First Java Program in Full Stack Java Development
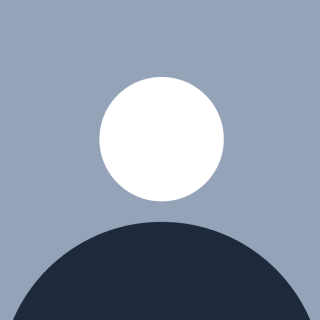
Table of contents
- Chapter 5: First Java Program in Full Stack Java Development
- 1. JShell: An Introduction
- Running Code in JShell
- JShell Commands
- 2. The Role of an Editor and an IDE
- Java: Verbose but Powerful
- Summary
- 3. Writing Your First Java Program
- Starting Point of a Java Program: The main() Method
- 4. Compiling and Interpreting Java Code
- Flowchart: Compiling and Running Java Code
- Key Steps in Compiling and Running Java Code
- Key Notes on Compiling and Running Java Code
- Commands for Compiling and Running Java Programs
- Important Rules for Compiling and Running Java Code
- Packaging Techniques in Java
- The Role of the Operating System (OS)
- Conclusion
- 5. Java Virtual Machine (JVM)
- Key Responsibilities of the JVM
- How the JVM Works: Step-by-Step Execution
- Example:
- Structure of the JVM
- JVM's Handling of the Main Method
- Types of JVM Implementations
- Conclusion
- 6. Java Methods
- 7. The Main Method and JVM
- Importance of the public static void main(String[] args) Signature
- Example of the Main Method
- Explanation of the JVM's Role in Program Execution
- Example: JVM Unable to Find Main Method
- Why is the public static Part Essential?
- Conclusion
- 8. Command-Line Arguments in Java
- 9. File Naming Conventions and Java Classes
- Conclusion
- 10. Packages and .jar Files
- 11. Static vs. Dynamic Typing
- 12. Phases of Java Code Execution
- Difference Between .class File and .war File
- The Role of JVM, JRE, and Compilation
- Conclusion
- 13. Understanding JDK, JRE, and JVM
- 14. Common Java Errors
- 15. Conclusion
- Further Reading and Resources
Chapter 5: First Java Program in Full Stack Java Development
Introduction
In this chapter, we’ll delve into writing and understanding your first Java program. Java, known for its simplicity and verbosity, is an excellent language to build robust applications. We will cover core concepts like JShell, methods, compiling and running Java code, JVM (Java Virtual Machine), command line arguments, and more. By the end of this chapter, you will be able to write, compile, and run basic Java programs, understand the working of JVM, and become familiar with concepts like static, public, and methods in Java.
1. JShell: An Introduction
JShell, introduced in Java 9, is a REPL (Read-Eval-Print Loop) tool that allows you to run Java code interactively. Instead of writing a complete program, you can test small snippets of code directly, making it an ideal tool for beginners who want to quickly understand how Java works. JShell is not intended for building full-scale applications but is perfect for quick tests, debugging, and learning Java basics.
Running Code in JShell
You can execute Java statements line by line in JShell. Each result is automatically stored in a temporary variable like $1
, $2
, etc., which can be reused later.
Example:
JShell> System.out.println(6 + 3);
$1 ==> 9
JShell> 5 + 72
$2 ==> 77
Here, 6 + 3
outputs 9
, and the result is stored in $1
. Similarly, 5 + 72
gives 77
, stored in $2
.
JShell provides an interactive and efficient way to experiment with code without writing full Java classes or methods.
JShell Commands
JShell comes with several useful commands for managing your session and the environment. Below are some important ones:
Command | Description |
/vars | Displays current variables and their values. |
/save <filename> | Saves the current code snippets to a file. |
/reset | Resets the environment, clearing all variables. |
/list | Lists all code snippets entered in the current session. |
Example of JShell Commands:
jshell> /vars
$1 ==> 9
$2 ==> 77
jshell> /save code.java
(Saves the code snippets to the file 'code.java')
jshell> /reset
(Resets the environment, removing variables $1, $2, etc.)
jshell> /vars
(No variables available after reset)
2. The Role of an Editor and an IDE
Before diving deeper into Java programming, it’s crucial to understand the difference between a Text Editor and an IDE (Integrated Development Environment).
