ACTIVITY 11: Research Angular Directives | Aligan, Rhed N.
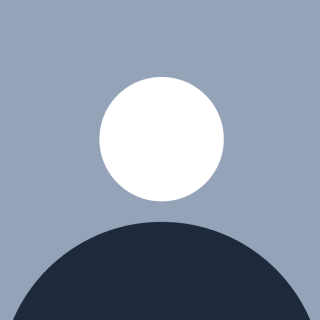
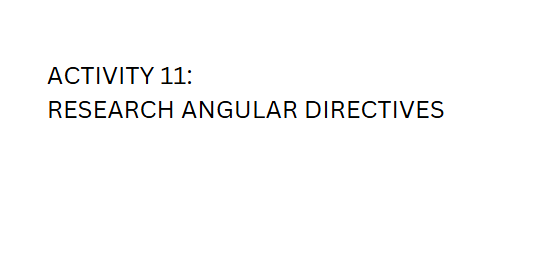
Instructions:
Research the Definition of Angular Directives:
Understand what Angular directives are.
Learn about the three types of directives: Component Directives, Structural Directives (e.g., ngIf, ngFor), and Attribute Directives (e.g., ngClass, ngStyle).
In my own understanding of Angular Directives, Angular directives are the marker or instruction for html to extend the elements that passed to our angular how it been react or control the elements of html. The purpose of these directives makes more dynamic and interactive our project or application. there are various directives and its attributes we can be used and some of the following are;
Component Directives, this directive are commonly used to interact with angular. Components provide a clear structure of every element to be eased to use and become maintainable and advance option for scalable for the future upgrade for elements of the application will be used.
Structure Directives, this directive, are use for adding and remove of DOM (Document Object Model). DOM is use to manipulate or modify the nodes inside webpage to make interactive or add features of elements.
Some behavior to example is:
*ngFor
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'angular-project'; friendslist = [ { name: 'Nishant', age: 25 }, { name: 'Shailesh', age: 45 }, { name: 'Abhishek', age: 36 }, { name: 'Akshay', age: 65 }, { name: 'Ashish', age: 12 }, { name: 'Uday', age: 31 }, { name: 'Mayank', age: 45 }, { name: 'Raju', age: 74 }, ] }
In your app component typescript file, example above the code snippet. It displays the basic properties such as selector, templateurl, and styleUrls. Also, it displays list of arrays named friendlist that include the name and age.
In html, create an unorderedlist with a *ngFor and attributes
<ul>
<li *ngFor="let item of friendslist">
{{ item.name }} {{ item.age }}
</li>
</ul>
In above code snippet, it shows where the array can access and display which is in unorderlist tags specifically in list item tags. the {{item. name}} and {{item.age}} use to access inside of array in our typescript. It called interpolation. Interpolation is a strategy to that allow user to bind a value to the webpage or UI elements. Using *ngFor use to show of how many lists inside of array so in the code snippet example, it has an 8 name and 8 age also.
So, the output of using *ngFor is:
Next is *ngIf. ngIf use to show some data or item based on condition provide in the code. Same component which has array include of names and age. It’s like a if else statement if the item shows or not based what trigger behavior attributes.
<ul *ngIf="isVisible">
<li *ngFor="let item of friendslist">
{{ item.name }} is {{ item.age }} years old
</li>
</ul>
<button (click)="hideList()">
Click to Hide List
</button>
In above code snippet, we added a button with a (click) so we can access what function in typescript with a value of hidelist() wherein button tags become foundation to use the *ngIf
In our typescript, add
isVisible: boolean = true;
Additionally, create a function so the button tags in html works.
hideList(){
this.isVisible = false;
}
So, in running the application, if the value of button is true it will trigger the hidelist attributes which is false and not shown the list of name and age. It will vice versa.
Example if the button is true:
If the button is false:
Attribute Directives, this directive use to modify the appearance or behavior of an element and components of application. To use this attribute below are example of code snippet.
In our app.component.html, create a h1 tags and paragpragh tags.
