Dependency Injection Made Easy to Understand

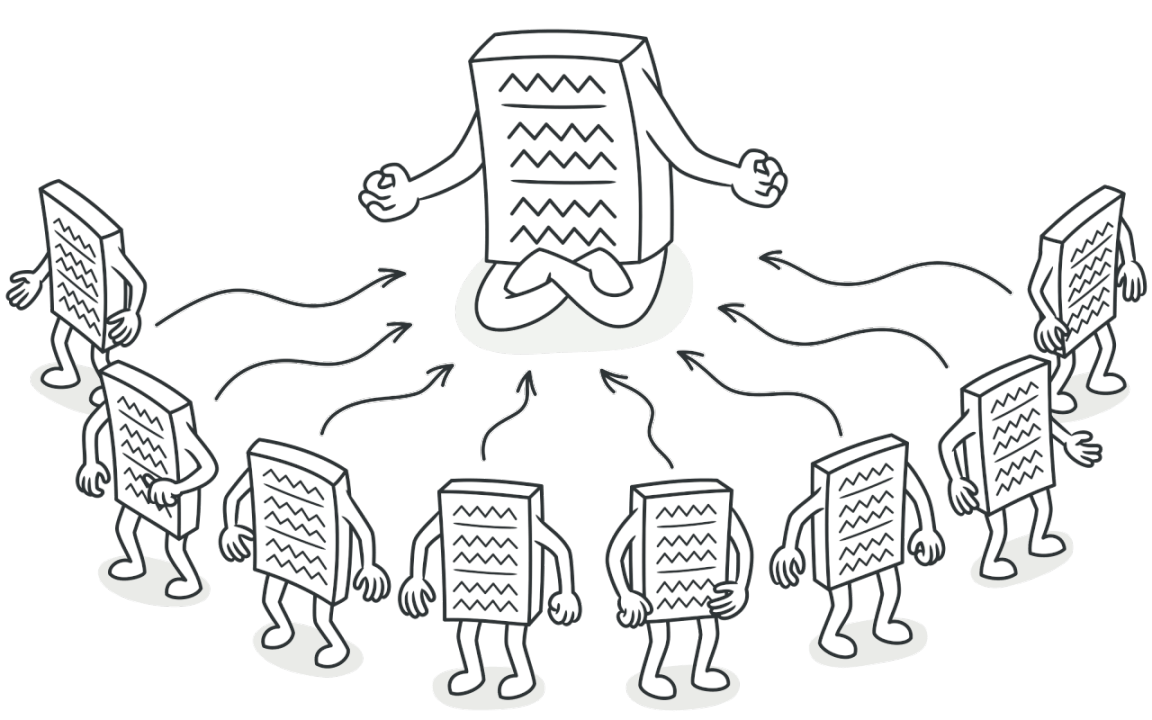
In software development, dependencies are like the ingredients in a recipe. Each ingredient serves a specific purpose, and without the right combination, your dish might not turn out the way you want it to.
Imagine you're baking a cake. You need flour, sugar, eggs, and other ingredients to create that fluffy, delectable treat. Similarly, in software development, different components rely on each other to function properly. These dependencies can be services, repositories, or even configurations.
So, how do we manage these dependencies effectively? That's where dependency injection comes into play.
Dependency Injection: The Secret Ingredient for Flexible Code
Dependency injection is a software design pattern that allows you to inject dependencies into your classes or components instead of creating them within the code itself. This might sound a bit abstract, but let's break it down:
Creating Dependencies: Just like you don't grow flour or sugar in your kitchen, you don't create dependencies within your code. Instead, you rely on external sources, like libraries or frameworks, to provide these dependencies.
Injecting Dependencies: Imagine you're following a cake recipe. You don't mix the ingredients directly into the oven; you add them one by one into the mixing bowl. Similarly, dependency injection allows you to pass dependencies into your code, giving you more control over how they are used.
Types of Dependencies: Understanding the Flavors
There are different types of dependencies, each with its own role:
Constructor Injection: Constructor injection is a popular and widely used method of dependency injection. It involves injecting dependencies into the constructor of a class. This approach ensures that the dependencies are always available to the class and are initialized before the class is used.
In this example, the UserService class requires a EmailService dependency to send emails. The dependency is injected into the UserService constructor, ensuring that the UserService always has access to the EmailService instance.
Setter Injection: Setter injection involves injecting dependencies into a class using setter methods. This approach allows for more flexibility in setting up dependencies, as the dependencies can be set after the class has been instantiated.
In this example, the UserService class also requires a EmailService dependency, but the dependency is injected using a setter method instead of the constructor. This allows the UserService instance to be configured with different EmailService implementations without modifying its constructor.
Interface Injection: Interface injection is a dependency injection technique in which dependencies are injected into a class through an interface. This means that the class does not need to know the concrete implementation of the dependency, it only needs to know the interface that the dependency must implement. Python doesn't have interfaces as a built-in language feature, so it can achieve the interface injection using abstract classes that define the abstract methods that do not have an implementation.
In this example, the UserService class requires a EmailService dependency, but the dependency must conform to the EmailService interface. This ensures that the UserService can work with any implementation of the EmailService interface, as long as it implements the required methods.
Benefits of Dependency Injection: A Tastier Treat
Dependency injection offers several benefits, making your code more:
Testable: By decoupling components, you can test them independently, ensuring each ingredient works as expected.
Flexible: Easily swap out or modify dependencies without affecting the rest of your code, like switching from vanilla to chocolate frosting.
Maintainable: Keeping dependencies separate makes your code easier to understand and modify, like following a clear recipe.
Conclusion: Baking Better Code with Dependency Injection
Dependency injection is like a baking technique that simplifies the process of creating delicious software. By understanding dependencies and how to inject them effectively, you can write code that is flexible, testable, and maintainable, making your software development journey a breeze. Happy coding!
Subscribe to my newsletter
Read articles from Nishorgo Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
