Tokenization with NLTK: A Deep Dive into the Fundamentals of Text Processing
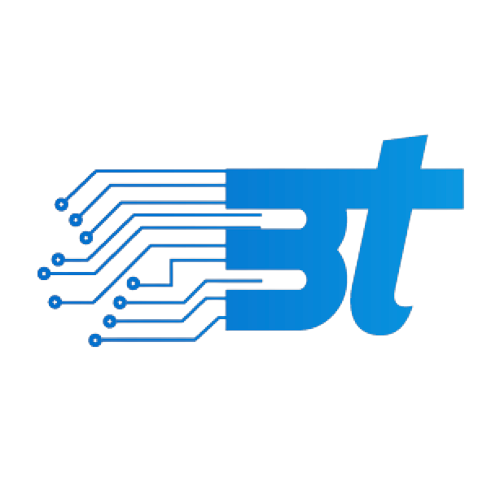
Table of contents
- 1. What is Tokenization?
- 2. Why Tokenization is Important
- 3. Different Types of Tokenization
- 4. Setting Up NLTK for Tokenization
- 5. Word Tokenization with NLTK
- 6. Sentence Tokenization with NLTK
- 7. Custom Tokenization with Regular Expressions
- 8. Tokenizing Text in Different Languages
- 9. Removing Stop Words
- 10. Tokenization in Real-World Applications
- References
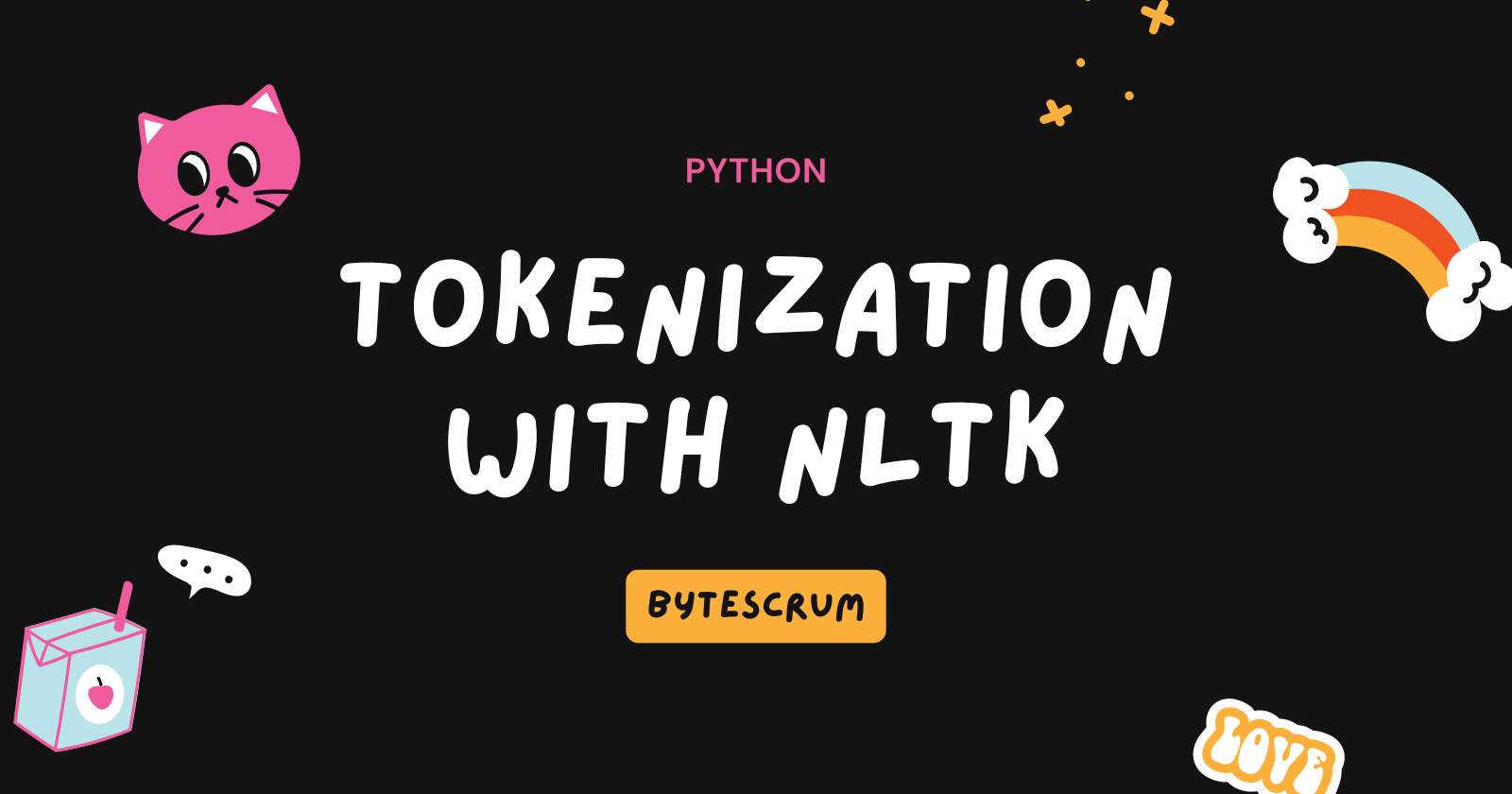
Natural Language Processing (NLP) has become an essential aspect of modern technology, powering everything from chatbots to sentiment analysis systems, voice assistants, and search engines. One of the foundational steps in NLP is tokenization, which involves breaking down text into manageable pieces for further analysis. These pieces, called tokens, can be words, sentences, or subwords.
