Playwright Testing: How to Run It in the Cloud Using Browserless?
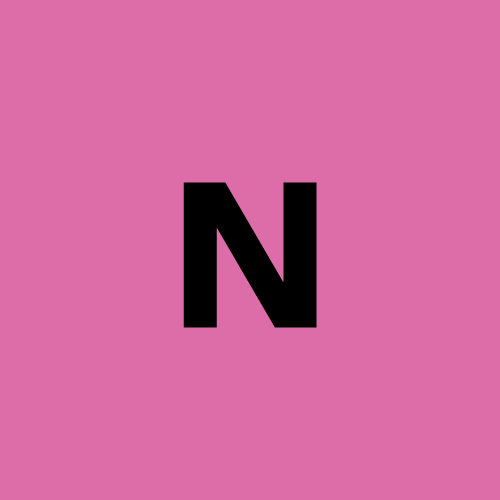
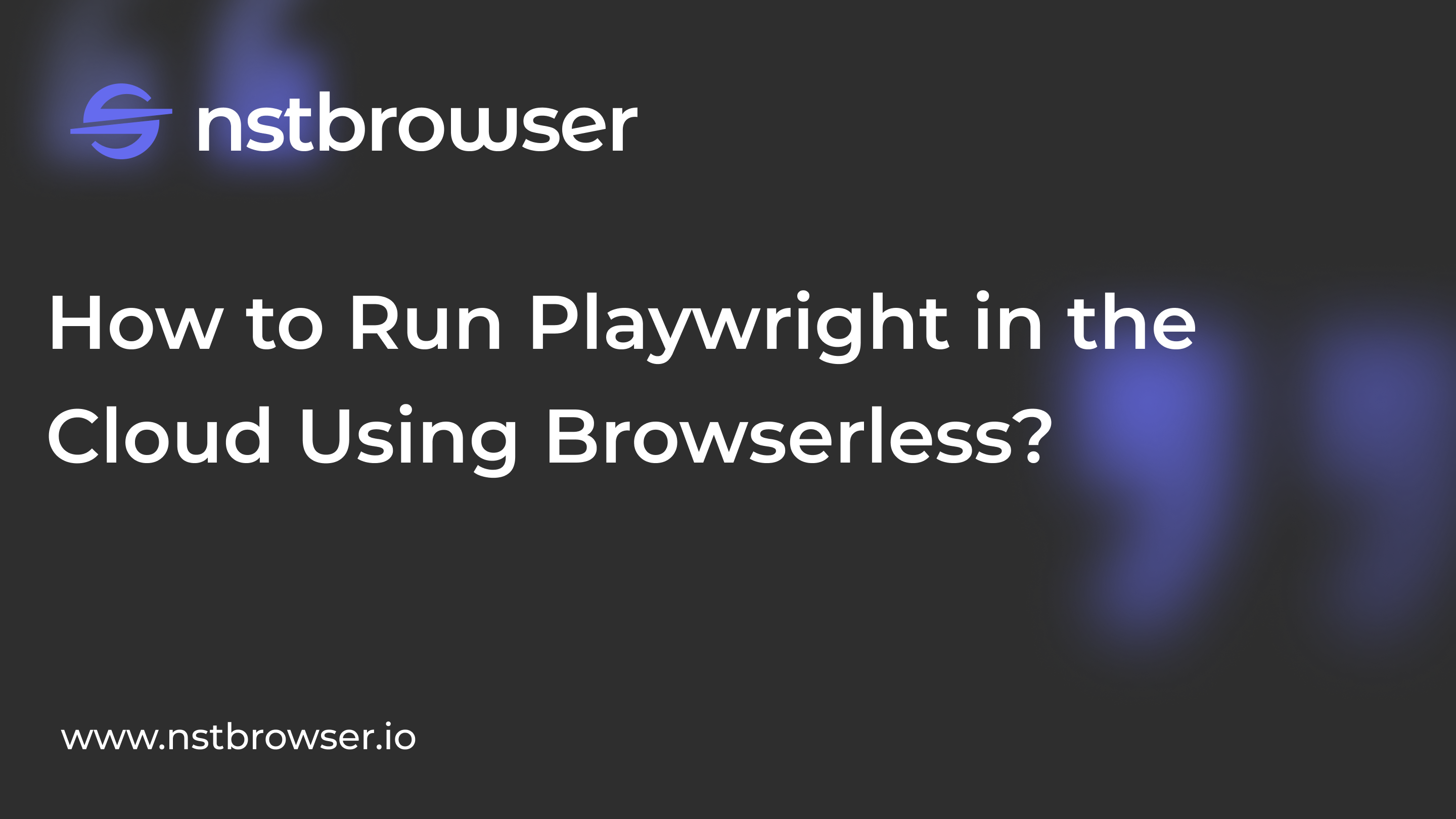
What Is Playwright?
Playwright is a powerful and versatile automation library developed by Microsoft. It enables developers and testers to automate web applications across multiple browsers easily.
