Spring Boot & Repository
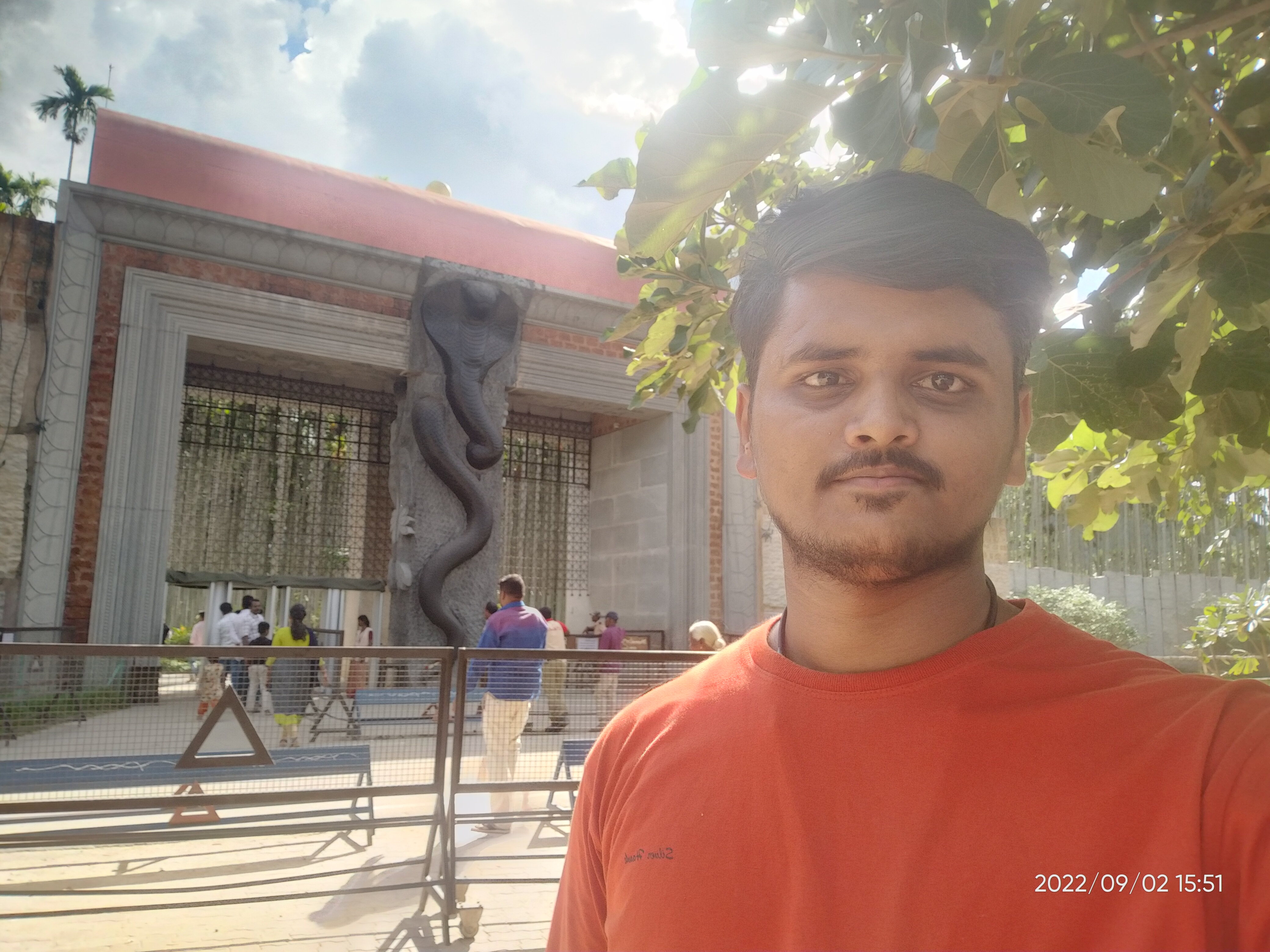
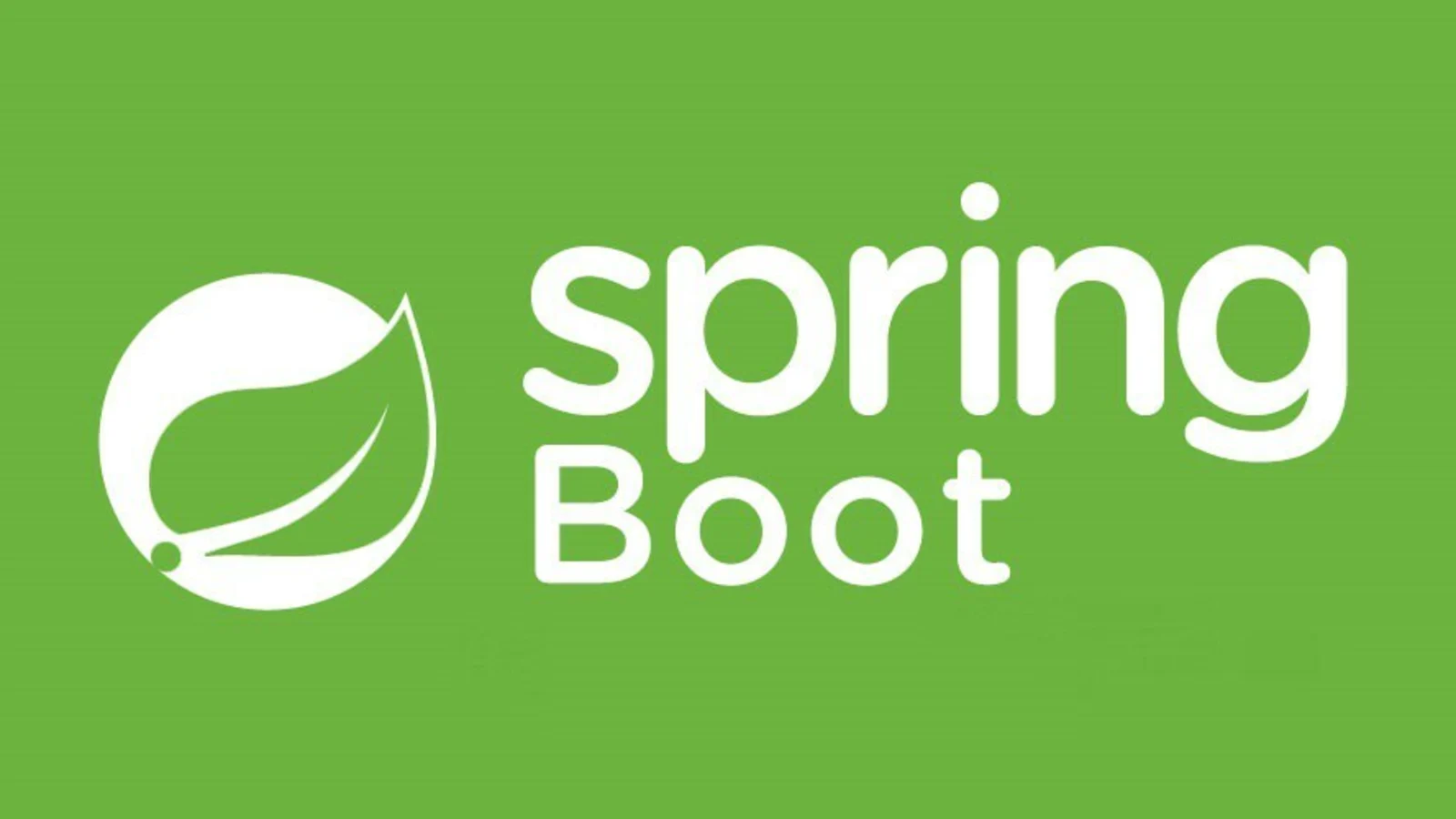
In Spring Boot, the Repository pattern is used to encapsulate the logic required to access data sources. The repository serves as a middle layer between the data source (database) and the business logic, promoting clean separation of concerns and better maintainability of the application.
Use of Repository in Spring Boot
Data Access Layer: It abstracts the data access details and provides a cleaner interface for interacting with the database.
Separation of Concerns: The business logic is separated from the data access logic, making the code more modular and maintainable.
Scalability: Changes in the data source (for example, switching from a relational database to NoSQL) will not impact the business logic as long as the repository interface remains the same.
Centralized Data Access Logic: All the database operations are managed in one place, ensuring consistency and reusability.
Efficiency of Repository
Code Reusability: The repository pattern encourages reuse of the same database logic across different parts of the application, reducing code duplication.