In this extensive guide, we will dive deep into tokenization, explaining what it is, why it is important, and how to implement it in Python using the Natural Language Toolkit (NLTK). By the end of this blog, you’ll be well-equipped to handle tokenization tasks and apply them to real-world projects.
1. What is Tokenization?
Tokenization is the process of splitting text into smaller units, or "tokens." These tokens can be individual words, sentences, or even subwords. Tokenization is one of the most fundamental steps in preparing text data for various NLP tasks such as sentiment analysis, machine translation, text classification, and keyword extraction.
For example:
Sentence Tokenization: Breaking a paragraph into individual sentences.
Word Tokenization: Breaking a sentence into individual words.
How Tokenization Works
When tokenizing a sentence like:
"The quick brown fox jumps over the lazy dog."
Word tokenization would split this sentence into:
['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog']
Why Tokens Matter
Tokens are essential because NLP models cannot work with raw text. They need structured data (i.e., tokens) to analyze the relationships between words, understand the context, and generate meaningful outputs.
2. Why Tokenization is Important
Tokenization serves as the foundation for almost every NLP task. Without proper tokenization, any higher-level text processing would be inaccurate or incomplete. Here’s why tokenization is crucial:
Prepares Text for Processing: Tokenization transforms raw text into a format suitable for analysis.
Facilitates Word Frequency Analysis: Counting word occurrences becomes possible after tokenization.
Improves Machine Learning Models: Most text-based models rely on tokens for input. For instance, a text classifier needs tokenized words to work effectively.
Captures Context: Sentences and words must be tokenized to understand the context, especially for tasks like Named Entity Recognition (NER) and Part-of-Speech (POS) tagging.
3. Different Types of Tokenization
There are various types of tokenization depending on the level of granularity required:
1. Word Tokenization
Word tokenization breaks down a sentence or paragraph into individual words. This is useful when you need to focus on each word’s meaning, frequency, or usage.
Example:
"Tokenization is essential for NLP tasks."
Would be tokenized as:
['Tokenization', 'is', 'essential', 'for', 'NLP', 'tasks', '.']
2. Sentence Tokenization
Sentence tokenization splits text into sentences, which is helpful in tasks like summarization, machine translation, or topic segmentation.
Example:
"I love Python. It is a great programming language."
Would be tokenized into:
['I love Python.', 'It is a great programming language.']
3. Character Tokenization
Character tokenization breaks down text into individual characters. This is less common but can be useful in specific cases like working with languages that don’t use spaces between words, such as Chinese or Japanese.
4. Setting Up NLTK for Tokenization
Before we dive into examples, let's set up NLTK for tokenization. NLTK is one of the most powerful libraries for NLP in Python, offering pre-built tools for a wide range of tasks including tokenization, stemming, POS tagging, and more.
Installing NLTK
First, you need to install NLTK:
pip install nltk
Once installed, you can download the necessary resources:
import nltk
nltk.download('punkt') # Pre-trained tokenizer models for many languages
The punkt
package contains data required for word and sentence tokenization.
5. Word Tokenization with NLTK
Word tokenization is one of the most common forms of tokenization. It breaks text into individual words while also identifying punctuation marks.
Example 1: Basic Word Tokenization
Let’s tokenize a simple sentence:
from nltk.tokenize import word_tokenize
text = "Tokenization is a key step in NLP."
tokens = word_tokenize(text)
print(tokens)
Output:
['Tokenization', 'is', 'a', 'key', 'step', 'in', 'NLP', '.']
In this example, word_tokenize
breaks the sentence into words and punctuation, treating each as a separate token.
Example 2: Tokenizing a Complex Paragraph
Now, let’s tokenize a longer piece of text:
paragraph = """
Tokenization is essential in NLP. It breaks down text for easier processing.
We can analyze text more effectively after tokenizing.
"""
tokens = word_tokenize(paragraph)
print(tokens)
Output:
['Tokenization', 'is', 'essential', 'in', 'NLP', '.', 'It', 'breaks', 'down', 'text', 'for', 'easier', 'processing', '.', 'We', 'can', 'analyze', 'text', 'more', 'effectively', 'after', 'tokenizing', '.']
