users List</h2>
<div class="mb-4">
<ul class="list-group">
@foreach ($users as $user)
<li class="list-group-item">{{ $user->name_of_firm }}</li>
@endforeach
</ul>
{{ $users->appends(['products' => $products->currentPage()])->links('pagination::bootstrap-5') }}
</div>
<!-- Products List -->
<h2 class="text-2xl font-bold mb-4">Products List</h2>
<div>
<ul class="list-group">
@foreach ($products as $product)
<li class="list-group-item">{{ $product->name }}</li>
@endforeach
</ul>
{{ $products->appends(['agents' => $users->currentPage()])->links('pagination::bootstrap-5') }}
</div>
</div>
<!-- Bootstrap JS (Optional) -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
In this Blade template:
e display a list of users and products.
Pagination links for each list are styled with Bootstrap 5.
We use
appends
to ensure that pagination links preserve the state of the other paginated list.
Styling Pagination Links with Bootstrap 5 and Tailwind CSS
Laravel provides several pagination views. For Bootstrap 5, you can use pagination::bootstrap-5
for Bootstrap styling. To style pagination links with Tailwind CSS, you can create a custom pagination view.
For Tailwind CSS, create a new pagination view file:
mkdir -p resources/views/vendor/pagination
touch resources/views/vendor/pagination/tailwind.blade.php
Add the following Tailwind CSS pagination styles to tailwind.blade.php
:
@if ($paginator->hasPages())
<nav>
<ul class="flex justify-between">
{{-- Previous Page Link --}}
@if ($paginator->onFirstPage())
<li class="disabled">
<span>«</span>
</li>
@else
<li>
<a href="{{ $paginator->previousPageUrl() }}" rel="prev">«</a>
</li>
@endif
{{-- Pagination Elements --}}
@foreach ($elements as $element)
{{-- "Three Dots" Separator --}}
@if (is_string($element))
<li class="disabled"><span>{{ $element }}</span></li>
@endif
{{-- Array Of Links --}}
@if (is_array($element))
@foreach ($element as $page => $url)
@if ($page == $paginator->currentPage())
<li class="active"><span>{{ $page }}</span></li>
@else
<li><a href="{{ $url }}">{{ $page }}</a></li>
@endif
@endforeach
@endif
@endforeach
{{-- Next Page Link --}}
@if ($paginator->hasMorePages())
<li>
<a href="{{ $paginator->nextPageUrl() }}" rel="next">»</a>
</li>
@else
<li class="disabled">
<span>»</span>
</li>
@endif
</ul>
</nav>
@endif
Use this Tailwind CSS pagination view in your Blade file by replacing:
{{ $users->links('pagination::bootstrap-5') }}
with:
{{ $users->links('pagination::tailwind') }}
Conclusion
Paginating multiple lists on the same Blade file in Laravel 11 is straightforward with the right approach. By using separate query parameters for each paginated list and styling your pagination links with Bootstrap 5 or Tailwind CSS, you can create a clean and user-friendly interface.
This setup ensures that each list’s pagination is independent, providing a smooth user experience. Whether you prefer Bootstrap or Tailwind for styling, Laravel’s flexibility allows you to customize the pagination to fit your application's design.
Feel free to tweak the provided examples to better fit your specific needs and don’t forget to check out the Laravel Pagination Documentation for more advanced usage.
Subscribe to my newsletter
Read articles from Asfia Aiman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfia Aiman
Asfia Aiman
Hey Hashnode community! I'm Asfia Aiman, a seasoned web developer with three years of experience. My expertise includes HTML, CSS, JavaScript, jQuery, AJAX for front-end, PHP, Bootstrap, Laravel for back-end, and MySQL for databases. I prioritize client satisfaction, delivering tailor-made solutions with a focus on quality. Currently expanding my skills with Vue.js. Let's connect and explore how I can bring my passion and experience to your projects! Reach out to discuss collaborations or learn more about my skills. Excited to build something amazing together! If you like my blogs, buy me a coffee here https://www.buymeacoffee.com/asfiaaiman