1. Editor:
An editor is a basic tool where you can type code, but it lacks additional features like compiling or debugging. Common examples include:
Notepad
Sublime Text
Visual Studio Code (with Java extensions)
2. IDE (Integrated Development Environment):
An IDE provides a comprehensive environment where you can:
Write code
Compile and run it
Debug errors
Test applications
Popular Java IDEs include:
Eclipse
IntelliJ IDEA
NetBeans
Comparison Table: Editor vs. IDE
Feature | Editor | IDE |
Code Typing | Yes | Yes |
Syntax Highlighting | Limited or Requires Plugins | Built-in |
Compiling Code | No | Yes |
Debugging Tools | No | Yes (Advanced Debugging Features) |
Code Suggestions | No | Yes (Auto-completion, Syntax Hints) |
Project Management | No | Yes (Supports Large Projects) |
Java: Verbose but Powerful
Java is known for being verbose, meaning you have to clearly specify every detail in your code. Unlike some other programming languages where you can omit certain syntactical elements, Java requires you to:
Specify data types for variables.
End every statement with a semicolon (;).
Use well-structured syntax, such as clearly defining classes and methods.
Example:
int sum = 6 + 3; // A simple statement must end with a semicolon (;)
System.out.println(sum); // Printing the result
While Java requires more typing compared to some languages, this verbosity ensures clarity, making it easier to understand, debug, and maintain large programs.
Summary
JShell, introduced in Java 9, is a fantastic way to experiment with Java in an interactive environment. For more substantial projects, you would use an IDE like Eclipse or IntelliJ IDEA, which offers features like debugging, project management, and code suggestion tools. Java’s verbosity, while requiring more detailed code, provides a clear and structured approach to programming.
If you're new to Java, using JShell to test snippets is a great way to learn before moving on to full IDEs for developing complete applications.
3. Writing Your First Java Program
In Java, everything is centered around classes. A class is essentially a blueprint for objects, and it's where all Java programs begin. The main()
method acts as the starting point of every Java program. Let’s walk through the structure of a basic Java program.
Starting Point of a Java Program: The main()
Method
The main method is where the Java Virtual Machine (JVM) begins the execution of your program. Below is a simple example of a Java program with the main()
method:
public class FirstProgram {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
Here is a detailed breakdown of the program:
Keyword | Explanation |
public | Makes the method accessible from anywhere (across all classes and projects). |
static | Allows the JVM to call the method without creating an object of the class. |
void | Indicates that the method does not return any value. |
String[] args | Represents an array of command-line arguments passed to the program during execution. |
Explanation:
Class Declaration:
public class FirstProgram
declares a class named FirstProgram. In Java, everything must be inside a class.main()
Method: This is the method where execution starts. The lineSystem.out.println("Hello, Java!");
prints the message "Hello, Java!" to the console.
4. Compiling and Interpreting Java Code
Once you've written your Java program, the process of execution involves two key steps:
Compiling
Interpreting
The compiler converts your Java source code into bytecode, which the JVM (Java Virtual Machine) then interprets and executes.
Let’s break down the steps visually:
Flowchart: Compiling and Running Java Code
.java (Source Code)
|
|---(Compiling using javac command)-->
|
.class (Bytecode)
|
|---(Interpreting using JVM)-->
|
Machine Language Code (Executed by CPU)
|
v
Output (Displayed on Screen)
Key Steps in Compiling and Running Java Code
Process | Description |
Compiling | The source code (written in .java files) is converted into bytecode (in .class files) using the javac command. |
Interpreting | The bytecode is read by the JVM, which translates it into machine language (binary code) that the CPU can execute. |
Example:
$ javac FirstProgram.java // This compiles the Java file into bytecode
$ java FirstProgram // This executes the compiled bytecode
The
javac
command compiles the Java source file into a.class
file containing bytecode.The
java
command runs the compiled bytecode, and the JVM executes it.
