Chapter 5: Advanced CSS (Part 2) - Pseudo-Selectors, Hover Effects, and CSS States
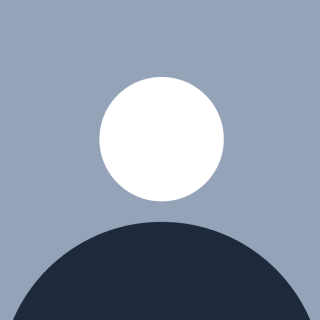
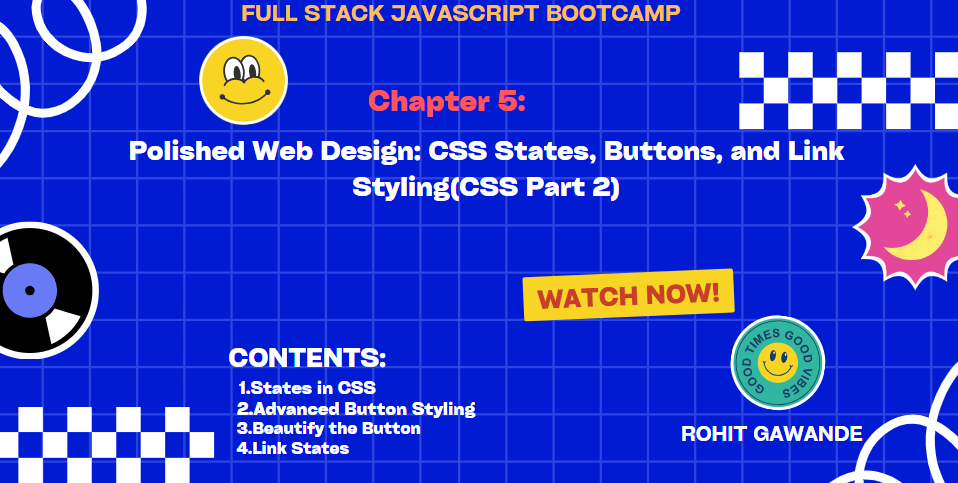
Welcome to the fifth chapter of the "Full Stack JavaScript BootCamp" series! In the previous chapter, we explored CSS fundamentals such as selectors and pseudo-selectors (:before
and :after
). Today, we’ll dive deeper into pseudo-selectors, hover states, and the practical styling of HTML elements. Before starting, let's recap the key concepts from the last session.
Recap of Chapter 4: Key Takeaways
Selectors in CSS: Target elements based on their ID, class, attributes, or position in the DOM.
Pseudo-Selectors: Add content or style dynamically before or after an element.
::before
and::after
are powerful tools for injecting additional content.
Practical Use Case of
::before
:.imp-label::before { content: 'YouTube '; display: inline; background-color: orange; border-radius: 10px; }
Output: Adds a "YouTube" label before the associated element.
1. Introduction to CSS Pseudo-Selectors
1.1 What are Pseudo-Selectors?
Pseudo-selectors are CSS constructs that allow you to style elements based on their state or position without altering the HTML structure. They inject styles before or after an element, or apply styles based on the element's state.
1.2 Understanding Element States
- Element State refers to the different conditions or interactions an element may have. For example, an element may be in its normal state, or it might be hovered over by the cursor.
1.3 Examples of Pseudo-Selectors
1.3.1 The :hover
Pseudo-Selector
The :hover
pseudo-selector applies styles when an element is being hovered over by the cursor.
Code Example:
Example: Hover Effect Using ::before
.imp-label:hover::before {
content: ' ';
display: block;
width: 20px;
height: 20px;
background-color: orange;
border-radius: 10px;
}
Explanation:
On hover, the
::before
pseudo-selector injects a circular orange block.Removing
:hover
applies a static content style.
.email:hover {
background-color: #089258;
padding: 4px;
}
Explanation:
.email:hover
: Targets the.email
class when hovered.background-color: #089258;
: Changes the background color on hover.padding: 4px;
: Adds padding to the element.
Output: When the user hovers over an element with the class .email
, the background color changes to green, and padding is applied.
1.3.2 The :focus
Pseudo-Selector
The :focus
pseudo-selector applies styles to an element when it gains focus, such as when a user clicks on an input field.
Code Example:
input:focus {
background-color: #800b0b;
color: #f1f3ed;
}
Explanation:
input:focus
: Targets the input field when focused.background-color: #800b0b;
: Changes background color to dark red when focused.color: #f1f3ed;
: Changes text color to a light shade.
Output: When an input field is focused, it will have a dark red background and light text color.
1.4 Using Pseudo-Selectors with Links
Different pseudo-selectors can style links based on their state:
Code Example:
a:hover {
background-color: #c41212;
color: aquamarine;
}
a:visited {
background-color: #715891;
}
a:link {
color: #11091b;
}
Explanation:
a:hover
: Styles links when hovered with a red background and aquamarine text.a:visited
: Styles visited links with a dark background.a:link
: Styles unvisited links with a specific color.
