Advanced Redux: Async Actions, Middleware, and Best Practices for Scalable State Management
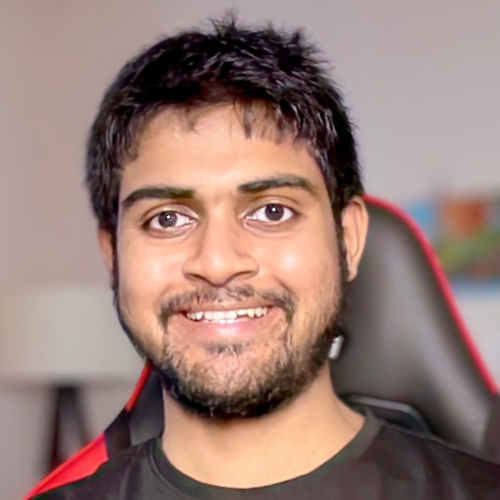
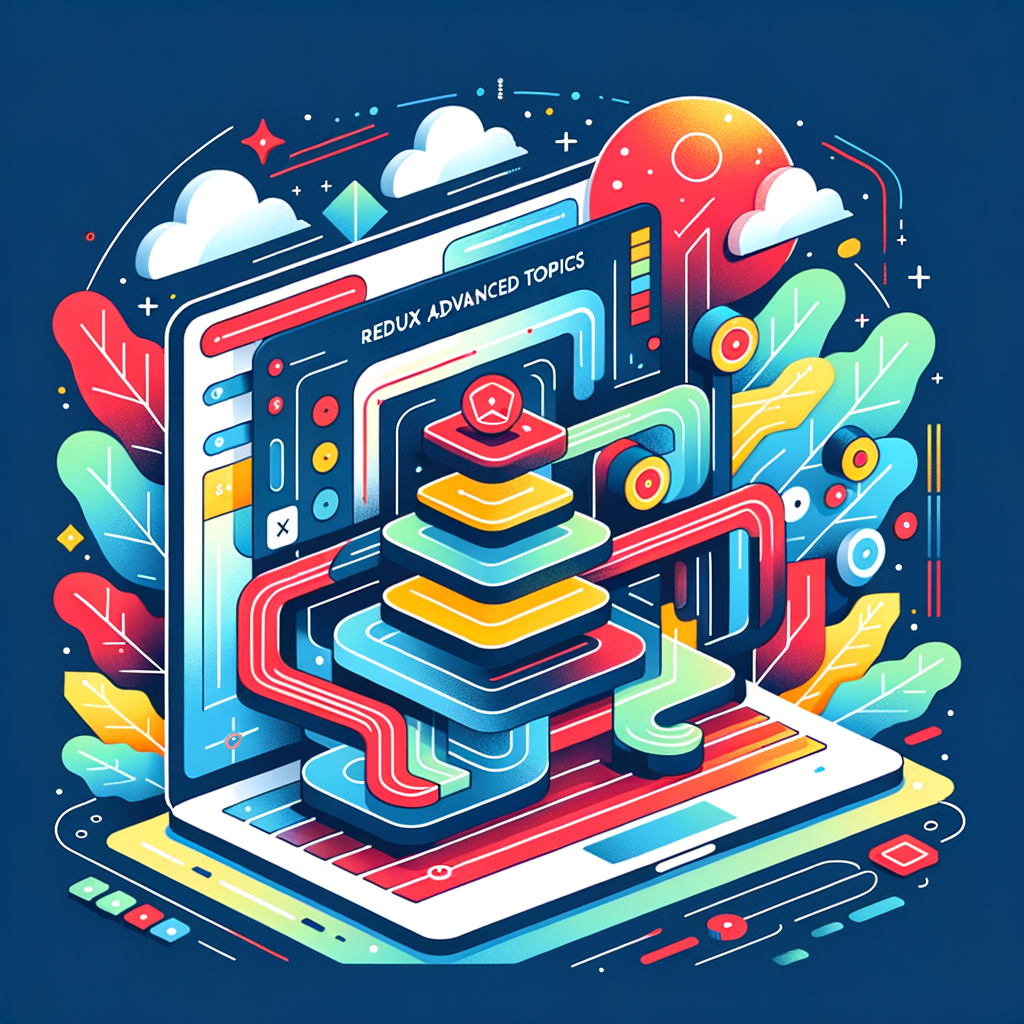
So, you’ve mastered the basics of Redux Core and can manage synchronous state changes like a pro. But as your application grows, you’ll start encountering scenarios where Redux’s core features aren’t enough. To scale your Redux logic efficiently, handle asynchronous operations, and organize your state management better, it’s time to explore advanced Redux concepts.
In this post, we’ll cover:
Middleware for handling side effects.
Combining reducers for modular code.
Asynchronous actions with Redux Thunk.
Using Redux DevTools for easier debugging.
Enforcing immutability with best practices.
Practical tips for scaling your Redux architecture.