Key Notes on Compiling and Running Java Code
Source Code (.java): This is the code you write, stored in a
.java
file.Compiling: You use the
javac
(Java Compiler) command to convert the.java
file into a.class
file (bytecode).Bytecode (.class): The compiled code that can be executed on any platform via the JVM.
JVM: The Java Virtual Machine reads and executes the bytecode, making Java platform-independent.
Machine Language: The code is further translated into machine-specific instructions that the CPU executes.
Commands for Compiling and Running Java Programs
Command | Description |
javac <file name> | Compiles the source file into bytecode. Example: javac FirstProgram.java |
java <class name> | Runs the compiled bytecode. Example: java FirstProgram |
Example Process:
Compiling:
javac FirstProgram.java
This command compiles the file
FirstProgram.java
and creates a file calledFirstProgram.class
.Running:
java FirstProgram
This command runs the compiled code using the JVM, and you’ll see the output:
Hello, Java!
Important Rules for Compiling and Running Java Code
File Name vs. Class Name:
There’s no compulsion for the file name and class name to be the same unless the class is public.
If the class is declared
public
, the file name must match the class name.
Example:
public class MyProgram {
public static void main(String[] args) {
System.out.println("This is MyProgram");
}
}
- If this class is declared
public
, the file should be saved asMyProgram.java
.
Multiple Classes in One File:
- You can define multiple classes in a single
.java
file, but only one class can be declared public.
- You can define multiple classes in a single
Example:
class ClassA {
// ClassA code
}
class ClassB {
// ClassB code
}
- In this case, both
ClassA
andClassB
can be present in the same file, but neither is public.
Packaging Techniques in Java
Java programs can be packaged for distribution across different operating systems. Here are some common packaging techniques:
Packaging | Platform | Description |
.exe | Windows | The program is packaged as an executable for Windows. |
.pkg | Mac | Used for Mac OS applications. |
.deb | Linux | A Debian package format used for Linux distributions. |
.jar | Java Archive (All OS) | Java Archive containing compiled class files and resources. |
Example of a .jar
File:
A
.jar
(Java ARchive) file can contain multiple.class
files, libraries, and metadata.Some
.jar
files are executable, meaning you can run them directly if they contain amain()
method.
java -jar MyProgram.jar
The Role of the Operating System (OS)
The Operating System (OS) manages the execution of all software programs, including Java applications. Here’s a simplified flow:
Source File (.java) --> Java Compiler (javac) --> Bytecode (.class) --> JVM --> CPU (Machine Code) --> Output
The JVM looks for the
main()
method as the entry point of the program.If the
main()
method is not found, the JVM won’t execute the program and throws an error.
Conclusion
Understanding the process of compiling and running Java code is critical for any Java developer. The use of commands like javac
and java
allows us to convert source code into machine-executable code. Java's Write Once, Run Anywhere (WORA) feature is facilitated by the JVM, which interprets bytecode into platform-specific instructions.
Java is a structured and verbose language, and the main()
method is the starting point for any application. Following these concepts ensures a solid foundation for learning Java programming.
By combining basic programming concepts with effective tools like JShell, Editors, and IDEs, you can efficiently write, compile, and run Java applications across platforms.
5. Java Virtual Machine (JVM)
The Java Virtual Machine (JVM) is a critical component of the Java ecosystem, allowing Java's "Write Once, Run Anywhere" (WORA) capability. It’s designed to run Java bytecode (.class files) on any platform, making Java programs platform-independent.
Role of JVM in Java's Architecture
When a Java program is written and compiled, it is converted into bytecode (.class file) by the Java compiler (javac
). The JVM is responsible for interpreting this bytecode and converting it into machine language that the underlying operating system (OS) can execute. This layer of abstraction allows Java programs to run on multiple platforms without modification.
