Mastering the Basics: A Guide to Functions, Methods, Classes, and Objects in Programming

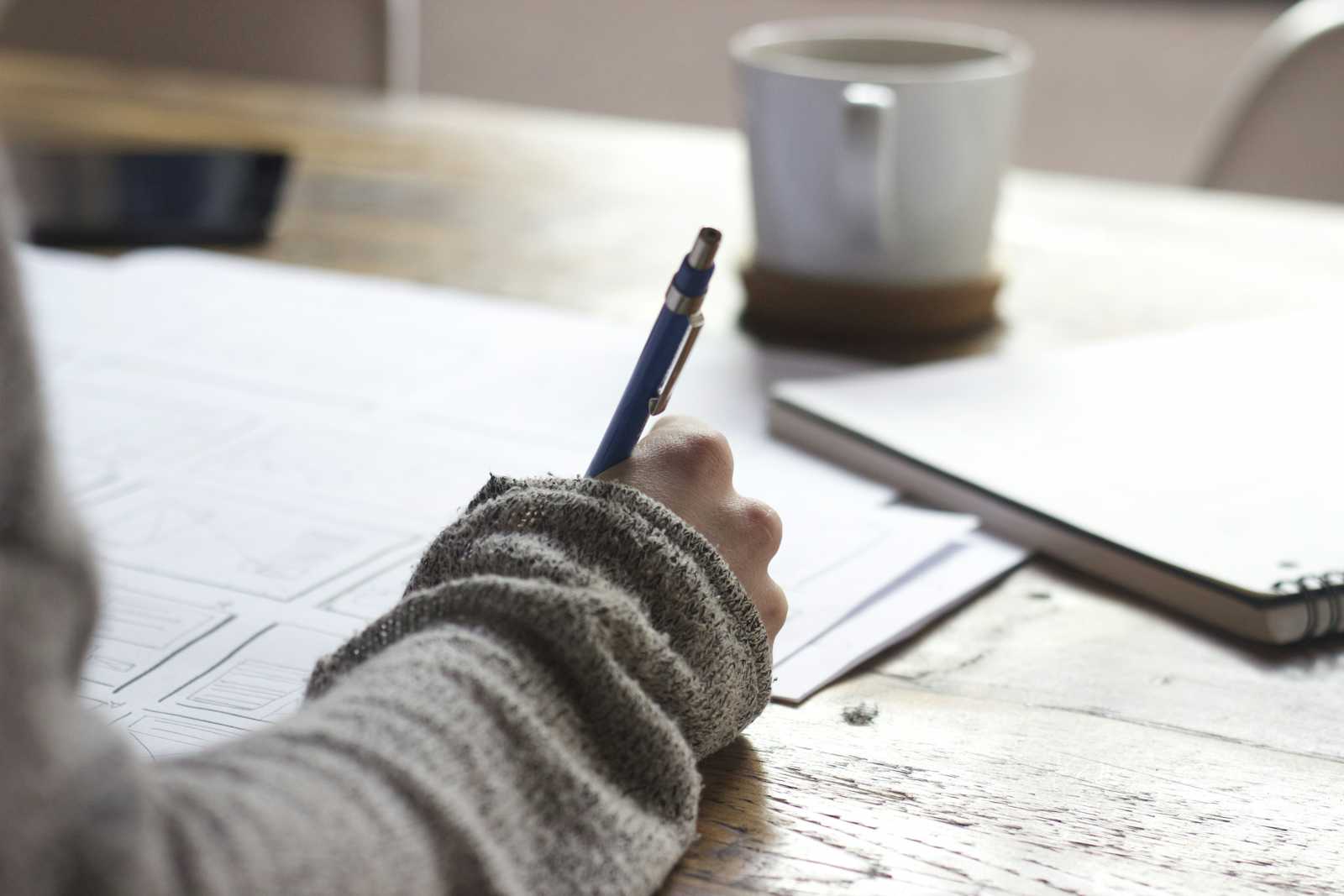
As a newcomer to programming, I initially found concepts like functions, methods, classes, and objects challenging. Through careful study and practice, I've gained a clearer understanding. Let me share my insights using a calculator example.
Functions: Fundamental Units of Code
Functions are reusable blocks of code that perform specific tasks. Consider this basic function:
function add(a: number, b: number): number {
return a + b;
}
console.log(add(5, 3)); // Output: 8
This add
function takes two numbers and returns their sum. It can be called from anywhere in the program.
The Evolution to Methods
Methods are similar to functions but are associated with a specific context. Let's examine this in a calculator class:
class Calculator {
add(a: number, b: number): number {
return a + b;
}
}
The add
function within the Calculator
class is a method. It performs the same operation as our previous function but is now part of the Calculator
class structure.
Distinguishing Functions and Methods
The key distinction lies in their scope and usage:
Functions are independent and globally accessible.
Methods are bound to specific class instances and accessed through those instances.
Classes: Blueprints for Objects
Classes serve as templates for creating objects. The Calculator
class defines the structure and behavior of calculator objects:
class Calculator {
add(a: number, b: number): number {
return a + b;
}
subtract(a: number, b: number): number {
return a - b;
}
}
This class outlines that calculator objects will have addition and subtraction capabilities.
Objects: Instances of Classes
To utilize a class, we create objects, which are instances of that class:
const myCalculator = new Calculator();
This myCalculator
object is a concrete instance of the Calculator
class, ready to perform calculations.
Utilizing Methods through Objects
With our calculator object created, we can invoke its methods:
console.log(myCalculator.add(10, 5)); // Output: 15
console.log(myCalculator.subtract(10, 5)); // Output: 5
We access the add
and subtract
methods through the myCalculator
object, demonstrating how methods are called on specific instances.
The Significance of These Concepts
Understanding these fundamentals is crucial because they:
Facilitate code organization and reusability
Enable the creation of multiple instances with shared behavior
Form the foundation for more advanced programming paradigms
Conclusion
Grasping these concepts - functions, methods, classes, and objects - is essential for progressing in programming. Regular practice and application of these principles will solidify your understanding and improve your coding skills.
If you found this explanation beneficial, please share it with fellow programming learners. Collective learning enhances our growth as developers.
Subscribe to my newsletter
Read articles from Abhishek Gowda H C directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhishek Gowda H C
Abhishek Gowda H C
I'm a skilled full-stack developer specializing in frontend technologies like React, Next.js, and Angular. With expertise in Node.js and MongoDB, I create seamless, JavaScript-based full-stack solutions. Currently working as a frontend developer at VGSL, Bangalore, I've tackled a range of complex to advanced projects, continuously pushing the boundaries of web development. My passion for problem-solving and UI/UX design drives me to create user-friendly, visually appealing websites. I'm committed to continuous learning and enjoy mentoring aspiring developers, guiding them towards successful tech careers. I'm also exploring innovative solutions in the tech industry.