Docker and Dockerized Applications

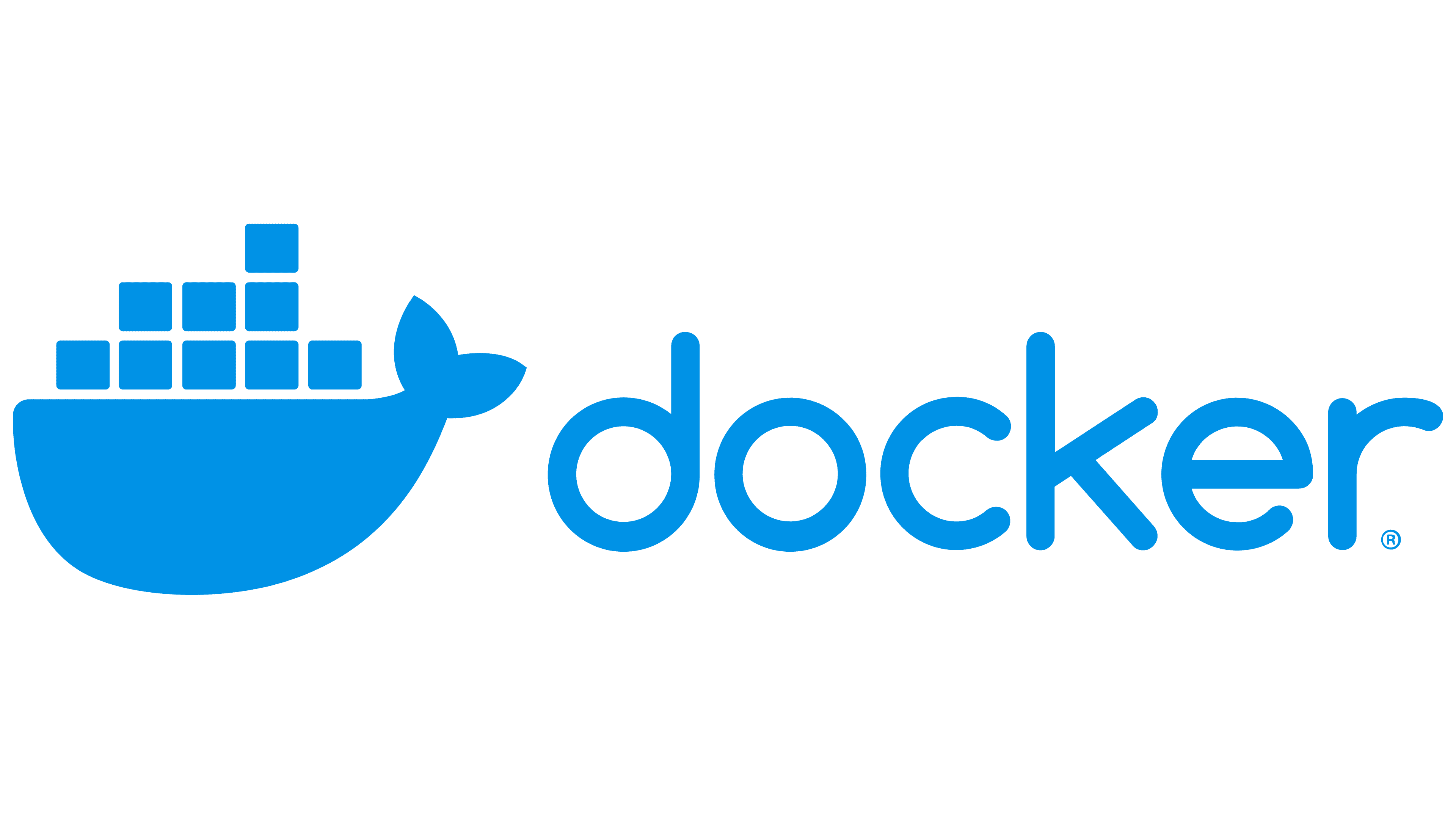
What is Docker?
Docker is a platform that allows developers to automate the deployment of applications inside lightweight, portable containers. Containers include everything an application needs to run, such as libraries, system tools, code, and runtime. By using Docker, you ensure that the application will work the same way across different environments, from development to production.
The key benefit of Docker is that it allows developers to package their applications and dependencies together in a "container" and ship them anywhere. Containers are lightweight and isolated but share the host machine's operating system, making them faster to start and use fewer resources than traditional virtual machines.