Key Responsibilities of the JVM
Responsibility | Explanation |
Bytecode Interpretation | The JVM reads and translates bytecode into machine code that is specific to the host operating system. |
Memory Management | The JVM manages memory allocation and garbage collection to free up unused objects. |
Security Management | The JVM enforces security rules through its sandbox model, protecting the host system from malicious code. |
Platform Independence | The JVM allows Java programs to run on different platforms (Windows, Linux, macOS) without modification. |
Execution of the Program | The JVM is responsible for starting and executing a program by locating the main() method. |
How the JVM Works: Step-by-Step Execution
Compilation to Bytecode:
- When you compile a Java file (
javac
MyProgram.java
), the compiler converts the source code into bytecode stored in.class
files.
- When you compile a Java file (
JVM Execution:
The JVM loads the
.class
file and reads the bytecode.It then converts this bytecode into machine-specific instructions based on the platform (e.g., Windows, macOS, Linux).
Main Method Check:
The JVM looks for the
main()
method as the entry point for execution. This method must follow the signature:public static void main(String[] args)
If the
main()
method is not found, the JVM throws an error and does not give control to the CPU for execution.
Example:
If you run a program without a main()
method:
public class NoMainExample {
static {
System.out.println("This will not be executed.");
}
}
When you try to run the above code with the following command:
java NoMainExample
The JVM will throw an error like:
Error: Main method not found in class NoMainExample, please define the main method as:
public static void main(String[] args)
Structure of the JVM
The JVM consists of three main components:
Component | Function |
Class Loader | Loads .class files into memory for execution. |
Runtime Memory | Manages different memory areas like Heap (for objects) and Stack (for methods and local variables). |
Execution Engine | Executes the bytecode, converting it into machine-specific code. It consists of the Interpreter (executes bytecode) and JIT Compiler (optimizes). |
JVM's Handling of the Main Method
JVM Initialization:
- The JVM starts by initializing its components (like the Class Loader and Memory Areas).
Class Loading:
- The Class Loader loads the
.class
file containing the main method.
- The Class Loader loads the
Main Method Invocation:
The Execution Engine looks for the signature of the
main()
method, and if found, it begins the execution of the program.If the
main()
method is missing, the JVM will terminate execution and throw an error.
Types of JVM Implementations
Java offers different implementations of the JVM based on the platform. Some common implementations include:
JVM Type | Platform |
HotSpot JVM | Used on most desktop environments (Windows, Linux, macOS). |
Dalvik VM | Used in Android-based systems. |
GraalVM | A polyglot virtual machine supporting multiple languages. |
Conclusion
The JVM is at the heart of Java's cross-platform capability. It is responsible for interpreting and executing Java bytecode and managing resources like memory and security. By handling the bytecode generated from Java source files, the JVM enables Java programs to run seamlessly on any operating system that has a JVM installed.
6. Java Methods
A method in Java is a reusable block of code that performs a specific task. Similar to functions in other programming languages, methods allow developers to divide complex problems into smaller, more manageable sections of code.
Components of a Java Method
A method in Java typically consists of the following elements:
Method Name: The identifier by which the method is called (e.g.,
sum
,printMessage
).Parameters: Optional inputs passed to the method for processing (e.g.,
int a
,int b
).Return Type: The type of value that the method will return (e.g.,
int
,String
,void
). If the method does not return a value, the return type isvoid
.Method Body: The block of code that executes when the method is called.
Syntax for Declaring a Method
Here’s the general syntax for a Java method:
returnType methodName(parameterType1 parameterName1, parameterType2 parameterName2, ...) {
// method body
return value; // (if returnType is not void)
}
returnType
: Specifies what the method will return.methodName
: The name used to call the method.parameters
: Optional values provided to the method to perform its task.return value
: The value returned from the method (if any).
