Building and Publishing Your First NPM Package: A Step-by-Step Guide

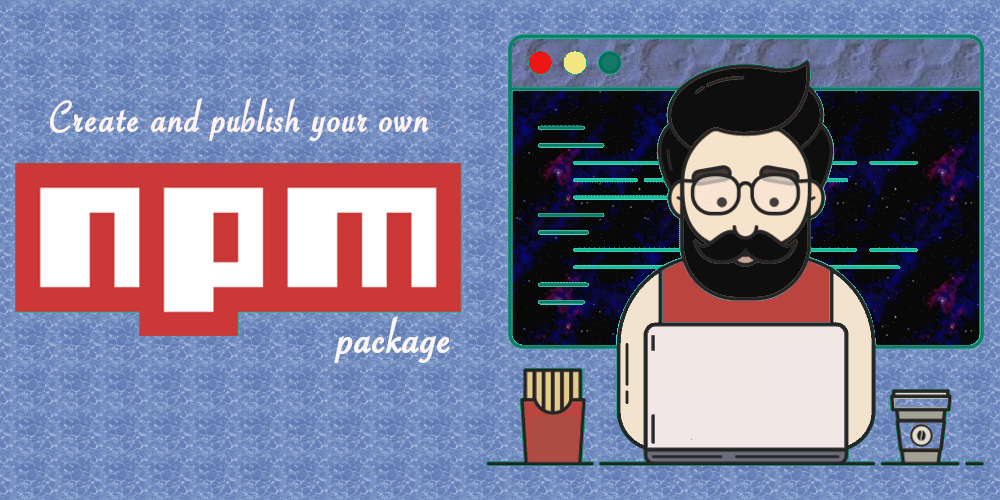
Creating and deploying an NPM (Node Package Manager) package is a great way to share reusable code with the developer community. Whether it’s a utility library, a configuration tool, or something more specific, NPM allows you to distribute your JavaScript code so others can use it. In this post, we'll walk through the process of creating an NPM package from scratch and deploying it to the public NPM registry.
Step 1: Setup Your Environment
Before creating your NPM package, ensure that you have the following:
Node.js and NPM: Make sure Node.js and NPM are installed. To check, run the following commands in your terminal:
node -v npm -v
If they're not installed, download them from Node.js.
NPM Account: To publish packages to the NPM registry, you need an NPM account. Create one at npmjs.com.
Step 2: Create the Project Directory
Create a New Directory: Begin by creating a directory for your project:
mkdir my-awesome-package cd my-awesome-package
Initialize NPM: Run
npm init
to generate thepackage.json
file. This file holds metadata about your project, such as its name, version, and dependencies.npm init
Follow the prompts and provide the required information (name, version, description, etc.). The important fields are:
name: The package name (should be unique on NPM).
version: Start with
1.0.0
or any version number of your choice.entry point: The main file of your project, typically
index.js
.
Step 3: Write Your Package Code
Now that your project is initialized, it’s time to write the code for your package. Create the main file (as specified in the entry point):
Create the main file: If you chose
index.js
as the entry point:touch index.js
Write Your Code: Let’s create a simple utility function that adds two numbers together. In
index.js
:function add(a, b) { return a + b; } module.exports = add;
This is just a basic example, but your package can be as complex as needed.
Step 4: Add a README
A good README file provides context and instructions on how to use your package. This file is essential, especially when publishing open-source projects.
Create a README.md
file:
touch README.md
Write a simple README:
# My Awesome Package
A simple NPM package that adds two numbers.
## Installation
```bash
npm install my-awesome-package
Usage
const add = require('my-awesome-package');
console.log(add(2, 3)); // Outputs 5
### Step 5: Create `.gitignore` and Prepare for Publishing
Create a `.gitignore` file to exclude unnecessary files when publishing to NPM:
```bash
touch .gitignore
Add these lines to .gitignore
to exclude node_modules
and other temporary files:
node_modules
*.log
Step 6: Version Control (Optional)
It’s good practice to track your changes using Git. Initialize a Git repository:
git init
git add .
git commit -m "Initial commit"
You can also push this project to a platform like GitHub, so users can view the source code.
Step 7: Test Your Package Locally
Before publishing, you should test your package locally to ensure it works correctly. You can do this by installing it locally using the npm link
command.
Run the following in your package directory:
npm link
In another directory (or a test project), use the package:
npm link my-awesome-package
Test it by importing and running the package:
const add = require('my-awesome-package'); console.log(add(10, 20)); // Outputs 30
Step 8: Publish to NPM
Now that your package is ready, it’s time to publish it to the NPM registry.
Login to NPM: First, log in to your NPM account using the CLI:
npm login
You’ll be prompted to enter your NPM username, password, and email.
Publish the Package: Once logged in, publish your package:
npm publish
If the package name is unique and everything is correct, your package will be successfully published to the NPM registry!
Versioning: In the future, as you make changes to your package, remember to increment the version number in
package.json
before publishing again. You can follow Semantic Versioning guidelines:npm version patch # For small fixes npm version minor # For adding new features without breaking backward compatibility npm version major # For breaking changes
Step 9: Use Your Package
Now that your package is live, others (and you) can install it using:
npm install my-awesome-package
After installation, anyone can import and use your package in their projects:
const add = require('my-awesome-package');
console.log(add(5, 6)); // Outputs 11
Bonus Tips:
Private Packages: If you want to publish a private package (only available to specific users), you can set
"private": true
in yourpackage.json
. Additionally, you can use NPM's paid plan to create private packages.Scoped Packages: To create a scoped package (usually for organizations or grouping packages), you can publish under a scope like
@myusername/my-awesome-package
by setting the name in yourpackage.json
accordingly.
Conclusion
And that’s it! You've successfully created, tested, and published your own NPM package. This process not only allows you to share your work with the world but also helps you maintain reusable code across projects.
Publishing an NPM package is a great way to contribute to the open-source ecosystem and improve your programming reputation. Once you get the hang of it, you can publish more sophisticated packages and even collaborate with other developers on larger projects.
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.