Improved Testing: By isolating the data access logic, it becomes easier to mock or stub out repositories in unit tests, thus improving testability.
Automatic CRUD Operations: Spring Data JPA, for instance, allows developers to use repositories without writing SQL or HQL queries by using method naming conventions, improving productivity.
Maintainability: Since repositories follow an interface-driven design, adding new features or refactoring becomes more manageable.
Performance: Efficient data access strategies like lazy loading, caching, and query optimization are supported, enhancing overall performance.
Types of Repository in Spring Boot
CrudRepository:
Provides generic CRUD (Create, Read, Update, Delete) operations for a particular entity.
Methods include:
save()
,findAll()
,delete()
,findById()
, etc.
PagingAndSortingRepository:
Extends
CrudRepository
and provides additional methods for pagination and sorting.Methods include:
findAll(Pageable pageable)
,findAll(Sort sort)
.
JpaRepository:
Extends
PagingAndSortingRepository
and provides JPA-specific methods likeflush()
,saveAndFlush()
,deleteInBatch()
, etc.More advanced than
CrudRepository
with additional methods for handling complex database operations.
MongoRepository:
- Similar to
JpaRepository
, but for MongoDB. It provides MongoDB-specific operations like query building and indexing.
- Similar to
Custom Repository:
- Developers can create their own custom repository interfaces and implementations, which extend one of the provided Spring repositories or define their own methods.
ReactiveCrudRepository (for reactive applications):
- Used for handling non-blocking, asynchronous data access with reactive programming paradigms in Spring WebFlux.
Each repository type is designed for different needs, from basic CRUD operations to advanced features like pagination, sorting, and reactive programming. The choice of repository depends on the database and the complexity of data access requirements in your Spring Boot application.
In Spring Boot, the most commonly used repository is JpaRepository, which is part of Spring Data JPA. It is widely used because of its comprehensive set of functionalities, ease of use, and flexibility for performing database operations in typical enterprise applications.
Why JpaRepository is Most Used:
Comprehensive Features:
It includes all the methods from
CrudRepository
andPagingAndSortingRepository
, along with additional JPA-specific operations.It supports CRUD operations, pagination, sorting, and more advanced database features like batch operations and flushing.
Auto-Generated Queries:
Spring Data JPA allows developers to define repository methods using method naming conventions (e.g.,
findByName()
,findByEmail()
). Spring automatically generates the necessary SQL or JPQL query.This minimizes boilerplate code, as no need to write actual SQL queries for most common operations.
Integration with JPA/Hibernate:
JPA (Java Persistence API) is a widely adopted specification for object-relational mapping (ORM) in Java.
JpaRepository
works seamlessly with Hibernate (the default JPA implementation in Spring Boot) to map Java objects to relational database tables.It provides advanced features like lazy loading, cascading, and custom query execution.
Custom Query Support:
- Developers can write custom JPQL or native SQL queries using the
@Query
annotation, offering flexibility for complex data retrieval or manipulation.
- Developers can write custom JPQL or native SQL queries using the
Support for Pagination and Sorting:
JpaRepository
offers built-in methods for paging and sorting large datasets, which are critical features in production-grade applications.
Transactional Management:
- The repository methods in Spring Data JPA are transactional by default, which means that all CRUD operations are wrapped in transactions, ensuring data consistency and integrity.
Example of JpaRepository:
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByLastName(String lastName);
@Query("SELECT u FROM User u WHERE u.email = ?1")
User findByEmail(String email);
}
Summary of JpaRepository Features:
CRUD Operations:
save()
,findAll()
,deleteById()
, etc.Pagination and Sorting:
findAll(Pageable pageable)
,findAll(Sort sort)
.Batch Operations:
saveAll()
,deleteInBatch()
.Custom Queries: Using
@Query
or derived query methods.JPA-Specific Methods:
flush()
,saveAndFlush()
,deleteInBatch()
, etc.
In most Spring Boot applications that interact with a relational database, JpaRepository
is the go-to repository due to its rich feature set, simplicity, and tight integration with JPA/Hibernate.
In Spring Boot and Spring Data JPA, annotations play a crucial role in simplifying the development process by reducing boilerplate code, managing the application context, configuring the database, and defining entity mappings. Below is a breakdown of the most commonly used annotations in both frameworks:
Annotations in Spring Boot
@SpringBootApplication:
Marks the main class of a Spring Boot application.