Playwright automation supports Chromium, Firefox, and WebKit, allowing you to test across different browsers. It supports programming languages such as Java, Python, C#, and NodeJS. Playwright comes with an Apache 2.0 license and is most popular in NodeJS and Javascript/Typescript.
What is Browserless?
Browserless is a cloud-based browser solution designed for efficient automation, web scraping, and testing. Utilizing Nstbrowser's fingerprint library, Browserless provides random fingerprint switching for smooth data collection and automation processes.
Its robust cloud infrastructure allows for easy management of multiple browser instances, simplifying the handling of automation tasks.
Do you have any wonderful ideas or doubts about web scraping and Browserless? Let's see what other developers are sharing on Discord and Telegram!
Why Choose Playwright Automation?
The advantages of Playwright automation include:
- Cross-browser support: Playwright works with Chromium, Firefox, and WebKit, enabling testing across multiple browsers with a single framework.
- End-to-end testing: It supports full-stack testing from front-end interactions to back-end APIs.
- Headless mode: Playwright can run tests in headless mode for faster execution and better resource efficiency.
- Multi-tab and multi-browser control: It allows for the simultaneous control of multiple tabs and browsers.
- Automatic waits: The Playwright automatically waits for elements to be ready before interacting, reducing flaky tests.
- Network interception: It can intercept network requests, making it easier to simulate different network conditions or mock APIs.
- Cross-platform support: Playwright works on Windows, macOS, and Linux, making it versatile for different environments.
- CI/CD integration support: Playwright supports CI/CD integration. It even provides docker images for some language bindings.
- Typescript support out of the box: Typescript language support requires zero configuration as it can understand your Typescript and JavaScript code.
- Debugging tool support: Playwright tests support different debugging options to make it developer-friendly. Some of the debugging options include Playwright Inspector, VSCode debugger, browser developer tools, and trace viewer console logs.
Playwright Automation Testing frame
The Playwright architecture is designed specifically for browser automation, providing a powerful, flexible, and high-performance framework. It supports multiple browser engines, independent browser environments, and powerful APIs, and is suitable for browser automation tasks such as web crawling and automated testing. Its architecture ensures the reliability, efficiency, and easy maintenance of tests and scripts.
Key Components and Processes
1. Client (Automation Script):
Playwright natively supports JavaScript and TypeScript, and also provides bindings for Java, Python, and C#, allowing users to write automation scripts in these languages. Users write test scripts in their preferred language, including test cases, interactive commands, and assertions. JSON is often used for configuration and data exchange to ensure efficient data transmission.
2. WebSocket connection:
- Handshake: When executing the test script, the client will establish a connection with the Playwright server (Node.js) and perform an initial handshake to ensure the establishment of communication.
- Full-duplex communication: After the connection is established, a two-way communication channel will be opened between the client and the server for sending commands to the browser in real time and receiving responses or events.
- Open and persistent connection: The WebSocket connection will remain open and persistent throughout the session, allowing the test script to continue to interact with the browser.
- Connection closure: When the test is completed, the client or server can close the connection, marking the end of the session.
3. Server-side (Node.js):
- Node.js server: The server side is managed by the Node.js application and coordinates the interaction between the client script and the browser.
- Handling commands and events: The Node.js server receives commands from the client, processes and converts them into browser instructions, listens to events from the browser, and forwards them to the client.
4. Browser Automation (CDP and CDP+):
- CDP (Chrome DevTools Protocol): Playwright uses CDP to interact with Chromium-based browsers. CDP manages the browser's rendering, session, and network processes to ensure smooth page rendering, session management, and network interactions.
- CDP+: For browsers such as Firefox and WebKit (Safari), Playwright uses a protocol extension similar to CDP to ensure consistency of the API across different browsers. Each browser process (such as rendering, and network) is precisely managed to simulate the actual interaction of users.
How to run Playwright automation in the Cloud using Browserless?
Environment Preset
Before we start, we need to have a Browserless service. Using Browserless can solve complex web crawling and large-scale automation tasks, and it has now achieved fully managed cloud deployment.
Browserless adopts a browser-centric approach, provides powerful headless deployment capabilities, and provides higher performance and reliability. For more information about Browserless, you can click here to learn more. Get the API KEY and go to the Browserless menu page of the Nstbrowser client, or you can click here to access
Determine the test goal
Before we start, let's determine the goal of this test. We will use Playwright
to perform form validation and obtain the error information provided by the website. From the normal page access interaction, we will experience:
- Visit the target website https://www.airbnb.com/.
- Click the "Personal Center Panel" in the upper right corner.
- Click the "Continue" button in the form to trigger the form submission.
- View the error feedback information.
