Using assert to fix PHPStan errors

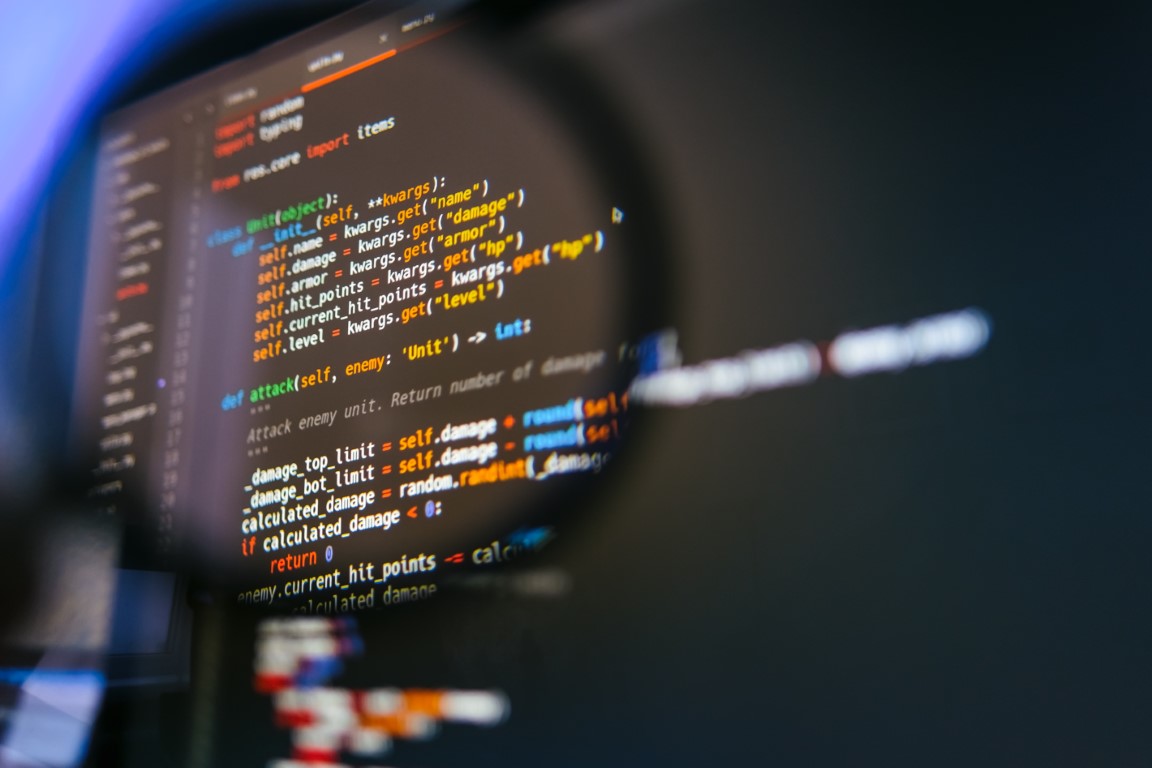
I’ve been using more and more PHPStan recently and it’s been a real pleasure to improve my codebases.
Being strict is nice, but sometimes it can also be inconvenient. A lot of built-in methods in php can return false. Or sometimes, you have a “mixed” result even though you know for sure it’s type.
Let’s say you have something like this:
<?php
function stringOrArray():string|array {
$v = someMethodThatReturnMixed();
return $v;
}
Of course, you can do this:
function stringOrArray():string|array {
$v = someMethodThatReturnMixed();
if(is_string($v) || is_array($v)) {
return $v;
}
throw new Exception("Invalid type");
}
But this means adding an if. Throwing an Exception. Not a big deal by itself, but if you have to fix hundreds of lines like this, it’s not great.
A quick(er) fix, is this:
function stringOrArray():string|array {
$v = someMethodThatReturnMixed();
assert(is_string($v)||is_array($v));
return $v;
}
Aaaaand… done! I really like using assert : it adds type checks during development, and is ignored at no performance cost in production. And if somehow, as a developer, you made a mistake, it’s very likely you are going to catch the AssertionException that gets thrown.
That’s all for today, I hope you liked my little tip :)
Subscribe to my newsletter
Read articles from Thomas Portelange directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Thomas Portelange
Thomas Portelange
A Web Developer based in Belgium, specialized in PHP & JS development