Output: Links change color depending on whether they are hovered over, visited, or unvisited.
2. Styling Buttons with Pseudo-Selectors
2.1 Button Hover Effects
Buttons often use :hover
to improve user interaction.
Code Example:
<button class="btn btn-primary" type="submit">Submit</button>
.btn-primary:hover {
background-color: #0e19be;
color: aqua;
padding: 30px 10px;
border-radius: 5px;
font-size: 14px;
}
Explanation:
.btn-primary:hover
: Applies styles to.btn-primary
on hover.background-color: #0e19be;
: Changes background to blue.color: aqua;
: Changes text color.padding: 30px 10px;
: Adjusts padding.border-radius: 5px;
: Rounds corners.font-size: 14px;
: Sets font size.
Output: The button background turns blue, text becomes aqua, padding increases, and the corners are rounded on hover.
2.2 Using Bootstrap for Styling
Bootstrap provides pre-designed styles that can be easily integrated into your project.
Code Example:
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet">
<button class="btn btn-primary" type="submit">Submit</button>
<button class="btn btn-warning" type="reset">Reset</button>
Explanation:
Bootstrap Link: Includes Bootstrap CSS from CDN.
btn-primary
andbtn-warning
: Bootstrap classes for styling buttons.
Output: Buttons styled using Bootstrap’s classes are automatically given predefined styles.
2.3 Overriding Bootstrap Styles
You can customize Bootstrap styles by overriding them.
Code Example:
.btn-warning:hover {
background-color: white;
}
Explanation:
.btn-warning:hover
: Customizes thebtn-warning
class on hover to change the background color to white.
Output: The btn-warning
button’s hover effect changes the background color to white.
3. Understanding CSS Layouts and Positions
3.1 CSS Box Model
Margin and padding are key concepts in the CSS box model.
Margin: Space outside the border.
Padding: Space inside the border.
Code Example:
.container {
margin: 50px;
height: 50px;
width: 150px;
}
.container:hover {
background-color: #e30707;
}
Explanation:
.container
: Applies a margin of 50px, with specific height and width.background-color: #e30707;
: Changes background color on hover.
Output: The .container
element has a margin around it and changes background color when hovered.
3.2 Positioning Elements
Positioning attributes control the placement of elements.
Code Example:
.Box1 {
height: 250px;
width: 250px;
border: solid black 5px;
border-radius: 33px;
position: fixed;
}
.Box2 {
height: 250px;
width: 250px;
border: solid black 5px;
border-radius: 33px;
background-color: #0a118d;
position: sticky;
}
Explanation:
position: fixed;
: Positions.Box1
fixed relative to the viewport.position: sticky;
: Positions.Box2
based on scroll position within its container.
Output: .Box1
remains fixed in the viewport, while .Box2
sticks to its position within its scrollable container.
4. Advanced CSS Properties
4.1 Float Property
The float property controls the alignment of elements within their containers.
Code Example:
li {
border: 1px solid black;
height: 70px;
width: 70px;
float: right;
}
Explanation:
float: right;
: Aligns list items to the right within their container.
Output: List items are aligned to the right.
4.2 nth-child Selector
nth-child
allows targeting specific children of a parent element.
Code Example:
li:nth-child(even) {
color: #c41212;
background-color: #120202;
}
li:nth-child(odd) {
background-color: #c41212;
color: #120202;
}
li:first-child {
background-color: #c41212;
color: #120202;
}
li:last-child {
background-color: #c41212;
color: #120202;
}
Explanation:
nth-child(even)
: Styles even-numbered list items.nth-child(odd)
: Styles odd-numbered list items.first-child
andlast-child
: Styles the first and last list items.
Output: List items alternate in color based on their position, with specific styles for the first and last items.
5. CSS Priority and Specificity
5.1 Understanding CSS Priority
CSS rules are applied based on their specificity and priority:
Inline Styles: Highest priority.
Internal Styles: Defined within the
<style>
tag in HTML.External Styles: Defined in external stylesheets.
5.2 CSS Shorthand Properties
Shorthand properties streamline CSS by combining related properties.
Example:
padding: 30px 10px 30px 10px;
Explanation:
- Top, Right, Bottom, Left: Padding values in a clockwise sequence.
Output: Padding is applied around an element according to the specified values.
Conclusion
In this revision session, we've delved into the intricacies of CSS pseudo-selectors, exploring how they influence the appearance and behavior of web elements. By understanding selectors like :hover
, :focus
, and :nth-child
, we've equipped ourselves with powerful tools to enhance web design and improve user experience. These insights not only refine our CSS skills but also empower us to create more dynamic and engaging web pages.
Appreciation
A big thank you to everyone who has followed along and contributed to this learning journey! Your enthusiasm and feedback are invaluable in making these sessions insightful and impactful. If you have any questions or need further clarification, feel free to reach out. Let’s continue to learn and grow together in the ever-evolving world of web development. 🌟
Related Posts in My Series:
Chapter 1:Mastering the Web: A Journey Through HTML and Beyond
Other Series:
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repository and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