Additionally in our typescript,
in our app.module.ts
OUTPUT:
So, the myHighlight, this attribute are included in the import of HighlightDirective at @NgModule function. In the attributes inside of <p> tags diplays the yellow background in the paragraph.
Using ngClass allow you to add or remove CSS classes that will be use to functionality. You can add an object but if you have a lot of data, best practices use an array which include the attributes and properties.
Example:
ng-class="{'deleted': isDeleted}"
which means when the isDeleted in the typescript trigger and become true, the class will be deleted.
you can add another with the same class such as
ng-class="{'deleted': isDeleted, 'highlight': isHighlighted}"
So much better especially if you have lot of data, it less space consume and organized.
Using ngStyle allow to set CSS dynamically based on the value data. Its reusable and avoid redundant code for implementing design.
Example of ngStyle:
- In our html
It will be result of:
but the cases of development, in my own understanding based on research, the ngStyle method is become useful especially of adding of features and CSS dynamically in our application or system since we always called the component method.
Use Cases of Angular Directives:
Research practical use cases for each type of directive.
Find examples of how directives enhance the behavior or structure of DOM elements in Angular applications.
Components Directive - are the cornerstone of Angular applications. It’s all about defining structure and behavior of User Interface elements. styling into a reusable unit.
ngFor practical use cases:
items = ['Item 1', 'Item 2', 'Item 3'];
Code snippet above are array that include in our app typescript
<ul> <li *ngFor="let item of items">{{ item }}</li> </ul>
This code above are for app component.html so, in the use cases using *ngFor, all item in array will display because we have a {{item}} to access the data in array.
Structure Directives - can be able to modify the structure of Document Object Model by adding and removing and manipulating the elements inside of application.
ngIf Example:
<div *ngIf="isLoggedIn"> Welcome, {{ userName }}! </div>
In the code snippet above displays the boolean operation in operation of ngIf when the isLoggedIn is become true, the text will be display or not, depends on how logic of the code.
Attributes Directives is a type of directives that can be able to change the appearance or behavior of an components to make dynamically and interactive applications.
ngClass use case example:
OUTPUT
in ngClass array, text-success is green color display when the isSuccess become true, while text-danger is a red color when the isSuccess is become false.
ngStyle use practical use cases example:
In code snippet above and its output shows that when the isSuccess is become true the font become 24px and the color background becomes yellow, while if the isSuccess value is becomes false, the output is fonts 20px and green background color.
Search for Code Snippets:
- Search online for relevant Angular code snippets that demonstrate the use of directives (e.g., conditional rendering, looping, dynamic class binding).
Conditional Rendering using ngIf
In the code snippet above shows of *ngIf, that when the value of isLoggedin is become true or its default, the Welcome and the data that holds of name “userName” will display. Otherwise, no display action.
Looping using ngFor
In the looping code snippets above, shows that using ngFor, by accessing the data from item.name will show all since we use as asterisk to all data been access like in SQL. So, if that is array it will show all data from that
Dynamic Class Binding using ngClass
In code snippets above shows that if the isActive becomes true, the active class is added to the <div> in our html, while if the isActive is false (! means opposite of result or negative) the active will not applied for html.
Document Your Research on Hashnode:
Write a blog post on Hashnode explaining Angular directives, their types, use cases, and examples.
Include code snippets and explain how they work.
Add references to the sources you used during your research.
Angular ngFor and ngIf Directives Explained for Beginners (freecodecamp.org)
css - What is the difference between ng-class and ng-style? - Stack Overflow
Angular ngClass and ngStyle: The Complete Guide (angular-university.io)
The Power of Angular Directives: A Comprehensive Guide - DEV Community
Add References:
Make sure to credit the websites or blogs where you found useful information and code snippets.
Include links to official Angular documentation or tutorials for further reading.
Subscribe to my newsletter
Read articles from Rhed Aligan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rhed Aligan
Rhed Aligan
I'm very enthusiast in things that gives me new material to learn.