Project initialization
Follow the steps below to install Playwright and run the test script
Step 1: Create a new folder in Vs code
Step 2: Open the Vs code terminal and run the following command
npm init -y
npm install playwright @playwright/test
Step3: Create related files
playwright.config.ts
file
import { defineConfig } from '@playwright/test';
export default defineConfig({
// test files to run
testDir: './tests',
// timeout for each test
timeout: 30 * 1000,
// timeout for the entire test run
expect: {
timeout: 30 * 1000,
},
// Reporter to use
reporter: 'html',
// Glob patterns or regular expressions to ignore test files.
testIgnore: '*test-assets',
// Glob patterns or regular expressions that match test files.
testMatch: '*tests/*.spec.ts',
});
tests/browserless.spec.ts
test scripts- Launching the browser requires passing in the relevant configuration (for complete configuration, see LaunchNewBrowser)
- The
proxy
parameter is required
import { chromium } from 'playwright';
import { test, expect, Page } from '@playwright/test';
let page: Page;
async function createBrowser() {
const token = 'your api token'; // required
const config = {
proxy:
'your proxy', // required; input format: schema://user:password@host:port eg: http://user:password@localhost:8080
// platform: 'windows', // support: windows, mac, linux
// kernel: 'chromium', // only support: chromium
// kernelMilestone: '124', // support: 113, 120, 124
// args: {
// '--proxy-bypass-list': 'detect.nstbrowser.io',
// }, // browser args
// fingerprint: {
// userAgent:
// 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/128.0.6613.85 Safari/537.36',
// },
};
const query = new URLSearchParams({
token: token, // required
config: JSON.stringify(config),
});
const browserWSEndpoint = `ws://less.nstbrowser.io/connect?${query.toString()}`;
const browser = await chromium.connectOverCDP(browserWSEndpoint);
const context = await browser.newContext();
const page = await context.newPage();
return page;
}
function logger(text: string) {
console.log(text);
}
test.beforeEach(async () => {
if (!page) {
page = await createBrowser();
}
await page.goto('https://www.airbnb.com/');
});
test.describe('Browserless Test', () => {
test('Verify Airbnb Website Login Error Message', async () => {
try {
logger(`go to the target page ${await page.url()}`);
// judge the title of the page
const title = await page.title();
logger(`page title: ${title}`);
// wait for select the button aria-label="Main navigation menu"
await page.waitForSelector('[aria-label="Main navigation menu"]');
// click the button aria-label="Main navigation menu"
await page.click('[aria-label="Main navigation menu"]');
// wait for the a text is Sign up
await page.waitForSelector('a:has-text("Sign up")');
// click the a text is Sign up
await page.click('a:has-text("Sign up")');
await page.click('span:has-text("Continue")');
// // get the error message by id="phone-number-error-phone-login"
const text = await page.textContent('#phone-number-error-phone-login');
logger(`error message: ${text}`);
expect(text).toBe('Phone number is required.');
await page.close();
await page.context().close();
} catch (error) {
console.error(error);
}
});
});
The project file structure is as follows
Project execution
After the above project initialization, we can start running our project! Now open the Vs code terminal and run:
npx playwright test
The commands here can be mainly broken down into
- Use the
npx
command to execute the playwright command - Playwright will find the playwright.config.ts configuration file of the current project and obtain basic configuration information
- Based on the content of the configuration file, find the matching test file in the current project directory and start the execution
Result output
After executing the above command, we will see the following output results. Here, each important result of the test execution process is output, and we can see that our test case has passed.
We can view our test report by running the following command:
npx playwright show-report
The report shows in detail each step of the execution command, execution status, and final output results.
It's a Wrap
Playwright provides a powerful and versatile test automation framework, making it a valuable tool for developers and testers. Its ability to handle multiple browsers, provide cross-platform support, and provide rich interaction and verification APIs make it stand out in the field of test automation.
Through the comprehensive step-by-step tutorial in this blog, we learned:
- The power of Browserless
- How to easily crawl data with Browserless
- Meaningful result analysis
Combined with Browserless, users can leverage the power of Playwright to create reliable, efficient, and scalable automated tests, simplifying the testing process, reducing manual workload, and ensuring a more powerful and seamless user experience.
Subscribe to my newsletter
Read articles from nstbrowser directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
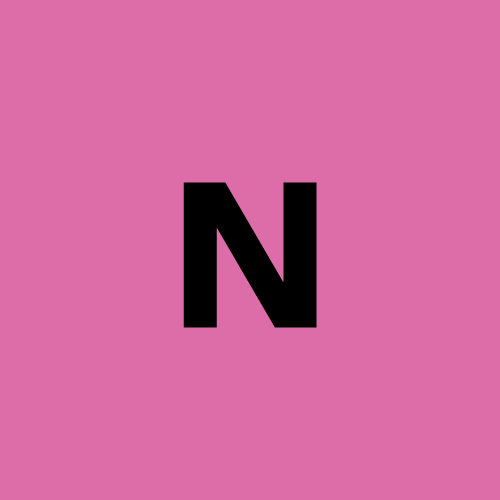