Master PHP Cookies & Sessions: A Complete Guide
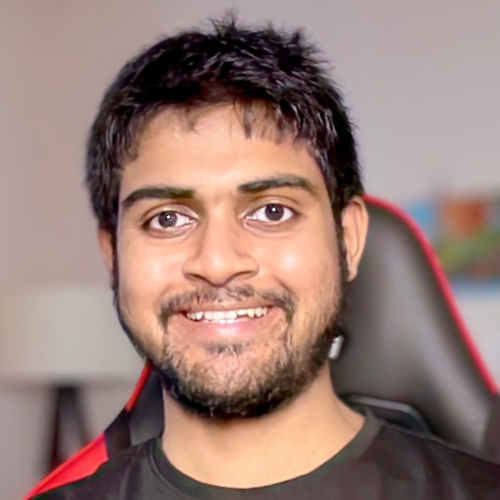
Table of contents
- What Are Cookies and Sessions Anyway? πͺπ¨βπ»
- Let's Break It Down with Code! π€
- Setting a Cookie
- Reading a Cookie
- Deleting a Cookie
- 2. PHP Sessions β The Short-Term Memory of the Server
- Starting a Session
- Accessing Session Data
- Destroying a Session
- Real-World Use Case for Sessions π¨βπ»
- Cookie vs. Session: When to Use What? π€
- Fun Challenge Time! π
- Common Pitfalls to Avoid π¨
- Wrapping Up with a Cookie πͺ and a Session π¨βπ»
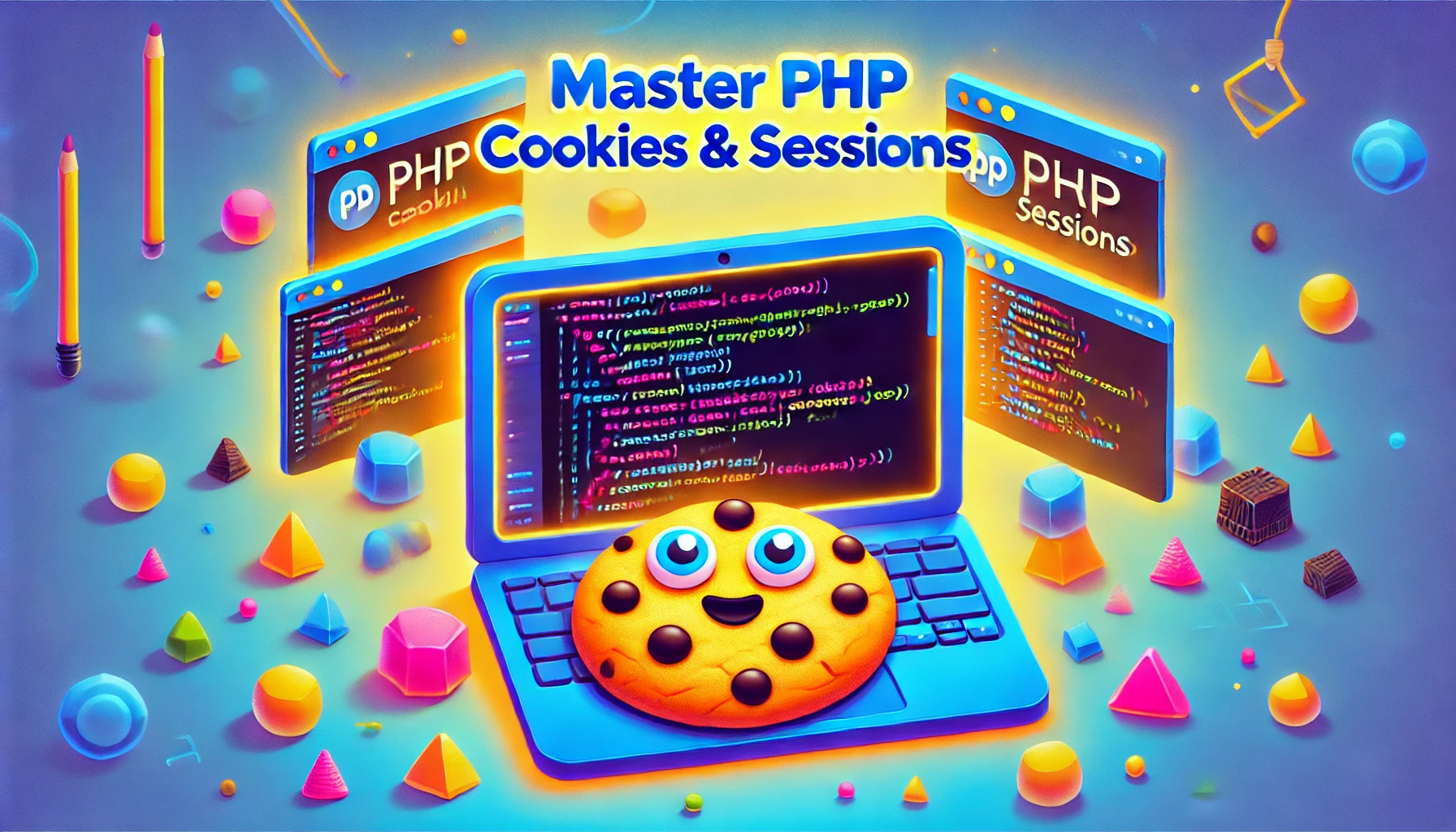
Hey there, future web dev superstars! π Welcome to a fun, exciting, and oh-so-practical tutorial on PHP cookies and sessions! By the end of this, you'll be handling user data like a pro and will know when to use cookies or sessions in real-world web applications. Letβs make this feel like a fun chat rather than a dry coding lecture, shall we?
