Adding Crypto Checkout to Your Website Using 100Pay

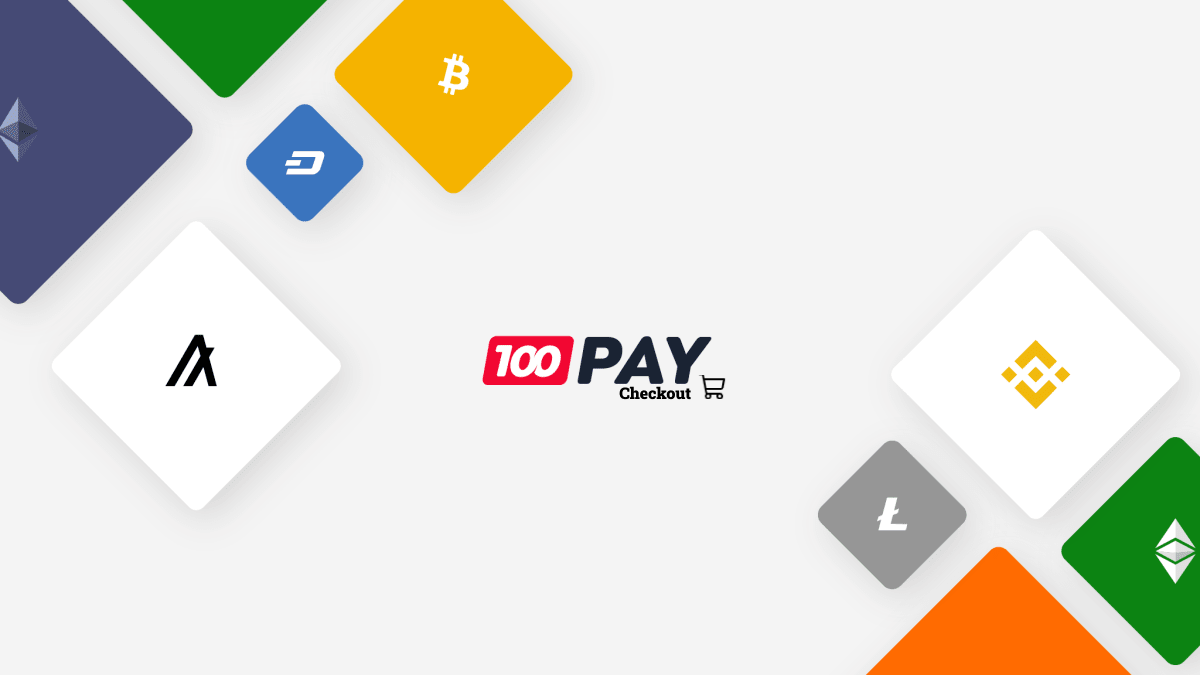
100Pay is a crypto payment gateway that allows businesses to accept cryptocurrency payments seamlessly. It's designed to simplify digital transactions for developers, businesses, and individuals by offering tools like billing, checkout, payment links, and invoicing.
What is 100Pay checkout?
100Pay Checkout is a feature of the 100Pay platform that enables businesses and developers to integrate cryptocurrency payments into their websites or applications seamlessly. It functions similarly to other payment gateways, allowing users to pay with various cryptocurrencies during checkout.
Benefits of Integrating 100Pay Checkout
Reaching a Broader Audience: By accepting multiple cryptocurrencies, businesses can tap into a global market of crypto users. This expansion can lead to increased sales, especially from customers who prefer using digital currencies for their transactions.
Simplifying International Payments: Cryptocurrencies transcend geographical boundaries, making international payments more straightforward. With 100Pay Checkout, businesses can avoid the complexities and fees associated with currency conversions and cross-border transactions, offering a smoother payment experience for international customers.
Reducing Transaction Fees: Traditional payment gateways often come with high transaction fee, 100pay Checkout offers lower fee compared to traditional methods allowing businesses to save on transactions in turn passing savings on to their customers.
Features of 100pay Checkout include.
Accept Cryptocurrency Payments: Seamlessly accept various cryptocurrencies directly on your website.
Flexible Withdrawals: Easily withdraw funds to your crypto wallet or fiat balance.
Generate Payment Invoices: Create detailed invoices for transactions.
Payment Links: Generate secure payment links for quick and easy transactions.
Custom Coin Creation: Develop and launch your own cryptocurrency on any supported network.
ICO/IDO Launch: Organize Initial Coin Offerings (ICOs) or Initial DEX Offerings (IDOs) to raise funds for your project.
Advanced Analytics: Utilize comprehensive analytics tools to monitor and analyze your business performance.
Crypto Swaps: Exchange one cryptocurrency for another within the platform.
Buy/Sell Cryptocurrency: Facilitate the buying and selling of various cryptocurrencies.
Installing the SDK
To integrate 100Pay Checkout into your website or application, you first need to install the 100Pay SDK in your development environment. Here's how to do it:
npm i @100pay-hq/checkout
Once installed, you can require or import the SDK in your application files where you'll handle payment processing.
import { shop100Pay } from "@100pay-hq/checkout";
Configuring API Keys
After installing the SDK, you need to authenticate your application with 100Pay using API keys. Here's how to generate and configure these keys:
Generate API Keys:
To generate your API key log in to your 100Pay account and navigate to the Developer section in the dashboard. Under API Keys, click on Generate New Key. You will receive a public and a private API key. Store these keys securely.
Configure API Keys in Your Application:
Create a .env.local file in the root of your project and add your keys:
NEXT_PUBLIC_100PAY_API_KEY='your-api_key'
Setting Up the Checkout Form
Next, you need to set up the 100Pay Checkout form on your website. This form will allow users to enter their payment details and complete transactions.
