How to connect the Golang app with MongoDB — Connect Infosoft
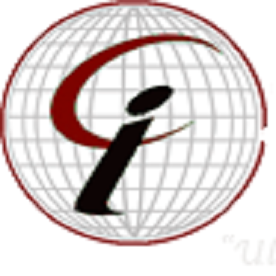
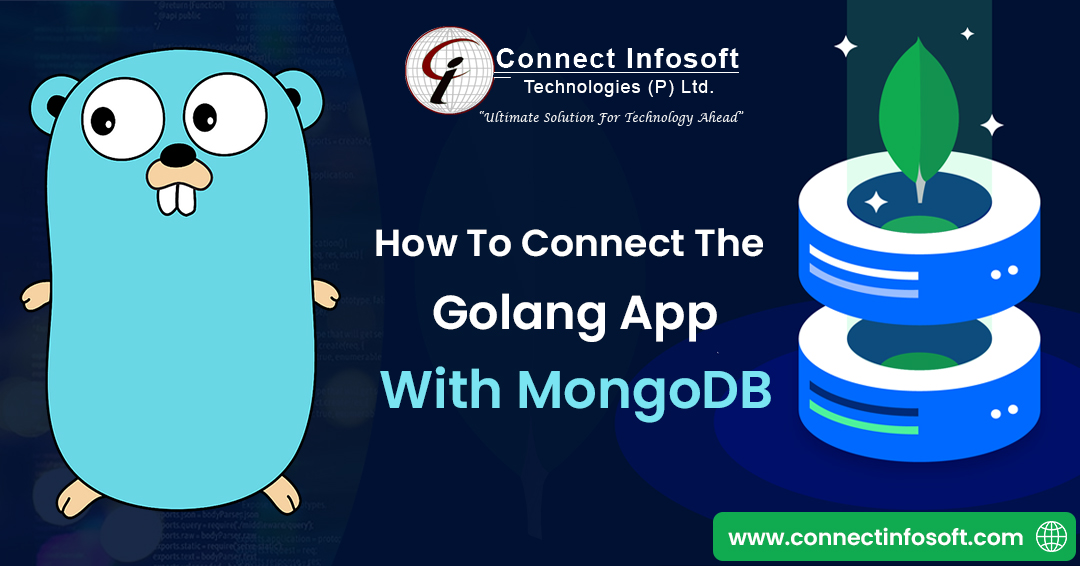
In today’s tech landscape, integrating databases with applications is a fundamental requirement. MongoDB, a popular NoSQL database, provides a flexible and scalable approach to data storage. For developers using Golang (Go), connecting your application to MongoDB can enhance its capability to handle various data types efficiently. In this comprehensive guide, we’ll walk you through the process of connecting a Golang app with MongoDB, leveraging Connect Infosoft's best practices and recommendations.
1. Understanding MongoDB and Golang
Before diving into the connection process, it's essential to understand what MongoDB and Golang offer:
- MongoDB: A NoSQL database known for its flexibility, scalability, and ability to handle unstructured data. It stores data in JSON-like documents, making it ideal for applications requiring dynamic schemas.
- Golang: A statically typed, compiled language developed by Google, known for its simplicity, efficiency, and concurrency support. It's particularly well-suited for building high-performance applications and services.
2. Prerequisites
Before connecting Golang with MongoDB, ensure you have the following:
- Go installed: Download and install Go from the [official website](https://golang.org/dl/).
- MongoDB installed: Set up MongoDB on your local machine or use a cloud-based service like MongoDB Atlas.
- MongoDB Go Driver: The official Go driver for MongoDB, known as mongo-go-driver
, is required to interface with the database.
3. Setting Up Your Golang Environment
3.1. Install Go
If you haven’t already installed Go, follow the installation instructions from the [Go website](https://golang.org/doc/install).
3.2. Create a New Go Project
Open your terminal and create a new directory for your project:
```bash
mkdir my-go-mongodb-app
cd my-go-mongodb-app
go mod init my-go-mongodb-app
```
This command initializes a new Go module, which will help manage your dependencies.
4. Installing the MongoDB Go Driver
Install the MongoDB Go driver using Go’s package manager:
```bash
go get go.mongodb.org/mongo-driver/mongo
go get go.mongodb.org/mongo-driver/bson
```
These packages include the core MongoDB driver and BSON (Binary JSON) support needed for handling MongoDB documents.
5. Connecting to MongoDB
5.1. Import Required Packages
In your Go file (e.g., main.go
), import the necessary packages:
```go
package main
import (
"context"
"fmt"
"log"
"go.mongodb.org/mongo-driver/bson"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/mongo/options"
)
```
5.2. Create a MongoDB Client
Initialize a MongoDB client and establish a connection to the database:
```go
func main() {
// Set up a connection URI
uri := "mongodb://localhost:27017"
// Create a new MongoDB client
client, err := mongo.Connect(context.TODO(), options.Client().ApplyURI(uri))
if err != nil {
log.Fatalf("Failed to connect to MongoDB: %v", err)
}
defer client.Disconnect(context.TODO())
fmt.Println("Connected to MongoDB!")
