Day 8 of 40DaysofKubernetes : Understanding Kubernetes Deployment, Replication Controller, and ReplicaSet

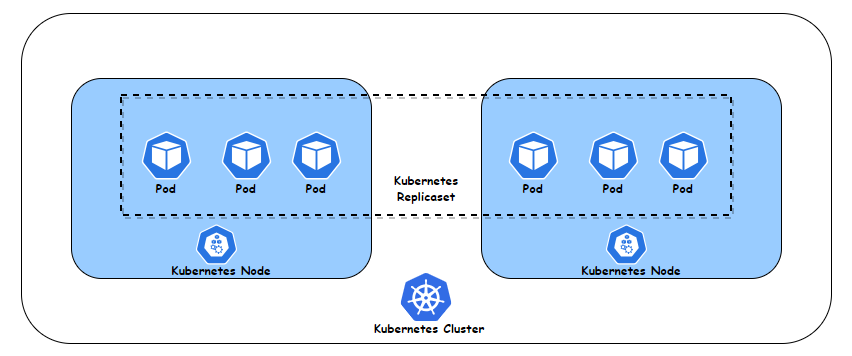
In the world of Kubernetes, managing containerized applications can seem overwhelming at first. However, Kubernetes provides powerful resources like Deployments, Replication Controllers, and ReplicaSets to simplify the process. These resources help maintain the desired state of applications, ensuring scalability, reliability, and fault tolerance.
In this blog, we’ll walk through the differences, functionalities, and purposes of Deployments, Replication Controllers, and ReplicaSets in Kubernetes.
What is Kubernetes Deployment?
Deployment is a Kubernetes resource that manages application deployments across a cluster. It provides declarative updates to applications, ensuring that they are running in the desired state at all times. You can scale, update, or roll back your applications seamlessly with Deployments.
Key Features of Deployments:
Declarative Updates: You define the desired state in the YAML configuration file, and Kubernetes ensures the application matches that state.
Rolling Updates: When you update your application (e.g., a new version of the container image), Kubernetes can perform a rolling update without downtime, gradually replacing old Pods with new ones.
Rollback: If an update fails or introduces a bug, you can roll back to a previous version of the Deployment.
Scaling: You can easily scale up or down the number of Pods running your application to handle varying loads.
Example YAML Configuration for a Deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-deployment
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app-container
image: nginx:1.19.3
ports:
- containerPort: 80
2. What is a Replication Controller?
A Replication Controller (RC) is an older Kubernetes resource responsible for ensuring a specified number of Pod replicas are running at any given time. If the number of replicas is less than desired (due to crashes or node failures), the Replication Controller will create new Pods. If there are more Pods than desired, it will terminate extra ones.
Key Features of Replication Controllers:
Ensure Pod Replicas: Replication Controllers guarantee that a specified number of Pods are always running.
Auto-healing: If a Pod crashes or fails, the Replication Controller will spin up new ones.
Manual Scaling: You can manually increase or decrease the number of replicas by updating the RC definition.
Example YAML Configuration for a Replication Controller:
apiVersion: v1
kind: ReplicationController
metadata:
name: my-app-rc
spec:
replicas: 3
selector:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app-container
image: nginx:1.19.3
ports:
- containerPort: 80
Replication Controllers are now largely replaced by ReplicaSets but are still functional in Kubernetes for backward compatibility.
What is a ReplicaSet?
A ReplicaSet is the modern replacement for Replication Controllers. It performs the same core function of ensuring that the correct number of Pod replicas are running, but with additional flexibility. A ReplicaSet’s primary advantage over a Replication Controller is its support for label selectors, which allows for more complex matching criteria when selecting Pods to manage.
Key Features of ReplicaSets:
Label Selectors: Allows matching Pods based on more complex criteria.
Scaling: Like Deployments, you can scale ReplicaSets up or down by adjusting the replica count.
Pod Management: ReplicaSets ensure that the specified number of Pod replicas are running and healthy.
Example YAML Configuration for a ReplicaSet:
apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: my-app-rs
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app-container
image: nginx:1.19.3
ports:
- containerPort: 80
While ReplicaSets can be used directly, they are typically used within Deployments, which provide higher-level management (like rolling updates and rollback).