Checkout Form
Add the checkout form to your website. You can customize the form to match your website’s design by adjusting the CSS (Tailwind).
<form onSubmit={payWith100pay} className="max-w-sm mx-auto">
<div>
<label>First Name:</label>
<input
type="text"
name="firstName"
value={formData.firstName}
onChange={handleChange}
required
/>
</div>
<div>
<label>Last Name:</label>
<input
type="text"
name="lastName"
value={formData.lastName}
onChange={handleChange}
required
/>
</div>
<div>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
required
/>
</div>
<div>
<label>Phone:</label>
<input
type="tel"
name="phone"
value={formData.phone}
onChange={handleChange}
required
/>
</div>
<div>
<label>Amount (NGN):</label>
<input
type="number"
name="amount"
value={formData.amount}
onChange={handleChange}
required
/>
</div>
<button type="submit">Pay with 100Pay</button>
</form>;
Outcome
Here is the complete code
"use client"
import { useState } from 'react';
import Script from 'next/script';
import { shop100Pay } from '@100pay-hq/checkout';
const PaymentForm = () => {
const [formData, setFormData] = useState({
firstName: '',
lastName: '',
email: '',
phone: '',
amount: ''
});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData({
...formData,
[name]: value
});
};
const payWith100pay = (e) => {
e.preventDefault();
if (typeof shop100Pay === 'undefined') {
alert("100Pay SDK not loaded. Please ensure the SDK script is included.");
return;
}
const { firstName, lastName, email, phone, amount } = formData;
shop100Pay.setup({
ref_id: "" + Math.floor(Math.random() * 1000000000 + 1),
api_key: "process.env.NEXT_PUBLIC_100PAY_PUBLIC_KEY", // Replace with your actual API key
customer: {
user_id: "1", // optional
name: ${firstName} ${lastName},
phone,
email
},
billing: {
amount,
currency: "NGN", // Naira currency code
description: "Test Payment",
country: "NG", // Nigeria country code
vat: 10, // optional
pricing_type: "fixed_price" // or partial
},
metadata: {
is_approved: "yes",
order_id: "OR2", // optional
charge_ref: "REF" // optional, you can add more fields
},
call_back_url: "http://localhost:3000/verifyorder/", // Change to your actual callback URL
onClose: (msg) => {
alert("You just closed the crypto payment modal.");
},
onPayment: (reference) => {
alert(`New Payment detected with reference ${reference}`);
// Make sure to verify the payment on your backend before proceeding.
},
onError: (error) => {
console.log(error);
alert("Sorry, something went wrong. Please try again.");
}
}, {
maxWidth: 1200
});
};
return (
<>
<Script src="https://100pay-sdk-url.js" strategy="beforeInteractive" />{" "}
<div className="mb-5">
<form onSubmit={payWith100pay} className="max-w-sm mx-auto">
<div>
<label className="block mb-2 text-sm font-medium text-gray-900 dark:text-white">
First Name:
</label>
<input
className="block w-full p-4 mb-5 text-gray-900 border border-gray-300 rounded-lg bg-gray-50 text-base focus:ring-blue-500 focus:border-blue-500"
type="text"
name="firstName"
value={formData.firstName}
onChange={handleChange}
required
/>
</div>
<div>
<label>Last Name:</label>
<input
className="block w-full p-4 mb-5 text-gray-900 border border-gray-300 rounded-lg bg-gray-50 text-base focus:ring-blue-500 focus:border-blue-500"
type="text"
name="lastName"
value={formData.lastName}
onChange={handleChange}
required
/>
</div>
<div>
<label>Email:</label>
<input
className="block w-full p-4 mb-5 text-gray-900 border border-gray-300 rounded-lg bg-gray-50 text-base focus:ring-blue-500 focus:border-blue-500"
type="email"
name="email"
value={formData.email}
onChange={handleChange}
required
/>
</div>
<div>
<label>Phone:</label>
<input
className="block w-full p-4 mb-5 text-gray-900 border border-gray-300 rounded-lg bg-gray-50 text-base focus:ring-blue-500 focus:border-blue-500"
type="tel"
name="phone"
value={formData.phone}
onChange={handleChange}
required
/>
</div>
<div>
<label>Amount (NGN):</label>
<input
className="bg-gray-50 border border-gray-300 mb-5 text-gray-900 text-sm rounded-lg focus:ring-blue-500 focus:border-blue-500 block w-full p-2.5"
type="number"
name="amount"
value={formData.amount}
onChange={handleChange}
required
/>
</div>
<button
className="focus:outline-none text-white bg-red-700 hover:bg-red-800 focus:ring-4 focus:ring-red-300 font-medium rounded-lg text-sm px-5 py-2.5 me-2 mb-2"
type="submit"
>
Pay with 100Pay
</button>
</form>
{/* Wrapper for the 100Pay checkout modal */}
<div id="show100Pay"></div>
{/* Load the 100Pay script */}
<Script src="https://js.100pay.co/" strategy="afterInteractive" />
</div>
</>;
);
};
export default PaymentForm;
Final Output
Conclusion
Integrating 100Pay Checkout into your Next.js application provides solutions for accepting cryptocurrency payments, automating invoicing, and managing multiple cryptocurrencies. The integration process involves straightforward steps, from installing the SDK and configuring API keys to setting up the checkout form and handling transactions. By utilizing these features you can enhance the flexibility and efficiency of your payment systems, catering to a broader audience and streamlining your business operations.
Subscribe to my newsletter
Read articles from Nweke Manuchimso Emmanuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
