Docker Containers: Running, Managing, and Debugging—A Detailed Guide for Beginners

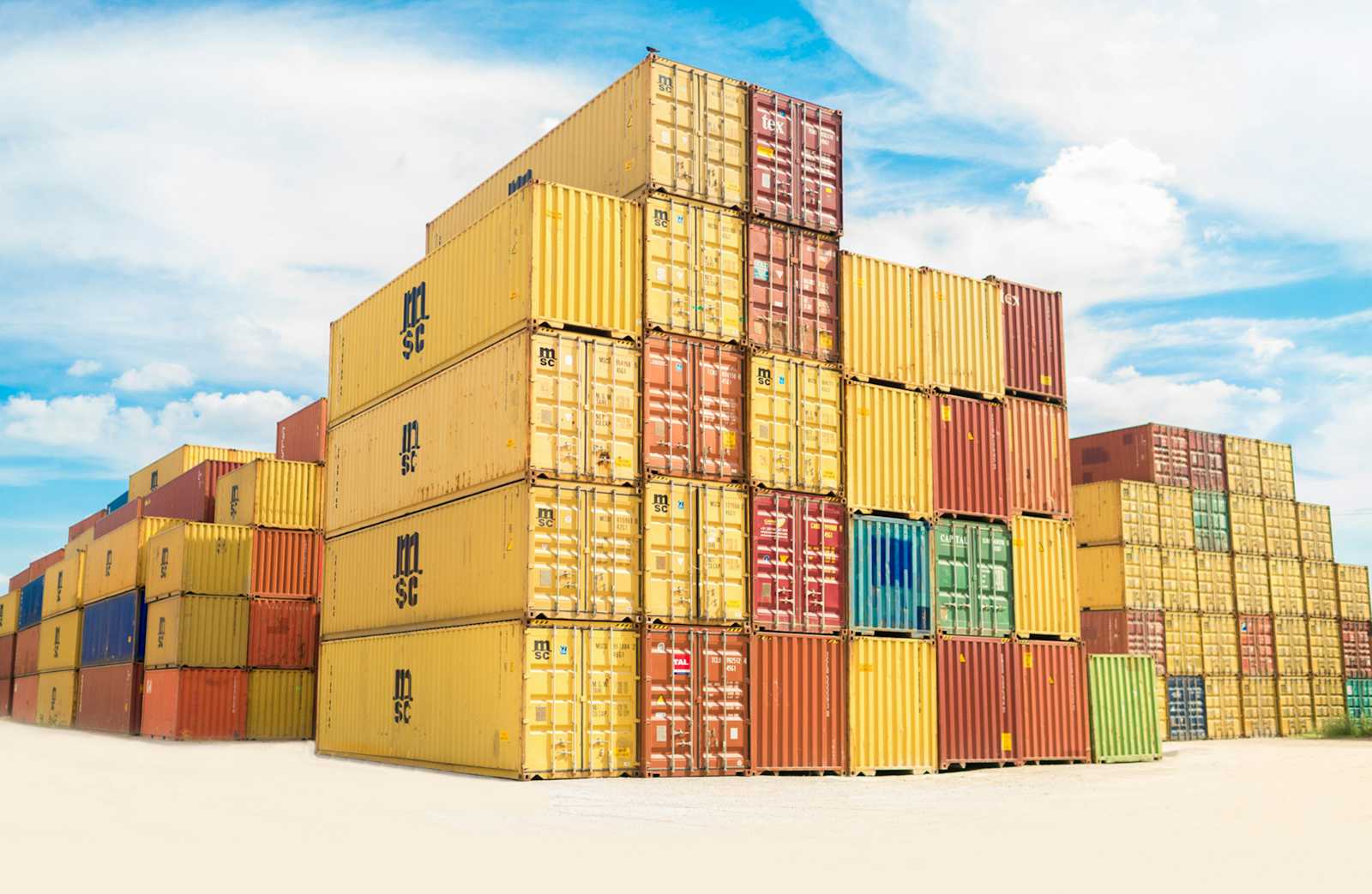
Hey there, welcome back to my Docker series! If you’ve been following along, we’ve already covered some important topics like Docker Basics (what containers are and how they work) and Docker Images (how to write a Dockerfile and build images). Now it’s time to talk about Docker Containers—the real stars of the show.
If Docker images are like blueprints or recipes, Docker containers are the actual thing in action: the app running in its own little environment, completely independent of everything else. In this post, we’re going to learn:
How to run containers.
How to manage container lifecycles (start, stop, restart, remove).
How to view logs and debug containers when things go wrong.
So let’s dive in and break everything down with real-life examples to make it easy to understand. Ready? Let’s go!
Why Are Containers So Important?
Before jumping into commands, let’s understand why containers are so important. Think of containers like renting your own little apartment:
You can decorate it however you like (set up your app).
It’s fully isolated from other apartments (containers don’t interfere with each other).
You can lock it up and leave (stop or remove it), and when you come back, it’ll be exactly how you left it (start it again from where you stopped).
In the world of software, containers allow you to run applications in isolated environments, making sure they don’t clash with other apps on your system. This isolation is the key to why containers are so popular—they’re predictable and stable. No more "works on my machine" issues!
Running a Container: The Basics with docker run
The most common command you’ll use is docker run
. This is the magic command that starts a container from a Docker image. Think of it as turning the key to move into your freshly rented apartment.