If you’re still getting familiar with the fundamentals of Redux, I recommend reading my article Mastering Redux Core: The Complete Guide to State Management with Plain JavaScript. There, you’ll learn how Redux works at its core, covering the store, actions, reducers, and dispatch in pure JavaScript. Once you have that foundation, come back here to dive deeper into these advanced concepts.
Middleware in Redux
Middleware is a way to extend Redux’s functionality and handle side effects like logging, making asynchronous API calls, or modifying actions before they reach the reducer.
Why Middleware?
Middleware sits between the action being dispatched and the reducer handling it. It intercepts actions, allowing you to:
Log actions and states for debugging purposes.
Handle asynchronous logic (e.g., API calls).
Conditionally modify or block actions before they reach the reducer.
Example: Logger Middleware
To make it easier to log actions and state changes, you can use redux-logger
, a middleware that automatically logs every dispatched action and the resulting state.
Install redux-logger
:
npm install redux-logger
After installing, you can add redux-logger
to your store configuration.
You can apply middleware to your Redux store using applyMiddleware()
:
const { createStore, applyMiddleware } = require('redux');
const logger = require('redux-logger').createLogger(); // redux-logger needs to be initialized
// Reducer
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
default:
return state;
}
};
// Create store with logger middleware
const store = createStore(counterReducer, applyMiddleware(logger));
// Dispatch an action
store.dispatch({ type: 'INCREMENT' });
Now every action dispatched and the updated state will be automatically logged in the console by redux-logger
.
Redux Thunk for Asynchronous Actions
Redux Thunk is the most popular middleware for handling asynchronous actions. It allows you to dispatch functions (instead of plain objects) to make API requests or perform side effects asynchronously.
In this section, I'll show you how to make an API call using Redux Thunk and Axios. Axios is a popular HTTP client for making requests, while Redux Thunk allows us to handle asynchronous logic in Redux by dispatching actions at different stages of an API request.
To use Axios and Redux Thunk in your project, you first need to install them. Run the following command in your project directory:
npm install axios redux-thunk
This installs both Axios for making HTTP requests and Redux Thunk for handling async actions in Redux.
Example: Fetching Data with Redux Thunk
Here’s an example of how to fetch data using Axios within a Redux Thunk action creator:
const { createStore, applyMiddleware } = require('redux');
const {thunk} = require('redux-thunk');
const axios = require('axios');
// Initial state
const initialState = {
data: [],
loading: false,
error: '',
};
// Reducer
const dataReducer = (state = initialState, action) => {
switch (action.type) {
case 'FETCH_DATA_REQUEST':
return {
...state,
loading: true,
};
case 'FETCH_DATA_SUCCESS':
return {
...state,
loading: false,
data: action.payload,
};
case 'FETCH_DATA_FAILURE':
return {
...state,
loading: false,
error: action.payload,
};
default:
return state;
}
};
// Thunk action creator for fetching data
const fetchData = () => {
return async (dispatch) => {
dispatch({ type: 'FETCH_DATA_REQUEST' });
try {
const response = await axios.get(
'https://jsonplaceholder.typicode.com/posts'
);
dispatch({ type: 'FETCH_DATA_SUCCESS', payload: response.data });
} catch (error) {
dispatch({ type: 'FETCH_DATA_FAILURE', payload: error.message });
}
};
};
// Create store with thunk middleware
const store = createStore(dataReducer, applyMiddleware(thunk));
// Subscribe to the store to log the state changes
store.subscribe(() => {
console.log('Updated state:', store.getState());
});
// Dispatch the fetchData action
store.dispatch(fetchData());
Breaking It Down:
Axios Installation: First, you need to install Axios and Redux Thunk in your project by running:
npm install axios redux-thunk
Initial State: The initial state contains:
data
: An array to store the fetched data.loading
: A boolean flag to indicate if the data is currently being loaded.error
: A string to store any error messages if the request fails.
Reducer: The
dataReducer
listens for three types of actions:FETCH_DATA_REQUEST
: Dispatched before the API call is made, signaling the beginning of a loading state.FETCH_DATA_SUCCESS
: Dispatched when the data is successfully fetched, updating the state with the fetched data.FETCH_DATA_FAILURE
: Dispatched if the API call fails, updating the state with the error message.
Thunk Action Creator (
fetchData
): This is an asynchronous action that uses Axios to fetch data from the APIhttps://jsonplaceholder.typicode.com/posts
. It dispatches:FETCH_DATA_REQUEST
before making the request to indicate that loading has started.FETCH_DATA_SUCCESS
when the request is successful, with the data received from the API.FETCH_DATA_FAILURE
if there is an error, with the error message.
Store Creation: The store is created with
createStore
, using thedataReducer
andredux-thunk
middleware. This allows asynchronous actions to be dispatched through Redux Thunk.Dispatching the Action: Finally, the
fetchData
action is dispatched. Redux Thunk allows us to handle asynchronous logic, and Axios makes the API call. The state is updated depending on whether the API request succeeds or fails.
Why Use Axios?
