Basics of Programming

Table of contents
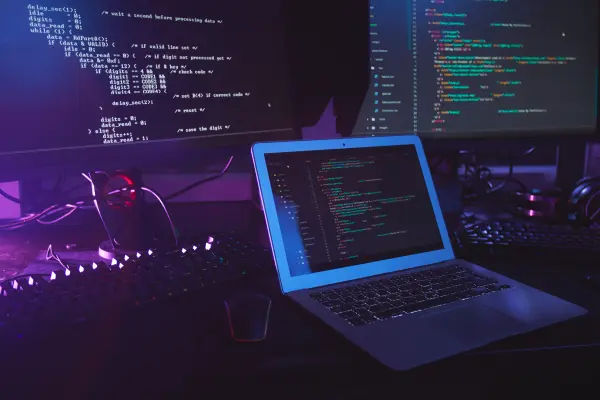
What is Programming?
Programming is the process of giving a computer a set of instructions to perform specific tasks. Think of it like writing a recipe, but instead of ingredients and steps for cooking, you're writing code that tells the computer exactly what to do. This set of instructions is called a program, and programming languages are the tools we use to communicate with computers.
Programming allows us to create everything from websites and apps to robots and AI systems. Whether you’re new to programming or have heard of it in passing, understanding its basics can open up a world of possibilities for you.
Key Concepts in Programming
Variables
Variables are like containers that store data. For example, you might want to store someone's age, name, or the result of a calculation. In programming, you can use variables to hold information that can be used later in the program.Example in Go (Golang):
goCopy codepackage main import "fmt" func main() { var name string = "Alice" var age int = 30 fmt.Println("Name:", name) fmt.Println("Age:", age) }
Control Structures
Control structures allow your program to make decisions and run certain code under specific conditions. The most common areif
statements, which help you perform tasks only when certain conditions are met.Example in Go (Golang):
goCopy codepackage main import "fmt" func main() { age := 18 if age >= 18 { fmt.Println("You are eligible to vote.") } else { fmt.Println("You are not eligible to vote yet.") } }
Loops
Loops are used when you want to repeat a block of code multiple times. This is useful when performing repetitive tasks, like iterating through a list of items.Example in Go (Golang):
goCopy codepackage main import "fmt" func main() { for i := 1; i <= 5; i++ { fmt.Println("Number:", i) } }
Functions
Functions help break your code into reusable pieces. Each function can take inputs (called parameters) and return an output. Think of them like mini-programs that do a specific job.Example in Go (Golang):
goCopy codepackage main import "fmt" func addNumbers(a int, b int) int { return a + b } func main() { result := addNumbers(5, 10) fmt.Println("The sum is:", result) }
Why Learn Programming?
Automation
One of the biggest advantages of programming is that you can automate repetitive tasks. Whether it's processing data, sending emails, or generating reports, programming allows you to perform these tasks with minimal human intervention.Problem Solving
Programming teaches you how to break down complex problems into smaller, manageable parts. You learn to approach problems logically, which is a valuable skill both in and outside the tech world.Career Opportunities
The demand for skilled programmers continues to grow across industries. From finance and healthcare to entertainment and social media, companies rely on technology and programmers to innovate and improve their services.
Practical Applications in Everyday Life
Task Automation
Let’s say you often need to rename files on your computer. Instead of manually renaming them one by one, you can write a small program that automates this task for you. This saves time and reduces human error.Home Automation
Many people use programming to create smart home systems. With a few lines of code, you can control your lights, thermostat, and security systems, all from your phone or computer.Personal Finances
You can write a program to track your expenses, calculate your savings goals, or even create alerts when your spending exceeds a certain threshold. Programming gives you the power to manage your data in ways that suit your needs.
Conclusion
Programming is not just for tech professionals—anyone can learn the basics and apply them in meaningful ways. Whether you’re interested in automating tasks, developing apps, or just exploring new problem-solving skills, learning to program can open up new doors. Starting with simple concepts like variables, control structures, and functions in a language like Go (Golang), you can begin your journey into the world of programming.
Useful Links
Here are some great resources to help you on your programming journey:
Exercism– Practice coding and get mentorship in various programming languages.
Useful Links
Here are some excellent resources to help you sharpen your programming skills:
Exercism – Real-world projects and mentorship with feedback on clean, idiomatic code. Supports multiple languages and offers a command-line interface for practice.
LeetCode – Over 2000+ coding challenges focused on Data Structures and Algorithms. Ideal for honing coding skills and preparing for technical interviews.
HackerRank – A platform that offers coding challenges and competitions on a variety of topics, including algorithms, AI, and databases. Great for coding competitions and interview prep.
GeeksforGeeks – A well-known platform that provides coding challenges, tutorials, and competitions focused on data structures, algorithms, and programming languages.
Codewars – A gamified coding platform where users solve community-driven challenges (katas). A fun way to improve your coding skills while learning from others.
Project Euler – A collection of challenging math-based coding problems. Ideal for improving problem-solving skills with programming techniques.
Codecademy – Interactive coding courses in various programming languages with hands-on projects and quizzes for immediate feedback. Offers a Pro plan with personalized learning paths.
TopCoder – A platform for coding challenges and competitions with topics ranging from algorithms to machine learning. Participate in real-world projects and connect with a global community.
CodeSignal – A comprehensive platform for coding challenges, interview preparation, and assessments. Track your progress and practice in real-time coding environments.
Edabit – Offers coding challenges for various skill levels, from beginner to advanced. Gamified learning keeps users engaged with points, badges, and rankings.
Subscribe to my newsletter
Read articles from Gift Ayodele directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