TASK
ReplicaSet
1. Create a new ReplicaSet based on the Nginx image with 3 replicas
- Create the following YAML file for the ReplicaSet:
apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: nginx-replicaset
spec:
replicas: 3
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx
ports:
- containerPort: 80
- Apply the YAML file to create the ReplicaSet:
kubectl apply -f nginx-replicaset.yaml
2. Update the replicas to 4 from the YAML
- Edit the YAML file and change the
replicas
field:
replicas: 4
- Apply the updated YAML file:
kubectl apply -f nginx-replicaset.yaml
3. Update the replicas to 6 from the command line
To scale the ReplicaSet using a command:
kubectl scale rs nginx-replicaset --replicas=6
Deployment
1. Create a Deployment named nginx with 3 replicas
Create the following YAML file for the Deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx
labels:
tier: backend
spec:
replicas: 3
selector:
matchLabels:
app: v1
template:
metadata:
labels:
app: v1
spec:
containers:
- name: nginx
image: nginx:1.23.0
ports:
- containerPort: 80
Apply the YAML file:
kubectl apply -f nginx-deployment.yaml
2. List the Deployment and ensure the correct number of replicas is running
Use the following command to list the Deployment and check the replicas:
kubectl get deployment nginx
3. Update the image to nginx:1.23.4
- You can update the image via a command:
kubectl set image deployment/nginx nginx=nginx:1.23.4
- Check the status to ensure the rollout is complete:
kubectl rollout status deployment/nginx
4. Assign the change cause "Pick up patch version" to the revision
- Annotate the deployment with the change cause:
kubectl annotate deployment/nginx kubernetes.io/change-cause="Pick up patch version"
5. Scale the Deployment to 5 replicas
Scale the Deployment using the following command:
kubectl scale deployment nginx --replicas=5
6. View the Deployment rollout history
To check the rollout history:
kubectl rollout history deployment/nginx
7. Revert the Deployment to revision 1
- Revert the Deployment to revision 1:
kubectl rollout undo deployment/nginx --to-revision=1
- Check the pods to ensure they are using the correct image (
nginx:1.23.0
):
kubectl describe deployment nginx | grep Image
Troubleshooting the YAML Issue
1. Apply the first YAML
Here’s the provided incorrect YAML:
apiVersion: v1
kind: Deployment
metadata:
name: nginx-deploy
labels:
env: demo
spec:
template:
metadata:
labels:
env: demo
name: nginx
spec:
containers:
- image: nginx
name: nginx
ports:
- containerPort: 80
replicas: 3
selector:
matchLabels:
env: demo
Issue: Incorrect apiVersion
This Deployment uses apiVersion: v1
, but it should be apps/v1
for Deployments.
Fix the YAML:
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deploy
labels:
env: demo
spec:
replicas: 3
selector:
matchLabels:
env: demo
template:
metadata:
labels:
env: demo
spec:
containers:
- name: nginx
image: nginx
ports:
- containerPort: 80
2. Apply the second YAML
Here’s the second provided incorrect YAML:
apiVersion: v1
kind: Deployment
metadata:
name: nginx-deploy
labels:
env: demo
spec:
template:
metadata:
labels:
env: demo
name: nginx
spec:
containers:
- image: nginx
name: nginx
ports:
- containerPort: 80
replicas: 3
selector:
matchLabels:
env: dev
Issue: Mismatched Labels
The matchLabels
selector is looking for env: dev
, but the Pod template has env: demo
. The labels in both the selector and the template should match.
Fix the YAML:
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deploy
labels:
env: demo
spec:
replicas: 3
selector:
matchLabels:
env: demo
template:
metadata:
labels:
env: demo
spec:
containers:
- name: nginx
image: nginx
ports:
- containerPort: 80
Once corrected, apply the YAML files using kubectl apply -f
and verify the results using kubectl get pods
or kubectl describe deployment
.
Reference
Video:
https://www.youtube.com/watch?v=oe2zjRb51F0&list=PLl4APkPHzsUUOkOv3i62UidrLmSB8DcGC&index=10
Documentation:
https://kubernetes.io/docs/reference/kubectl/quick-reference/
https://kubernetes.io/docs/concepts/workloads/controllers/replicaset/
https://kubernetes.io/docs/concepts/workloads/controllers/deployment/
https://kubernetes.io/docs/concepts/workloads/controllers/replicationcontroller/
Subscribe to my newsletter
Read articles from Rahul Vadakkiniyil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