Example: Sum of Two Numbers
Let’s look at an example where a method calculates the sum of two numbers:
public class Calculator {
// Method to add two numbers and return the result
public int sum(int a, int b) {
return a + b; // returns the sum of a and b
}
}
Method Name:
sum
Parameters: Two integers
a
andb
Return Type:
int
(since the method returns the sum)Body: The addition operation (
a + b
)
Example: Void Method
If a method doesn’t need to return a value, we use void
as the return type. For example:
public class Printer {
// Method to print a message
public void printMessage() {
System.out.println("Hello, Java!");
}
}
In this case:
Return Type:
void
(no return value)Method Name:
printMessage
Body: Prints "Hello, Java!" to the console.
Pseudocode Example: Sum of Two Numbers
Here is a simplified pseudocode example for calculating the sum of two numbers:
method sum(a, b)
result = a + b
return result
The pseudocode highlights the key elements of a method:
The method
sum()
takes two parametersa
andb
.The body contains an addition operation.
The result is returned.
7. The Main Method and JVM
The main()
method is crucial in every standalone Java program. It serves as the starting point for execution. Without it, the Java Virtual Machine (JVM) wouldn’t know where to begin the execution process.
Importance of the public static void main(String[] args)
Signature
Let's break down why this specific signature is necessary:
public: The
main()
method needs to be accessible by the JVM from anywhere. Withoutpublic
, the JVM will not be able to access the method, and the program will not run.static: The
main()
method is called without creating an object of the class. The JVM directly invokes it without needing to instantiate the class.void: The
main()
method doesn’t return any value.String[] args: This array stores any command-line arguments passed to the program during execution.
Example of the Main Method
public class MainExample {
public static void main(String[] args) {
System.out.println("This is the main method.");
}
}
When you run this code, the JVM looks for the main()
method and starts execution from there. The System.out.println()
statement prints the message to the console.
Explanation of the JVM's Role in Program Execution
The Java Virtual Machine (JVM) plays a crucial role in running a Java program. Here's how the process works:
JVM Initialization: When you run a Java program, the JVM is initialized. It is responsible for loading, verifying, and interpreting the bytecode (.class file).
Class Loading: The JVM loads the class containing the
main()
method.Main Method Check: The JVM searches for the
main()
method inside the class.If it finds the
main()
method with the correct signature (public static void main(String[] args)
), it starts execution.If the
main()
method is missing, or its signature is incorrect, the JVM throws an error and stops execution.
Example: JVM Unable to Find Main Method
If a class does not contain a main()
method, the JVM will not execute the program. For example:
public class NoMainExample {
public void greet() {
System.out.println("Hello, there!");
}
}
When you try to run the code:
$ java NoMainExample
The JVM will throw an error like:
Error: Main method not found in class NoMainExample, please define the main method as:
public static void main(String[] args)
This demonstrates that the JVM requires the main()
method to begin execution.
Why is the public static
Part Essential?
public: The JVM needs to access the
main()
method from anywhere, hence the method must be public.static: The method should be called without creating an object of the class. For example:
public class MainExample {
public static void main(String[] args) {
System.out.println("This is the static main method.");
}
}
In this code:
The
main()
method is static, so it can be called by the JVM without creating an object.If the
main()
method were not static, the JVM would not be able to call it automatically.
Conclusion
Java Methods: Methods in Java allow you to organize code into reusable blocks. Each method has a name, parameters, a return type, and a body.
Main Method and JVM: The
main()
method is the entry point for Java programs, and without it, the JVM cannot execute the program. Thepublic static void main(String[] args)
signature is critical because it makes the method accessible and callable without creating an object.
8. Command-Line Arguments in Java
Command-line arguments allow you to pass data to your Java program during execution via the terminal or command prompt. These arguments are captured in the String[] args
array in the main()
method and can be used by the program to perform different tasks depending on the input provided.
How Command-Line Arguments Work
String[] args
: This array holds the command-line arguments passed when running a Java program.The arguments are always stored as strings, regardless of the type of data (e.g., integers, characters, or strings). If needed, you can convert them to the appropriate type inside the program.
Syntax of the Main Method with Command-Line Arguments
public static void main(String[] args) {
// args[0], args[1], ..., args[n] represent the arguments passed to the program
}
args[0]
refers to the first command-line argument.args[1]
refers to the second command-line argument, and so on.