Axios is an easy-to-use HTTP client that simplifies making API requests in JavaScript. It supports methods like GET
, POST
, PUT
, and DELETE
, making it a powerful tool for handling API requests in Redux applications. Additionally, it provides features like request cancellation, interceptors, and automatic transformation of JSON responses.
For more details on Axios and its full capabilities, check out the official Axios documentation.
Combining Reducers
As your application grows, managing all your state with a single reducer becomes impractical. Redux provides combineReducers()
, which allows you to split your reducers into smaller, more manageable pieces.
Example: Combining Reducers
Let’s say we’re managing counter
and user
state separately. We’ll create separate reducers for each and combine them:
const { createStore, combineReducers } = require('redux');
// Counter reducer
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 }; // Copy existing state and update 'count'
default:
return state;
}
};
// User reducer
const userReducer = (state = { name: 'Anonymous' }, action) => {
switch (action.type) {
case 'SET_USER':
return { ...state, name: action.payload }; // Copy existing state and update 'name'
default:
return state;
}
};
// Combine reducers
const rootReducer = combineReducers({
counter: counterReducer,
user: userReducer
});
// Create store
const store = createStore(rootReducer);
// Dispatch actions
store.dispatch({ type: 'INCREMENT' });
store.dispatch({ type: 'SET_USER', payload: 'John Doe' });
console.log(store.getState()); // { counter: { count: 1 }, user: { name: 'John Doe' } }
By using combineReducers()
, you ensure that each slice of your state is managed independently, keeping your code modular and maintainable.
Redux DevTools for Debugging
If you’ve been working with Redux, you’ll want to make your life easier by using Redux DevTools. It allows you to inspect actions, monitor state changes, and time-travel through state transitions, making debugging a breeze.
Setting Up Redux DevTools
To enable Redux DevTools in your application, add this line when creating the store:
const store = createStore(
rootReducer,
window.__REDUX_DEVTOOLS_EXTENSION__ && window.__REDUX_DEVTOOLS_EXTENSION__()
);
Once you add this, you’ll be able to open the Redux DevTools extension in your browser and track all state and action changes.
Enforcing Immutability in Redux
Immutability is a key principle in Redux. You must ensure that the state is never mutated directly, but rather, you return a new copy of the state.
Example: Enforcing Immutability
In Redux reducers, you typically use the spread operator (...
) to copy the state immutably:
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return {
...state, // Spread operator to copy the state
count: state.count + 1
};
default:
return state;
}
};
Tools to Help with Immutability
While the spread operator works for shallow state objects, if your state becomes more complex, you can use libraries like Immer or Immutable.js to handle deep immutability.
Best Practices for Scaling Redux
As your Redux app grows, it’s important to follow best practices for scalability, maintainability, and organization. Here are a few tips:
1. Use Constants for Action Types
Define action types as constants to avoid typos and keep your code consistent:
const INCREMENT = 'INCREMENT';
const DECREMENT = 'DECREMENT';
// Action creators
const incrementAction = () => ({ type: INCREMENT });
const decrementAction = () => ({ type: DECREMENT });
2. Organize by Feature
Instead of separating files by reducers, actions, and constants, organize your code by feature. This keeps things modular and easier to maintain as your app grows.
/src
/features
/counter
- counterActions.js
- counterReducer.js
/user
- userActions.js
- userReducer.js
3. Selectors for Encapsulating State Access
Use selectors to abstract away the structure of your state from the components. This ensures that components don’t depend on the specific shape of the state.
const getCount = (state) => state.counter.count;
4. Normalize State
When managing complex, nested state structures, use normalized data patterns to flatten your state, which simplifies updates and retrievals.
Conclusion
By mastering middleware for handling side effects, learning how to combine reducers to manage state modularly, understanding asynchronous actions with Redux Thunk, using Redux DevTools for debugging, enforcing immutability, and following best practices, you’ll be well-equipped to build scalable Redux applications.
If you’re new to Redux or need to refresh the basics, check out my Mastering Redux Core: The Complete Guide to State Management with Plain JavaScript. Once you’re comfortable with Redux core, dive into these advanced topics to take your state management to the next level!
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
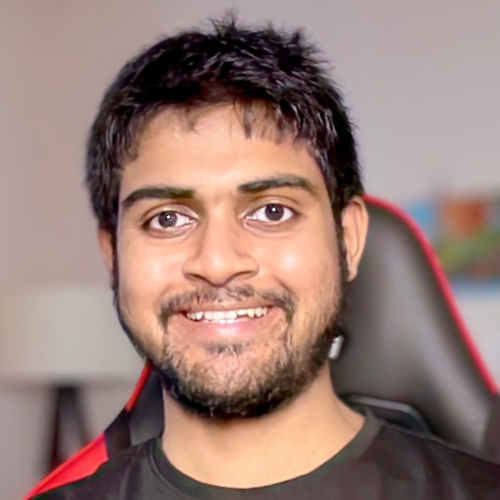
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.