Here, NLTK efficiently splits the paragraph into individual words and punctuation marks.
6. Sentence Tokenization with NLTK
Sentence tokenization splits a paragraph or document into sentences. This is particularly useful in tasks like text summarization and question-answering systems where sentence boundaries matter.
Example 3: Basic Sentence Tokenization
Let’s tokenize a paragraph into sentences:
from nltk.tokenize import sent_tokenize
text = "Tokenization is essential. It helps in many NLP tasks."
sentences = sent_tokenize(text)
print(sentences)
Output:
['Tokenization is essential.', 'It helps in many NLP tasks.']
NLTK uses punctuation marks like periods, exclamation points, and question marks to identify sentence boundaries.
7. Custom Tokenization with Regular Expressions
In some cases, you may need to customize tokenization for specific needs, such as handling specific punctuation or text structures. NLTK allows you to define your own tokenization rules using regular expressions.
Example 4: Custom Tokenization
Here’s how to tokenize text based on custom rules:
from nltk.tokenize import regexp_tokenize
text = "Hello World! Let's tokenize this sentence with custom rules."
# Custom pattern: Words, contractions, and punctuations are considered separate tokens
pattern = r"\w+|[^\w\s]+"
tokens = regexp_tokenize(text, pattern)
print(tokens)
Output:
['Hello', 'World', '!', 'Let', "'s", 'tokenize', 'this', 'sentence', 'with', 'custom', 'rules', '.']
In this example, the regular expression pattern splits words and punctuation into separate tokens while keeping contractions intact.
8. Tokenizing Text in Different Languages
Tokenization in languages other than English is just as essential. NLTK’s punkt
package supports various languages, including French, German, and Italian. This enables multilingual tokenization.
Example 5: Tokenizing French Text
Let’s tokenize a French sentence:
french_text = "La tokenisation est importante. Elle aide à analyser le texte."
tokens = word_tokenize(french_text)
print(tokens)
Output:
['La', 'tokenisation', 'est', 'importante', '.', 'Elle', 'aide', 'à', 'analyser', 'le', 'texte', '.']
As you can see, NLTK can handle languages with accents and other non-English characters.
9. Removing Stop Words
After tokenizing text, the next step in many NLP tasks is to remove stop words. Stop words are common words like "the", "is", "in", which often do not add significant meaning to the text. NLTK provides a list of stop words for various languages.
Example 6: Removing Stop Words
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
text = "This is a simple sentence to demonstrate removing stop words."
tokens = word_tokenize(text)
# Filter out stop words
filtered_tokens = [word for word in tokens if word.lower() not in stop_words]
print(filtered_tokens)
Output:
['This', 'simple', 'sentence', 'demonstrate', 'removing', 'stop', 'words', '.']
Removing stop words helps focus on the more important content in the text, making analysis more meaningful.
10. Tokenization in Real-World Applications
Tokenization plays a critical role in several real-world applications:
Chatbots: Tokenizing user input to understand queries and formulate appropriate responses.
Sentiment Analysis: Tokenizing text to classify it as positive, negative, or neutral.
Search Engines: Tokenizing search queries to retrieve relevant documents.
Machine Translation: Tokenizing sentences in one language to translate them into another.
By accurately breaking down text into manageable pieces, tokenization helps lay the groundwork for more advanced NLP tasks.
Conclusion
In this guide, we explored the basics of tokenization, its different types, and how to implement it using NLTK. We also discussed advanced concepts like custom tokenization and removing stop words. With this knowledge, you’re now ready to apply tokenization to your own projects and dive deeper into the exciting world of NLP.
Next steps could include:
Exploring more complex text preprocessing techniques.
Applying tokenization in machine learning models.
Working with tokenization for specific languages or domains.
Tokenization is just the beginning. Stay tuned for more NLP tutorials that will take your text processing skills to the next level!
References
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
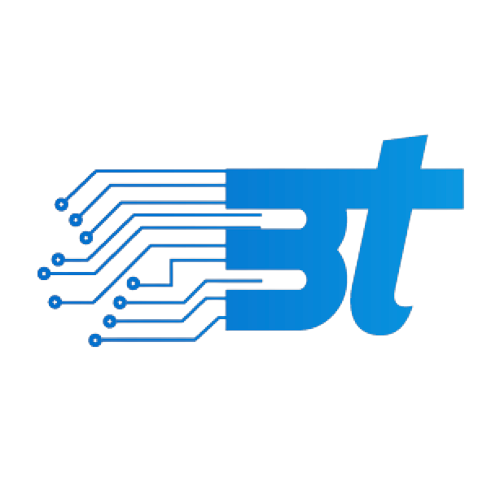
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.