Example: Passing Arguments from Command Line
public class ArgumentExample {
public static void main(String[] args) {
System.out.println("Argument 1: " + args[0]);
System.out.println("Argument 2: " + args[1]);
}
}
Explanation
String[] args
: This parameter allows the program to receive and store any arguments passed during execution.args[0]
: Represents the first argument passed (e.g., "Hello").args[1]
: Represents the second argument passed (e.g., "Java").
Running the Program with Arguments
If you compile and run the above program with the following command:
$ java ArgumentExample Hello Java
The output will be:
Argument 1: Hello
Argument 2: Java
Memory Map for Command-Line Arguments
Memory Location | Stored Value | Description |
args[0] | "Hello" | First command-line argument |
args[1] | "Java" | Second command-line argument |
Each index in the args
array holds a string value representing the argument passed. If no arguments are passed, the args
array will be empty (args.length == 0
).
Handling Command-Line Arguments in Code
Here’s another example where we handle the arguments dynamically based on the number of arguments passed.
public class DynamicArguments {
public static void main(String[] args) {
// Check if arguments are passed
if (args.length == 0) {
System.out.println("No arguments passed.");
} else {
// Print all arguments
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + (i + 1) + ": " + args[i]);
}
}
}
}
Running the Program Without Arguments
$ java DynamicArguments
Output:
No arguments passed.
Running the Program With Arguments
$ java DynamicArguments Data Science
Output:
Argument 1: Data
Argument 2: Science
9. File Naming Conventions and Java Classes
In Java, file naming conventions play a crucial role in ensuring that the program runs correctly. The file name and the class name need to follow specific rules, especially when dealing with public classes.
Key Rules for File Naming
Class and File Name: If a class is marked as
public
, the file name must match the class name.Non-public Classes: If the class is not marked as public, the file name does not have to match the class name. You can have multiple non-public classes in the same file.
File Extension: Every Java file should have the
.java
extension.
Example 1: Public Class
public class Test {
public static void main(String[] args) {
System.out.println("Test class.");
}
}
File Name:
Test.java
(must match the public classTest
).Main Method: The entry point for the program, where the JVM starts execution.
To compile and run this code:
$ javac Test.java
$ java Test
Output:
Test class.
Example 2: Non-public Class
class Example {
public static void main(String[] args) {
System.out.println("Example class.");
}
}
File Name: Can be
Anything.java
(does not need to match the class name).The class
Example
is not public, so the file name is flexible.
How Command-Line Arguments Work with Main Method
The String[] args
parameter in the main()
method allows the program to accept input from the command line. Even if no arguments are passed, you must include String[] args
in the main()
method signature for the program to compile and run.
For example:
public class PrintArguments {
public static void main(String[] args) {
System.out.println("Argument: " + args[0]); // Access first argument
}
}
If you run this program like this:
$ java PrintArguments Hello
Output:
Argument: Hello
Command-Line Argument Type
Regardless of what type of argument you pass (whether it's an integer, float, or string), all command-line arguments are received as strings in Java.
For example, if you pass an integer:
$ java PrintArguments 123
The program will treat 123
as a string:
Argument: 123
If you want to treat the command-line argument as a specific type, you need to convert it. For example, to convert a string to an integer:
public class ConvertArgument {
public static void main(String[] args) {
// Convert the first argument from String to int
int num = Integer.parseInt(args[0]);
System.out.println("Converted Number: " + num);
}
}
Running this program:
$ java ConvertArgument 123
Output:
Converted Number: 123
Code Explanation: Command-Line Argument Handling
Here’s a more detailed breakdown of handling command-line arguments:
public class ExampleArgument {
public static void main(String[] args) {
// Example for handling command-line arguments
if (args.length > 0) {
// Print each argument
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + (i + 1) + ": " + args[i]);
}
} else {
System.out.println("No arguments were passed.");
}
}
}
Memory Storage of Arguments: Each argument is stored as a separate string in the
args[]
array.Conditional Check:
args.length > 0
checks if any arguments were passed.Loop: The loop iterates over all the arguments and prints them.