Popular Docker Tools
Docker Engine: The core tool for creating and running containers. It provides the environment to execute containers and manage them.
Docker Compose: A tool that allows you to define and manage multi-container Docker applications. You can describe multiple services in one YAML file and manage them with a single command.
Docker Swarm: Docker’s native clustering tool that turns a pool of Docker hosts into a single, virtual Docker host. Swarm manages containers across multiple Docker hosts and enables the scaling of containerized applications.
Kubernetes: Though not Docker-specific, it’s a powerful orchestration tool used for deploying, scaling, and managing containerized applications in a distributed environment. It integrates well with Docker containers.
Docker Hub: A cloud-based registry where Docker images can be stored, shared, and accessed. Developers use Docker Hub to upload and pull Docker images for use in their applications.
How to Dockerize an Application
Dockerizing an application means packaging the application along with all of its dependencies into a Docker container so that it can be run anywhere. Below are the steps to Dockerize an application:
Install Docker: First, install Docker on your machine (either on Linux, macOS, or Windows).
Prepare the Application: Ensure your application files and dependencies are in a single directory. For example, if you have a Node.js application, your
app.js
file andpackage.json
file should be in one folder.Create a Dockerfile: A Dockerfile is used to specify the steps required to build a Docker image for your application. This file will include instructions such as installing dependencies, copying the application code, and defining how the application should run.
Build the Docker Image: After creating the Dockerfile, you can use the
docker build
command to create a Docker image from the Dockerfile. For example:docker build -t myapp .
Run the Docker Container: Once the image is built, you can start the container using the
docker run
command:docker run -d -it -p 3000:3000 --name="container_name" "image_name"
What is a Dockerfile?
A Dockerfile is a text file that contains a series of commands and instructions that are executed to assemble a Docker image. Each command in a Dockerfile creates a layer in the image, and together they define everything needed to run an application.
FROM: Specifies the base image to use (e.g.,
FROM node:14
means starting with a Node.js environment).WORKDIR: Sets the working directory inside the container.
COPY: Copies files from your host machine to the container.
RUN: Runs commands during the image build (e.g., installing dependencies).
CMD: Specifies the command to run when the container starts (e.g., starting a web server).
Example of a Dockerfile for a Python app:
FROM python:3.9 WORKDIR /app COPY requirements.txt ./ RUN pip install -r requirements.txt COPY . . CMD ["python", "app.py"]
What is a Docker Compose File?
A Docker Compose file (usually named docker-compose.yml
) is a YAML file that defines how to run multi-container Docker applications. It allows you to specify the services your application needs, such as databases, web servers, and other backend services, and manage them together.
With Docker Compose, you can define your entire application's infrastructure in one file and then spin it up with a single command (docker-compose up
). It’s especially useful for development and testing environments where you need to run multiple services together.
Example of a Docker Compose file:
Let's say you have a web app that requires a Node.js server and a MongoDB database. Here's how a docker-compose.yml
file might look:
version: '3'
services:
web:
image: node:14
working_dir: /app
volumes:
- .:/app
ports:
- "3000:3000"
command: npm start
database:
image: mongo
ports:
- "27017:27017"
volumes:
- ./data/db:/data/db
version: Defines the version of the Docker Compose file.
services: Defines the different containers (e.g.,
web
anddatabase
).volumes: Mounts a directory from your host machine into the container.
ports: Maps a port from the container to your local machine (e.g., container port
3000
to host port3000
).
Why Docker Compose is Needed:
Simplifies multi-container applications: Instead of running multiple
docker run
commands for different containers, you can define everything in a single file and run them all with one command.Environment configuration: You can define all necessary services, networks, and volumes for your application in one file.
Consistency: Using Docker Compose ensures that everyone working on the project uses the same environment, reducing the “works on my machine” issues.
Subscribe to my newsletter
Read articles from Nahid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nahid
Nahid
I am Mozahidul Islam Nahid, an engineer driven by a passion for continuous learning and growth. With six years of diverse professional experience. Which includes one year as DevOps engineer and four and a half years as administration and procurement specialist. Now I am dedicated to advance my career in DevOps engineering and cloud engineering.I am particularly passionate about server management and ongoing maintenance of websites post-deployment and I aspire to be a crucial part of these essential tasks for any company . Thank you!