}
```
5.3. Access a Database and Collection
Once connected, access a specific database and collection:
```go
// Access the database and collection
db := client.Database("mydatabase")
collection := db.Collection("mycollection")
```
5.4. Performing CRUD Operations
Create a Document
Insert a new document into the collection:
```go
func insertDocument(collection *mongo.Collection) {
doc := bson.D{{"name", "John Doe"}, {"age", 30}}
_, err := collection.InsertOne(context.TODO(), doc)
if err != nil {
log.Fatalf("Failed to insert document: %v", err)
}
fmt.Println("Document inserted successfully!")
}
```
Read Documents
Query documents from the collection:
```go
func findDocuments(collection *mongo.Collection) {
cur, err := collection.Find(context.TODO(), bson.D{})
if err != nil {
log.Fatalf("Failed to find documents: %v", err)
}
defer cur.Close(context.TODO())
for cur.Next(context.TODO()) {
var result bson.D
if err := cur.Decode(&result); err != nil {
log.Fatalf("Failed to decode document: %v", err)
}
fmt.Println(result)
}
}
```
Update a Document
Update an existing document:
```go
func updateDocument(collection *mongo.Collection) {
filter := bson.D{{"name", "John Doe"}}
update := bson.D{{"$set", bson.D{{"age", 31}}}}
_, err := collection.UpdateOne(context.TODO(), filter, update)
if err != nil {
log.Fatalf("Failed to update document: %v", err)
}
fmt.Println("Document updated successfully!")
}
```
Delete a Document
Remove a document from the collection:
```go
func deleteDocument(collection *mongo.Collection) {
filter := bson.D{{"name", "John Doe"}}
_, err := collection.DeleteOne(context.TODO(), filter)
if err != nil {
log.Fatalf("Failed to delete document: %v", err)
}
fmt.Println("Document deleted successfully!")
}
```
6. Error Handling and Best Practices
- Error Handling: Ensure proper error handling at each stage of the database interaction to avoid crashes and unexpected behavior.
- Context Management: Use context for timeout and cancellation to manage database operations effectively.
- Connection Pooling: MongoDB handles connection pooling internally, but understanding this can help optimize performance for high-traffic applications.
- Security: Use environment variables for sensitive information like database URIs and credentials. Ensure that MongoDB is properly secured, especially in production environments.
Conclusion
Connecting a Golang app with MongoDB involves setting up your development environment, installing necessary drivers, and performing CRUD operations using the MongoDB Go driver. By following these steps and best practices, you can effectively leverage MongoDB’s powerful features within your Go applications, ensuring robust data handling and improved performance.
For any additional support or custom development needs, Connect Infosoft is here to help you harness the full potential of MongoDB and Golang in your projects. Feel free to reach out to us for expert advice and solutions tailored to your business requirements.
Tags: How to connect the Golang app with MongoDB, Looking for Golang Development Service Company in India, Connect Infosoft, Golang Web Development Company, Looking for Golang developers in India, Hire Golang Development Company India, Hire Golang Developers Team Company
Subscribe to my newsletter
Read articles from Connect Infosoft directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
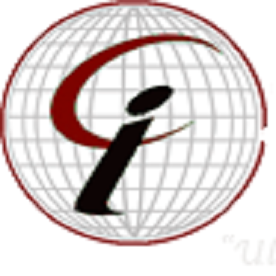
Connect Infosoft
Connect Infosoft
Connect Infosoft Technologies Pvt. Ltd. provides Website design and Mobile App development services to global clients. Connect Infosoft has more than 25 years of experience in software development with a strong focus on mobile app development for all kinds of platforms including iOS and Android. We were established in 1999, and have been serving our customers everywhere throughout the world. Connect Infosoft's Head Office is based in New Delhi, India, and has Branch Office in Orissa. It also has a portrayal in the United States. In business for more than 25 years, we are constantly prepared for confronting any kind of challenge. Major Service Offerings: -Web Application Development -ETL Services -SaaS & MVP Development -Mobile App Development -Data Science & Analytics -Artificial Intelligence -Digital Marketing -Search Engine Optimization -Pay-Per-Click advertising campaigns -Blockchain -DevOps -Amazon Web Services -Product Engineering -UI/UX Founder of Connect Infosoft Mr. Sanjay Sahoo with his astounding and clear vision has achieved numerous accolades around the world for his excellence in the field of IT. We can make world-class ventures, which upgrade the organization brand image colossally. We work in close coordination in light of the customers, bearing in mind doing outstanding and quality work. Expert Developer Award from Digital Dujour and Best Developer from Pepsico, NY, US honors are confirmation of this truth. Kindly message me for an in-depth conversation. Book a Free 30-minute consultation with our experts: https://calendly.com/connectinfosoft Contact Number: +1 323-522-5635 Email: info@connectinfosoft.com Visit us: https://www.connectinfosoft.com/ Send us your Queries: https://www.connectinfosoft.com/lets-work-together/