Running the Program with Multiple Arguments
$ java ExampleArgument Apple Banana Cherry
Output:
Argument 1: Apple
Argument 2: Banana
Argument 3: Cherry
Table: Command-Line Arguments Handling
Command | Output | Explanation |
$ java ExampleArgument | No arguments were passed. | No command-line arguments provided. |
$ java ExampleArgument Hi | Argument 1: Hi | One argument passed: "Hi" |
$ java ExampleArgument 123 | Argument 1: 123 | Passed as a string, not a number |
$ java ExampleArgument A B | Argument 1: A | |
Argument 2: B | Two arguments passed: "A" and "B" | |
$ java ExampleArgument 1 2 | Argument 1: 1 | |
Argument 2: 2 | Numbers treated as strings |
Conclusion
Command-Line Arguments: Java allows you to pass arguments to your program from the command line. These arguments are captured in the
String[] args
array.File Naming and Classes: Public classes require the file name to match the class name. Non-public classes can have flexible file names.
Arguments Handling: All command-line arguments are passed as strings, even if they look like numbers. You need to convert them to the appropriate type if necessary.
10. Packages and .jar Files
In Java, packages are used to group related classes and interfaces into a single namespace, making it easier to organize and manage large applications. When distributing Java applications, these packages and their .class
files can be bundled into a .jar
(Java Archive) file.
Key Points About .jar
Files
.jar
File: A compressed archive that contains compiled.class
files and metadata. It can include resources like images, sound files, and configuration files.Purpose:
.jar
files allow for easy distribution and execution of Java applications as a single unit. Instead of handling multiple files, you can work with one.jar
file.Execution: If the
.jar
file contains an entry point (i.e., amain()
method), it can be executed with the following command:$ java -jar application.jar
Real-World Example of a .jar
File
Imagine you download a calculator application packaged as calculator.jar
. Inside this .jar
file, you may have the following:
Add.class: Handles addition.
Subtract.class: Handles subtraction.
Multiply.class: Handles multiplication.
Divide.class: Handles division.
resources/: Contains configuration files or icons.
This makes distribution and execution convenient as everything is packaged into a single .jar
file.
11. Static vs. Dynamic Typing
Java is a statically typed language, meaning the type of a variable is known and enforced during compile time. In contrast, languages like Python and JavaScript are dynamically typed, where variable types are determined at runtime.
Difference Between Statically Typed and Dynamically Typed Languages
Feature | Statically Typed | Dynamically Typed |
Type Check | At compile time | At runtime |
Error Detection | Errors are detected early (during compile) | Errors are detected later (during execution) |
Variable Declaration | Type must be declared explicitly | Type is inferred automatically |
Examples | Java, C++ | Python, JavaScript |
Performance | Generally faster, as type checks are done beforehand | Can be slower due to runtime type checking |
12. Phases of Java Code Execution
Java applications go through different phases before they are deployed and used by end-users. These phases involve multiple stakeholders, including developers, testers, and end-users.
The Three Phases of Java Code Execution
Development:
This is where the code is written by developers.
Code is compiled into
.class
files using the Java compiler (javac
).During this phase, developers may pass command-line arguments to test specific features of the program.
Testing:
The application is tested by QA engineers.
Testers run the application to ensure that all features behave as expected.
Command-line arguments can be used to simulate different test cases.
Production:
The final phase where the code is released to end-users.
In production environments, Java applications may use different JVM configurations (e.g., memory allocation) to suit the hardware and operating system.
Command-line arguments may be passed to the JVM to configure the environment or provide inputs for execution.
Command-Line Arguments in Eclipse
To pass command-line arguments in Eclipse:
Right-click on your Java file and select Run As > Run Configurations.
In the Run Configurations window, select the Arguments tab.
Under the Program Arguments section, type in the arguments you want to pass.