Combines three annotations:
@Configuration
,@EnableAutoConfiguration
, and@ComponentScan
.Example:
@SpringBootApplication public class MyApplication { public static void main(String[] args) { SpringApplication.run(MyApplication.class, args); } }
@RestController:
Marks a class as a REST controller, combining
@Controller
and@ResponseBody
.It allows the class to handle web requests and automatically convert the response to JSON/XML.
Example:
@RestController public class UserController { @GetMapping("/users") public List<User> getUsers() { return userService.getAllUsers(); } }
@RequestMapping (and related annotations like
@GetMapping
,@PostMapping
, etc.):Maps HTTP requests to handler methods in controller classes.
Example:
@GetMapping("/users") public List<User> getUsers() { return userService.getAllUsers(); }
@Autowired:
Enables automatic dependency injection in Spring. Spring will automatically inject the required bean into the annotated field, constructor, or setter method.
Example:
@Service public class UserService { @Autowired private UserRepository userRepository; }
@Service, @Component, @Repository:
These annotations are used to register classes as beans in the Spring container.
@Service
is for service classes,@Component
is a generic stereotype, and@Repository
is used for data access layer beans.Example:
@Service public class UserService { ... } @Repository public interface UserRepository extends JpaRepository<User, Long> { ... }
@Configuration:
Marks a class as a source of bean definitions. It can be used to define beans programmatically rather than relying on component scanning.
Example:
@Configuration public class AppConfig { @Bean public DataSource dataSource() { return new HikariDataSource(); } }
@Value:
Used to inject values from the application properties or YAML configuration file into Spring-managed beans.
Example:
@Value("${app.name}") private String appName;
Annotations in Spring Data JPA
@Entity:
Marks a class as a JPA entity, meaning it maps to a database table.
Example:
@Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; }
@Id:
Marks a field as the primary key of the entity.
Example:
@Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id;
@GeneratedValue:
Specifies how the primary key value should be generated (e.g., auto-increment).
Example:
@Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id;
@Table:
Specifies the table name in the database that the entity will map to (optional, by default the table name is derived from the entity class name).
Example:
@Entity @Table(name = "users") public class User { ... }
@Column:
Defines specific attributes for the mapped database column (like name, length, etc.). If not used, defaults are applied based on field names.
Example:
@Column(name = "user_name", length = 100) private String name;
@OneToMany, @ManyToOne, @OneToOne, @ManyToMany:
Used to define the relationships between entities.
Example of
@ManyToOne
:@ManyToOne @JoinColumn(name = "department_id") private Department department;
@JoinColumn:
Specifies the foreign key column for a relationship between two entities.
Example:
@ManyToOne @JoinColumn(name = "department_id") private Department department;
@Query:
Used to define custom JPQL or native SQL queries in repository interfaces.
Example:
@Query("SELECT u FROM User u WHERE u.email = ?1") User findByEmail(String email);
@Transactional:
Specifies that a method or class should be wrapped in a database transaction. By default, methods in
JpaRepository
are transactional.Example:
@Transactional public void saveUser(User user) { userRepository.save(user); }
@Modifying:
Used with
@Query
to indicate that a query is modifying data (INSERT, UPDATE, DELETE) rather than just selecting.Example:
@Modifying @Query("UPDATE User u SET u.status = ?1 WHERE u.id = ?2") void updateUserStatus(String status, Long id);
Summary of Key Annotations:
Spring Boot:
@SpringBootApplication
,@RestController
,@RequestMapping
,@Autowired
,@Service
,@Component
,@Repository
,@Configuration
.Spring Data JPA:
@Entity
,@Id
,@GeneratedValue
,@Table
,@Column
,@OneToMany
,@ManyToOne
,@Query
,@Transactional
,@Modifying
.
These annotations simplify the development process by providing declarative configurations and managing a variety of tasks such as dependency injection, transaction management, and data access in Spring Boot and Spring Data JPA applications.