What Are Cookies and Sessions Anyway? πͺπ¨βπ»
Imagine you're at your favorite coffee shop (weβll call it WebDev CafΓ©). Every time you visit, the barista (your web server) needs to know what kind of coffee you like (your data). If you don't have a way to tell them, you'd have to repeat your order every time you walk through the door. Thatβs where cookies and sessions come in!
Cookies are like leaving a note for the barista every time you leave the shop. "Hey, Junaid likes a double-shot latte with caramel drizzle!" They remember you the next time you walk in.
Sessions are like the barista remembering your order while youβre in the shop. "Junaid is still here, I know his order until he leaves!"
Got the picture? πͺβ‘οΈ Cookies store info directly on the user's browser. π¨βπ»β‘οΈ Sessions store it on the server while the user is still hanging around.
Let's Break It Down with Code! π€
1. PHP Cookies β The Little Notes Your Browser Stores
A cookie is a small file that the server sends to the user's browser, and the browser stores it. Next time the user visits the website, the cookie is sent back to the server to identify them.
Setting a Cookie
<?php
// Set a cookie named "username" with the value "Junaid"
setcookie("username", "Junaid", time() + 86400, "/"); // Expires in 1 day
?>
setcookie()
: This is the function that sets the cookie.username
: The name of the cookie.Junaid
: The value of the cookie.time() + 86400
: How long the cookie should last (in this case, one day)./
: Makes the cookie available site-wide.
Reading a Cookie
<?php
// Check if the cookie is set
if(isset($_COOKIE["username"])) {
echo "Welcome back, " . $_COOKIE["username"] . "!";
} else {
echo "Hello, guest!";
}
?>
Deleting a Cookie
<?php
// Delete the cookie by setting its expiration time to the past
setcookie("username", "", time() - 3600, "/");
?>
Real-World Use Case for Cookies πͺ
Remembering User Preferences: Letβs say your website has a dark mode and light mode. You can store the userβs theme preference in a cookie so the next time they visit, you load the site with the same theme!
2. PHP Sessions β The Short-Term Memory of the Server
Now, a session is like a memory that sticks around only while the user is still active on your site. Sessions are great when you need to store sensitive data securely because everything is saved on the server, not the user's machine.
Starting a Session
<?php
// Start the session
session_start();
// Store session data
$_SESSION["username"] = "Junaid";
?>
session_start()
: This function must be called at the beginning of every page that uses sessions.$_SESSION["username"]
: Stores the value "Junaid" under the key "username".
Accessing Session Data
<?php
session_start(); // Always start the session
if(isset($_SESSION["username"])) {
echo "Hello, " . $_SESSION["username"] . "!";
} else {
echo "Session data not found.";
}
?>
Destroying a Session
<?php
session_start();
// Unset all session variables
session_unset();
// Destroy the session
session_destroy();
?>
Real-World Use Case for Sessions π¨βπ»
User Login System: When a user logs into your site, you donβt want to store sensitive information like passwords in cookies. Instead, store the user's session ID on the server to maintain their login status until they log out or their session expires.
Cookie vs. Session: When to Use What? π€
Cookies are great when you want to remember something long-term (like remembering the user's language preference or theme choice).
Sessions are perfect for temporary, sensitive information (like login status or cart items in an online store).
Pro Tip: Use sessions for things like login systems and sensitive user data because they store the information on the server. For things like non-sensitive preferences (e.g., theme or language), use cookies!
Fun Challenge Time! π
Letβs make this interactive! Try creating a simple login system using both cookies and sessions.
Challenge: Cookie-Based "Remember Me" System
Create a login form.
On successful login, ask the user if they want to be remembered (checkbox).
If the user checks "Remember Me", set a cookie with their username.
Next time they visit, greet them by name using the cookie.
Challenge: Session-Based Login
Create a login form.
Store the userβs session information on successful login.
Display a welcome message when they are logged in.
Add a logout button to end the session.
This is an excellent way to see cookies and sessions in action!
Common Pitfalls to Avoid π¨
Cookie Size: Remember, cookies can only store up to 4KB of data. So donβt store too much in them!
Session Timeouts: If sessions time out too quickly, users may get logged out unexpectedly. Set an appropriate session timeout period based on your use case.
Security Considerations: Be cautious about storing sensitive data in cookies. Always use HTTPS to prevent session hijacking.
Wrapping Up with a Cookie πͺ and a Session π¨βπ»
By now, you're armed with the power of cookies and sessions! They may seem like small tools, but they pack a punch when it comes to handling user data and enhancing the overall user experience on your website.
With cookies, you can save preferences and make your site feel personalized. With sessions, you can safely manage user data during their visit. Now, go build something awesome! π
Did you enjoy this PHP deep dive into cookies and sessions? π Share your thoughts in the comments or let me know what other fun topics you'd love to explore next. Let's keep the coding party going! π¨βπ»π»πͺ
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
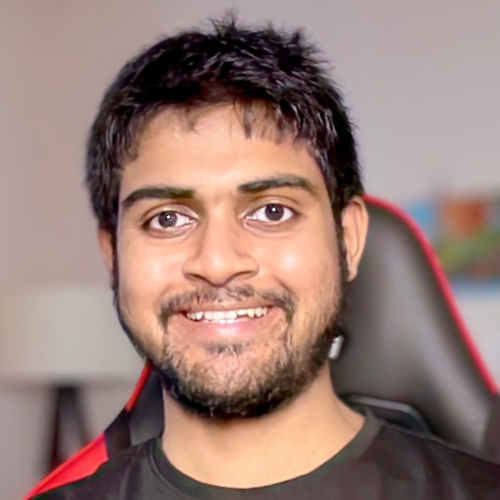
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.