Day 63 - Terraform Variables
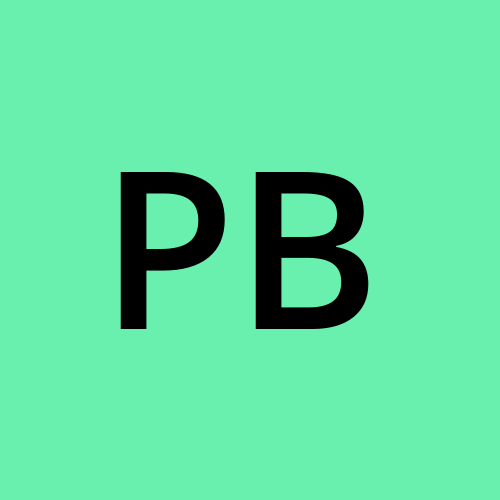
3 min read
- variables in Terraform are quite important, as you need to hold values of names of instance, configs , etc.
mkdir terraform-variables && cd terraform-variables
We can create a variables.tf file which will hold all the variables.
variable "filename" {
default = "/home/ubuntu/terraform-variables/demo-var.txt"
}
variable "content" {
default = "This is coming from a variable which was updated"
}
These variables can be accessed by var object in
main.tf
Task-01
- Create a local file using Terraform Hint:
resource "local_file" "demo-var" {
filename = var.filename
content = var.content
}
- After completing all the steps mentioned in Day 61's blog, we can use a command to get our
demo-var.txt
file.
terraform init
terraform plan
terraform apply
ls && cat demo-var.txt
Data Types in Terraform
Map
Terraform supports various data types like maps, lists, sets, and objects.
Using Map Data Type
For that create a folder for
data-type
and enter into itCreate a file
variables.tf
indata-type
folder and write below command
variable "filename" {
default = "/home/ubuntu/terraform-variables/data-type/map-file.txt"
}
variable "map_file_contents" {
type = map
default = {
"content1" = "this is cool"
"content2" = "this is cool feature"
}
}
- Again create a
main.tf
file to get ourmap_file_contents
Copy
Copy
resource "local_file" "map-file1" {
filename = var.filename
content = var.map_file_contents["content1"]
}
resource "local_file" "map-file2" {
filename = var.filename
content = var.map_file_contents["content2"]
}
- After completing all the steps mentioned in Day 61's blog, we can use a command to get our
map-file.txt
file.
terraform init
terraform validate
terraform plan
terraform apply
ls && cat map-file.txt
terraform apply
ls && cat map-file.txt
Task-02
Demonstrate usage of List, Set and Object datatypes
- Use terraform to demonstrate usage of List, Set and Object datatypes in your
data-type
folder
# vim variable.tf
-------------------------------------------------------------------------------
# List
variable "regions" {
type = list(string)
default = ["us-east-1", "us-east-2", "us-west-1"]
}
# Set
variable "environments" {
type = set(string)
default = ["dev", "staging"]
}
# Object
variable "instances" {
type = map(object({
instance_type = string
ami = string
}))
default = {
web = {
instance_type = "t2.micro"
ami = "ami-0c7217cdde317cfec"
}
db = {
instance_type = "t2.small"
ami = "ami-0c7217cdde317cfec"
}
}
}
- Create a file named
main.tf
with the following content:
# vim main.tf
-------------------------------------------------------------------------------
# List
output "regions_output" {
value = var.regions
}
# Set
output "environments_output" {
value = var.environments
}
# Object
output "instances_output" {
value = var.instances
}
- After completing all the steps mentioned in Day 61's blog, we can use a command to get our demonstrate usage of List, Set and Object datatypes
terraform init
terraform validate
terraform plan
terraform apply
- Run the following Terraform command to refresh the state and capture outputs:
terraform refresh
0
Subscribe to my newsletter
Read articles from Pooja Bhavani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
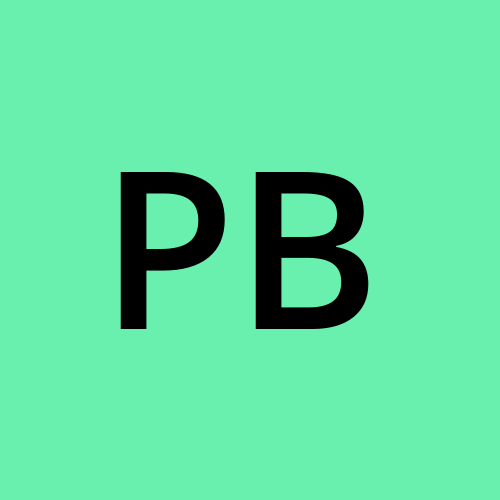