Click Run to execute the program with the specified arguments.
Purpose of Command-Line Arguments
Input Flexibility: Allows the user to pass dynamic input at runtime without modifying the code.
Configuration: Used to change JVM or program settings (e.g., memory size, file paths).
Testing: Facilitates testing various cases without hardcoding values into the program.
Difference Between .class
File and .war
File
Feature | .class File | .war File |
Definition | A compiled Java file containing bytecode | A Web Application Archive file |
Purpose | Holds bytecode for one Java class | Packages web applications (JavaServer Pages, Servlets, HTML, etc.) |
Usage | Can be executed by the JVM | Used to deploy web applications on a server |
Contains | A single class | Multiple files including .class , configuration files, HTML, JSP, etc. |
Deployed | On any Java runtime environment (JVM) | On web servers such as Apache Tomcat |
The Role of JVM, JRE, and Compilation
When a Java program is executed, the following process takes place:
Compilation:
Source code (
.java
files) is compiled into bytecode (.class
files) by the Java compiler.At this stage, the JVM is not involved. Only the compiler (
javac
) is used.
Execution:
The JVM (Java Virtual Machine) loads and executes the
.class
files.The JVM interacts with the JRE (Java Runtime Environment), which includes the libraries and components needed to run the program.
The
main()
method is the entry point for Java execution. Every program must have amain()
method, which the JVM calls to start execution.
Example: Execution Process
Consider the following simple Java program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Execution Steps
Development: The developer writes the
HelloWorld.java
file.Code is compiled:
$ javac
HelloWorld.java
.This generates
HelloWorld.class
.
Testing: Testers run the
.class
file in various environments.Program execution:
$ java HelloWorld
.Output:
Hello, World!
Production: End-users receive the
.jar
or.war
file, which they can run with the JVM.
Key Components
JVM: Executes the bytecode.
JRE: Provides the necessary libraries and runtime environment.
Compiler (
javac
): Converts.java
files to.class
files.
Conclusion
Packages and .jar Files: Packages organize code, and
.jar
files bundle applications for easy distribution.Static vs. Dynamic Typing: Java uses static typing for early error detection, while languages like Python use dynamic typing for flexibility.
Phases of Code Execution: Java applications go through development, testing, and production phases, with different configurations at each stage.
JVM and JRE: The JVM executes bytecode with the help of the JRE, while the compiler converts code into
.class
files.
13. Understanding JDK, JRE, and JVM
JDK (Java Development Kit): Includes the tools needed to write, compile, and run Java code (like the compiler and JRE).
JRE (Java Runtime Environment): Used to run Java programs (contains the JVM).
JVM (Java Virtual Machine): Executes bytecode and runs the program.
Component | Function |
JDK | Developer tool (compile & run code) |
JRE |
| Runtime (run code) | | JVM | Executes the bytecode |
14. Common Java Errors
Compile-Time Errors: Detected before the program runs (e.g., missing semicolons). Runtime Errors: Detected while the program runs (e.g., division by zero). Logical Errors: Program runs but produces incorrect results (e.g., incorrect logic).
Examples of Errors
- Syntax Error
System.out.println("Hello);
Output: Compile-time error due to missing quotation mark.
- Runtime Error
int a = 5/0;
Output: Runtime error due to division by zero.
15. Conclusion
In this chapter, we’ve walked through writing our first Java program, understanding the role of JShell, exploring the Java development and execution process, and learning how the JVM works. Armed with these foundational concepts, you're now ready to tackle more complex Java programs in your journey to becoming a Full Stack Java Developer.
Further Reading and Resources
If you found this post insightful, you might be interested in exploring more detailed topics in my ongoing series:
Full Stack Java Development: A comprehensive guide to becoming a full stack Java developer.
DSA in Java: Dive into data structures and algorithms in Java with detailed explanations and examples.
HashMap Implementation Explained: Understand the underlying mechanics of Java’s HashMap.
Inner Classes and Interfaces in Java: Explore the intricacies of inner classes and interfaces.
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repositories and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