Mastering Java Classes and Objects: A Comprehensive Beginner's Guide

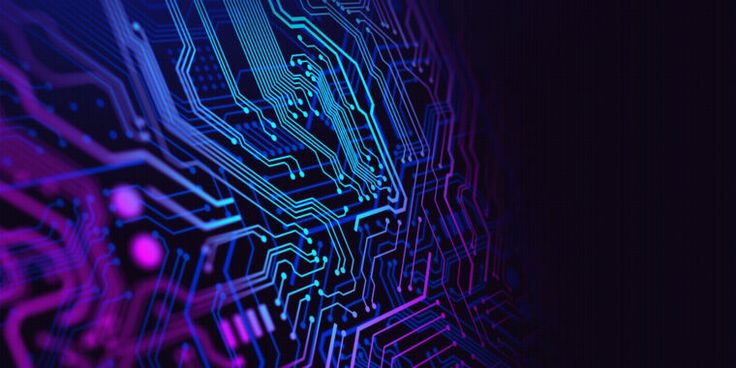
Object-Oriented Programming (OOP) is a powerful paradigm that helps developers create flexible, modular, and reusable code.Before diving into OOP, let’s first understand what an object is, how it behaves, and get a brief description of a class.
What is a Class?
A class is a blueprint for creating objects. It defines the properties (attributes) and behaviors (methods) that the objects created from the class will have. Classes are fundamental to Java's object-oriented programming paradigm, allowing for code reuse, organization, and encapsulation.
Let’s consider the example of a car. The Car
class is like a blueprint for a car. It defines what properties a car should have (color, make, model, speed) and what actions it can perform (accelerate, brake, honk).
Why Use Classes?
Organization: Classes help organize your code into manageable sections.
Reusability: Once you create a class, you can use it to create multiple objects without rewriting the code.
Modularity: Classes allow you to break down complex programs into simpler, more understandable pieces.
Syntax
class ClassName {
// Attributes (fields)
DataType attributeName;
// Constructor
ClassName(DataType parameter) {
// Initialize attributes
this.attributeName = parameter;
}
// Methods
ReturnType methodName(ParameterType parameter) {
// Method body
}
}
Explanation
class ClassName: This line defines a new class. Replace
ClassName
with the name you want to give your class. By convention, class names start with an uppercase letter.Attributes (fields): These are variables that define the state or properties of the class. For example, if you're creating a
Dog
class, you might have attributes likeString name;
to store the dog's name.Constructor: A constructor is a special method that is called when an object of the class is created. It often initializes the class attributes. The constructor has the same name as the class and does not have a return type. In the constructor, you can use the
this
keyword to refer to the current object's attributes, differentiating them from the constructor parameters.Methods: Methods define the behaviors or actions that objects of the class can perform. They can take parameters and return values. The syntax
ReturnType methodName(ParameterType parameter)
specifies that a method namedmethodName
returns a type defined byReturnType
and accepts a parameter of typeParameterType
.
What is an Object?
An object is an instance of a class that represents a specific entity. Each object has attributes (or properties) that define its state and methods (or functions) that define its behavior. Think of an object as a self-contained unit that bundles data and operations that can be performed on that data. An object is a real-world entity that contains the behavior and properties defined by the class.
Let’s consider the example of the Car
class. Suppose the Car
class contains behaviors like start, stop, accelerate, brake, and play music, and properties such as 4 wheels, a steering wheel, a music system, an engine, wipers, and mirrors. Objects occupy memory space, whereas classes do not.
Consider another example of a Dog
class. The Dog
class defines a blueprint with 4 legs, 2 eyes, 2 ears, and various colors. A dog object will be a specific dog with properties like being red in color and behaviors like barking, eating, and sleeping.
How to Create an Object
To create an object in Java, follow these steps:
Define a Class: Create a class that serves as a blueprint.
Instantiate the Object: Use the
new
keyword to create an instance of the class.Initialize the Object: Optionally, initialize the object's properties using a constructor or by directly setting the values.
Example: Creating a Car Object
public class Car {
// Properties
String color;
String make;
String model;
int speed;
// Constructor
public Car(String color, String make, String model) {
this.color = color;
this.make = make;
this.model = model;
this.speed = 0; // Default speed
}
// Methods
void accelerate() {
speed += 10;
}
void brake() {
speed -= 10;
}
}
public class Main {
public static void main(String[] args) {
// Creating an object of the Car class using the constructor
Car myCar = new Car("Red", "Toyota", "Corolla");
// Displaying initial state
System.out.println("Car make: " + myCar.make); // Output: Car make: Toyota
System.out.println("Car model: " + myCar.model); // Output: Car model: Corolla
System.out.println("Car color: " + myCar.color); // Output: Car color: Red
System.out.println("Car speed: " + myCar.speed); // Output: Car speed: 0
// Using the object's methods
myCar.accelerate();
System.out.println("Car speed after accelerating: " + myCar.speed); // Output: Car speed after accelerating: 10
}
}
Understanding Constructors
A constructor in Java is a special method that is called when an object of a class is created. It is used to initialize the object's properties and set up any necessary initial state. Constructors have the same name as the class and do not have a return type, not even void
.
Key Points About Constructors
Name: The constructor's name must exactly match the class name.
No Return Type: Constructors do not have a return type.
Initialization: They are primarily used to initialize the object's attributes.
Overloading: You can have multiple constructors in a class with different parameters (constructor overloading).
Why Use Constructors?
Initialization: Constructors allow you to set initial values for an object's properties.
Encapsulation: They help encapsulate the setup logic within the class, making the code cleaner and more maintainable.
Flexibility: With constructor overloading, you can provide multiple ways to initialize an object, offering flexibility in object creation.
By understanding and using constructors, you can ensure that your objects are properly initialized and ready to use right from the moment they are created. This is a fundamental aspect of object-oriented programming in Java.
By understanding these basic concepts, you can start creating and working with objects in Java, leveraging the power of object-oriented programming to build flexible, modular, and reusable code. Happy coding!
Subscribe to my newsletter
Read articles from Akash Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akash Das
Akash Das
Java Developer | Tech Blogger | Spring Boot Enthusiast | DSA Advocate*I specialize in Java, Spring Boot, and Data Structures & Algorithms (DSA). Follow my blog for in-depth tutorials, best practices, and insights on Java development, Spring Boot, and DSA. Join me as I explore the latest trends and share valuable knowledge to help developers grow.