Command Breakdown
docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
Let’s say you want to run a basic web server in a container using the official Nginx image (which is like a pre-built blueprint for a web server). The command would look like this:
docker run -d -p 8080:80 nginx
Here’s what’s going on:
-d
: This flag means "detached," so the container runs in the background. Think of it like turning on a light and walking away—it keeps running even if you’re not watching.-p 8080:80
: This maps the container’s port 80 (where the web server listens) to port 8080 on your computer. Now, when you visithttp://localhost:8080
, you’ll see the web server running inside the container!nginx
: This is the image we’re using. It's like saying, "I want to use this pre-furnished apartment."
Just like that, we’ve created and run a web server inside a container with a single command! 🎉
Running Commands Inside a Container: docker exec
Now imagine you’re inside your new apartment, but you want to run some tasks, like maybe changing the wallpaper (i.e., running a command inside the container). For that, we use docker exec
.
Let’s say you want to open a terminal inside the running Nginx container to explore its files:
docker exec -it <container_id> /bin/bash
This command gives you a bash shell inside the container. It’s like walking into the apartment and interacting with everything inside.
Managing the Container Lifecycle: Starting, Stopping, and Removing Containers
Once you’ve run a container, you’ll want to know how to manage it—like how to stop it, restart it, or remove it when you’re done. This is what we call the container lifecycle.
1. Creating a Container
When you use docker run
, Docker creates the container for you automatically. But if you want to prepare everything without running it right away, you can use the docker create
command. It’s like leasing an apartment, furnishing it, but not moving in just yet.
docker create <image_name>
This command prepares the container, but doesn’t start it. You’ve got your apartment ready, but you’re not living in it yet.
2. Starting a Container
Once the container is created, you can start it whenever you’re ready:
docker start <container_name_or_id>
This is like finally moving into your apartment and turning on the lights. The container is now up and running.
3. Stopping a Container
Let’s say you’re done for the day and want to stop the container (like turning off the lights and leaving the apartment for a while):
docker stop <container_name_or_id>
The container stops running, but everything inside is preserved. You can come back and start it again whenever you need.
4. Restarting a Container
Need to quickly restart the container without fully stopping it? You can restart it like rebooting your computer:
docker restart <container_name_or_id>
This is like turning the lights off and on quickly, so everything resets but the setup remains the same.
5. Removing a Container
When you no longer need the container, you can remove it:
docker rm <container_name_or_id>
This is like packing up, moving out, and cleaning the apartment—there’s no trace of you left behind. However, you can always create a new container from the image if needed.
Pro Tip: If you want to stop and remove the container all at once, you can use:
docker rm -f <container_name_or_id>
This forces the removal of a running container—like quickly clearing out the apartment.
Viewing Logs and Debugging Containers
One of the best things about Docker is its ability to keep track of everything happening inside your container. If something goes wrong, Docker gives you tools to troubleshoot, just like checking security cameras or maintenance reports in an apartment.
Viewing Logs: docker logs
If your app inside the container is misbehaving, you can check the logs to see what’s going on. It’s like checking the maintenance reports for any issues in your apartment.
docker logs <container_name_or_id>
This command shows you all the logs from the container. If you want to keep an eye on the logs as they come in (like watching live security footage), you can use the -f
flag to follow the logs:
docker logs -f <container_name_or_id>
Inspecting a Container: docker inspect
For a more detailed view of what’s going on inside your container (its network, environment variables, configurations, etc.), use the docker inspect
command:
docker inspect <container_name_or_id>
This command gives you all the technical details about the container, like getting a full blueprint of your apartment’s wiring, plumbing, and layout. Super helpful for troubleshooting!
Real-Life Example: Debugging a Web App in a Container
Imagine you’re running a simple web app inside a container, but it’s not working, and you’re not sure why. Here’s how you could debug it:
Check the logs: Run
docker logs <container_id>
to see if any error messages pop up. Maybe the app crashed because a library is missing, or a file path is incorrect.Run commands inside the container: If the logs aren’t helpful, run
docker exec -it <container_id> /bin/bash
to open a shell inside the container. Now you can manually inspect the files, run commands, or even install missing software to troubleshoot the issue.Inspect the container: Use
docker inspect <container_id>
to check for any configuration issues, such as incorrect environment variables or network settings that might be causing the problem.
With these tools, debugging Docker containers becomes much easier—just like figuring out what went wrong in an apartment based on maintenance reports or physical inspections.
Wrapping It Up
And there you have it—your deep dive into Docker containers! We’ve covered:
How to run containers with
docker run
.How to manage their lifecycle (start, stop, restart, remove).
How to view logs and debug when things go wrong.
Docker containers are powerful, flexible, and make working with apps easy and efficient. They’re like your own little apartments for running applications—isolated, easy to set up, and ready to go whenever you need them.
In our next post, we’ll explore how containers communicate with each other and the outside world through Docker networking. So stay tuned for that! 🐳
And if you missed my previous posts on Docker Basics or Docker Images, be sure to check them out—they’ll give you a solid foundation as you continue your Docker journey.
If you have any questions or thoughts, drop them in the comments, or reach out to me on Twitter! Let’s learn Docker together! 👨💻
Subscribe to my newsletter
Read articles from Shivam Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shivam Jha
Shivam Jha
LFX'24 @Kyverno | Web Dev | DevOps | OpenSource | Exploring Cloud Native Technologies.