Subscribe to my newsletter
Read articles from OBULIPURUSOTHAMAN K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
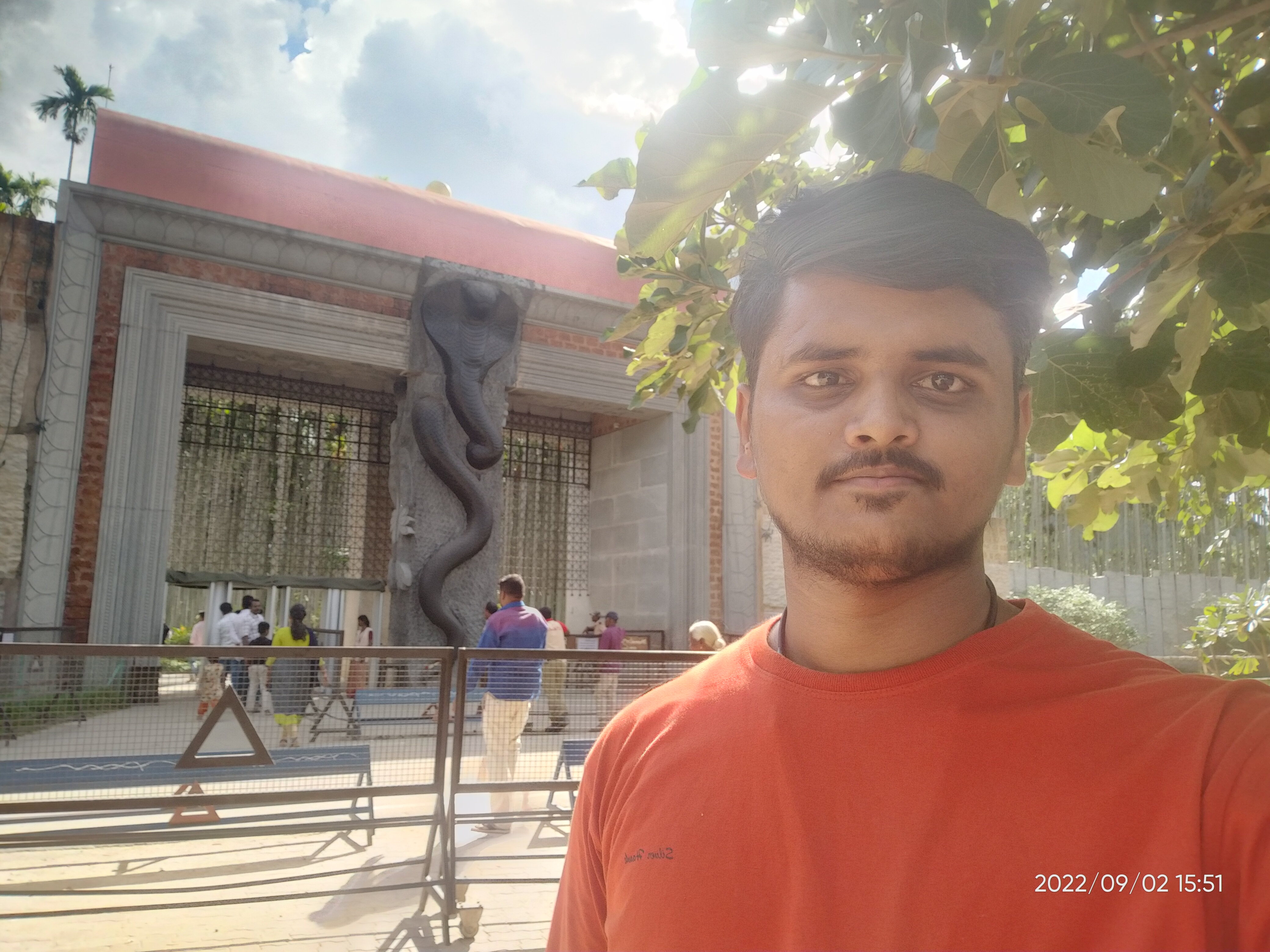
OBULIPURUSOTHAMAN K
OBULIPURUSOTHAMAN K
As a Computer Science and Engineering graduate, I have cultivated a deep understanding of software development principles and technologies. With a strong foundation in Java programming, coupled with expertise in frontend and backend development, I thrive in crafting robust and scalable solutions. Currently, I am leveraging my skills as a Java Full Stack Engineer at Cognizant, where I am involved in designing and implementing end-to-end solutions that meet the complex requirements of our clients. I am passionate about leveraging emerging technologies to drive innovation and deliver tangible business value. My goal is to continually enhance my expertise and contribute to the advancement of software engineering practices in the industry.